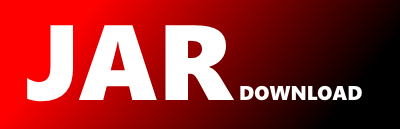
com.prowidesoftware.swift.model.mx.dic.PaymentResponse2 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Data to respond to a Payment request.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PaymentResponse2", propOrder = {
"saleTxId",
"saleRefId",
"poiTxId",
"poiRcncltnId",
"issrRefData",
"rtlrPmtRslt",
"pmtRct",
"lltyRslt",
"cstmrOrdr"
})
public class PaymentResponse2 {
@XmlElement(name = "SaleTxId", required = true)
protected TransactionIdentifier1 saleTxId;
@XmlElement(name = "SaleRefId")
protected String saleRefId;
@XmlElement(name = "POITxId", required = true)
protected TransactionIdentifier1 poiTxId;
@XmlElement(name = "POIRcncltnId")
protected String poiRcncltnId;
@XmlElement(name = "IssrRefData")
protected String issrRefData;
@XmlElement(name = "RtlrPmtRslt", required = true)
protected RetailerPaymentResult2 rtlrPmtRslt;
@XmlElement(name = "PmtRct")
protected List pmtRct;
@XmlElement(name = "LltyRslt")
protected List lltyRslt;
@XmlElement(name = "CstmrOrdr")
protected List cstmrOrdr;
/**
* Gets the value of the saleTxId property.
*
* @return
* possible object is
* {@link TransactionIdentifier1 }
*
*/
public TransactionIdentifier1 getSaleTxId() {
return saleTxId;
}
/**
* Sets the value of the saleTxId property.
*
* @param value
* allowed object is
* {@link TransactionIdentifier1 }
*
*/
public PaymentResponse2 setSaleTxId(TransactionIdentifier1 value) {
this.saleTxId = value;
return this;
}
/**
* Gets the value of the saleRefId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSaleRefId() {
return saleRefId;
}
/**
* Sets the value of the saleRefId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentResponse2 setSaleRefId(String value) {
this.saleRefId = value;
return this;
}
/**
* Gets the value of the poiTxId property.
*
* @return
* possible object is
* {@link TransactionIdentifier1 }
*
*/
public TransactionIdentifier1 getPOITxId() {
return poiTxId;
}
/**
* Sets the value of the poiTxId property.
*
* @param value
* allowed object is
* {@link TransactionIdentifier1 }
*
*/
public PaymentResponse2 setPOITxId(TransactionIdentifier1 value) {
this.poiTxId = value;
return this;
}
/**
* Gets the value of the poiRcncltnId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPOIRcncltnId() {
return poiRcncltnId;
}
/**
* Sets the value of the poiRcncltnId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentResponse2 setPOIRcncltnId(String value) {
this.poiRcncltnId = value;
return this;
}
/**
* Gets the value of the issrRefData property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIssrRefData() {
return issrRefData;
}
/**
* Sets the value of the issrRefData property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentResponse2 setIssrRefData(String value) {
this.issrRefData = value;
return this;
}
/**
* Gets the value of the rtlrPmtRslt property.
*
* @return
* possible object is
* {@link RetailerPaymentResult2 }
*
*/
public RetailerPaymentResult2 getRtlrPmtRslt() {
return rtlrPmtRslt;
}
/**
* Sets the value of the rtlrPmtRslt property.
*
* @param value
* allowed object is
* {@link RetailerPaymentResult2 }
*
*/
public PaymentResponse2 setRtlrPmtRslt(RetailerPaymentResult2 value) {
this.rtlrPmtRslt = value;
return this;
}
/**
* Gets the value of the pmtRct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtRct property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtRct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentReceipt2 }
*
*
* @return
* The value of the pmtRct property.
*/
public List getPmtRct() {
if (pmtRct == null) {
pmtRct = new ArrayList<>();
}
return this.pmtRct;
}
/**
* Gets the value of the lltyRslt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the lltyRslt property.
*
*
* For example, to add a new item, do as follows:
*
* getLltyRslt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LoyaltyResult2 }
*
*
* @return
* The value of the lltyRslt property.
*/
public List getLltyRslt() {
if (lltyRslt == null) {
lltyRslt = new ArrayList<>();
}
return this.lltyRslt;
}
/**
* Gets the value of the cstmrOrdr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cstmrOrdr property.
*
*
* For example, to add a new item, do as follows:
*
* getCstmrOrdr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CustomerOrder1 }
*
*
* @return
* The value of the cstmrOrdr property.
*/
public List getCstmrOrdr() {
if (cstmrOrdr == null) {
cstmrOrdr = new ArrayList<>();
}
return this.cstmrOrdr;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the pmtRct list.
* @see #getPmtRct()
*
*/
public PaymentResponse2 addPmtRct(PaymentReceipt2 pmtRct) {
getPmtRct().add(pmtRct);
return this;
}
/**
* Adds a new item to the lltyRslt list.
* @see #getLltyRslt()
*
*/
public PaymentResponse2 addLltyRslt(LoyaltyResult2 lltyRslt) {
getLltyRslt().add(lltyRslt);
return this;
}
/**
* Adds a new item to the cstmrOrdr list.
* @see #getCstmrOrdr()
*
*/
public PaymentResponse2 addCstmrOrdr(CustomerOrder1 cstmrOrdr) {
getCstmrOrdr().add(cstmrOrdr);
return this;
}
}