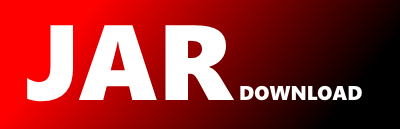
com.prowidesoftware.swift.model.mx.dic.SecuritiesFinancingConfirmationV02Subset Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* A securities financing transaction account servicer sends a SecuritiesFinancingConfirmation to an account owner to confirm or advise of the partial or full settlement of the opening or closing leg of a securities financing transaction.
* The account servicer/owner relationship may be:
* - a central securities depository or another settlement market infrastructure managing securities financing transactions on behalf of their participants
* - an agent (sub-custodian) managing securities financing transactions on behalf of their global custodian customer, or
* - a custodian managing securities financing transactions on behalf of an investment management institution or a broker/dealer.
* Usage
* The message may also be used to:
* - re-send a message previously sent,
* - provide a third party with a copy of a message for information,
* - re-send to a third party a copy of a message for information.
* using the relevant elements in the Business Application Header.
* ISO 15022 - 20022 Coexistence Subset
* This message definition is a subset of an ISO 20022 message that was reversed engineered from ISO 15022. A subset is a message definition that is compatible with another definition, but is more restrictive
* The ISO 15022 and ISO 20022 standards will coexist for a number of years. Until this coexistence period ends, the usage of certain data types is restricted to ensure interoperability between ISO 15022 and 20022 users. These restrictions, which are described by textual usage rules in the ISO 20022 message, have been made mandatory in this subset.
* NOTE: The ISO 20022 message coexistence textual rules have been kept in the subset to explain why specific data types have been restricted. These textual rules are identified as follows: CoexistenceXxxxRule.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesFinancingConfirmationV02Subset", propOrder = {
"txIdDtls",
"addtlParams",
"tradDtls",
"finInstrmId",
"finInstrmAttrbts",
"qtyAndAcctDtls",
"sctiesFincgDtls",
"stgSttlmInstrDtls",
"sttlmParams",
"dlvrgSttlmPties",
"rcvgSttlmPties",
"cshPties",
"sttldAmt",
"othrAmts",
"othrBizPties",
"splmtryData"
})
public class SecuritiesFinancingConfirmationV02Subset {
@XmlElement(name = "TxIdDtls", required = true)
protected TransactionTypeAndAdditionalParameters6 txIdDtls;
@XmlElement(name = "AddtlParams")
protected AdditionalParameters6 addtlParams;
@XmlElement(name = "TradDtls", required = true)
protected SecuritiesTradeDetails13 tradDtls;
@XmlElement(name = "FinInstrmId", required = true)
protected SecurityIdentification15 finInstrmId;
@XmlElement(name = "FinInstrmAttrbts")
protected FinancialInstrumentAttributes28 finInstrmAttrbts;
@XmlElement(name = "QtyAndAcctDtls", required = true)
protected QuantityAndAccount19 qtyAndAcctDtls;
@XmlElement(name = "SctiesFincgDtls")
protected SecuritiesFinancingTransactionDetails5 sctiesFincgDtls;
@XmlElement(name = "StgSttlmInstrDtls")
protected StandingSettlementInstruction6 stgSttlmInstrDtls;
@XmlElement(name = "SttlmParams")
protected SettlementDetails32 sttlmParams;
@XmlElement(name = "DlvrgSttlmPties")
protected SettlementParties17 dlvrgSttlmPties;
@XmlElement(name = "RcvgSttlmPties")
protected SettlementParties17 rcvgSttlmPties;
@XmlElement(name = "CshPties")
protected CashParties13 cshPties;
@XmlElement(name = "SttldAmt")
protected AmountAndDirection16 sttldAmt;
@XmlElement(name = "OthrAmts")
protected OtherAmounts5 othrAmts;
@XmlElement(name = "OthrBizPties")
protected OtherParties14 othrBizPties;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the txIdDtls property.
*
* @return
* possible object is
* {@link TransactionTypeAndAdditionalParameters6 }
*
*/
public TransactionTypeAndAdditionalParameters6 getTxIdDtls() {
return txIdDtls;
}
/**
* Sets the value of the txIdDtls property.
*
* @param value
* allowed object is
* {@link TransactionTypeAndAdditionalParameters6 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setTxIdDtls(TransactionTypeAndAdditionalParameters6 value) {
this.txIdDtls = value;
return this;
}
/**
* Gets the value of the addtlParams property.
*
* @return
* possible object is
* {@link AdditionalParameters6 }
*
*/
public AdditionalParameters6 getAddtlParams() {
return addtlParams;
}
/**
* Sets the value of the addtlParams property.
*
* @param value
* allowed object is
* {@link AdditionalParameters6 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setAddtlParams(AdditionalParameters6 value) {
this.addtlParams = value;
return this;
}
/**
* Gets the value of the tradDtls property.
*
* @return
* possible object is
* {@link SecuritiesTradeDetails13 }
*
*/
public SecuritiesTradeDetails13 getTradDtls() {
return tradDtls;
}
/**
* Sets the value of the tradDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesTradeDetails13 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setTradDtls(SecuritiesTradeDetails13 value) {
this.tradDtls = value;
return this;
}
/**
* Gets the value of the finInstrmId property.
*
* @return
* possible object is
* {@link SecurityIdentification15 }
*
*/
public SecurityIdentification15 getFinInstrmId() {
return finInstrmId;
}
/**
* Sets the value of the finInstrmId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification15 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setFinInstrmId(SecurityIdentification15 value) {
this.finInstrmId = value;
return this;
}
/**
* Gets the value of the finInstrmAttrbts property.
*
* @return
* possible object is
* {@link FinancialInstrumentAttributes28 }
*
*/
public FinancialInstrumentAttributes28 getFinInstrmAttrbts() {
return finInstrmAttrbts;
}
/**
* Sets the value of the finInstrmAttrbts property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentAttributes28 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setFinInstrmAttrbts(FinancialInstrumentAttributes28 value) {
this.finInstrmAttrbts = value;
return this;
}
/**
* Gets the value of the qtyAndAcctDtls property.
*
* @return
* possible object is
* {@link QuantityAndAccount19 }
*
*/
public QuantityAndAccount19 getQtyAndAcctDtls() {
return qtyAndAcctDtls;
}
/**
* Sets the value of the qtyAndAcctDtls property.
*
* @param value
* allowed object is
* {@link QuantityAndAccount19 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setQtyAndAcctDtls(QuantityAndAccount19 value) {
this.qtyAndAcctDtls = value;
return this;
}
/**
* Gets the value of the sctiesFincgDtls property.
*
* @return
* possible object is
* {@link SecuritiesFinancingTransactionDetails5 }
*
*/
public SecuritiesFinancingTransactionDetails5 getSctiesFincgDtls() {
return sctiesFincgDtls;
}
/**
* Sets the value of the sctiesFincgDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesFinancingTransactionDetails5 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setSctiesFincgDtls(SecuritiesFinancingTransactionDetails5 value) {
this.sctiesFincgDtls = value;
return this;
}
/**
* Gets the value of the stgSttlmInstrDtls property.
*
* @return
* possible object is
* {@link StandingSettlementInstruction6 }
*
*/
public StandingSettlementInstruction6 getStgSttlmInstrDtls() {
return stgSttlmInstrDtls;
}
/**
* Sets the value of the stgSttlmInstrDtls property.
*
* @param value
* allowed object is
* {@link StandingSettlementInstruction6 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setStgSttlmInstrDtls(StandingSettlementInstruction6 value) {
this.stgSttlmInstrDtls = value;
return this;
}
/**
* Gets the value of the sttlmParams property.
*
* @return
* possible object is
* {@link SettlementDetails32 }
*
*/
public SettlementDetails32 getSttlmParams() {
return sttlmParams;
}
/**
* Sets the value of the sttlmParams property.
*
* @param value
* allowed object is
* {@link SettlementDetails32 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setSttlmParams(SettlementDetails32 value) {
this.sttlmParams = value;
return this;
}
/**
* Gets the value of the dlvrgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties17 }
*
*/
public SettlementParties17 getDlvrgSttlmPties() {
return dlvrgSttlmPties;
}
/**
* Sets the value of the dlvrgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties17 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setDlvrgSttlmPties(SettlementParties17 value) {
this.dlvrgSttlmPties = value;
return this;
}
/**
* Gets the value of the rcvgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties17 }
*
*/
public SettlementParties17 getRcvgSttlmPties() {
return rcvgSttlmPties;
}
/**
* Sets the value of the rcvgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties17 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setRcvgSttlmPties(SettlementParties17 value) {
this.rcvgSttlmPties = value;
return this;
}
/**
* Gets the value of the cshPties property.
*
* @return
* possible object is
* {@link CashParties13 }
*
*/
public CashParties13 getCshPties() {
return cshPties;
}
/**
* Sets the value of the cshPties property.
*
* @param value
* allowed object is
* {@link CashParties13 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setCshPties(CashParties13 value) {
this.cshPties = value;
return this;
}
/**
* Gets the value of the sttldAmt property.
*
* @return
* possible object is
* {@link AmountAndDirection16 }
*
*/
public AmountAndDirection16 getSttldAmt() {
return sttldAmt;
}
/**
* Sets the value of the sttldAmt property.
*
* @param value
* allowed object is
* {@link AmountAndDirection16 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setSttldAmt(AmountAndDirection16 value) {
this.sttldAmt = value;
return this;
}
/**
* Gets the value of the othrAmts property.
*
* @return
* possible object is
* {@link OtherAmounts5 }
*
*/
public OtherAmounts5 getOthrAmts() {
return othrAmts;
}
/**
* Sets the value of the othrAmts property.
*
* @param value
* allowed object is
* {@link OtherAmounts5 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setOthrAmts(OtherAmounts5 value) {
this.othrAmts = value;
return this;
}
/**
* Gets the value of the othrBizPties property.
*
* @return
* possible object is
* {@link OtherParties14 }
*
*/
public OtherParties14 getOthrBizPties() {
return othrBizPties;
}
/**
* Sets the value of the othrBizPties property.
*
* @param value
* allowed object is
* {@link OtherParties14 }
*
*/
public SecuritiesFinancingConfirmationV02Subset setOthrBizPties(OtherParties14 value) {
this.othrBizPties = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public SecuritiesFinancingConfirmationV02Subset addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}