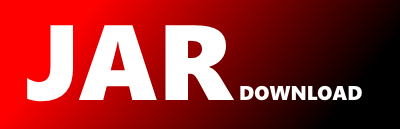
com.prowidesoftware.swift.model.mx.dic.LoanData143 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Specifies the loan data details in case of a repurchase trade transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "LoanData143", propOrder = {
"unqTradIdr",
"evtDt",
"exctnDtTm",
"clrSts",
"tradgVn",
"mstrAgrmt",
"valDt",
"gnlColl",
"dlvryByVal",
"collDlvryMtd",
"term",
"intrstRate",
"prncplAmt",
"termntnDt",
"unitPric"
})
public class LoanData143 {
@XmlElement(name = "UnqTradIdr", required = true)
protected String unqTradIdr;
@XmlElement(name = "EvtDt", required = true, type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate evtDt;
@XmlElement(name = "ExctnDtTm", required = true, type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime exctnDtTm;
@XmlElement(name = "ClrSts", required = true)
protected Cleared16Choice clrSts;
@XmlElement(name = "TradgVn", required = true)
protected String tradgVn;
@XmlElement(name = "MstrAgrmt")
protected MasterAgreement7 mstrAgrmt;
@XmlElement(name = "ValDt", required = true, type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate valDt;
@XmlElement(name = "GnlColl")
@XmlSchemaType(name = "string")
protected SpecialCollateral1Code gnlColl;
@XmlElement(name = "DlvryByVal")
protected boolean dlvryByVal;
@XmlElement(name = "CollDlvryMtd", required = true)
@XmlSchemaType(name = "string")
protected CollateralDeliveryMethod1Code collDlvryMtd;
@XmlElement(name = "Term")
protected List term;
@XmlElement(name = "IntrstRate")
protected InterestRate27Choice intrstRate;
@XmlElement(name = "PrncplAmt")
protected PrincipalAmount3 prncplAmt;
@XmlElement(name = "TermntnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate termntnDt;
@XmlElement(name = "UnitPric")
protected SecuritiesTransactionPrice19Choice unitPric;
/**
* Gets the value of the unqTradIdr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnqTradIdr() {
return unqTradIdr;
}
/**
* Sets the value of the unqTradIdr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData143 setUnqTradIdr(String value) {
this.unqTradIdr = value;
return this;
}
/**
* Gets the value of the evtDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getEvtDt() {
return evtDt;
}
/**
* Sets the value of the evtDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData143 setEvtDt(LocalDate value) {
this.evtDt = value;
return this;
}
/**
* Gets the value of the exctnDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getExctnDtTm() {
return exctnDtTm;
}
/**
* Sets the value of the exctnDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData143 setExctnDtTm(OffsetDateTime value) {
this.exctnDtTm = value;
return this;
}
/**
* Gets the value of the clrSts property.
*
* @return
* possible object is
* {@link Cleared16Choice }
*
*/
public Cleared16Choice getClrSts() {
return clrSts;
}
/**
* Sets the value of the clrSts property.
*
* @param value
* allowed object is
* {@link Cleared16Choice }
*
*/
public LoanData143 setClrSts(Cleared16Choice value) {
this.clrSts = value;
return this;
}
/**
* Gets the value of the tradgVn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTradgVn() {
return tradgVn;
}
/**
* Sets the value of the tradgVn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData143 setTradgVn(String value) {
this.tradgVn = value;
return this;
}
/**
* Gets the value of the mstrAgrmt property.
*
* @return
* possible object is
* {@link MasterAgreement7 }
*
*/
public MasterAgreement7 getMstrAgrmt() {
return mstrAgrmt;
}
/**
* Sets the value of the mstrAgrmt property.
*
* @param value
* allowed object is
* {@link MasterAgreement7 }
*
*/
public LoanData143 setMstrAgrmt(MasterAgreement7 value) {
this.mstrAgrmt = value;
return this;
}
/**
* Gets the value of the valDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getValDt() {
return valDt;
}
/**
* Sets the value of the valDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData143 setValDt(LocalDate value) {
this.valDt = value;
return this;
}
/**
* Gets the value of the gnlColl property.
*
* @return
* possible object is
* {@link SpecialCollateral1Code }
*
*/
public SpecialCollateral1Code getGnlColl() {
return gnlColl;
}
/**
* Sets the value of the gnlColl property.
*
* @param value
* allowed object is
* {@link SpecialCollateral1Code }
*
*/
public LoanData143 setGnlColl(SpecialCollateral1Code value) {
this.gnlColl = value;
return this;
}
/**
* Gets the value of the dlvryByVal property.
*
*/
public boolean isDlvryByVal() {
return dlvryByVal;
}
/**
* Sets the value of the dlvryByVal property.
*
*/
public LoanData143 setDlvryByVal(boolean value) {
this.dlvryByVal = value;
return this;
}
/**
* Gets the value of the collDlvryMtd property.
*
* @return
* possible object is
* {@link CollateralDeliveryMethod1Code }
*
*/
public CollateralDeliveryMethod1Code getCollDlvryMtd() {
return collDlvryMtd;
}
/**
* Sets the value of the collDlvryMtd property.
*
* @param value
* allowed object is
* {@link CollateralDeliveryMethod1Code }
*
*/
public LoanData143 setCollDlvryMtd(CollateralDeliveryMethod1Code value) {
this.collDlvryMtd = value;
return this;
}
/**
* Gets the value of the term property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the term property.
*
*
* For example, to add a new item, do as follows:
*
* getTerm().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ContractTerm7Choice }
*
*
* @return
* The value of the term property.
*/
public List getTerm() {
if (term == null) {
term = new ArrayList<>();
}
return this.term;
}
/**
* Gets the value of the intrstRate property.
*
* @return
* possible object is
* {@link InterestRate27Choice }
*
*/
public InterestRate27Choice getIntrstRate() {
return intrstRate;
}
/**
* Sets the value of the intrstRate property.
*
* @param value
* allowed object is
* {@link InterestRate27Choice }
*
*/
public LoanData143 setIntrstRate(InterestRate27Choice value) {
this.intrstRate = value;
return this;
}
/**
* Gets the value of the prncplAmt property.
*
* @return
* possible object is
* {@link PrincipalAmount3 }
*
*/
public PrincipalAmount3 getPrncplAmt() {
return prncplAmt;
}
/**
* Sets the value of the prncplAmt property.
*
* @param value
* allowed object is
* {@link PrincipalAmount3 }
*
*/
public LoanData143 setPrncplAmt(PrincipalAmount3 value) {
this.prncplAmt = value;
return this;
}
/**
* Gets the value of the termntnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getTermntnDt() {
return termntnDt;
}
/**
* Sets the value of the termntnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData143 setTermntnDt(LocalDate value) {
this.termntnDt = value;
return this;
}
/**
* Gets the value of the unitPric property.
*
* @return
* possible object is
* {@link SecuritiesTransactionPrice19Choice }
*
*/
public SecuritiesTransactionPrice19Choice getUnitPric() {
return unitPric;
}
/**
* Sets the value of the unitPric property.
*
* @param value
* allowed object is
* {@link SecuritiesTransactionPrice19Choice }
*
*/
public LoanData143 setUnitPric(SecuritiesTransactionPrice19Choice value) {
this.unitPric = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the term list.
* @see #getTerm()
*
*/
public LoanData143 addTerm(ContractTerm7Choice term) {
getTerm().add(term);
return this;
}
}