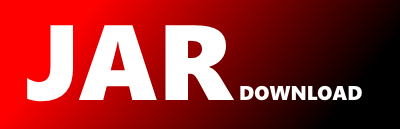
com.prowidesoftware.swift.model.mx.dic.PortfolioTransferNotificationV02 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An account servicer sends a PortfolioTransferNotification to another account servicer to exchange transfer settlement details information during a retail or institutional client portfolio transfers.
* The account servicers will typically be local agents or global custodians acting on behalf of an investment management institution, a broker/dealer or a retail client.
* Usage
* By exchange of transfer settlement details, it is understood the providing, by the delivering account servicer to the receiving account servicer, of the settlement details (trade date, settlement date, delivering settlement chain, quantities, etc.) of the individual transfers that will take place during a full or partial portfolio transfer. This delivering account servicer message may also include, for validation, the receiving settlement chain as provided by the client. In case the receiving settlement chain is not available to the delivering account servicer, the receiving account servicer may in return provide to the delivering account servicer the receiving settlement chain using the same message.
* The message may also be used to:
* - re-send a message previously sent,
* - provide a third party with a copy of a message for information,
* - re-send to a third party a copy of a message for information.
* using the relevant elements in the Business Application Header.
* ISO 15022 - 20022 Coexistence
* This ISO 20022 message is reversed engineered from ISO 15022. Both standards will coexist for a certain number of years. Until this coexistence period ends, the usage of certain data types is restricted to ensure interoperability between ISO 15022 and 20022 users. Compliance to these rules is mandatory in a coexistence environment. The coexistence restrictions are described in a Textual Rule linked to the Message Items they concern. These coexistence textual rules are clearly identified as follows: “CoexistenceXxxxRule”.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PortfolioTransferNotificationV02", propOrder = {
"pgntn",
"stmtGnlDtls",
"acctOwnr",
"sfkpgAcct",
"trfNtfctnDtls"
})
public class PortfolioTransferNotificationV02 {
@XmlElement(name = "Pgntn", required = true)
protected Pagination pgntn;
@XmlElement(name = "StmtGnlDtls", required = true)
protected Statement19 stmtGnlDtls;
@XmlElement(name = "AcctOwnr")
protected PartyIdentification36Choice acctOwnr;
@XmlElement(name = "SfkpgAcct", required = true)
protected SecuritiesAccount13 sfkpgAcct;
@XmlElement(name = "TrfNtfctnDtls")
protected List trfNtfctnDtls;
/**
* Gets the value of the pgntn property.
*
* @return
* possible object is
* {@link Pagination }
*
*/
public Pagination getPgntn() {
return pgntn;
}
/**
* Sets the value of the pgntn property.
*
* @param value
* allowed object is
* {@link Pagination }
*
*/
public PortfolioTransferNotificationV02 setPgntn(Pagination value) {
this.pgntn = value;
return this;
}
/**
* Gets the value of the stmtGnlDtls property.
*
* @return
* possible object is
* {@link Statement19 }
*
*/
public Statement19 getStmtGnlDtls() {
return stmtGnlDtls;
}
/**
* Sets the value of the stmtGnlDtls property.
*
* @param value
* allowed object is
* {@link Statement19 }
*
*/
public PortfolioTransferNotificationV02 setStmtGnlDtls(Statement19 value) {
this.stmtGnlDtls = value;
return this;
}
/**
* Gets the value of the acctOwnr property.
*
* @return
* possible object is
* {@link PartyIdentification36Choice }
*
*/
public PartyIdentification36Choice getAcctOwnr() {
return acctOwnr;
}
/**
* Sets the value of the acctOwnr property.
*
* @param value
* allowed object is
* {@link PartyIdentification36Choice }
*
*/
public PortfolioTransferNotificationV02 setAcctOwnr(PartyIdentification36Choice value) {
this.acctOwnr = value;
return this;
}
/**
* Gets the value of the sfkpgAcct property.
*
* @return
* possible object is
* {@link SecuritiesAccount13 }
*
*/
public SecuritiesAccount13 getSfkpgAcct() {
return sfkpgAcct;
}
/**
* Sets the value of the sfkpgAcct property.
*
* @param value
* allowed object is
* {@link SecuritiesAccount13 }
*
*/
public PortfolioTransferNotificationV02 setSfkpgAcct(SecuritiesAccount13 value) {
this.sfkpgAcct = value;
return this;
}
/**
* Gets the value of the trfNtfctnDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the trfNtfctnDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getTrfNtfctnDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SecuritiesTradeDetails19 }
*
*
* @return
* The value of the trfNtfctnDtls property.
*/
public List getTrfNtfctnDtls() {
if (trfNtfctnDtls == null) {
trfNtfctnDtls = new ArrayList<>();
}
return this.trfNtfctnDtls;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the trfNtfctnDtls list.
* @see #getTrfNtfctnDtls()
*
*/
public PortfolioTransferNotificationV02 addTrfNtfctnDtls(SecuritiesTradeDetails19 trfNtfctnDtls) {
getTrfNtfctnDtls().add(trfNtfctnDtls);
return this;
}
}