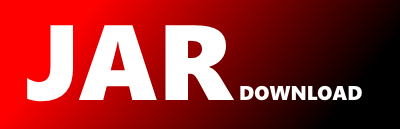
com.prowidesoftware.swift.model.mx.dic.PaymentCard32 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Payment card performing the transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PaymentCard32", propOrder = {
"prtctdCardData",
"prvtCardData",
"plainCardData",
"pmtAcctRef",
"mskdPAN",
"issrBIN",
"cardCtryCd",
"cardCcyCd",
"cardPdctPrfl",
"cardBrnd",
"cardPdctTp",
"cardPdctSubTp",
"intrnlCard",
"allwdPdct",
"svcOptn",
"addtlCardData"
})
public class PaymentCard32 {
@XmlElement(name = "PrtctdCardData")
protected ContentInformationType32 prtctdCardData;
@XmlElement(name = "PrvtCardData")
protected byte[] prvtCardData;
@XmlElement(name = "PlainCardData")
protected PlainCardData15 plainCardData;
@XmlElement(name = "PmtAcctRef")
protected String pmtAcctRef;
@XmlElement(name = "MskdPAN")
protected String mskdPAN;
@XmlElement(name = "IssrBIN")
protected String issrBIN;
@XmlElement(name = "CardCtryCd")
protected String cardCtryCd;
@XmlElement(name = "CardCcyCd")
protected String cardCcyCd;
@XmlElement(name = "CardPdctPrfl")
protected String cardPdctPrfl;
@XmlElement(name = "CardBrnd")
protected String cardBrnd;
@XmlElement(name = "CardPdctTp")
@XmlSchemaType(name = "string")
protected CardProductType1Code cardPdctTp;
@XmlElement(name = "CardPdctSubTp")
protected String cardPdctSubTp;
@XmlElement(name = "IntrnlCard")
protected Boolean intrnlCard;
@XmlElement(name = "AllwdPdct")
protected List allwdPdct;
@XmlElement(name = "SvcOptn")
protected String svcOptn;
@XmlElement(name = "AddtlCardData")
protected String addtlCardData;
/**
* Gets the value of the prtctdCardData property.
*
* @return
* possible object is
* {@link ContentInformationType32 }
*
*/
public ContentInformationType32 getPrtctdCardData() {
return prtctdCardData;
}
/**
* Sets the value of the prtctdCardData property.
*
* @param value
* allowed object is
* {@link ContentInformationType32 }
*
*/
public PaymentCard32 setPrtctdCardData(ContentInformationType32 value) {
this.prtctdCardData = value;
return this;
}
/**
* Gets the value of the prvtCardData property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getPrvtCardData() {
return prvtCardData;
}
/**
* Sets the value of the prvtCardData property.
*
* @param value
* allowed object is
* byte[]
*/
public PaymentCard32 setPrvtCardData(byte[] value) {
this.prvtCardData = value;
return this;
}
/**
* Gets the value of the plainCardData property.
*
* @return
* possible object is
* {@link PlainCardData15 }
*
*/
public PlainCardData15 getPlainCardData() {
return plainCardData;
}
/**
* Sets the value of the plainCardData property.
*
* @param value
* allowed object is
* {@link PlainCardData15 }
*
*/
public PaymentCard32 setPlainCardData(PlainCardData15 value) {
this.plainCardData = value;
return this;
}
/**
* Gets the value of the pmtAcctRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPmtAcctRef() {
return pmtAcctRef;
}
/**
* Sets the value of the pmtAcctRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setPmtAcctRef(String value) {
this.pmtAcctRef = value;
return this;
}
/**
* Gets the value of the mskdPAN property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMskdPAN() {
return mskdPAN;
}
/**
* Sets the value of the mskdPAN property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setMskdPAN(String value) {
this.mskdPAN = value;
return this;
}
/**
* Gets the value of the issrBIN property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIssrBIN() {
return issrBIN;
}
/**
* Sets the value of the issrBIN property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setIssrBIN(String value) {
this.issrBIN = value;
return this;
}
/**
* Gets the value of the cardCtryCd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardCtryCd() {
return cardCtryCd;
}
/**
* Sets the value of the cardCtryCd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setCardCtryCd(String value) {
this.cardCtryCd = value;
return this;
}
/**
* Gets the value of the cardCcyCd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardCcyCd() {
return cardCcyCd;
}
/**
* Sets the value of the cardCcyCd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setCardCcyCd(String value) {
this.cardCcyCd = value;
return this;
}
/**
* Gets the value of the cardPdctPrfl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardPdctPrfl() {
return cardPdctPrfl;
}
/**
* Sets the value of the cardPdctPrfl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setCardPdctPrfl(String value) {
this.cardPdctPrfl = value;
return this;
}
/**
* Gets the value of the cardBrnd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardBrnd() {
return cardBrnd;
}
/**
* Sets the value of the cardBrnd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setCardBrnd(String value) {
this.cardBrnd = value;
return this;
}
/**
* Gets the value of the cardPdctTp property.
*
* @return
* possible object is
* {@link CardProductType1Code }
*
*/
public CardProductType1Code getCardPdctTp() {
return cardPdctTp;
}
/**
* Sets the value of the cardPdctTp property.
*
* @param value
* allowed object is
* {@link CardProductType1Code }
*
*/
public PaymentCard32 setCardPdctTp(CardProductType1Code value) {
this.cardPdctTp = value;
return this;
}
/**
* Gets the value of the cardPdctSubTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardPdctSubTp() {
return cardPdctSubTp;
}
/**
* Sets the value of the cardPdctSubTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setCardPdctSubTp(String value) {
this.cardPdctSubTp = value;
return this;
}
/**
* Gets the value of the intrnlCard property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIntrnlCard() {
return intrnlCard;
}
/**
* Sets the value of the intrnlCard property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public PaymentCard32 setIntrnlCard(Boolean value) {
this.intrnlCard = value;
return this;
}
/**
* Gets the value of the allwdPdct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the allwdPdct property.
*
*
* For example, to add a new item, do as follows:
*
* getAllwdPdct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the allwdPdct property.
*/
public List getAllwdPdct() {
if (allwdPdct == null) {
allwdPdct = new ArrayList<>();
}
return this.allwdPdct;
}
/**
* Gets the value of the svcOptn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSvcOptn() {
return svcOptn;
}
/**
* Sets the value of the svcOptn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setSvcOptn(String value) {
this.svcOptn = value;
return this;
}
/**
* Gets the value of the addtlCardData property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAddtlCardData() {
return addtlCardData;
}
/**
* Sets the value of the addtlCardData property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentCard32 setAddtlCardData(String value) {
this.addtlCardData = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the allwdPdct list.
* @see #getAllwdPdct()
*
*/
public PaymentCard32 addAllwdPdct(String allwdPdct) {
getAllwdPdct().add(allwdPdct);
return this;
}
}