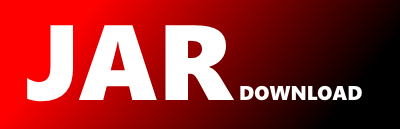
com.prowidesoftware.swift.model.mx.dic.MeetingNotificationV10 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The MeetingNotification message is sent by a notifying party, for example, an issuer, its agent or an intermediary to another intermediary, a party holding the right to vote, a registered security holder or to a beneficial holder to announce a meeting.
* Usage
* The MeetingNotification message is used to announce a meeting, for example, it provides information on the participation details and requirements for the meeting, the vote parameters and the resolutions. The MeetingNotification message may also be used to announce an update.
* This message definition is intended for use with the Business Application Header (BAH).
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "MeetingNotificationV10", propOrder = {
"pgntn",
"ntfctnGnlInf",
"ntfctnUpd",
"evtsLkg",
"mtg",
"mtgDtls",
"issr",
"issrAgt",
"scty",
"rsltn",
"vote",
"pwrOfAttnyRqrmnts",
"addtlInf",
"splmtryData"
})
public class MeetingNotificationV10 {
@XmlElement(name = "Pgntn")
protected Pagination1 pgntn;
@XmlElement(name = "NtfctnGnlInf", required = true)
protected NotificationGeneralInformation4 ntfctnGnlInf;
@XmlElement(name = "NtfctnUpd")
protected NotificationUpdate2 ntfctnUpd;
@XmlElement(name = "EvtsLkg")
protected List evtsLkg;
@XmlElement(name = "Mtg", required = true)
protected MeetingNotice8 mtg;
@XmlElement(name = "MtgDtls", required = true)
protected List mtgDtls;
@XmlElement(name = "Issr", required = true)
protected IssuerInformation3 issr;
@XmlElement(name = "IssrAgt")
protected List issrAgt;
@XmlElement(name = "Scty", required = true)
protected List scty;
@XmlElement(name = "Rsltn")
protected List rsltn;
@XmlElement(name = "Vote")
protected VoteParameters8 vote;
@XmlElement(name = "PwrOfAttnyRqrmnts")
protected PowerOfAttorneyRequirements4 pwrOfAttnyRqrmnts;
@XmlElement(name = "AddtlInf")
protected CorporateEventNarrative4 addtlInf;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the pgntn property.
*
* @return
* possible object is
* {@link Pagination1 }
*
*/
public Pagination1 getPgntn() {
return pgntn;
}
/**
* Sets the value of the pgntn property.
*
* @param value
* allowed object is
* {@link Pagination1 }
*
*/
public MeetingNotificationV10 setPgntn(Pagination1 value) {
this.pgntn = value;
return this;
}
/**
* Gets the value of the ntfctnGnlInf property.
*
* @return
* possible object is
* {@link NotificationGeneralInformation4 }
*
*/
public NotificationGeneralInformation4 getNtfctnGnlInf() {
return ntfctnGnlInf;
}
/**
* Sets the value of the ntfctnGnlInf property.
*
* @param value
* allowed object is
* {@link NotificationGeneralInformation4 }
*
*/
public MeetingNotificationV10 setNtfctnGnlInf(NotificationGeneralInformation4 value) {
this.ntfctnGnlInf = value;
return this;
}
/**
* Gets the value of the ntfctnUpd property.
*
* @return
* possible object is
* {@link NotificationUpdate2 }
*
*/
public NotificationUpdate2 getNtfctnUpd() {
return ntfctnUpd;
}
/**
* Sets the value of the ntfctnUpd property.
*
* @param value
* allowed object is
* {@link NotificationUpdate2 }
*
*/
public MeetingNotificationV10 setNtfctnUpd(NotificationUpdate2 value) {
this.ntfctnUpd = value;
return this;
}
/**
* Gets the value of the evtsLkg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the evtsLkg property.
*
*
* For example, to add a new item, do as follows:
*
* getEvtsLkg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link MeetingEventReference1 }
*
*
* @return
* The value of the evtsLkg property.
*/
public List getEvtsLkg() {
if (evtsLkg == null) {
evtsLkg = new ArrayList<>();
}
return this.evtsLkg;
}
/**
* Gets the value of the mtg property.
*
* @return
* possible object is
* {@link MeetingNotice8 }
*
*/
public MeetingNotice8 getMtg() {
return mtg;
}
/**
* Sets the value of the mtg property.
*
* @param value
* allowed object is
* {@link MeetingNotice8 }
*
*/
public MeetingNotificationV10 setMtg(MeetingNotice8 value) {
this.mtg = value;
return this;
}
/**
* Gets the value of the mtgDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the mtgDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getMtgDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Meeting6 }
*
*
* @return
* The value of the mtgDtls property.
*/
public List getMtgDtls() {
if (mtgDtls == null) {
mtgDtls = new ArrayList<>();
}
return this.mtgDtls;
}
/**
* Gets the value of the issr property.
*
* @return
* possible object is
* {@link IssuerInformation3 }
*
*/
public IssuerInformation3 getIssr() {
return issr;
}
/**
* Sets the value of the issr property.
*
* @param value
* allowed object is
* {@link IssuerInformation3 }
*
*/
public MeetingNotificationV10 setIssr(IssuerInformation3 value) {
this.issr = value;
return this;
}
/**
* Gets the value of the issrAgt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the issrAgt property.
*
*
* For example, to add a new item, do as follows:
*
* getIssrAgt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IssuerAgent3 }
*
*
* @return
* The value of the issrAgt property.
*/
public List getIssrAgt() {
if (issrAgt == null) {
issrAgt = new ArrayList<>();
}
return this.issrAgt;
}
/**
* Gets the value of the scty property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the scty property.
*
*
* For example, to add a new item, do as follows:
*
* getScty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SecurityPosition16 }
*
*
* @return
* The value of the scty property.
*/
public List getScty() {
if (scty == null) {
scty = new ArrayList<>();
}
return this.scty;
}
/**
* Gets the value of the rsltn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rsltn property.
*
*
* For example, to add a new item, do as follows:
*
* getRsltn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Resolution6 }
*
*
* @return
* The value of the rsltn property.
*/
public List getRsltn() {
if (rsltn == null) {
rsltn = new ArrayList<>();
}
return this.rsltn;
}
/**
* Gets the value of the vote property.
*
* @return
* possible object is
* {@link VoteParameters8 }
*
*/
public VoteParameters8 getVote() {
return vote;
}
/**
* Sets the value of the vote property.
*
* @param value
* allowed object is
* {@link VoteParameters8 }
*
*/
public MeetingNotificationV10 setVote(VoteParameters8 value) {
this.vote = value;
return this;
}
/**
* Gets the value of the pwrOfAttnyRqrmnts property.
*
* @return
* possible object is
* {@link PowerOfAttorneyRequirements4 }
*
*/
public PowerOfAttorneyRequirements4 getPwrOfAttnyRqrmnts() {
return pwrOfAttnyRqrmnts;
}
/**
* Sets the value of the pwrOfAttnyRqrmnts property.
*
* @param value
* allowed object is
* {@link PowerOfAttorneyRequirements4 }
*
*/
public MeetingNotificationV10 setPwrOfAttnyRqrmnts(PowerOfAttorneyRequirements4 value) {
this.pwrOfAttnyRqrmnts = value;
return this;
}
/**
* Gets the value of the addtlInf property.
*
* @return
* possible object is
* {@link CorporateEventNarrative4 }
*
*/
public CorporateEventNarrative4 getAddtlInf() {
return addtlInf;
}
/**
* Sets the value of the addtlInf property.
*
* @param value
* allowed object is
* {@link CorporateEventNarrative4 }
*
*/
public MeetingNotificationV10 setAddtlInf(CorporateEventNarrative4 value) {
this.addtlInf = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the evtsLkg list.
* @see #getEvtsLkg()
*
*/
public MeetingNotificationV10 addEvtsLkg(MeetingEventReference1 evtsLkg) {
getEvtsLkg().add(evtsLkg);
return this;
}
/**
* Adds a new item to the mtgDtls list.
* @see #getMtgDtls()
*
*/
public MeetingNotificationV10 addMtgDtls(Meeting6 mtgDtls) {
getMtgDtls().add(mtgDtls);
return this;
}
/**
* Adds a new item to the issrAgt list.
* @see #getIssrAgt()
*
*/
public MeetingNotificationV10 addIssrAgt(IssuerAgent3 issrAgt) {
getIssrAgt().add(issrAgt);
return this;
}
/**
* Adds a new item to the scty list.
* @see #getScty()
*
*/
public MeetingNotificationV10 addScty(SecurityPosition16 scty) {
getScty().add(scty);
return this;
}
/**
* Adds a new item to the rsltn list.
* @see #getRsltn()
*
*/
public MeetingNotificationV10 addRsltn(Resolution6 rsltn) {
getRsltn().add(rsltn);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public MeetingNotificationV10 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}