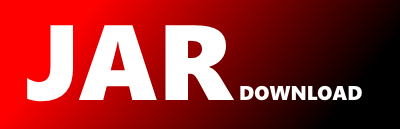
com.prowidesoftware.swift.model.mx.dic.PaymentSearch7 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Defines the criteria used to search for a payment.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PaymentSearch7", propOrder = {
"msgId",
"reqdExctnDt",
"pmtId",
"sts",
"instdAmt",
"instdAmtCcy",
"cdtDbtInd",
"intrBkSttlmAmt",
"intrBkSttlmAmtCcy",
"pmtMtd",
"pmtTp",
"prty",
"prcgVldtyTm",
"instr",
"txId",
"intrBkSttlmDt",
"endToEndId",
"pties"
})
public class PaymentSearch7 {
@XmlElement(name = "MsgId")
protected List msgId;
@XmlElement(name = "ReqdExctnDt")
protected List reqdExctnDt;
@XmlElement(name = "PmtId")
protected List pmtId;
@XmlElement(name = "Sts")
protected List sts;
@XmlElement(name = "InstdAmt")
protected List instdAmt;
@XmlElement(name = "InstdAmtCcy")
protected List instdAmtCcy;
@XmlElement(name = "CdtDbtInd")
@XmlSchemaType(name = "string")
protected CreditDebitCode cdtDbtInd;
@XmlElement(name = "IntrBkSttlmAmt")
protected List intrBkSttlmAmt;
@XmlElement(name = "IntrBkSttlmAmtCcy")
protected List intrBkSttlmAmtCcy;
@XmlElement(name = "PmtMtd")
protected List pmtMtd;
@XmlElement(name = "PmtTp")
protected List pmtTp;
@XmlElement(name = "Prty")
protected List prty;
@XmlElement(name = "PrcgVldtyTm")
protected List prcgVldtyTm;
@XmlElement(name = "Instr")
@XmlSchemaType(name = "string")
protected List instr;
@XmlElement(name = "TxId")
protected List txId;
@XmlElement(name = "IntrBkSttlmDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected List intrBkSttlmDt;
@XmlElement(name = "EndToEndId")
protected List endToEndId;
@XmlElement(name = "Pties")
protected PaymentTransactionParty2 pties;
/**
* Gets the value of the msgId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the msgId property.
*
*
* For example, to add a new item, do as follows:
*
* getMsgId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the msgId property.
*/
public List getMsgId() {
if (msgId == null) {
msgId = new ArrayList<>();
}
return this.msgId;
}
/**
* Gets the value of the reqdExctnDt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the reqdExctnDt property.
*
*
* For example, to add a new item, do as follows:
*
* getReqdExctnDt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DateAndDateTimeSearch3Choice }
*
*
* @return
* The value of the reqdExctnDt property.
*/
public List getReqdExctnDt() {
if (reqdExctnDt == null) {
reqdExctnDt = new ArrayList<>();
}
return this.reqdExctnDt;
}
/**
* Gets the value of the pmtId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtId property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentIdentification5Choice }
*
*
* @return
* The value of the pmtId property.
*/
public List getPmtId() {
if (pmtId == null) {
pmtId = new ArrayList<>();
}
return this.pmtId;
}
/**
* Gets the value of the sts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the sts property.
*
*
* For example, to add a new item, do as follows:
*
* getSts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InstructionStatusSearch4 }
*
*
* @return
* The value of the sts property.
*/
public List getSts() {
if (sts == null) {
sts = new ArrayList<>();
}
return this.sts;
}
/**
* Gets the value of the instdAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the instdAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getInstdAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ActiveOrHistoricAmountRange2Choice }
*
*
* @return
* The value of the instdAmt property.
*/
public List getInstdAmt() {
if (instdAmt == null) {
instdAmt = new ArrayList<>();
}
return this.instdAmt;
}
/**
* Gets the value of the instdAmtCcy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the instdAmtCcy property.
*
*
* For example, to add a new item, do as follows:
*
* getInstdAmtCcy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the instdAmtCcy property.
*/
public List getInstdAmtCcy() {
if (instdAmtCcy == null) {
instdAmtCcy = new ArrayList<>();
}
return this.instdAmtCcy;
}
/**
* Gets the value of the cdtDbtInd property.
*
* @return
* possible object is
* {@link CreditDebitCode }
*
*/
public CreditDebitCode getCdtDbtInd() {
return cdtDbtInd;
}
/**
* Sets the value of the cdtDbtInd property.
*
* @param value
* allowed object is
* {@link CreditDebitCode }
*
*/
public PaymentSearch7 setCdtDbtInd(CreditDebitCode value) {
this.cdtDbtInd = value;
return this;
}
/**
* Gets the value of the intrBkSttlmAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrBkSttlmAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrBkSttlmAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ActiveAmountRange3Choice }
*
*
* @return
* The value of the intrBkSttlmAmt property.
*/
public List getIntrBkSttlmAmt() {
if (intrBkSttlmAmt == null) {
intrBkSttlmAmt = new ArrayList<>();
}
return this.intrBkSttlmAmt;
}
/**
* Gets the value of the intrBkSttlmAmtCcy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrBkSttlmAmtCcy property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrBkSttlmAmtCcy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the intrBkSttlmAmtCcy property.
*/
public List getIntrBkSttlmAmtCcy() {
if (intrBkSttlmAmtCcy == null) {
intrBkSttlmAmtCcy = new ArrayList<>();
}
return this.intrBkSttlmAmtCcy;
}
/**
* Gets the value of the pmtMtd property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtMtd property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtMtd().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentOrigin1Choice }
*
*
* @return
* The value of the pmtMtd property.
*/
public List getPmtMtd() {
if (pmtMtd == null) {
pmtMtd = new ArrayList<>();
}
return this.pmtMtd;
}
/**
* Gets the value of the pmtTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtTp property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentType4Choice }
*
*
* @return
* The value of the pmtTp property.
*/
public List getPmtTp() {
if (pmtTp == null) {
pmtTp = new ArrayList<>();
}
return this.pmtTp;
}
/**
* Gets the value of the prty property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the prty property.
*
*
* For example, to add a new item, do as follows:
*
* getPrty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PriorityCode3Choice }
*
*
* @return
* The value of the prty property.
*/
public List getPrty() {
if (prty == null) {
prty = new ArrayList<>();
}
return this.prty;
}
/**
* Gets the value of the prcgVldtyTm property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the prcgVldtyTm property.
*
*
* For example, to add a new item, do as follows:
*
* getPrcgVldtyTm().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DateTimePeriod1Choice }
*
*
* @return
* The value of the prcgVldtyTm property.
*/
public List getPrcgVldtyTm() {
if (prcgVldtyTm == null) {
prcgVldtyTm = new ArrayList<>();
}
return this.prcgVldtyTm;
}
/**
* Gets the value of the instr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the instr property.
*
*
* For example, to add a new item, do as follows:
*
* getInstr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Instruction1Code }
*
*
* @return
* The value of the instr property.
*/
public List getInstr() {
if (instr == null) {
instr = new ArrayList<>();
}
return this.instr;
}
/**
* Gets the value of the txId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the txId property.
*
*
* For example, to add a new item, do as follows:
*
* getTxId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the txId property.
*/
public List getTxId() {
if (txId == null) {
txId = new ArrayList<>();
}
return this.txId;
}
/**
* Gets the value of the intrBkSttlmDt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrBkSttlmDt property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrBkSttlmDt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the intrBkSttlmDt property.
*/
public List getIntrBkSttlmDt() {
if (intrBkSttlmDt == null) {
intrBkSttlmDt = new ArrayList<>();
}
return this.intrBkSttlmDt;
}
/**
* Gets the value of the endToEndId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the endToEndId property.
*
*
* For example, to add a new item, do as follows:
*
* getEndToEndId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the endToEndId property.
*/
public List getEndToEndId() {
if (endToEndId == null) {
endToEndId = new ArrayList<>();
}
return this.endToEndId;
}
/**
* Gets the value of the pties property.
*
* @return
* possible object is
* {@link PaymentTransactionParty2 }
*
*/
public PaymentTransactionParty2 getPties() {
return pties;
}
/**
* Sets the value of the pties property.
*
* @param value
* allowed object is
* {@link PaymentTransactionParty2 }
*
*/
public PaymentSearch7 setPties(PaymentTransactionParty2 value) {
this.pties = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the msgId list.
* @see #getMsgId()
*
*/
public PaymentSearch7 addMsgId(String msgId) {
getMsgId().add(msgId);
return this;
}
/**
* Adds a new item to the reqdExctnDt list.
* @see #getReqdExctnDt()
*
*/
public PaymentSearch7 addReqdExctnDt(DateAndDateTimeSearch3Choice reqdExctnDt) {
getReqdExctnDt().add(reqdExctnDt);
return this;
}
/**
* Adds a new item to the pmtId list.
* @see #getPmtId()
*
*/
public PaymentSearch7 addPmtId(PaymentIdentification5Choice pmtId) {
getPmtId().add(pmtId);
return this;
}
/**
* Adds a new item to the sts list.
* @see #getSts()
*
*/
public PaymentSearch7 addSts(InstructionStatusSearch4 sts) {
getSts().add(sts);
return this;
}
/**
* Adds a new item to the instdAmt list.
* @see #getInstdAmt()
*
*/
public PaymentSearch7 addInstdAmt(ActiveOrHistoricAmountRange2Choice instdAmt) {
getInstdAmt().add(instdAmt);
return this;
}
/**
* Adds a new item to the instdAmtCcy list.
* @see #getInstdAmtCcy()
*
*/
public PaymentSearch7 addInstdAmtCcy(String instdAmtCcy) {
getInstdAmtCcy().add(instdAmtCcy);
return this;
}
/**
* Adds a new item to the intrBkSttlmAmt list.
* @see #getIntrBkSttlmAmt()
*
*/
public PaymentSearch7 addIntrBkSttlmAmt(ActiveAmountRange3Choice intrBkSttlmAmt) {
getIntrBkSttlmAmt().add(intrBkSttlmAmt);
return this;
}
/**
* Adds a new item to the intrBkSttlmAmtCcy list.
* @see #getIntrBkSttlmAmtCcy()
*
*/
public PaymentSearch7 addIntrBkSttlmAmtCcy(String intrBkSttlmAmtCcy) {
getIntrBkSttlmAmtCcy().add(intrBkSttlmAmtCcy);
return this;
}
/**
* Adds a new item to the pmtMtd list.
* @see #getPmtMtd()
*
*/
public PaymentSearch7 addPmtMtd(PaymentOrigin1Choice pmtMtd) {
getPmtMtd().add(pmtMtd);
return this;
}
/**
* Adds a new item to the pmtTp list.
* @see #getPmtTp()
*
*/
public PaymentSearch7 addPmtTp(PaymentType4Choice pmtTp) {
getPmtTp().add(pmtTp);
return this;
}
/**
* Adds a new item to the prty list.
* @see #getPrty()
*
*/
public PaymentSearch7 addPrty(PriorityCode3Choice prty) {
getPrty().add(prty);
return this;
}
/**
* Adds a new item to the prcgVldtyTm list.
* @see #getPrcgVldtyTm()
*
*/
public PaymentSearch7 addPrcgVldtyTm(DateTimePeriod1Choice prcgVldtyTm) {
getPrcgVldtyTm().add(prcgVldtyTm);
return this;
}
/**
* Adds a new item to the instr list.
* @see #getInstr()
*
*/
public PaymentSearch7 addInstr(Instruction1Code instr) {
getInstr().add(instr);
return this;
}
/**
* Adds a new item to the txId list.
* @see #getTxId()
*
*/
public PaymentSearch7 addTxId(String txId) {
getTxId().add(txId);
return this;
}
/**
* Adds a new item to the intrBkSttlmDt list.
* @see #getIntrBkSttlmDt()
*
*/
public PaymentSearch7 addIntrBkSttlmDt(LocalDate intrBkSttlmDt) {
getIntrBkSttlmDt().add(intrBkSttlmDt);
return this;
}
/**
* Adds a new item to the endToEndId list.
* @see #getEndToEndId()
*
*/
public PaymentSearch7 addEndToEndId(String endToEndId) {
getEndToEndId().add(endToEndId);
return this;
}
}