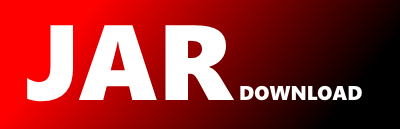
com.prowidesoftware.swift.model.mx.dic.MandateRelatedInformation6 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Set of elements used to provide further details related to a direct debit mandate signed between the creditor and the debtor.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "MandateRelatedInformation6", propOrder = {
"mndtId",
"dtOfSgntr",
"amdmntInd",
"amdmntInfDtls",
"elctrncSgntr",
"frstColltnDt",
"fnlColltnDt",
"frqcy"
})
public class MandateRelatedInformation6 {
@XmlElement(name = "MndtId")
protected String mndtId;
@XmlElement(name = "DtOfSgntr", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate dtOfSgntr;
@XmlElement(name = "AmdmntInd")
protected Boolean amdmntInd;
@XmlElement(name = "AmdmntInfDtls")
protected AmendmentInformationDetails6 amdmntInfDtls;
@XmlElement(name = "ElctrncSgntr")
protected String elctrncSgntr;
@XmlElement(name = "FrstColltnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate frstColltnDt;
@XmlElement(name = "FnlColltnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate fnlColltnDt;
@XmlElement(name = "Frqcy")
@XmlSchemaType(name = "string")
protected Frequency1Code frqcy;
/**
* Gets the value of the mndtId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMndtId() {
return mndtId;
}
/**
* Sets the value of the mndtId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public MandateRelatedInformation6 setMndtId(String value) {
this.mndtId = value;
return this;
}
/**
* Gets the value of the dtOfSgntr property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getDtOfSgntr() {
return dtOfSgntr;
}
/**
* Sets the value of the dtOfSgntr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public MandateRelatedInformation6 setDtOfSgntr(LocalDate value) {
this.dtOfSgntr = value;
return this;
}
/**
* Gets the value of the amdmntInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAmdmntInd() {
return amdmntInd;
}
/**
* Sets the value of the amdmntInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public MandateRelatedInformation6 setAmdmntInd(Boolean value) {
this.amdmntInd = value;
return this;
}
/**
* Gets the value of the amdmntInfDtls property.
*
* @return
* possible object is
* {@link AmendmentInformationDetails6 }
*
*/
public AmendmentInformationDetails6 getAmdmntInfDtls() {
return amdmntInfDtls;
}
/**
* Sets the value of the amdmntInfDtls property.
*
* @param value
* allowed object is
* {@link AmendmentInformationDetails6 }
*
*/
public MandateRelatedInformation6 setAmdmntInfDtls(AmendmentInformationDetails6 value) {
this.amdmntInfDtls = value;
return this;
}
/**
* Gets the value of the elctrncSgntr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getElctrncSgntr() {
return elctrncSgntr;
}
/**
* Sets the value of the elctrncSgntr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public MandateRelatedInformation6 setElctrncSgntr(String value) {
this.elctrncSgntr = value;
return this;
}
/**
* Gets the value of the frstColltnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getFrstColltnDt() {
return frstColltnDt;
}
/**
* Sets the value of the frstColltnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public MandateRelatedInformation6 setFrstColltnDt(LocalDate value) {
this.frstColltnDt = value;
return this;
}
/**
* Gets the value of the fnlColltnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getFnlColltnDt() {
return fnlColltnDt;
}
/**
* Sets the value of the fnlColltnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public MandateRelatedInformation6 setFnlColltnDt(LocalDate value) {
this.fnlColltnDt = value;
return this;
}
/**
* Gets the value of the frqcy property.
*
* @return
* possible object is
* {@link Frequency1Code }
*
*/
public Frequency1Code getFrqcy() {
return frqcy;
}
/**
* Sets the value of the frqcy property.
*
* @param value
* allowed object is
* {@link Frequency1Code }
*
*/
public MandateRelatedInformation6 setFrqcy(Frequency1Code value) {
this.frqcy = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy