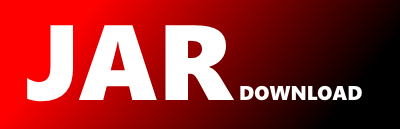
com.prowidesoftware.swift.model.mx.dic.PaymentTerminalParameters6 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Manufacturer configuration parameters of the point of interaction (POI).
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PaymentTerminalParameters6", propOrder = {
"actnTp",
"vndrId",
"vrsn",
"paramFrmtIdr",
"clckSynctn",
"tmZoneLine",
"lclDtTm",
"othrParams"
})
public class PaymentTerminalParameters6 {
@XmlElement(name = "ActnTp", required = true)
@XmlSchemaType(name = "string")
protected TerminalManagementAction3Code actnTp;
@XmlElement(name = "VndrId")
protected String vndrId;
@XmlElement(name = "Vrsn")
protected String vrsn;
@XmlElement(name = "ParamFrmtIdr")
protected String paramFrmtIdr;
@XmlElement(name = "ClckSynctn")
protected ClockSynchronisation2 clckSynctn;
@XmlElement(name = "TmZoneLine")
protected List tmZoneLine;
@XmlElement(name = "LclDtTm")
protected List lclDtTm;
@XmlElement(name = "OthrParams")
protected byte[] othrParams;
/**
* Gets the value of the actnTp property.
*
* @return
* possible object is
* {@link TerminalManagementAction3Code }
*
*/
public TerminalManagementAction3Code getActnTp() {
return actnTp;
}
/**
* Sets the value of the actnTp property.
*
* @param value
* allowed object is
* {@link TerminalManagementAction3Code }
*
*/
public PaymentTerminalParameters6 setActnTp(TerminalManagementAction3Code value) {
this.actnTp = value;
return this;
}
/**
* Gets the value of the vndrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVndrId() {
return vndrId;
}
/**
* Sets the value of the vndrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTerminalParameters6 setVndrId(String value) {
this.vndrId = value;
return this;
}
/**
* Gets the value of the vrsn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVrsn() {
return vrsn;
}
/**
* Sets the value of the vrsn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTerminalParameters6 setVrsn(String value) {
this.vrsn = value;
return this;
}
/**
* Gets the value of the paramFrmtIdr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getParamFrmtIdr() {
return paramFrmtIdr;
}
/**
* Sets the value of the paramFrmtIdr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTerminalParameters6 setParamFrmtIdr(String value) {
this.paramFrmtIdr = value;
return this;
}
/**
* Gets the value of the clckSynctn property.
*
* @return
* possible object is
* {@link ClockSynchronisation2 }
*
*/
public ClockSynchronisation2 getClckSynctn() {
return clckSynctn;
}
/**
* Sets the value of the clckSynctn property.
*
* @param value
* allowed object is
* {@link ClockSynchronisation2 }
*
*/
public PaymentTerminalParameters6 setClckSynctn(ClockSynchronisation2 value) {
this.clckSynctn = value;
return this;
}
/**
* Gets the value of the tmZoneLine property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tmZoneLine property.
*
*
* For example, to add a new item, do as follows:
*
* getTmZoneLine().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the tmZoneLine property.
*/
public List getTmZoneLine() {
if (tmZoneLine == null) {
tmZoneLine = new ArrayList<>();
}
return this.tmZoneLine;
}
/**
* Gets the value of the lclDtTm property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the lclDtTm property.
*
*
* For example, to add a new item, do as follows:
*
* getLclDtTm().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LocalDateTime1 }
*
*
* @return
* The value of the lclDtTm property.
*/
public List getLclDtTm() {
if (lclDtTm == null) {
lclDtTm = new ArrayList<>();
}
return this.lclDtTm;
}
/**
* Gets the value of the othrParams property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getOthrParams() {
return othrParams;
}
/**
* Sets the value of the othrParams property.
*
* @param value
* allowed object is
* byte[]
*/
public PaymentTerminalParameters6 setOthrParams(byte[] value) {
this.othrParams = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the tmZoneLine list.
* @see #getTmZoneLine()
*
*/
public PaymentTerminalParameters6 addTmZoneLine(String tmZoneLine) {
getTmZoneLine().add(tmZoneLine);
return this;
}
/**
* Adds a new item to the lclDtTm list.
* @see #getLclDtTm()
*
*/
public PaymentTerminalParameters6 addLclDtTm(LocalDateTime1 lclDtTm) {
getLclDtTm().add(lclDtTm);
return this;
}
}