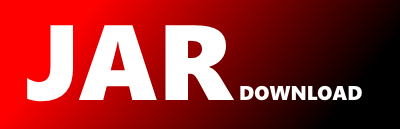
com.prowidesoftware.swift.model.mx.dic.ReorganisationInstructionSD12 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Provides additional information regarding corporate action reorganisation instruction details.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ReorganisationInstructionSD12", propOrder = {
"plcAndNm",
"txId",
"txSeq",
"txIdSts",
"prtctTxSts",
"rsn",
"sctiesQtyDtls",
"cstmrRefId",
"ackDtls",
"ctctPrsn",
"warrtSbcptChrgAmt",
"cert",
"usrRefNb",
"dcsdBnfclOwnrDtls",
"txRcrdNb",
"bidPric",
"shrhldrNb"
})
public class ReorganisationInstructionSD12 {
@XmlElement(name = "PlcAndNm")
protected String plcAndNm;
@XmlElement(name = "TxId")
protected String txId;
@XmlElement(name = "TxSeq")
protected List txSeq;
@XmlElement(name = "TxIdSts")
@XmlSchemaType(name = "string")
protected DTCInstructionStatus2Code txIdSts;
@XmlElement(name = "PrtctTxSts")
@XmlSchemaType(name = "string")
protected DTCProtectInstructionStatus3Code prtctTxSts;
@XmlElement(name = "Rsn")
@XmlSchemaType(name = "string")
protected List rsn;
@XmlElement(name = "SctiesQtyDtls")
protected SecuritiesQuantitySD9 sctiesQtyDtls;
@XmlElement(name = "CstmrRefId")
protected String cstmrRefId;
@XmlElement(name = "AckDtls")
protected CorporateActionAcknowledgementSD1 ackDtls;
@XmlElement(name = "CtctPrsn")
protected ContactIdentification5 ctctPrsn;
@XmlElement(name = "WarrtSbcptChrgAmt")
protected RestrictedFINActiveCurrencyAndAmount warrtSbcptChrgAmt;
@XmlElement(name = "Cert")
protected CorporateActionCertificateSD1 cert;
@XmlElement(name = "UsrRefNb")
protected String usrRefNb;
@XmlElement(name = "DcsdBnfclOwnrDtls")
protected DeceasedStatusSD1 dcsdBnfclOwnrDtls;
@XmlElement(name = "TxRcrdNb")
protected String txRcrdNb;
@XmlElement(name = "BidPric")
protected PriceFormat63Choice bidPric;
@XmlElement(name = "ShrhldrNb")
protected String shrhldrNb;
/**
* Gets the value of the plcAndNm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPlcAndNm() {
return plcAndNm;
}
/**
* Sets the value of the plcAndNm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ReorganisationInstructionSD12 setPlcAndNm(String value) {
this.plcAndNm = value;
return this;
}
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ReorganisationInstructionSD12 setTxId(String value) {
this.txId = value;
return this;
}
/**
* Gets the value of the txSeq property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the txSeq property.
*
*
* For example, to add a new item, do as follows:
*
* getTxSeq().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InstructionsTransactionsSequence2 }
*
*
* @return
* The value of the txSeq property.
*/
public List getTxSeq() {
if (txSeq == null) {
txSeq = new ArrayList<>();
}
return this.txSeq;
}
/**
* Gets the value of the txIdSts property.
*
* @return
* possible object is
* {@link DTCInstructionStatus2Code }
*
*/
public DTCInstructionStatus2Code getTxIdSts() {
return txIdSts;
}
/**
* Sets the value of the txIdSts property.
*
* @param value
* allowed object is
* {@link DTCInstructionStatus2Code }
*
*/
public ReorganisationInstructionSD12 setTxIdSts(DTCInstructionStatus2Code value) {
this.txIdSts = value;
return this;
}
/**
* Gets the value of the prtctTxSts property.
*
* @return
* possible object is
* {@link DTCProtectInstructionStatus3Code }
*
*/
public DTCProtectInstructionStatus3Code getPrtctTxSts() {
return prtctTxSts;
}
/**
* Sets the value of the prtctTxSts property.
*
* @param value
* allowed object is
* {@link DTCProtectInstructionStatus3Code }
*
*/
public ReorganisationInstructionSD12 setPrtctTxSts(DTCProtectInstructionStatus3Code value) {
this.prtctTxSts = value;
return this;
}
/**
* Gets the value of the rsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rsn property.
*
*
* For example, to add a new item, do as follows:
*
* getRsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InstructionReason4Code }
*
*
* @return
* The value of the rsn property.
*/
public List getRsn() {
if (rsn == null) {
rsn = new ArrayList<>();
}
return this.rsn;
}
/**
* Gets the value of the sctiesQtyDtls property.
*
* @return
* possible object is
* {@link SecuritiesQuantitySD9 }
*
*/
public SecuritiesQuantitySD9 getSctiesQtyDtls() {
return sctiesQtyDtls;
}
/**
* Sets the value of the sctiesQtyDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesQuantitySD9 }
*
*/
public ReorganisationInstructionSD12 setSctiesQtyDtls(SecuritiesQuantitySD9 value) {
this.sctiesQtyDtls = value;
return this;
}
/**
* Gets the value of the cstmrRefId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCstmrRefId() {
return cstmrRefId;
}
/**
* Sets the value of the cstmrRefId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ReorganisationInstructionSD12 setCstmrRefId(String value) {
this.cstmrRefId = value;
return this;
}
/**
* Gets the value of the ackDtls property.
*
* @return
* possible object is
* {@link CorporateActionAcknowledgementSD1 }
*
*/
public CorporateActionAcknowledgementSD1 getAckDtls() {
return ackDtls;
}
/**
* Sets the value of the ackDtls property.
*
* @param value
* allowed object is
* {@link CorporateActionAcknowledgementSD1 }
*
*/
public ReorganisationInstructionSD12 setAckDtls(CorporateActionAcknowledgementSD1 value) {
this.ackDtls = value;
return this;
}
/**
* Gets the value of the ctctPrsn property.
*
* @return
* possible object is
* {@link ContactIdentification5 }
*
*/
public ContactIdentification5 getCtctPrsn() {
return ctctPrsn;
}
/**
* Sets the value of the ctctPrsn property.
*
* @param value
* allowed object is
* {@link ContactIdentification5 }
*
*/
public ReorganisationInstructionSD12 setCtctPrsn(ContactIdentification5 value) {
this.ctctPrsn = value;
return this;
}
/**
* Gets the value of the warrtSbcptChrgAmt property.
*
* @return
* possible object is
* {@link RestrictedFINActiveCurrencyAndAmount }
*
*/
public RestrictedFINActiveCurrencyAndAmount getWarrtSbcptChrgAmt() {
return warrtSbcptChrgAmt;
}
/**
* Sets the value of the warrtSbcptChrgAmt property.
*
* @param value
* allowed object is
* {@link RestrictedFINActiveCurrencyAndAmount }
*
*/
public ReorganisationInstructionSD12 setWarrtSbcptChrgAmt(RestrictedFINActiveCurrencyAndAmount value) {
this.warrtSbcptChrgAmt = value;
return this;
}
/**
* Gets the value of the cert property.
*
* @return
* possible object is
* {@link CorporateActionCertificateSD1 }
*
*/
public CorporateActionCertificateSD1 getCert() {
return cert;
}
/**
* Sets the value of the cert property.
*
* @param value
* allowed object is
* {@link CorporateActionCertificateSD1 }
*
*/
public ReorganisationInstructionSD12 setCert(CorporateActionCertificateSD1 value) {
this.cert = value;
return this;
}
/**
* Gets the value of the usrRefNb property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUsrRefNb() {
return usrRefNb;
}
/**
* Sets the value of the usrRefNb property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ReorganisationInstructionSD12 setUsrRefNb(String value) {
this.usrRefNb = value;
return this;
}
/**
* Gets the value of the dcsdBnfclOwnrDtls property.
*
* @return
* possible object is
* {@link DeceasedStatusSD1 }
*
*/
public DeceasedStatusSD1 getDcsdBnfclOwnrDtls() {
return dcsdBnfclOwnrDtls;
}
/**
* Sets the value of the dcsdBnfclOwnrDtls property.
*
* @param value
* allowed object is
* {@link DeceasedStatusSD1 }
*
*/
public ReorganisationInstructionSD12 setDcsdBnfclOwnrDtls(DeceasedStatusSD1 value) {
this.dcsdBnfclOwnrDtls = value;
return this;
}
/**
* Gets the value of the txRcrdNb property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxRcrdNb() {
return txRcrdNb;
}
/**
* Sets the value of the txRcrdNb property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ReorganisationInstructionSD12 setTxRcrdNb(String value) {
this.txRcrdNb = value;
return this;
}
/**
* Gets the value of the bidPric property.
*
* @return
* possible object is
* {@link PriceFormat63Choice }
*
*/
public PriceFormat63Choice getBidPric() {
return bidPric;
}
/**
* Sets the value of the bidPric property.
*
* @param value
* allowed object is
* {@link PriceFormat63Choice }
*
*/
public ReorganisationInstructionSD12 setBidPric(PriceFormat63Choice value) {
this.bidPric = value;
return this;
}
/**
* Gets the value of the shrhldrNb property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getShrhldrNb() {
return shrhldrNb;
}
/**
* Sets the value of the shrhldrNb property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ReorganisationInstructionSD12 setShrhldrNb(String value) {
this.shrhldrNb = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the txSeq list.
* @see #getTxSeq()
*
*/
public ReorganisationInstructionSD12 addTxSeq(InstructionsTransactionsSequence2 txSeq) {
getTxSeq().add(txSeq);
return this;
}
/**
* Adds a new item to the rsn list.
* @see #getRsn()
*
*/
public ReorganisationInstructionSD12 addRsn(InstructionReason4Code rsn) {
getRsn().add(rsn);
return this;
}
}