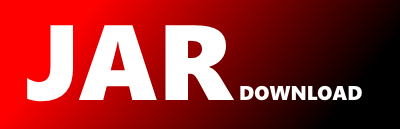
com.prowidesoftware.swift.model.mx.dic.RedemptionOrder7 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Instruction from an investor to sell investment fund units back to the fund.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RedemptionOrder7", propOrder = {
"ordrRef",
"clntRef",
"invstmtAcctDtls",
"ordrTp",
"bnfcryDtls",
"unitsNb",
"grssAmt",
"netAmt",
"hldgsRedRate",
"rndg",
"sttlmAmt",
"cshSttlmDt",
"sttlmMtd",
"fxDtls",
"incmPref",
"grp1Or2Units",
"chrgDtls",
"comssnDtls",
"taxDtls",
"sttlmAndCtdyDtls",
"physDlvryInd",
"physDlvryDtls",
"cshSttlmDtls",
"nonStdSttlmInf",
"stffClntBrkdwn",
"finAdvc",
"ngtdTrad",
"rltdPtyDtls",
"equlstn"
})
public class RedemptionOrder7 {
@XmlElement(name = "OrdrRef", required = true)
protected String ordrRef;
@XmlElement(name = "ClntRef")
protected String clntRef;
@XmlElement(name = "InvstmtAcctDtls", required = true)
protected InvestmentAccount21 invstmtAcctDtls;
@XmlElement(name = "OrdrTp")
protected List ordrTp;
@XmlElement(name = "BnfcryDtls")
protected IndividualPerson12 bnfcryDtls;
@XmlElement(name = "UnitsNb")
protected FinancialInstrumentQuantity1 unitsNb;
@XmlElement(name = "GrssAmt")
protected ActiveOrHistoricCurrencyAndAmount grssAmt;
@XmlElement(name = "NetAmt")
protected ActiveOrHistoricCurrencyAndAmount netAmt;
@XmlElement(name = "HldgsRedRate")
protected BigDecimal hldgsRedRate;
@XmlElement(name = "Rndg")
@XmlSchemaType(name = "string")
protected RoundingDirection2Code rndg;
@XmlElement(name = "SttlmAmt")
protected ActiveCurrencyAndAmount sttlmAmt;
@XmlElement(name = "CshSttlmDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate cshSttlmDt;
@XmlElement(name = "SttlmMtd")
@XmlSchemaType(name = "string")
protected DeliveryReceiptType2Code sttlmMtd;
@XmlElement(name = "FXDtls")
protected ForeignExchangeTerms6 fxDtls;
@XmlElement(name = "IncmPref")
@XmlSchemaType(name = "string")
protected IncomePreference1Code incmPref;
@XmlElement(name = "Grp1Or2Units")
@XmlSchemaType(name = "string")
protected UKTaxGroupUnitCode grp1Or2Units;
@XmlElement(name = "ChrgDtls")
protected List chrgDtls;
@XmlElement(name = "ComssnDtls")
protected List comssnDtls;
@XmlElement(name = "TaxDtls")
protected List taxDtls;
@XmlElement(name = "SttlmAndCtdyDtls")
protected FundSettlementParameters3 sttlmAndCtdyDtls;
@XmlElement(name = "PhysDlvryInd")
protected boolean physDlvryInd;
@XmlElement(name = "PhysDlvryDtls")
protected DeliveryParameters3 physDlvryDtls;
@XmlElement(name = "CshSttlmDtls")
protected PaymentTransaction21 cshSttlmDtls;
@XmlElement(name = "NonStdSttlmInf")
protected String nonStdSttlmInf;
@XmlElement(name = "StffClntBrkdwn")
protected List stffClntBrkdwn;
@XmlElement(name = "FinAdvc")
@XmlSchemaType(name = "string")
protected FinancialAdvice1Code finAdvc;
@XmlElement(name = "NgtdTrad")
@XmlSchemaType(name = "string")
protected NegotiatedTrade1Code ngtdTrad;
@XmlElement(name = "RltdPtyDtls")
protected List rltdPtyDtls;
@XmlElement(name = "Equlstn")
protected Equalisation1 equlstn;
/**
* Gets the value of the ordrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrdrRef() {
return ordrRef;
}
/**
* Sets the value of the ordrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder7 setOrdrRef(String value) {
this.ordrRef = value;
return this;
}
/**
* Gets the value of the clntRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClntRef() {
return clntRef;
}
/**
* Sets the value of the clntRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder7 setClntRef(String value) {
this.clntRef = value;
return this;
}
/**
* Gets the value of the invstmtAcctDtls property.
*
* @return
* possible object is
* {@link InvestmentAccount21 }
*
*/
public InvestmentAccount21 getInvstmtAcctDtls() {
return invstmtAcctDtls;
}
/**
* Sets the value of the invstmtAcctDtls property.
*
* @param value
* allowed object is
* {@link InvestmentAccount21 }
*
*/
public RedemptionOrder7 setInvstmtAcctDtls(InvestmentAccount21 value) {
this.invstmtAcctDtls = value;
return this;
}
/**
* Gets the value of the ordrTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the ordrTp property.
*
*
* For example, to add a new item, do as follows:
*
* getOrdrTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FundOrderType2 }
*
*
* @return
* The value of the ordrTp property.
*/
public List getOrdrTp() {
if (ordrTp == null) {
ordrTp = new ArrayList<>();
}
return this.ordrTp;
}
/**
* Gets the value of the bnfcryDtls property.
*
* @return
* possible object is
* {@link IndividualPerson12 }
*
*/
public IndividualPerson12 getBnfcryDtls() {
return bnfcryDtls;
}
/**
* Sets the value of the bnfcryDtls property.
*
* @param value
* allowed object is
* {@link IndividualPerson12 }
*
*/
public RedemptionOrder7 setBnfcryDtls(IndividualPerson12 value) {
this.bnfcryDtls = value;
return this;
}
/**
* Gets the value of the unitsNb property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FinancialInstrumentQuantity1 getUnitsNb() {
return unitsNb;
}
/**
* Sets the value of the unitsNb property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public RedemptionOrder7 setUnitsNb(FinancialInstrumentQuantity1 value) {
this.unitsNb = value;
return this;
}
/**
* Gets the value of the grssAmt property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getGrssAmt() {
return grssAmt;
}
/**
* Sets the value of the grssAmt property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public RedemptionOrder7 setGrssAmt(ActiveOrHistoricCurrencyAndAmount value) {
this.grssAmt = value;
return this;
}
/**
* Gets the value of the netAmt property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getNetAmt() {
return netAmt;
}
/**
* Sets the value of the netAmt property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public RedemptionOrder7 setNetAmt(ActiveOrHistoricCurrencyAndAmount value) {
this.netAmt = value;
return this;
}
/**
* Gets the value of the hldgsRedRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getHldgsRedRate() {
return hldgsRedRate;
}
/**
* Sets the value of the hldgsRedRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public RedemptionOrder7 setHldgsRedRate(BigDecimal value) {
this.hldgsRedRate = value;
return this;
}
/**
* Gets the value of the rndg property.
*
* @return
* possible object is
* {@link RoundingDirection2Code }
*
*/
public RoundingDirection2Code getRndg() {
return rndg;
}
/**
* Sets the value of the rndg property.
*
* @param value
* allowed object is
* {@link RoundingDirection2Code }
*
*/
public RedemptionOrder7 setRndg(RoundingDirection2Code value) {
this.rndg = value;
return this;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public RedemptionOrder7 setSttlmAmt(ActiveCurrencyAndAmount value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the cshSttlmDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getCshSttlmDt() {
return cshSttlmDt;
}
/**
* Sets the value of the cshSttlmDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder7 setCshSttlmDt(LocalDate value) {
this.cshSttlmDt = value;
return this;
}
/**
* Gets the value of the sttlmMtd property.
*
* @return
* possible object is
* {@link DeliveryReceiptType2Code }
*
*/
public DeliveryReceiptType2Code getSttlmMtd() {
return sttlmMtd;
}
/**
* Sets the value of the sttlmMtd property.
*
* @param value
* allowed object is
* {@link DeliveryReceiptType2Code }
*
*/
public RedemptionOrder7 setSttlmMtd(DeliveryReceiptType2Code value) {
this.sttlmMtd = value;
return this;
}
/**
* Gets the value of the fxDtls property.
*
* @return
* possible object is
* {@link ForeignExchangeTerms6 }
*
*/
public ForeignExchangeTerms6 getFXDtls() {
return fxDtls;
}
/**
* Sets the value of the fxDtls property.
*
* @param value
* allowed object is
* {@link ForeignExchangeTerms6 }
*
*/
public RedemptionOrder7 setFXDtls(ForeignExchangeTerms6 value) {
this.fxDtls = value;
return this;
}
/**
* Gets the value of the incmPref property.
*
* @return
* possible object is
* {@link IncomePreference1Code }
*
*/
public IncomePreference1Code getIncmPref() {
return incmPref;
}
/**
* Sets the value of the incmPref property.
*
* @param value
* allowed object is
* {@link IncomePreference1Code }
*
*/
public RedemptionOrder7 setIncmPref(IncomePreference1Code value) {
this.incmPref = value;
return this;
}
/**
* Gets the value of the grp1Or2Units property.
*
* @return
* possible object is
* {@link UKTaxGroupUnitCode }
*
*/
public UKTaxGroupUnitCode getGrp1Or2Units() {
return grp1Or2Units;
}
/**
* Sets the value of the grp1Or2Units property.
*
* @param value
* allowed object is
* {@link UKTaxGroupUnitCode }
*
*/
public RedemptionOrder7 setGrp1Or2Units(UKTaxGroupUnitCode value) {
this.grp1Or2Units = value;
return this;
}
/**
* Gets the value of the chrgDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the chrgDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getChrgDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Charge17 }
*
*
* @return
* The value of the chrgDtls property.
*/
public List getChrgDtls() {
if (chrgDtls == null) {
chrgDtls = new ArrayList<>();
}
return this.chrgDtls;
}
/**
* Gets the value of the comssnDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the comssnDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getComssnDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Commission10 }
*
*
* @return
* The value of the comssnDtls property.
*/
public List getComssnDtls() {
if (comssnDtls == null) {
comssnDtls = new ArrayList<>();
}
return this.comssnDtls;
}
/**
* Gets the value of the taxDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the taxDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getTaxDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Tax16 }
*
*
* @return
* The value of the taxDtls property.
*/
public List getTaxDtls() {
if (taxDtls == null) {
taxDtls = new ArrayList<>();
}
return this.taxDtls;
}
/**
* Gets the value of the sttlmAndCtdyDtls property.
*
* @return
* possible object is
* {@link FundSettlementParameters3 }
*
*/
public FundSettlementParameters3 getSttlmAndCtdyDtls() {
return sttlmAndCtdyDtls;
}
/**
* Sets the value of the sttlmAndCtdyDtls property.
*
* @param value
* allowed object is
* {@link FundSettlementParameters3 }
*
*/
public RedemptionOrder7 setSttlmAndCtdyDtls(FundSettlementParameters3 value) {
this.sttlmAndCtdyDtls = value;
return this;
}
/**
* Gets the value of the physDlvryInd property.
*
*/
public boolean isPhysDlvryInd() {
return physDlvryInd;
}
/**
* Sets the value of the physDlvryInd property.
*
*/
public RedemptionOrder7 setPhysDlvryInd(boolean value) {
this.physDlvryInd = value;
return this;
}
/**
* Gets the value of the physDlvryDtls property.
*
* @return
* possible object is
* {@link DeliveryParameters3 }
*
*/
public DeliveryParameters3 getPhysDlvryDtls() {
return physDlvryDtls;
}
/**
* Sets the value of the physDlvryDtls property.
*
* @param value
* allowed object is
* {@link DeliveryParameters3 }
*
*/
public RedemptionOrder7 setPhysDlvryDtls(DeliveryParameters3 value) {
this.physDlvryDtls = value;
return this;
}
/**
* Gets the value of the cshSttlmDtls property.
*
* @return
* possible object is
* {@link PaymentTransaction21 }
*
*/
public PaymentTransaction21 getCshSttlmDtls() {
return cshSttlmDtls;
}
/**
* Sets the value of the cshSttlmDtls property.
*
* @param value
* allowed object is
* {@link PaymentTransaction21 }
*
*/
public RedemptionOrder7 setCshSttlmDtls(PaymentTransaction21 value) {
this.cshSttlmDtls = value;
return this;
}
/**
* Gets the value of the nonStdSttlmInf property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNonStdSttlmInf() {
return nonStdSttlmInf;
}
/**
* Sets the value of the nonStdSttlmInf property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public RedemptionOrder7 setNonStdSttlmInf(String value) {
this.nonStdSttlmInf = value;
return this;
}
/**
* Gets the value of the stffClntBrkdwn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the stffClntBrkdwn property.
*
*
* For example, to add a new item, do as follows:
*
* getStffClntBrkdwn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentFundsOrderBreakdown1 }
*
*
* @return
* The value of the stffClntBrkdwn property.
*/
public List getStffClntBrkdwn() {
if (stffClntBrkdwn == null) {
stffClntBrkdwn = new ArrayList<>();
}
return this.stffClntBrkdwn;
}
/**
* Gets the value of the finAdvc property.
*
* @return
* possible object is
* {@link FinancialAdvice1Code }
*
*/
public FinancialAdvice1Code getFinAdvc() {
return finAdvc;
}
/**
* Sets the value of the finAdvc property.
*
* @param value
* allowed object is
* {@link FinancialAdvice1Code }
*
*/
public RedemptionOrder7 setFinAdvc(FinancialAdvice1Code value) {
this.finAdvc = value;
return this;
}
/**
* Gets the value of the ngtdTrad property.
*
* @return
* possible object is
* {@link NegotiatedTrade1Code }
*
*/
public NegotiatedTrade1Code getNgtdTrad() {
return ngtdTrad;
}
/**
* Sets the value of the ngtdTrad property.
*
* @param value
* allowed object is
* {@link NegotiatedTrade1Code }
*
*/
public RedemptionOrder7 setNgtdTrad(NegotiatedTrade1Code value) {
this.ngtdTrad = value;
return this;
}
/**
* Gets the value of the rltdPtyDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rltdPtyDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getRltdPtyDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary8 }
*
*
* @return
* The value of the rltdPtyDtls property.
*/
public List getRltdPtyDtls() {
if (rltdPtyDtls == null) {
rltdPtyDtls = new ArrayList<>();
}
return this.rltdPtyDtls;
}
/**
* Gets the value of the equlstn property.
*
* @return
* possible object is
* {@link Equalisation1 }
*
*/
public Equalisation1 getEqulstn() {
return equlstn;
}
/**
* Sets the value of the equlstn property.
*
* @param value
* allowed object is
* {@link Equalisation1 }
*
*/
public RedemptionOrder7 setEqulstn(Equalisation1 value) {
this.equlstn = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the ordrTp list.
* @see #getOrdrTp()
*
*/
public RedemptionOrder7 addOrdrTp(FundOrderType2 ordrTp) {
getOrdrTp().add(ordrTp);
return this;
}
/**
* Adds a new item to the chrgDtls list.
* @see #getChrgDtls()
*
*/
public RedemptionOrder7 addChrgDtls(Charge17 chrgDtls) {
getChrgDtls().add(chrgDtls);
return this;
}
/**
* Adds a new item to the comssnDtls list.
* @see #getComssnDtls()
*
*/
public RedemptionOrder7 addComssnDtls(Commission10 comssnDtls) {
getComssnDtls().add(comssnDtls);
return this;
}
/**
* Adds a new item to the taxDtls list.
* @see #getTaxDtls()
*
*/
public RedemptionOrder7 addTaxDtls(Tax16 taxDtls) {
getTaxDtls().add(taxDtls);
return this;
}
/**
* Adds a new item to the stffClntBrkdwn list.
* @see #getStffClntBrkdwn()
*
*/
public RedemptionOrder7 addStffClntBrkdwn(InvestmentFundsOrderBreakdown1 stffClntBrkdwn) {
getStffClntBrkdwn().add(stffClntBrkdwn);
return this;
}
/**
* Adds a new item to the rltdPtyDtls list.
* @see #getRltdPtyDtls()
*
*/
public RedemptionOrder7 addRltdPtyDtls(Intermediary8 rltdPtyDtls) {
getRltdPtyDtls().add(rltdPtyDtls);
return this;
}
}