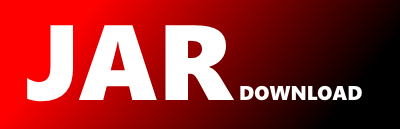
com.prowidesoftware.swift.model.mx.dic.LineItemDetails4 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Goods or services that are part of a commercial trade agreement.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "LineItemDetails4", propOrder = {
"lineItmId",
"qty",
"qtyTlrnce",
"unitPric",
"pricTlrnce",
"pdctNm",
"pdctIdr",
"pdctChrtcs",
"pdctCtgy",
"pdctOrgn",
"latstShipmntDt",
"rtgSummry",
"incotrms",
"adjstmnt",
"frghtChrgs",
"tax",
"ttlAmt"
})
public class LineItemDetails4 {
@XmlElement(name = "LineItmId", required = true)
protected String lineItmId;
@XmlElement(name = "Qty", required = true)
protected Quantity2 qty;
@XmlElement(name = "QtyTlrnce")
protected PercentageTolerance1 qtyTlrnce;
@XmlElement(name = "UnitPric")
protected UnitPrice8 unitPric;
@XmlElement(name = "PricTlrnce")
protected PercentageTolerance1 pricTlrnce;
@XmlElement(name = "PdctNm")
protected String pdctNm;
@XmlElement(name = "PdctIdr")
protected List pdctIdr;
@XmlElement(name = "PdctChrtcs")
protected List pdctChrtcs;
@XmlElement(name = "PdctCtgy")
protected List pdctCtgy;
@XmlElement(name = "PdctOrgn")
protected String pdctOrgn;
@XmlElement(name = "LatstShipmntDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate latstShipmntDt;
@XmlElement(name = "RtgSummry")
protected TransportMeans1Choice rtgSummry;
@XmlElement(name = "Incotrms")
protected List incotrms;
@XmlElement(name = "Adjstmnt")
protected List adjstmnt;
@XmlElement(name = "FrghtChrgs")
protected Charge12 frghtChrgs;
@XmlElement(name = "Tax")
protected List tax;
@XmlElement(name = "TtlAmt", required = true)
protected CurrencyAndAmount ttlAmt;
/**
* Gets the value of the lineItmId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLineItmId() {
return lineItmId;
}
/**
* Sets the value of the lineItmId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LineItemDetails4 setLineItmId(String value) {
this.lineItmId = value;
return this;
}
/**
* Gets the value of the qty property.
*
* @return
* possible object is
* {@link Quantity2 }
*
*/
public Quantity2 getQty() {
return qty;
}
/**
* Sets the value of the qty property.
*
* @param value
* allowed object is
* {@link Quantity2 }
*
*/
public LineItemDetails4 setQty(Quantity2 value) {
this.qty = value;
return this;
}
/**
* Gets the value of the qtyTlrnce property.
*
* @return
* possible object is
* {@link PercentageTolerance1 }
*
*/
public PercentageTolerance1 getQtyTlrnce() {
return qtyTlrnce;
}
/**
* Sets the value of the qtyTlrnce property.
*
* @param value
* allowed object is
* {@link PercentageTolerance1 }
*
*/
public LineItemDetails4 setQtyTlrnce(PercentageTolerance1 value) {
this.qtyTlrnce = value;
return this;
}
/**
* Gets the value of the unitPric property.
*
* @return
* possible object is
* {@link UnitPrice8 }
*
*/
public UnitPrice8 getUnitPric() {
return unitPric;
}
/**
* Sets the value of the unitPric property.
*
* @param value
* allowed object is
* {@link UnitPrice8 }
*
*/
public LineItemDetails4 setUnitPric(UnitPrice8 value) {
this.unitPric = value;
return this;
}
/**
* Gets the value of the pricTlrnce property.
*
* @return
* possible object is
* {@link PercentageTolerance1 }
*
*/
public PercentageTolerance1 getPricTlrnce() {
return pricTlrnce;
}
/**
* Sets the value of the pricTlrnce property.
*
* @param value
* allowed object is
* {@link PercentageTolerance1 }
*
*/
public LineItemDetails4 setPricTlrnce(PercentageTolerance1 value) {
this.pricTlrnce = value;
return this;
}
/**
* Gets the value of the pdctNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPdctNm() {
return pdctNm;
}
/**
* Sets the value of the pdctNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LineItemDetails4 setPdctNm(String value) {
this.pdctNm = value;
return this;
}
/**
* Gets the value of the pdctIdr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pdctIdr property.
*
*
* For example, to add a new item, do as follows:
*
* getPdctIdr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProductIdentifier2Choice }
*
*
* @return
* The value of the pdctIdr property.
*/
public List getPdctIdr() {
if (pdctIdr == null) {
pdctIdr = new ArrayList<>();
}
return this.pdctIdr;
}
/**
* Gets the value of the pdctChrtcs property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pdctChrtcs property.
*
*
* For example, to add a new item, do as follows:
*
* getPdctChrtcs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProductCharacteristics1Choice }
*
*
* @return
* The value of the pdctChrtcs property.
*/
public List getPdctChrtcs() {
if (pdctChrtcs == null) {
pdctChrtcs = new ArrayList<>();
}
return this.pdctChrtcs;
}
/**
* Gets the value of the pdctCtgy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pdctCtgy property.
*
*
* For example, to add a new item, do as follows:
*
* getPdctCtgy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ProductCategory1Choice }
*
*
* @return
* The value of the pdctCtgy property.
*/
public List getPdctCtgy() {
if (pdctCtgy == null) {
pdctCtgy = new ArrayList<>();
}
return this.pdctCtgy;
}
/**
* Gets the value of the pdctOrgn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPdctOrgn() {
return pdctOrgn;
}
/**
* Sets the value of the pdctOrgn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LineItemDetails4 setPdctOrgn(String value) {
this.pdctOrgn = value;
return this;
}
/**
* Gets the value of the latstShipmntDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getLatstShipmntDt() {
return latstShipmntDt;
}
/**
* Sets the value of the latstShipmntDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LineItemDetails4 setLatstShipmntDt(LocalDate value) {
this.latstShipmntDt = value;
return this;
}
/**
* Gets the value of the rtgSummry property.
*
* @return
* possible object is
* {@link TransportMeans1Choice }
*
*/
public TransportMeans1Choice getRtgSummry() {
return rtgSummry;
}
/**
* Sets the value of the rtgSummry property.
*
* @param value
* allowed object is
* {@link TransportMeans1Choice }
*
*/
public LineItemDetails4 setRtgSummry(TransportMeans1Choice value) {
this.rtgSummry = value;
return this;
}
/**
* Gets the value of the incotrms property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the incotrms property.
*
*
* For example, to add a new item, do as follows:
*
* getIncotrms().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Incoterms1 }
*
*
* @return
* The value of the incotrms property.
*/
public List getIncotrms() {
if (incotrms == null) {
incotrms = new ArrayList<>();
}
return this.incotrms;
}
/**
* Gets the value of the adjstmnt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the adjstmnt property.
*
*
* For example, to add a new item, do as follows:
*
* getAdjstmnt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Adjustment3 }
*
*
* @return
* The value of the adjstmnt property.
*/
public List getAdjstmnt() {
if (adjstmnt == null) {
adjstmnt = new ArrayList<>();
}
return this.adjstmnt;
}
/**
* Gets the value of the frghtChrgs property.
*
* @return
* possible object is
* {@link Charge12 }
*
*/
public Charge12 getFrghtChrgs() {
return frghtChrgs;
}
/**
* Sets the value of the frghtChrgs property.
*
* @param value
* allowed object is
* {@link Charge12 }
*
*/
public LineItemDetails4 setFrghtChrgs(Charge12 value) {
this.frghtChrgs = value;
return this;
}
/**
* Gets the value of the tax property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tax property.
*
*
* For example, to add a new item, do as follows:
*
* getTax().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Tax13 }
*
*
* @return
* The value of the tax property.
*/
public List getTax() {
if (tax == null) {
tax = new ArrayList<>();
}
return this.tax;
}
/**
* Gets the value of the ttlAmt property.
*
* @return
* possible object is
* {@link CurrencyAndAmount }
*
*/
public CurrencyAndAmount getTtlAmt() {
return ttlAmt;
}
/**
* Sets the value of the ttlAmt property.
*
* @param value
* allowed object is
* {@link CurrencyAndAmount }
*
*/
public LineItemDetails4 setTtlAmt(CurrencyAndAmount value) {
this.ttlAmt = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the pdctIdr list.
* @see #getPdctIdr()
*
*/
public LineItemDetails4 addPdctIdr(ProductIdentifier2Choice pdctIdr) {
getPdctIdr().add(pdctIdr);
return this;
}
/**
* Adds a new item to the pdctChrtcs list.
* @see #getPdctChrtcs()
*
*/
public LineItemDetails4 addPdctChrtcs(ProductCharacteristics1Choice pdctChrtcs) {
getPdctChrtcs().add(pdctChrtcs);
return this;
}
/**
* Adds a new item to the pdctCtgy list.
* @see #getPdctCtgy()
*
*/
public LineItemDetails4 addPdctCtgy(ProductCategory1Choice pdctCtgy) {
getPdctCtgy().add(pdctCtgy);
return this;
}
/**
* Adds a new item to the incotrms list.
* @see #getIncotrms()
*
*/
public LineItemDetails4 addIncotrms(Incoterms1 incotrms) {
getIncotrms().add(incotrms);
return this;
}
/**
* Adds a new item to the adjstmnt list.
* @see #getAdjstmnt()
*
*/
public LineItemDetails4 addAdjstmnt(Adjustment3 adjstmnt) {
getAdjstmnt().add(adjstmnt);
return this;
}
/**
* Adds a new item to the tax list.
* @see #getTax()
*
*/
public LineItemDetails4 addTax(Tax13 tax) {
getTax().add(tax);
return this;
}
}