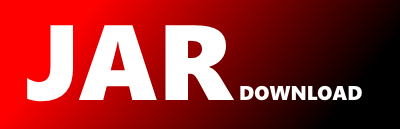
com.prowidesoftware.swift.model.mx.dic.PostalAddress27 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Information that locates and identifies a specific address, as defined by postal services.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PostalAddress27", propOrder = {
"adrTp",
"careOf",
"dept",
"subDept",
"strtNm",
"bldgNb",
"bldgNm",
"flr",
"unitNb",
"pstBx",
"room",
"pstCd",
"twnNm",
"twnLctnNm",
"dstrctNm",
"ctrySubDvsn",
"ctry",
"adrLine"
})
public class PostalAddress27 {
@XmlElement(name = "AdrTp")
protected AddressType3Choice adrTp;
@XmlElement(name = "CareOf")
protected String careOf;
@XmlElement(name = "Dept")
protected String dept;
@XmlElement(name = "SubDept")
protected String subDept;
@XmlElement(name = "StrtNm")
protected String strtNm;
@XmlElement(name = "BldgNb")
protected String bldgNb;
@XmlElement(name = "BldgNm")
protected String bldgNm;
@XmlElement(name = "Flr")
protected String flr;
@XmlElement(name = "UnitNb")
protected String unitNb;
@XmlElement(name = "PstBx")
protected String pstBx;
@XmlElement(name = "Room")
protected String room;
@XmlElement(name = "PstCd")
protected String pstCd;
@XmlElement(name = "TwnNm")
protected String twnNm;
@XmlElement(name = "TwnLctnNm")
protected String twnLctnNm;
@XmlElement(name = "DstrctNm")
protected String dstrctNm;
@XmlElement(name = "CtrySubDvsn")
protected String ctrySubDvsn;
@XmlElement(name = "Ctry")
protected String ctry;
@XmlElement(name = "AdrLine")
protected List adrLine;
/**
* Gets the value of the adrTp property.
*
* @return
* possible object is
* {@link AddressType3Choice }
*
*/
public AddressType3Choice getAdrTp() {
return adrTp;
}
/**
* Sets the value of the adrTp property.
*
* @param value
* allowed object is
* {@link AddressType3Choice }
*
*/
public PostalAddress27 setAdrTp(AddressType3Choice value) {
this.adrTp = value;
return this;
}
/**
* Gets the value of the careOf property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCareOf() {
return careOf;
}
/**
* Sets the value of the careOf property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setCareOf(String value) {
this.careOf = value;
return this;
}
/**
* Gets the value of the dept property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDept() {
return dept;
}
/**
* Sets the value of the dept property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setDept(String value) {
this.dept = value;
return this;
}
/**
* Gets the value of the subDept property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSubDept() {
return subDept;
}
/**
* Sets the value of the subDept property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setSubDept(String value) {
this.subDept = value;
return this;
}
/**
* Gets the value of the strtNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStrtNm() {
return strtNm;
}
/**
* Sets the value of the strtNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setStrtNm(String value) {
this.strtNm = value;
return this;
}
/**
* Gets the value of the bldgNb property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBldgNb() {
return bldgNb;
}
/**
* Sets the value of the bldgNb property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setBldgNb(String value) {
this.bldgNb = value;
return this;
}
/**
* Gets the value of the bldgNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBldgNm() {
return bldgNm;
}
/**
* Sets the value of the bldgNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setBldgNm(String value) {
this.bldgNm = value;
return this;
}
/**
* Gets the value of the flr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFlr() {
return flr;
}
/**
* Sets the value of the flr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setFlr(String value) {
this.flr = value;
return this;
}
/**
* Gets the value of the unitNb property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnitNb() {
return unitNb;
}
/**
* Sets the value of the unitNb property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setUnitNb(String value) {
this.unitNb = value;
return this;
}
/**
* Gets the value of the pstBx property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPstBx() {
return pstBx;
}
/**
* Sets the value of the pstBx property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setPstBx(String value) {
this.pstBx = value;
return this;
}
/**
* Gets the value of the room property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoom() {
return room;
}
/**
* Sets the value of the room property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setRoom(String value) {
this.room = value;
return this;
}
/**
* Gets the value of the pstCd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPstCd() {
return pstCd;
}
/**
* Sets the value of the pstCd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setPstCd(String value) {
this.pstCd = value;
return this;
}
/**
* Gets the value of the twnNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTwnNm() {
return twnNm;
}
/**
* Sets the value of the twnNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setTwnNm(String value) {
this.twnNm = value;
return this;
}
/**
* Gets the value of the twnLctnNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTwnLctnNm() {
return twnLctnNm;
}
/**
* Sets the value of the twnLctnNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setTwnLctnNm(String value) {
this.twnLctnNm = value;
return this;
}
/**
* Gets the value of the dstrctNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDstrctNm() {
return dstrctNm;
}
/**
* Sets the value of the dstrctNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setDstrctNm(String value) {
this.dstrctNm = value;
return this;
}
/**
* Gets the value of the ctrySubDvsn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCtrySubDvsn() {
return ctrySubDvsn;
}
/**
* Sets the value of the ctrySubDvsn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setCtrySubDvsn(String value) {
this.ctrySubDvsn = value;
return this;
}
/**
* Gets the value of the ctry property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCtry() {
return ctry;
}
/**
* Sets the value of the ctry property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public PostalAddress27 setCtry(String value) {
this.ctry = value;
return this;
}
/**
* Gets the value of the adrLine property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the adrLine property.
*
*
* For example, to add a new item, do as follows:
*
* getAdrLine().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the adrLine property.
*/
public List getAdrLine() {
if (adrLine == null) {
adrLine = new ArrayList<>();
}
return this.adrLine;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the adrLine list.
* @see #getAdrLine()
*
*/
public PostalAddress27 addAdrLine(String adrLine) {
getAdrLine().add(adrLine);
return this;
}
}