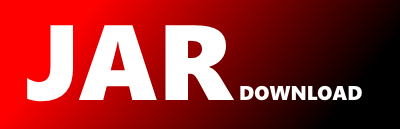
com.prowidesoftware.swift.model.mx.dic.MandateAcceptanceReportV02 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The MandateAcceptanceReport message is sent from the agent of the receiver (debtor or creditor) of the MandateRequest message (initiation, amendment or cancellation) to the agent of the initiator of the MandateRequest message (debtor or creditor).
* A MandateAcceptanceReport message is used to confirm the acceptance or rejection of a MandateRequest message. Where acceptance is part of the full process flow, a MandateRequest message only becomes valid after a confirmation of acceptance is received through a MandateAcceptanceReport message from the agent of the receiver.
* Usage
* The MandateAcceptanceReport message can contain only one confirmation of acceptance of rejection of one specific MandateRequest message.
* The messages can be exchanged between debtor agent and creditor agent and between debtor agent and debtor and creditor agent and creditor.
* The MandateAcceptanceReport message can be used in domestic and cross-border scenarios.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "MandateAcceptanceReportV02", propOrder = {
"grpHdr",
"undrlygAccptncDtls",
"splmtryData"
})
public class MandateAcceptanceReportV02 {
@XmlElement(name = "GrpHdr", required = true)
protected GroupHeader47 grpHdr;
@XmlElement(name = "UndrlygAccptncDtls", required = true)
protected MandateAcceptance2 undrlygAccptncDtls;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the grpHdr property.
*
* @return
* possible object is
* {@link GroupHeader47 }
*
*/
public GroupHeader47 getGrpHdr() {
return grpHdr;
}
/**
* Sets the value of the grpHdr property.
*
* @param value
* allowed object is
* {@link GroupHeader47 }
*
*/
public MandateAcceptanceReportV02 setGrpHdr(GroupHeader47 value) {
this.grpHdr = value;
return this;
}
/**
* Gets the value of the undrlygAccptncDtls property.
*
* @return
* possible object is
* {@link MandateAcceptance2 }
*
*/
public MandateAcceptance2 getUndrlygAccptncDtls() {
return undrlygAccptncDtls;
}
/**
* Sets the value of the undrlygAccptncDtls property.
*
* @param value
* allowed object is
* {@link MandateAcceptance2 }
*
*/
public MandateAcceptanceReportV02 setUndrlygAccptncDtls(MandateAcceptance2 value) {
this.undrlygAccptncDtls = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public MandateAcceptanceReportV02 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}