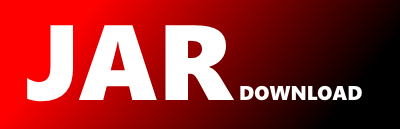
com.prowidesoftware.swift.model.mx.dic.PortfolioTransferInstructionV08 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The PortfolioTransferInstruction message is sent by an instructing party, for example, a (new) plan manager (transferee), to the executing party, for example, a (old) plan manager (transferor), on behalf of the initiating party, for example, an investor (client), to instruct the transfer of financial instruments from the client’s account at the old plan manager (transferor) to the clients account at the new plan manager (transferee) through a nominee account.
* Usage
* The PortfolioTransferInstruction message is used to instruct the withdrawal of one or more ISA or portfolio products from one account and deliver them to another account.
* The PortfolioTransferInstruction message is used to instruct one or more transfers for one client. Each transfer is for delivery to the same account. The account may be owned by one or more individual investors or one or more corporate investors. Each transfer is identified in TransferIdentification.
* If the instructing party does not have enough information to instruct the transfer, then it must first send a AccountHoldingInformationRequest message to the executing party in order to receive a AccountHoldingInformation message.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PortfolioTransferInstructionV08", propOrder = {
"msgRef",
"poolRef",
"prvsRef",
"rltdRef",
"pmryIndvInvstr",
"scndryIndvInvstr",
"othrIndvInvstr",
"pmryCorpInvstr",
"scndryCorpInvstr",
"othrCorpInvstr",
"trfrAcct",
"nmneeAcct",
"trfee",
"intrmyInf",
"cshAcct",
"pdctTrf",
"mktPrctcVrsn",
"xtnsn"
})
public class PortfolioTransferInstructionV08 {
@XmlElement(name = "MsgRef", required = true)
protected MessageIdentification1 msgRef;
@XmlElement(name = "PoolRef")
protected AdditionalReference9 poolRef;
@XmlElement(name = "PrvsRef")
protected AdditionalReference8 prvsRef;
@XmlElement(name = "RltdRef")
protected AdditionalReference8 rltdRef;
@XmlElement(name = "PmryIndvInvstr")
protected IndividualPerson8 pmryIndvInvstr;
@XmlElement(name = "ScndryIndvInvstr")
protected IndividualPerson8 scndryIndvInvstr;
@XmlElement(name = "OthrIndvInvstr")
protected List othrIndvInvstr;
@XmlElement(name = "PmryCorpInvstr")
protected Organisation31 pmryCorpInvstr;
@XmlElement(name = "ScndryCorpInvstr")
protected Organisation31 scndryCorpInvstr;
@XmlElement(name = "OthrCorpInvstr")
protected List othrCorpInvstr;
@XmlElement(name = "TrfrAcct", required = true)
protected Account24 trfrAcct;
@XmlElement(name = "NmneeAcct")
protected Account24 nmneeAcct;
@XmlElement(name = "Trfee", required = true)
protected PartyIdentification113 trfee;
@XmlElement(name = "IntrmyInf")
protected List intrmyInf;
@XmlElement(name = "CshAcct")
protected CashAccount201 cshAcct;
@XmlElement(name = "PdctTrf", required = true)
protected List pdctTrf;
@XmlElement(name = "MktPrctcVrsn")
protected MarketPracticeVersion1 mktPrctcVrsn;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgRef property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getMsgRef() {
return msgRef;
}
/**
* Sets the value of the msgRef property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public PortfolioTransferInstructionV08 setMsgRef(MessageIdentification1 value) {
this.msgRef = value;
return this;
}
/**
* Gets the value of the poolRef property.
*
* @return
* possible object is
* {@link AdditionalReference9 }
*
*/
public AdditionalReference9 getPoolRef() {
return poolRef;
}
/**
* Sets the value of the poolRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference9 }
*
*/
public PortfolioTransferInstructionV08 setPoolRef(AdditionalReference9 value) {
this.poolRef = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
* @return
* possible object is
* {@link AdditionalReference8 }
*
*/
public AdditionalReference8 getPrvsRef() {
return prvsRef;
}
/**
* Sets the value of the prvsRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference8 }
*
*/
public PortfolioTransferInstructionV08 setPrvsRef(AdditionalReference8 value) {
this.prvsRef = value;
return this;
}
/**
* Gets the value of the rltdRef property.
*
* @return
* possible object is
* {@link AdditionalReference8 }
*
*/
public AdditionalReference8 getRltdRef() {
return rltdRef;
}
/**
* Sets the value of the rltdRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference8 }
*
*/
public PortfolioTransferInstructionV08 setRltdRef(AdditionalReference8 value) {
this.rltdRef = value;
return this;
}
/**
* Gets the value of the pmryIndvInvstr property.
*
* @return
* possible object is
* {@link IndividualPerson8 }
*
*/
public IndividualPerson8 getPmryIndvInvstr() {
return pmryIndvInvstr;
}
/**
* Sets the value of the pmryIndvInvstr property.
*
* @param value
* allowed object is
* {@link IndividualPerson8 }
*
*/
public PortfolioTransferInstructionV08 setPmryIndvInvstr(IndividualPerson8 value) {
this.pmryIndvInvstr = value;
return this;
}
/**
* Gets the value of the scndryIndvInvstr property.
*
* @return
* possible object is
* {@link IndividualPerson8 }
*
*/
public IndividualPerson8 getScndryIndvInvstr() {
return scndryIndvInvstr;
}
/**
* Sets the value of the scndryIndvInvstr property.
*
* @param value
* allowed object is
* {@link IndividualPerson8 }
*
*/
public PortfolioTransferInstructionV08 setScndryIndvInvstr(IndividualPerson8 value) {
this.scndryIndvInvstr = value;
return this;
}
/**
* Gets the value of the othrIndvInvstr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrIndvInvstr property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrIndvInvstr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IndividualPerson8 }
*
*
* @return
* The value of the othrIndvInvstr property.
*/
public List getOthrIndvInvstr() {
if (othrIndvInvstr == null) {
othrIndvInvstr = new ArrayList<>();
}
return this.othrIndvInvstr;
}
/**
* Gets the value of the pmryCorpInvstr property.
*
* @return
* possible object is
* {@link Organisation31 }
*
*/
public Organisation31 getPmryCorpInvstr() {
return pmryCorpInvstr;
}
/**
* Sets the value of the pmryCorpInvstr property.
*
* @param value
* allowed object is
* {@link Organisation31 }
*
*/
public PortfolioTransferInstructionV08 setPmryCorpInvstr(Organisation31 value) {
this.pmryCorpInvstr = value;
return this;
}
/**
* Gets the value of the scndryCorpInvstr property.
*
* @return
* possible object is
* {@link Organisation31 }
*
*/
public Organisation31 getScndryCorpInvstr() {
return scndryCorpInvstr;
}
/**
* Sets the value of the scndryCorpInvstr property.
*
* @param value
* allowed object is
* {@link Organisation31 }
*
*/
public PortfolioTransferInstructionV08 setScndryCorpInvstr(Organisation31 value) {
this.scndryCorpInvstr = value;
return this;
}
/**
* Gets the value of the othrCorpInvstr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrCorpInvstr property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrCorpInvstr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Organisation31 }
*
*
* @return
* The value of the othrCorpInvstr property.
*/
public List getOthrCorpInvstr() {
if (othrCorpInvstr == null) {
othrCorpInvstr = new ArrayList<>();
}
return this.othrCorpInvstr;
}
/**
* Gets the value of the trfrAcct property.
*
* @return
* possible object is
* {@link Account24 }
*
*/
public Account24 getTrfrAcct() {
return trfrAcct;
}
/**
* Sets the value of the trfrAcct property.
*
* @param value
* allowed object is
* {@link Account24 }
*
*/
public PortfolioTransferInstructionV08 setTrfrAcct(Account24 value) {
this.trfrAcct = value;
return this;
}
/**
* Gets the value of the nmneeAcct property.
*
* @return
* possible object is
* {@link Account24 }
*
*/
public Account24 getNmneeAcct() {
return nmneeAcct;
}
/**
* Sets the value of the nmneeAcct property.
*
* @param value
* allowed object is
* {@link Account24 }
*
*/
public PortfolioTransferInstructionV08 setNmneeAcct(Account24 value) {
this.nmneeAcct = value;
return this;
}
/**
* Gets the value of the trfee property.
*
* @return
* possible object is
* {@link PartyIdentification113 }
*
*/
public PartyIdentification113 getTrfee() {
return trfee;
}
/**
* Sets the value of the trfee property.
*
* @param value
* allowed object is
* {@link PartyIdentification113 }
*
*/
public PortfolioTransferInstructionV08 setTrfee(PartyIdentification113 value) {
this.trfee = value;
return this;
}
/**
* Gets the value of the intrmyInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrmyInf property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrmyInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary41 }
*
*
* @return
* The value of the intrmyInf property.
*/
public List getIntrmyInf() {
if (intrmyInf == null) {
intrmyInf = new ArrayList<>();
}
return this.intrmyInf;
}
/**
* Gets the value of the cshAcct property.
*
* @return
* possible object is
* {@link CashAccount201 }
*
*/
public CashAccount201 getCshAcct() {
return cshAcct;
}
/**
* Sets the value of the cshAcct property.
*
* @param value
* allowed object is
* {@link CashAccount201 }
*
*/
public PortfolioTransferInstructionV08 setCshAcct(CashAccount201 value) {
this.cshAcct = value;
return this;
}
/**
* Gets the value of the pdctTrf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pdctTrf property.
*
*
* For example, to add a new item, do as follows:
*
* getPdctTrf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ISATransfer29 }
*
*
* @return
* The value of the pdctTrf property.
*/
public List getPdctTrf() {
if (pdctTrf == null) {
pdctTrf = new ArrayList<>();
}
return this.pdctTrf;
}
/**
* Gets the value of the mktPrctcVrsn property.
*
* @return
* possible object is
* {@link MarketPracticeVersion1 }
*
*/
public MarketPracticeVersion1 getMktPrctcVrsn() {
return mktPrctcVrsn;
}
/**
* Sets the value of the mktPrctcVrsn property.
*
* @param value
* allowed object is
* {@link MarketPracticeVersion1 }
*
*/
public PortfolioTransferInstructionV08 setMktPrctcVrsn(MarketPracticeVersion1 value) {
this.mktPrctcVrsn = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the othrIndvInvstr list.
* @see #getOthrIndvInvstr()
*
*/
public PortfolioTransferInstructionV08 addOthrIndvInvstr(IndividualPerson8 othrIndvInvstr) {
getOthrIndvInvstr().add(othrIndvInvstr);
return this;
}
/**
* Adds a new item to the othrCorpInvstr list.
* @see #getOthrCorpInvstr()
*
*/
public PortfolioTransferInstructionV08 addOthrCorpInvstr(Organisation31 othrCorpInvstr) {
getOthrCorpInvstr().add(othrCorpInvstr);
return this;
}
/**
* Adds a new item to the intrmyInf list.
* @see #getIntrmyInf()
*
*/
public PortfolioTransferInstructionV08 addIntrmyInf(Intermediary41 intrmyInf) {
getIntrmyInf().add(intrmyInf);
return this;
}
/**
* Adds a new item to the pdctTrf list.
* @see #getPdctTrf()
*
*/
public PortfolioTransferInstructionV08 addPdctTrf(ISATransfer29 pdctTrf) {
getPdctTrf().add(pdctTrf);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public PortfolioTransferInstructionV08 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}