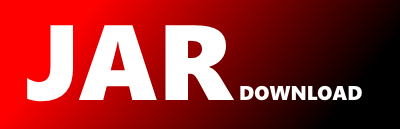
com.prowidesoftware.swift.model.mx.dic.MeetingInstructionV09 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The MeetingInstruction message is sent by a party holding the right to vote to an intermediary, the issuer or its agent to request the receiving party to act upon one or several instructions.
* Usage
* The MeetingInstruction message is used to vote, to require attendance to a meeting, to request registration of securities and assign a proxy. One instruction or multiple instructions can be carried within the same message.
* Once the message is sent, it cannot be modified. It must be cancelled by a MeetingInstructionCancellationRequest. Only after receipt of a confirmed cancelled status via the
* MeetingInstructionStatus message, a new MeetingInstruction message can be sent.
* This message definition is intended for use with the Business Application Header (BAH).
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "MeetingInstructionV09", propOrder = {
"pgntn",
"mtgInstrId",
"mtgRef",
"finInstrmId",
"instrCxlReqId",
"cancInstrId",
"othrDocId",
"instr",
"splmtryData"
})
public class MeetingInstructionV09 {
@XmlElement(name = "Pgntn")
protected Pagination1 pgntn;
@XmlElement(name = "MtgInstrId")
protected String mtgInstrId;
@XmlElement(name = "MtgRef", required = true)
protected MeetingReference10 mtgRef;
@XmlElement(name = "FinInstrmId", required = true)
protected SecurityIdentification19 finInstrmId;
@XmlElement(name = "InstrCxlReqId")
protected List instrCxlReqId;
@XmlElement(name = "CancInstrId")
protected List cancInstrId;
@XmlElement(name = "OthrDocId")
protected List othrDocId;
@XmlElement(name = "Instr", required = true)
protected List instr;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the pgntn property.
*
* @return
* possible object is
* {@link Pagination1 }
*
*/
public Pagination1 getPgntn() {
return pgntn;
}
/**
* Sets the value of the pgntn property.
*
* @param value
* allowed object is
* {@link Pagination1 }
*
*/
public MeetingInstructionV09 setPgntn(Pagination1 value) {
this.pgntn = value;
return this;
}
/**
* Gets the value of the mtgInstrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMtgInstrId() {
return mtgInstrId;
}
/**
* Sets the value of the mtgInstrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public MeetingInstructionV09 setMtgInstrId(String value) {
this.mtgInstrId = value;
return this;
}
/**
* Gets the value of the mtgRef property.
*
* @return
* possible object is
* {@link MeetingReference10 }
*
*/
public MeetingReference10 getMtgRef() {
return mtgRef;
}
/**
* Sets the value of the mtgRef property.
*
* @param value
* allowed object is
* {@link MeetingReference10 }
*
*/
public MeetingInstructionV09 setMtgRef(MeetingReference10 value) {
this.mtgRef = value;
return this;
}
/**
* Gets the value of the finInstrmId property.
*
* @return
* possible object is
* {@link SecurityIdentification19 }
*
*/
public SecurityIdentification19 getFinInstrmId() {
return finInstrmId;
}
/**
* Sets the value of the finInstrmId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification19 }
*
*/
public MeetingInstructionV09 setFinInstrmId(SecurityIdentification19 value) {
this.finInstrmId = value;
return this;
}
/**
* Gets the value of the instrCxlReqId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the instrCxlReqId property.
*
*
* For example, to add a new item, do as follows:
*
* getInstrCxlReqId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link MeetingInstructionCancellation1 }
*
*
* @return
* The value of the instrCxlReqId property.
*/
public List getInstrCxlReqId() {
if (instrCxlReqId == null) {
instrCxlReqId = new ArrayList<>();
}
return this.instrCxlReqId;
}
/**
* Gets the value of the cancInstrId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cancInstrId property.
*
*
* For example, to add a new item, do as follows:
*
* getCancInstrId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link MeetingInstructionIdentification1 }
*
*
* @return
* The value of the cancInstrId property.
*/
public List getCancInstrId() {
if (cancInstrId == null) {
cancInstrId = new ArrayList<>();
}
return this.cancInstrId;
}
/**
* Gets the value of the othrDocId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrDocId property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrDocId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DocumentIdentification32 }
*
*
* @return
* The value of the othrDocId property.
*/
public List getOthrDocId() {
if (othrDocId == null) {
othrDocId = new ArrayList<>();
}
return this.othrDocId;
}
/**
* Gets the value of the instr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the instr property.
*
*
* For example, to add a new item, do as follows:
*
* getInstr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Instruction7 }
*
*
* @return
* The value of the instr property.
*/
public List getInstr() {
if (instr == null) {
instr = new ArrayList<>();
}
return this.instr;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the instrCxlReqId list.
* @see #getInstrCxlReqId()
*
*/
public MeetingInstructionV09 addInstrCxlReqId(MeetingInstructionCancellation1 instrCxlReqId) {
getInstrCxlReqId().add(instrCxlReqId);
return this;
}
/**
* Adds a new item to the cancInstrId list.
* @see #getCancInstrId()
*
*/
public MeetingInstructionV09 addCancInstrId(MeetingInstructionIdentification1 cancInstrId) {
getCancInstrId().add(cancInstrId);
return this;
}
/**
* Adds a new item to the othrDocId list.
* @see #getOthrDocId()
*
*/
public MeetingInstructionV09 addOthrDocId(DocumentIdentification32 othrDocId) {
getOthrDocId().add(othrDocId);
return this;
}
/**
* Adds a new item to the instr list.
* @see #getInstr()
*
*/
public MeetingInstructionV09 addInstr(Instruction7 instr) {
getInstr().add(instr);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public MeetingInstructionV09 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}