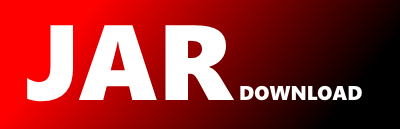
com.prowidesoftware.swift.model.mx.dic.PortfolioTransferConfirmationV11 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The PortfolioTransferConfirmation message is sent by an executing party, for example, a (old) plan manager (transferor), to the instructing party for example, a (new) plan manager (transferee), to confirm the transfer of financial instruments from the client’s account at the old plan manager (transferor) to the clients account at the new plan manager (transferee).
* Usage
* The PortfolioTransferConfirmation message is used to confirm one or more portfolio transfers for one client.
* The reference of each portfolio transfer confirmation is identified in TransferConfirmationIdentification. The reference of the original portfolio transfer as assigned by the instructing party is specified in TransferInstructionReference. The message identification of the PortfolioTransferInstruction message in which the portfolio transfers were conveyed may also be quoted in RelatedReference but this is not recommended.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PortfolioTransferConfirmationV11", propOrder = {
"msgRef",
"poolRef",
"prvsRef",
"rltdRef",
"pmryIndvInvstr",
"regdHldr",
"scndryIndvInvstr",
"othrIndvInvstr",
"pmryCorpInvstr",
"scndryCorpInvstr",
"othrCorpInvstr",
"trfrAcct",
"nmneeAcct",
"trfee",
"pdctTrf",
"mktPrctcVrsn",
"xtnsn"
})
public class PortfolioTransferConfirmationV11 {
@XmlElement(name = "MsgRef", required = true)
protected MessageIdentification1 msgRef;
@XmlElement(name = "PoolRef")
protected AdditionalReference11 poolRef;
@XmlElement(name = "PrvsRef")
protected AdditionalReference10 prvsRef;
@XmlElement(name = "RltdRef")
protected AdditionalReference10 rltdRef;
@XmlElement(name = "PmryIndvInvstr")
protected IndividualPerson8 pmryIndvInvstr;
@XmlElement(name = "RegdHldr")
protected IndividualPerson8 regdHldr;
@XmlElement(name = "ScndryIndvInvstr")
protected IndividualPerson8 scndryIndvInvstr;
@XmlElement(name = "OthrIndvInvstr")
protected List othrIndvInvstr;
@XmlElement(name = "PmryCorpInvstr")
protected Organisation36 pmryCorpInvstr;
@XmlElement(name = "ScndryCorpInvstr")
protected Organisation36 scndryCorpInvstr;
@XmlElement(name = "OthrCorpInvstr")
protected List othrCorpInvstr;
@XmlElement(name = "TrfrAcct", required = true)
protected InvestmentAccount69 trfrAcct;
@XmlElement(name = "NmneeAcct")
protected InvestmentAccount69 nmneeAcct;
@XmlElement(name = "Trfee", required = true)
protected PartyIdentification132 trfee;
@XmlElement(name = "PdctTrf", required = true)
protected List pdctTrf;
@XmlElement(name = "MktPrctcVrsn")
protected MarketPracticeVersion1 mktPrctcVrsn;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgRef property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getMsgRef() {
return msgRef;
}
/**
* Sets the value of the msgRef property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public PortfolioTransferConfirmationV11 setMsgRef(MessageIdentification1 value) {
this.msgRef = value;
return this;
}
/**
* Gets the value of the poolRef property.
*
* @return
* possible object is
* {@link AdditionalReference11 }
*
*/
public AdditionalReference11 getPoolRef() {
return poolRef;
}
/**
* Sets the value of the poolRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference11 }
*
*/
public PortfolioTransferConfirmationV11 setPoolRef(AdditionalReference11 value) {
this.poolRef = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
* @return
* possible object is
* {@link AdditionalReference10 }
*
*/
public AdditionalReference10 getPrvsRef() {
return prvsRef;
}
/**
* Sets the value of the prvsRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference10 }
*
*/
public PortfolioTransferConfirmationV11 setPrvsRef(AdditionalReference10 value) {
this.prvsRef = value;
return this;
}
/**
* Gets the value of the rltdRef property.
*
* @return
* possible object is
* {@link AdditionalReference10 }
*
*/
public AdditionalReference10 getRltdRef() {
return rltdRef;
}
/**
* Sets the value of the rltdRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference10 }
*
*/
public PortfolioTransferConfirmationV11 setRltdRef(AdditionalReference10 value) {
this.rltdRef = value;
return this;
}
/**
* Gets the value of the pmryIndvInvstr property.
*
* @return
* possible object is
* {@link IndividualPerson8 }
*
*/
public IndividualPerson8 getPmryIndvInvstr() {
return pmryIndvInvstr;
}
/**
* Sets the value of the pmryIndvInvstr property.
*
* @param value
* allowed object is
* {@link IndividualPerson8 }
*
*/
public PortfolioTransferConfirmationV11 setPmryIndvInvstr(IndividualPerson8 value) {
this.pmryIndvInvstr = value;
return this;
}
/**
* Gets the value of the regdHldr property.
*
* @return
* possible object is
* {@link IndividualPerson8 }
*
*/
public IndividualPerson8 getRegdHldr() {
return regdHldr;
}
/**
* Sets the value of the regdHldr property.
*
* @param value
* allowed object is
* {@link IndividualPerson8 }
*
*/
public PortfolioTransferConfirmationV11 setRegdHldr(IndividualPerson8 value) {
this.regdHldr = value;
return this;
}
/**
* Gets the value of the scndryIndvInvstr property.
*
* @return
* possible object is
* {@link IndividualPerson8 }
*
*/
public IndividualPerson8 getScndryIndvInvstr() {
return scndryIndvInvstr;
}
/**
* Sets the value of the scndryIndvInvstr property.
*
* @param value
* allowed object is
* {@link IndividualPerson8 }
*
*/
public PortfolioTransferConfirmationV11 setScndryIndvInvstr(IndividualPerson8 value) {
this.scndryIndvInvstr = value;
return this;
}
/**
* Gets the value of the othrIndvInvstr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrIndvInvstr property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrIndvInvstr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IndividualPerson8 }
*
*
* @return
* The value of the othrIndvInvstr property.
*/
public List getOthrIndvInvstr() {
if (othrIndvInvstr == null) {
othrIndvInvstr = new ArrayList<>();
}
return this.othrIndvInvstr;
}
/**
* Gets the value of the pmryCorpInvstr property.
*
* @return
* possible object is
* {@link Organisation36 }
*
*/
public Organisation36 getPmryCorpInvstr() {
return pmryCorpInvstr;
}
/**
* Sets the value of the pmryCorpInvstr property.
*
* @param value
* allowed object is
* {@link Organisation36 }
*
*/
public PortfolioTransferConfirmationV11 setPmryCorpInvstr(Organisation36 value) {
this.pmryCorpInvstr = value;
return this;
}
/**
* Gets the value of the scndryCorpInvstr property.
*
* @return
* possible object is
* {@link Organisation36 }
*
*/
public Organisation36 getScndryCorpInvstr() {
return scndryCorpInvstr;
}
/**
* Sets the value of the scndryCorpInvstr property.
*
* @param value
* allowed object is
* {@link Organisation36 }
*
*/
public PortfolioTransferConfirmationV11 setScndryCorpInvstr(Organisation36 value) {
this.scndryCorpInvstr = value;
return this;
}
/**
* Gets the value of the othrCorpInvstr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrCorpInvstr property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrCorpInvstr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Organisation36 }
*
*
* @return
* The value of the othrCorpInvstr property.
*/
public List getOthrCorpInvstr() {
if (othrCorpInvstr == null) {
othrCorpInvstr = new ArrayList<>();
}
return this.othrCorpInvstr;
}
/**
* Gets the value of the trfrAcct property.
*
* @return
* possible object is
* {@link InvestmentAccount69 }
*
*/
public InvestmentAccount69 getTrfrAcct() {
return trfrAcct;
}
/**
* Sets the value of the trfrAcct property.
*
* @param value
* allowed object is
* {@link InvestmentAccount69 }
*
*/
public PortfolioTransferConfirmationV11 setTrfrAcct(InvestmentAccount69 value) {
this.trfrAcct = value;
return this;
}
/**
* Gets the value of the nmneeAcct property.
*
* @return
* possible object is
* {@link InvestmentAccount69 }
*
*/
public InvestmentAccount69 getNmneeAcct() {
return nmneeAcct;
}
/**
* Sets the value of the nmneeAcct property.
*
* @param value
* allowed object is
* {@link InvestmentAccount69 }
*
*/
public PortfolioTransferConfirmationV11 setNmneeAcct(InvestmentAccount69 value) {
this.nmneeAcct = value;
return this;
}
/**
* Gets the value of the trfee property.
*
* @return
* possible object is
* {@link PartyIdentification132 }
*
*/
public PartyIdentification132 getTrfee() {
return trfee;
}
/**
* Sets the value of the trfee property.
*
* @param value
* allowed object is
* {@link PartyIdentification132 }
*
*/
public PortfolioTransferConfirmationV11 setTrfee(PartyIdentification132 value) {
this.trfee = value;
return this;
}
/**
* Gets the value of the pdctTrf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pdctTrf property.
*
*
* For example, to add a new item, do as follows:
*
* getPdctTrf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PortfolioTransfer10 }
*
*
* @return
* The value of the pdctTrf property.
*/
public List getPdctTrf() {
if (pdctTrf == null) {
pdctTrf = new ArrayList<>();
}
return this.pdctTrf;
}
/**
* Gets the value of the mktPrctcVrsn property.
*
* @return
* possible object is
* {@link MarketPracticeVersion1 }
*
*/
public MarketPracticeVersion1 getMktPrctcVrsn() {
return mktPrctcVrsn;
}
/**
* Sets the value of the mktPrctcVrsn property.
*
* @param value
* allowed object is
* {@link MarketPracticeVersion1 }
*
*/
public PortfolioTransferConfirmationV11 setMktPrctcVrsn(MarketPracticeVersion1 value) {
this.mktPrctcVrsn = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the othrIndvInvstr list.
* @see #getOthrIndvInvstr()
*
*/
public PortfolioTransferConfirmationV11 addOthrIndvInvstr(IndividualPerson8 othrIndvInvstr) {
getOthrIndvInvstr().add(othrIndvInvstr);
return this;
}
/**
* Adds a new item to the othrCorpInvstr list.
* @see #getOthrCorpInvstr()
*
*/
public PortfolioTransferConfirmationV11 addOthrCorpInvstr(Organisation36 othrCorpInvstr) {
getOthrCorpInvstr().add(othrCorpInvstr);
return this;
}
/**
* Adds a new item to the pdctTrf list.
* @see #getPdctTrf()
*
*/
public PortfolioTransferConfirmationV11 addPdctTrf(PortfolioTransfer10 pdctTrf) {
getPdctTrf().add(pdctTrf);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public PortfolioTransferConfirmationV11 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}