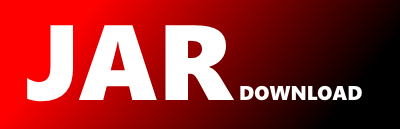
com.prowidesoftware.swift.model.mx.dic.PaymentTransaction123 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Provides further details on the original transactions, to which the status report message refers.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PaymentTransaction123", propOrder = {
"stsId",
"orgnlGrpInf",
"orgnlInstrId",
"orgnlEndToEndId",
"orgnlTxId",
"orgnlUETR",
"txSts",
"stsRsnInf",
"chrgsInf",
"accptncDtTm",
"fctvIntrBkSttlmDt",
"acctSvcrRef",
"clrSysRef",
"instgAgt",
"instdAgt",
"orgnlTxRef",
"splmtryData"
})
public class PaymentTransaction123 {
@XmlElement(name = "StsId")
protected String stsId;
@XmlElement(name = "OrgnlGrpInf")
protected OriginalGroupInformation29 orgnlGrpInf;
@XmlElement(name = "OrgnlInstrId")
protected String orgnlInstrId;
@XmlElement(name = "OrgnlEndToEndId")
protected String orgnlEndToEndId;
@XmlElement(name = "OrgnlTxId")
protected String orgnlTxId;
@XmlElement(name = "OrgnlUETR")
protected String orgnlUETR;
@XmlElement(name = "TxSts")
protected String txSts;
@XmlElement(name = "StsRsnInf")
protected List stsRsnInf;
@XmlElement(name = "ChrgsInf")
protected List chrgsInf;
@XmlElement(name = "AccptncDtTm", type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime accptncDtTm;
@XmlElement(name = "FctvIntrBkSttlmDt")
protected DateAndDateTime2Choice fctvIntrBkSttlmDt;
@XmlElement(name = "AcctSvcrRef")
protected String acctSvcrRef;
@XmlElement(name = "ClrSysRef")
protected String clrSysRef;
@XmlElement(name = "InstgAgt")
protected BranchAndFinancialInstitutionIdentification6 instgAgt;
@XmlElement(name = "InstdAgt")
protected BranchAndFinancialInstitutionIdentification6 instdAgt;
@XmlElement(name = "OrgnlTxRef")
protected OriginalTransactionReference31 orgnlTxRef;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the stsId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStsId() {
return stsId;
}
/**
* Sets the value of the stsId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setStsId(String value) {
this.stsId = value;
return this;
}
/**
* Gets the value of the orgnlGrpInf property.
*
* @return
* possible object is
* {@link OriginalGroupInformation29 }
*
*/
public OriginalGroupInformation29 getOrgnlGrpInf() {
return orgnlGrpInf;
}
/**
* Sets the value of the orgnlGrpInf property.
*
* @param value
* allowed object is
* {@link OriginalGroupInformation29 }
*
*/
public PaymentTransaction123 setOrgnlGrpInf(OriginalGroupInformation29 value) {
this.orgnlGrpInf = value;
return this;
}
/**
* Gets the value of the orgnlInstrId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgnlInstrId() {
return orgnlInstrId;
}
/**
* Sets the value of the orgnlInstrId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setOrgnlInstrId(String value) {
this.orgnlInstrId = value;
return this;
}
/**
* Gets the value of the orgnlEndToEndId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgnlEndToEndId() {
return orgnlEndToEndId;
}
/**
* Sets the value of the orgnlEndToEndId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setOrgnlEndToEndId(String value) {
this.orgnlEndToEndId = value;
return this;
}
/**
* Gets the value of the orgnlTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgnlTxId() {
return orgnlTxId;
}
/**
* Sets the value of the orgnlTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setOrgnlTxId(String value) {
this.orgnlTxId = value;
return this;
}
/**
* Gets the value of the orgnlUETR property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrgnlUETR() {
return orgnlUETR;
}
/**
* Sets the value of the orgnlUETR property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setOrgnlUETR(String value) {
this.orgnlUETR = value;
return this;
}
/**
* Gets the value of the txSts property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxSts() {
return txSts;
}
/**
* Sets the value of the txSts property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setTxSts(String value) {
this.txSts = value;
return this;
}
/**
* Gets the value of the stsRsnInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the stsRsnInf property.
*
*
* For example, to add a new item, do as follows:
*
* getStsRsnInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link StatusReasonInformation12 }
*
*
* @return
* The value of the stsRsnInf property.
*/
public List getStsRsnInf() {
if (stsRsnInf == null) {
stsRsnInf = new ArrayList<>();
}
return this.stsRsnInf;
}
/**
* Gets the value of the chrgsInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the chrgsInf property.
*
*
* For example, to add a new item, do as follows:
*
* getChrgsInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Charges7 }
*
*
* @return
* The value of the chrgsInf property.
*/
public List getChrgsInf() {
if (chrgsInf == null) {
chrgsInf = new ArrayList<>();
}
return this.chrgsInf;
}
/**
* Gets the value of the accptncDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getAccptncDtTm() {
return accptncDtTm;
}
/**
* Sets the value of the accptncDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setAccptncDtTm(OffsetDateTime value) {
this.accptncDtTm = value;
return this;
}
/**
* Gets the value of the fctvIntrBkSttlmDt property.
*
* @return
* possible object is
* {@link DateAndDateTime2Choice }
*
*/
public DateAndDateTime2Choice getFctvIntrBkSttlmDt() {
return fctvIntrBkSttlmDt;
}
/**
* Sets the value of the fctvIntrBkSttlmDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTime2Choice }
*
*/
public PaymentTransaction123 setFctvIntrBkSttlmDt(DateAndDateTime2Choice value) {
this.fctvIntrBkSttlmDt = value;
return this;
}
/**
* Gets the value of the acctSvcrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAcctSvcrRef() {
return acctSvcrRef;
}
/**
* Sets the value of the acctSvcrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setAcctSvcrRef(String value) {
this.acctSvcrRef = value;
return this;
}
/**
* Gets the value of the clrSysRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClrSysRef() {
return clrSysRef;
}
/**
* Sets the value of the clrSysRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PaymentTransaction123 setClrSysRef(String value) {
this.clrSysRef = value;
return this;
}
/**
* Gets the value of the instgAgt property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification6 }
*
*/
public BranchAndFinancialInstitutionIdentification6 getInstgAgt() {
return instgAgt;
}
/**
* Sets the value of the instgAgt property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification6 }
*
*/
public PaymentTransaction123 setInstgAgt(BranchAndFinancialInstitutionIdentification6 value) {
this.instgAgt = value;
return this;
}
/**
* Gets the value of the instdAgt property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification6 }
*
*/
public BranchAndFinancialInstitutionIdentification6 getInstdAgt() {
return instdAgt;
}
/**
* Sets the value of the instdAgt property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification6 }
*
*/
public PaymentTransaction123 setInstdAgt(BranchAndFinancialInstitutionIdentification6 value) {
this.instdAgt = value;
return this;
}
/**
* Gets the value of the orgnlTxRef property.
*
* @return
* possible object is
* {@link OriginalTransactionReference31 }
*
*/
public OriginalTransactionReference31 getOrgnlTxRef() {
return orgnlTxRef;
}
/**
* Sets the value of the orgnlTxRef property.
*
* @param value
* allowed object is
* {@link OriginalTransactionReference31 }
*
*/
public PaymentTransaction123 setOrgnlTxRef(OriginalTransactionReference31 value) {
this.orgnlTxRef = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the stsRsnInf list.
* @see #getStsRsnInf()
*
*/
public PaymentTransaction123 addStsRsnInf(StatusReasonInformation12 stsRsnInf) {
getStsRsnInf().add(stsRsnInf);
return this;
}
/**
* Adds a new item to the chrgsInf list.
* @see #getChrgsInf()
*
*/
public PaymentTransaction123 addChrgsInf(Charges7 chrgsInf) {
getChrgsInf().add(chrgsInf);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public PaymentTransaction123 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}