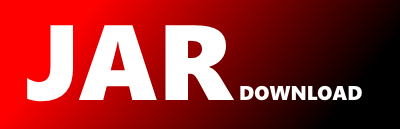
com.prowidesoftware.swift.model.mx.dic.PaymentTransaction103 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Provides reference and status information on the original transactions, included in the original instruction, to which the cancellation request message applies.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PaymentTransaction103", propOrder = {
"cxlStsId",
"rslvdCase",
"orgnlInstrId",
"orgnlEndToEndId",
"uetr",
"txCxlSts",
"cxlStsRsnInf",
"orgnlInstdAmt",
"orgnlReqdExctnDt",
"orgnlReqdColltnDt",
"orgnlTxRef"
})
public class PaymentTransaction103 {
@XmlElement(name = "CxlStsId")
protected String cxlStsId;
@XmlElement(name = "RslvdCase")
protected Case5 rslvdCase;
@XmlElement(name = "OrgnlInstrId")
protected String orgnlInstrId;
@XmlElement(name = "OrgnlEndToEndId")
protected String orgnlEndToEndId;
@XmlElement(name = "UETR")
protected String uetr;
@XmlElement(name = "TxCxlSts")
@XmlSchemaType(name = "string")
protected CancellationIndividualStatus1Code txCxlSts;
@XmlElement(name = "CxlStsRsnInf")
protected List cxlStsRsnInf;
@XmlElement(name = "OrgnlInstdAmt")
protected ActiveOrHistoricCurrencyAndAmount orgnlInstdAmt;
@XmlElement(name = "OrgnlReqdExctnDt")
protected DateAndDateTime2Choice orgnlReqdExctnDt;
@XmlElement(name = "OrgnlReqdColltnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate orgnlReqdColltnDt;
@XmlElement(name = "OrgnlTxRef")
protected OriginalTransactionReference28 orgnlTxRef;
/**
* Gets the value of the cxlStsId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCxlStsId() {
return cxlStsId;
}
/**
* Sets the value of the cxlStsId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction103 setCxlStsId(String value) {
this.cxlStsId = value;
return this;
}
/**
* Gets the value of the rslvdCase property.
*
* @return
* possible object is
* {@link Case5 }
*
*/
public Case5 getRslvdCase() {
return rslvdCase;
}
/**
* Sets the value of the rslvdCase property.
*
* @param value
* allowed object is
* {@link Case5 }
*
*/
public PaymentTransaction103 setRslvdCase(Case5 value) {
this.rslvdCase = value;
return this;
}
/**
* Gets the value of the orgnlInstrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgnlInstrId() {
return orgnlInstrId;
}
/**
* Sets the value of the orgnlInstrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction103 setOrgnlInstrId(String value) {
this.orgnlInstrId = value;
return this;
}
/**
* Gets the value of the orgnlEndToEndId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgnlEndToEndId() {
return orgnlEndToEndId;
}
/**
* Sets the value of the orgnlEndToEndId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction103 setOrgnlEndToEndId(String value) {
this.orgnlEndToEndId = value;
return this;
}
/**
* Gets the value of the uetr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUETR() {
return uetr;
}
/**
* Sets the value of the uetr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction103 setUETR(String value) {
this.uetr = value;
return this;
}
/**
* Gets the value of the txCxlSts property.
*
* @return
* possible object is
* {@link CancellationIndividualStatus1Code }
*
*/
public CancellationIndividualStatus1Code getTxCxlSts() {
return txCxlSts;
}
/**
* Sets the value of the txCxlSts property.
*
* @param value
* allowed object is
* {@link CancellationIndividualStatus1Code }
*
*/
public PaymentTransaction103 setTxCxlSts(CancellationIndividualStatus1Code value) {
this.txCxlSts = value;
return this;
}
/**
* Gets the value of the cxlStsRsnInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cxlStsRsnInf property.
*
*
* For example, to add a new item, do as follows:
*
* getCxlStsRsnInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CancellationStatusReason4 }
*
*
* @return
* The value of the cxlStsRsnInf property.
*/
public List getCxlStsRsnInf() {
if (cxlStsRsnInf == null) {
cxlStsRsnInf = new ArrayList<>();
}
return this.cxlStsRsnInf;
}
/**
* Gets the value of the orgnlInstdAmt property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getOrgnlInstdAmt() {
return orgnlInstdAmt;
}
/**
* Sets the value of the orgnlInstdAmt property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public PaymentTransaction103 setOrgnlInstdAmt(ActiveOrHistoricCurrencyAndAmount value) {
this.orgnlInstdAmt = value;
return this;
}
/**
* Gets the value of the orgnlReqdExctnDt property.
*
* @return
* possible object is
* {@link DateAndDateTime2Choice }
*
*/
public DateAndDateTime2Choice getOrgnlReqdExctnDt() {
return orgnlReqdExctnDt;
}
/**
* Sets the value of the orgnlReqdExctnDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTime2Choice }
*
*/
public PaymentTransaction103 setOrgnlReqdExctnDt(DateAndDateTime2Choice value) {
this.orgnlReqdExctnDt = value;
return this;
}
/**
* Gets the value of the orgnlReqdColltnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getOrgnlReqdColltnDt() {
return orgnlReqdColltnDt;
}
/**
* Sets the value of the orgnlReqdColltnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction103 setOrgnlReqdColltnDt(LocalDate value) {
this.orgnlReqdColltnDt = value;
return this;
}
/**
* Gets the value of the orgnlTxRef property.
*
* @return
* possible object is
* {@link OriginalTransactionReference28 }
*
*/
public OriginalTransactionReference28 getOrgnlTxRef() {
return orgnlTxRef;
}
/**
* Sets the value of the orgnlTxRef property.
*
* @param value
* allowed object is
* {@link OriginalTransactionReference28 }
*
*/
public PaymentTransaction103 setOrgnlTxRef(OriginalTransactionReference28 value) {
this.orgnlTxRef = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the cxlStsRsnInf list.
* @see #getCxlStsRsnInf()
*
*/
public PaymentTransaction103 addCxlStsRsnInf(CancellationStatusReason4 cxlStsRsnInf) {
getCxlStsRsnInf().add(cxlStsRsnInf);
return this;
}
}