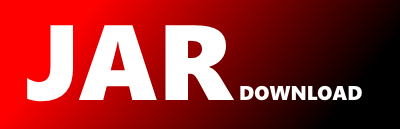
com.prowidesoftware.swift.model.mx.dic.LoanData57 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Specifies the loan data details in case of a buy sell back transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "LoanData57", propOrder = {
"unqTradIdr",
"evtDt",
"exctnDtTm",
"clrSts",
"tradgVn",
"mstrAgrmt",
"valDt",
"mtrtyDt",
"gnlColl",
"prncplAmt",
"unitPric",
"termntnDt"
})
public class LoanData57 {
@XmlElement(name = "UnqTradIdr")
protected String unqTradIdr;
@XmlElement(name = "EvtDt", required = true, type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate evtDt;
@XmlElement(name = "ExctnDtTm", type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime exctnDtTm;
@XmlElement(name = "ClrSts")
protected Cleared10Choice clrSts;
@XmlElement(name = "TradgVn")
protected String tradgVn;
@XmlElement(name = "MstrAgrmt")
protected MasterAgreement6 mstrAgrmt;
@XmlElement(name = "ValDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate valDt;
@XmlElement(name = "MtrtyDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate mtrtyDt;
@XmlElement(name = "GnlColl")
@XmlSchemaType(name = "string")
protected SpecialCollateral1Code gnlColl;
@XmlElement(name = "PrncplAmt")
protected PrincipalAmount2 prncplAmt;
@XmlElement(name = "UnitPric")
protected SecuritiesTransactionPrice11Choice unitPric;
@XmlElement(name = "TermntnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate termntnDt;
/**
* Gets the value of the unqTradIdr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnqTradIdr() {
return unqTradIdr;
}
/**
* Sets the value of the unqTradIdr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setUnqTradIdr(String value) {
this.unqTradIdr = value;
return this;
}
/**
* Gets the value of the evtDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getEvtDt() {
return evtDt;
}
/**
* Sets the value of the evtDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setEvtDt(LocalDate value) {
this.evtDt = value;
return this;
}
/**
* Gets the value of the exctnDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getExctnDtTm() {
return exctnDtTm;
}
/**
* Sets the value of the exctnDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setExctnDtTm(OffsetDateTime value) {
this.exctnDtTm = value;
return this;
}
/**
* Gets the value of the clrSts property.
*
* @return
* possible object is
* {@link Cleared10Choice }
*
*/
public Cleared10Choice getClrSts() {
return clrSts;
}
/**
* Sets the value of the clrSts property.
*
* @param value
* allowed object is
* {@link Cleared10Choice }
*
*/
public LoanData57 setClrSts(Cleared10Choice value) {
this.clrSts = value;
return this;
}
/**
* Gets the value of the tradgVn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTradgVn() {
return tradgVn;
}
/**
* Sets the value of the tradgVn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setTradgVn(String value) {
this.tradgVn = value;
return this;
}
/**
* Gets the value of the mstrAgrmt property.
*
* @return
* possible object is
* {@link MasterAgreement6 }
*
*/
public MasterAgreement6 getMstrAgrmt() {
return mstrAgrmt;
}
/**
* Sets the value of the mstrAgrmt property.
*
* @param value
* allowed object is
* {@link MasterAgreement6 }
*
*/
public LoanData57 setMstrAgrmt(MasterAgreement6 value) {
this.mstrAgrmt = value;
return this;
}
/**
* Gets the value of the valDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getValDt() {
return valDt;
}
/**
* Sets the value of the valDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setValDt(LocalDate value) {
this.valDt = value;
return this;
}
/**
* Gets the value of the mtrtyDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getMtrtyDt() {
return mtrtyDt;
}
/**
* Sets the value of the mtrtyDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setMtrtyDt(LocalDate value) {
this.mtrtyDt = value;
return this;
}
/**
* Gets the value of the gnlColl property.
*
* @return
* possible object is
* {@link SpecialCollateral1Code }
*
*/
public SpecialCollateral1Code getGnlColl() {
return gnlColl;
}
/**
* Sets the value of the gnlColl property.
*
* @param value
* allowed object is
* {@link SpecialCollateral1Code }
*
*/
public LoanData57 setGnlColl(SpecialCollateral1Code value) {
this.gnlColl = value;
return this;
}
/**
* Gets the value of the prncplAmt property.
*
* @return
* possible object is
* {@link PrincipalAmount2 }
*
*/
public PrincipalAmount2 getPrncplAmt() {
return prncplAmt;
}
/**
* Sets the value of the prncplAmt property.
*
* @param value
* allowed object is
* {@link PrincipalAmount2 }
*
*/
public LoanData57 setPrncplAmt(PrincipalAmount2 value) {
this.prncplAmt = value;
return this;
}
/**
* Gets the value of the unitPric property.
*
* @return
* possible object is
* {@link SecuritiesTransactionPrice11Choice }
*
*/
public SecuritiesTransactionPrice11Choice getUnitPric() {
return unitPric;
}
/**
* Sets the value of the unitPric property.
*
* @param value
* allowed object is
* {@link SecuritiesTransactionPrice11Choice }
*
*/
public LoanData57 setUnitPric(SecuritiesTransactionPrice11Choice value) {
this.unitPric = value;
return this;
}
/**
* Gets the value of the termntnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getTermntnDt() {
return termntnDt;
}
/**
* Sets the value of the termntnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public LoanData57 setTermntnDt(LocalDate value) {
this.termntnDt = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy