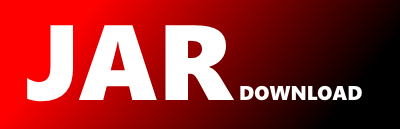
com.prowidesoftware.swift.model.mx.dic.RedemptionOrder14 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Instruction from an investor to sell investment fund units back to the fund.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RedemptionOrder14", propOrder = {
"ordrRef",
"clntRef",
"ordrTp",
"finInstrmDtls",
"subAcctForHldg",
"amtOrUnitsOrPctg",
"rndg",
"sttlmAmt",
"cshSttlmDt",
"sttlmMtd",
"fxDtls",
"incmPref",
"grp1Or2Units",
"txOvrhd",
"sttlmAndCtdyDtls",
"physDlvryInd",
"physDlvryDtls",
"reqdSttlmCcy",
"reqdNAVCcy",
"cshSttlmDtls",
"nonStdSttlmInf",
"stffClntBrkdwn",
"finAdvc",
"ngtdTrad",
"rltdPtyDtls",
"equlstn",
"cstmrCndctClssfctn",
"txChanlTp",
"sgntrTp",
"ordrWvrDtls"
})
public class RedemptionOrder14 {
@XmlElement(name = "OrdrRef", required = true)
protected String ordrRef;
@XmlElement(name = "ClntRef")
protected String clntRef;
@XmlElement(name = "OrdrTp")
protected List ordrTp;
@XmlElement(name = "FinInstrmDtls", required = true)
protected FinancialInstrument57 finInstrmDtls;
@XmlElement(name = "SubAcctForHldg")
protected SubAccount6 subAcctForHldg;
@XmlElement(name = "AmtOrUnitsOrPctg", required = true)
protected FinancialInstrumentQuantity28Choice amtOrUnitsOrPctg;
@XmlElement(name = "Rndg")
@XmlSchemaType(name = "string")
protected RoundingDirection2Code rndg;
@XmlElement(name = "SttlmAmt")
protected ActiveCurrencyAndAmount sttlmAmt;
@XmlElement(name = "CshSttlmDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate cshSttlmDt;
@XmlElement(name = "SttlmMtd")
@XmlSchemaType(name = "string")
protected DeliveryReceiptType2Code sttlmMtd;
@XmlElement(name = "FXDtls")
protected ForeignExchangeTerms32 fxDtls;
@XmlElement(name = "IncmPref")
@XmlSchemaType(name = "string")
protected IncomePreference1Code incmPref;
@XmlElement(name = "Grp1Or2Units")
@XmlSchemaType(name = "string")
protected UKTaxGroupUnit1Code grp1Or2Units;
@XmlElement(name = "TxOvrhd")
protected FeeAndTax1 txOvrhd;
@XmlElement(name = "SttlmAndCtdyDtls")
protected FundSettlementParameters12 sttlmAndCtdyDtls;
@XmlElement(name = "PhysDlvryInd")
protected boolean physDlvryInd;
@XmlElement(name = "PhysDlvryDtls")
protected DeliveryParameters3 physDlvryDtls;
@XmlElement(name = "ReqdSttlmCcy")
protected String reqdSttlmCcy;
@XmlElement(name = "ReqdNAVCcy")
protected String reqdNAVCcy;
@XmlElement(name = "CshSttlmDtls")
protected PaymentTransaction72 cshSttlmDtls;
@XmlElement(name = "NonStdSttlmInf")
protected String nonStdSttlmInf;
@XmlElement(name = "StffClntBrkdwn")
protected List stffClntBrkdwn;
@XmlElement(name = "FinAdvc")
@XmlSchemaType(name = "string")
protected FinancialAdvice1Code finAdvc;
@XmlElement(name = "NgtdTrad")
@XmlSchemaType(name = "string")
protected NegotiatedTrade1Code ngtdTrad;
@XmlElement(name = "RltdPtyDtls")
protected List rltdPtyDtls;
@XmlElement(name = "Equlstn")
protected Equalisation1 equlstn;
@XmlElement(name = "CstmrCndctClssfctn")
protected CustomerConductClassification1Choice cstmrCndctClssfctn;
@XmlElement(name = "TxChanlTp")
protected TransactionChannelType1Choice txChanlTp;
@XmlElement(name = "SgntrTp")
protected SignatureType1Choice sgntrTp;
@XmlElement(name = "OrdrWvrDtls")
protected OrderWaiver1 ordrWvrDtls;
/**
* Gets the value of the ordrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrdrRef() {
return ordrRef;
}
/**
* Sets the value of the ordrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder14 setOrdrRef(String value) {
this.ordrRef = value;
return this;
}
/**
* Gets the value of the clntRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClntRef() {
return clntRef;
}
/**
* Sets the value of the clntRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder14 setClntRef(String value) {
this.clntRef = value;
return this;
}
/**
* Gets the value of the ordrTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the ordrTp property.
*
*
* For example, to add a new item, do as follows:
*
* getOrdrTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FundOrderType4Choice }
*
*
* @return
* The value of the ordrTp property.
*/
public List getOrdrTp() {
if (ordrTp == null) {
ordrTp = new ArrayList<>();
}
return this.ordrTp;
}
/**
* Gets the value of the finInstrmDtls property.
*
* @return
* possible object is
* {@link FinancialInstrument57 }
*
*/
public FinancialInstrument57 getFinInstrmDtls() {
return finInstrmDtls;
}
/**
* Sets the value of the finInstrmDtls property.
*
* @param value
* allowed object is
* {@link FinancialInstrument57 }
*
*/
public RedemptionOrder14 setFinInstrmDtls(FinancialInstrument57 value) {
this.finInstrmDtls = value;
return this;
}
/**
* Gets the value of the subAcctForHldg property.
*
* @return
* possible object is
* {@link SubAccount6 }
*
*/
public SubAccount6 getSubAcctForHldg() {
return subAcctForHldg;
}
/**
* Sets the value of the subAcctForHldg property.
*
* @param value
* allowed object is
* {@link SubAccount6 }
*
*/
public RedemptionOrder14 setSubAcctForHldg(SubAccount6 value) {
this.subAcctForHldg = value;
return this;
}
/**
* Gets the value of the amtOrUnitsOrPctg property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity28Choice }
*
*/
public FinancialInstrumentQuantity28Choice getAmtOrUnitsOrPctg() {
return amtOrUnitsOrPctg;
}
/**
* Sets the value of the amtOrUnitsOrPctg property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity28Choice }
*
*/
public RedemptionOrder14 setAmtOrUnitsOrPctg(FinancialInstrumentQuantity28Choice value) {
this.amtOrUnitsOrPctg = value;
return this;
}
/**
* Gets the value of the rndg property.
*
* @return
* possible object is
* {@link RoundingDirection2Code }
*
*/
public RoundingDirection2Code getRndg() {
return rndg;
}
/**
* Sets the value of the rndg property.
*
* @param value
* allowed object is
* {@link RoundingDirection2Code }
*
*/
public RedemptionOrder14 setRndg(RoundingDirection2Code value) {
this.rndg = value;
return this;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public RedemptionOrder14 setSttlmAmt(ActiveCurrencyAndAmount value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the cshSttlmDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getCshSttlmDt() {
return cshSttlmDt;
}
/**
* Sets the value of the cshSttlmDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder14 setCshSttlmDt(LocalDate value) {
this.cshSttlmDt = value;
return this;
}
/**
* Gets the value of the sttlmMtd property.
*
* @return
* possible object is
* {@link DeliveryReceiptType2Code }
*
*/
public DeliveryReceiptType2Code getSttlmMtd() {
return sttlmMtd;
}
/**
* Sets the value of the sttlmMtd property.
*
* @param value
* allowed object is
* {@link DeliveryReceiptType2Code }
*
*/
public RedemptionOrder14 setSttlmMtd(DeliveryReceiptType2Code value) {
this.sttlmMtd = value;
return this;
}
/**
* Gets the value of the fxDtls property.
*
* @return
* possible object is
* {@link ForeignExchangeTerms32 }
*
*/
public ForeignExchangeTerms32 getFXDtls() {
return fxDtls;
}
/**
* Sets the value of the fxDtls property.
*
* @param value
* allowed object is
* {@link ForeignExchangeTerms32 }
*
*/
public RedemptionOrder14 setFXDtls(ForeignExchangeTerms32 value) {
this.fxDtls = value;
return this;
}
/**
* Gets the value of the incmPref property.
*
* @return
* possible object is
* {@link IncomePreference1Code }
*
*/
public IncomePreference1Code getIncmPref() {
return incmPref;
}
/**
* Sets the value of the incmPref property.
*
* @param value
* allowed object is
* {@link IncomePreference1Code }
*
*/
public RedemptionOrder14 setIncmPref(IncomePreference1Code value) {
this.incmPref = value;
return this;
}
/**
* Gets the value of the grp1Or2Units property.
*
* @return
* possible object is
* {@link UKTaxGroupUnit1Code }
*
*/
public UKTaxGroupUnit1Code getGrp1Or2Units() {
return grp1Or2Units;
}
/**
* Sets the value of the grp1Or2Units property.
*
* @param value
* allowed object is
* {@link UKTaxGroupUnit1Code }
*
*/
public RedemptionOrder14 setGrp1Or2Units(UKTaxGroupUnit1Code value) {
this.grp1Or2Units = value;
return this;
}
/**
* Gets the value of the txOvrhd property.
*
* @return
* possible object is
* {@link FeeAndTax1 }
*
*/
public FeeAndTax1 getTxOvrhd() {
return txOvrhd;
}
/**
* Sets the value of the txOvrhd property.
*
* @param value
* allowed object is
* {@link FeeAndTax1 }
*
*/
public RedemptionOrder14 setTxOvrhd(FeeAndTax1 value) {
this.txOvrhd = value;
return this;
}
/**
* Gets the value of the sttlmAndCtdyDtls property.
*
* @return
* possible object is
* {@link FundSettlementParameters12 }
*
*/
public FundSettlementParameters12 getSttlmAndCtdyDtls() {
return sttlmAndCtdyDtls;
}
/**
* Sets the value of the sttlmAndCtdyDtls property.
*
* @param value
* allowed object is
* {@link FundSettlementParameters12 }
*
*/
public RedemptionOrder14 setSttlmAndCtdyDtls(FundSettlementParameters12 value) {
this.sttlmAndCtdyDtls = value;
return this;
}
/**
* Gets the value of the physDlvryInd property.
*
*/
public boolean isPhysDlvryInd() {
return physDlvryInd;
}
/**
* Sets the value of the physDlvryInd property.
*
*/
public RedemptionOrder14 setPhysDlvryInd(boolean value) {
this.physDlvryInd = value;
return this;
}
/**
* Gets the value of the physDlvryDtls property.
*
* @return
* possible object is
* {@link DeliveryParameters3 }
*
*/
public DeliveryParameters3 getPhysDlvryDtls() {
return physDlvryDtls;
}
/**
* Sets the value of the physDlvryDtls property.
*
* @param value
* allowed object is
* {@link DeliveryParameters3 }
*
*/
public RedemptionOrder14 setPhysDlvryDtls(DeliveryParameters3 value) {
this.physDlvryDtls = value;
return this;
}
/**
* Gets the value of the reqdSttlmCcy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReqdSttlmCcy() {
return reqdSttlmCcy;
}
/**
* Sets the value of the reqdSttlmCcy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder14 setReqdSttlmCcy(String value) {
this.reqdSttlmCcy = value;
return this;
}
/**
* Gets the value of the reqdNAVCcy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReqdNAVCcy() {
return reqdNAVCcy;
}
/**
* Sets the value of the reqdNAVCcy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder14 setReqdNAVCcy(String value) {
this.reqdNAVCcy = value;
return this;
}
/**
* Gets the value of the cshSttlmDtls property.
*
* @return
* possible object is
* {@link PaymentTransaction72 }
*
*/
public PaymentTransaction72 getCshSttlmDtls() {
return cshSttlmDtls;
}
/**
* Sets the value of the cshSttlmDtls property.
*
* @param value
* allowed object is
* {@link PaymentTransaction72 }
*
*/
public RedemptionOrder14 setCshSttlmDtls(PaymentTransaction72 value) {
this.cshSttlmDtls = value;
return this;
}
/**
* Gets the value of the nonStdSttlmInf property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNonStdSttlmInf() {
return nonStdSttlmInf;
}
/**
* Sets the value of the nonStdSttlmInf property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder14 setNonStdSttlmInf(String value) {
this.nonStdSttlmInf = value;
return this;
}
/**
* Gets the value of the stffClntBrkdwn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the stffClntBrkdwn property.
*
*
* For example, to add a new item, do as follows:
*
* getStffClntBrkdwn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentFundsOrderBreakdown2 }
*
*
* @return
* The value of the stffClntBrkdwn property.
*/
public List getStffClntBrkdwn() {
if (stffClntBrkdwn == null) {
stffClntBrkdwn = new ArrayList<>();
}
return this.stffClntBrkdwn;
}
/**
* Gets the value of the finAdvc property.
*
* @return
* possible object is
* {@link FinancialAdvice1Code }
*
*/
public FinancialAdvice1Code getFinAdvc() {
return finAdvc;
}
/**
* Sets the value of the finAdvc property.
*
* @param value
* allowed object is
* {@link FinancialAdvice1Code }
*
*/
public RedemptionOrder14 setFinAdvc(FinancialAdvice1Code value) {
this.finAdvc = value;
return this;
}
/**
* Gets the value of the ngtdTrad property.
*
* @return
* possible object is
* {@link NegotiatedTrade1Code }
*
*/
public NegotiatedTrade1Code getNgtdTrad() {
return ngtdTrad;
}
/**
* Sets the value of the ngtdTrad property.
*
* @param value
* allowed object is
* {@link NegotiatedTrade1Code }
*
*/
public RedemptionOrder14 setNgtdTrad(NegotiatedTrade1Code value) {
this.ngtdTrad = value;
return this;
}
/**
* Gets the value of the rltdPtyDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rltdPtyDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getRltdPtyDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary40 }
*
*
* @return
* The value of the rltdPtyDtls property.
*/
public List getRltdPtyDtls() {
if (rltdPtyDtls == null) {
rltdPtyDtls = new ArrayList<>();
}
return this.rltdPtyDtls;
}
/**
* Gets the value of the equlstn property.
*
* @return
* possible object is
* {@link Equalisation1 }
*
*/
public Equalisation1 getEqulstn() {
return equlstn;
}
/**
* Sets the value of the equlstn property.
*
* @param value
* allowed object is
* {@link Equalisation1 }
*
*/
public RedemptionOrder14 setEqulstn(Equalisation1 value) {
this.equlstn = value;
return this;
}
/**
* Gets the value of the cstmrCndctClssfctn property.
*
* @return
* possible object is
* {@link CustomerConductClassification1Choice }
*
*/
public CustomerConductClassification1Choice getCstmrCndctClssfctn() {
return cstmrCndctClssfctn;
}
/**
* Sets the value of the cstmrCndctClssfctn property.
*
* @param value
* allowed object is
* {@link CustomerConductClassification1Choice }
*
*/
public RedemptionOrder14 setCstmrCndctClssfctn(CustomerConductClassification1Choice value) {
this.cstmrCndctClssfctn = value;
return this;
}
/**
* Gets the value of the txChanlTp property.
*
* @return
* possible object is
* {@link TransactionChannelType1Choice }
*
*/
public TransactionChannelType1Choice getTxChanlTp() {
return txChanlTp;
}
/**
* Sets the value of the txChanlTp property.
*
* @param value
* allowed object is
* {@link TransactionChannelType1Choice }
*
*/
public RedemptionOrder14 setTxChanlTp(TransactionChannelType1Choice value) {
this.txChanlTp = value;
return this;
}
/**
* Gets the value of the sgntrTp property.
*
* @return
* possible object is
* {@link SignatureType1Choice }
*
*/
public SignatureType1Choice getSgntrTp() {
return sgntrTp;
}
/**
* Sets the value of the sgntrTp property.
*
* @param value
* allowed object is
* {@link SignatureType1Choice }
*
*/
public RedemptionOrder14 setSgntrTp(SignatureType1Choice value) {
this.sgntrTp = value;
return this;
}
/**
* Gets the value of the ordrWvrDtls property.
*
* @return
* possible object is
* {@link OrderWaiver1 }
*
*/
public OrderWaiver1 getOrdrWvrDtls() {
return ordrWvrDtls;
}
/**
* Sets the value of the ordrWvrDtls property.
*
* @param value
* allowed object is
* {@link OrderWaiver1 }
*
*/
public RedemptionOrder14 setOrdrWvrDtls(OrderWaiver1 value) {
this.ordrWvrDtls = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the ordrTp list.
* @see #getOrdrTp()
*
*/
public RedemptionOrder14 addOrdrTp(FundOrderType4Choice ordrTp) {
getOrdrTp().add(ordrTp);
return this;
}
/**
* Adds a new item to the stffClntBrkdwn list.
* @see #getStffClntBrkdwn()
*
*/
public RedemptionOrder14 addStffClntBrkdwn(InvestmentFundsOrderBreakdown2 stffClntBrkdwn) {
getStffClntBrkdwn().add(stffClntBrkdwn);
return this;
}
/**
* Adds a new item to the rltdPtyDtls list.
* @see #getRltdPtyDtls()
*
*/
public RedemptionOrder14 addRltdPtyDtls(Intermediary40 rltdPtyDtls) {
getRltdPtyDtls().add(rltdPtyDtls);
return this;
}
}