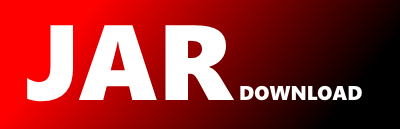
com.prowidesoftware.swift.model.mx.dic.SecuritiesOption107 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Provides information about the corporate action security option.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesOption107", propOrder = {
"sctyDtls",
"cdtDbtInd",
"tempFinInstrmInd",
"nonElgblPrcdsInd",
"issrOfferrTaxbltyInd",
"newSctiesIssncInd",
"incmTp",
"othrIncmTp",
"xmptnTp",
"entitldQty",
"sfkpgPlc",
"ctryOfIncmSrc",
"frctnDspstn",
"ccyOptn",
"tradgPrd",
"dtDtls",
"rateDtls",
"pricDtls",
"amtDtls"
})
public class SecuritiesOption107 {
@XmlElement(name = "SctyDtls", required = true)
protected FinancialInstrumentAttributes115 sctyDtls;
@XmlElement(name = "CdtDbtInd", required = true)
@XmlSchemaType(name = "string")
protected CreditDebitCode cdtDbtInd;
@XmlElement(name = "TempFinInstrmInd")
protected TemporaryFinancialInstrumentIndicator4Choice tempFinInstrmInd;
@XmlElement(name = "NonElgblPrcdsInd")
protected NonEligibleProceedsIndicator6Choice nonElgblPrcdsInd;
@XmlElement(name = "IssrOfferrTaxbltyInd")
protected IssuerOfferorTaxabilityIndicator1Choice issrOfferrTaxbltyInd;
@XmlElement(name = "NewSctiesIssncInd")
@XmlSchemaType(name = "string")
protected NewSecuritiesIssuanceType5Code newSctiesIssncInd;
@XmlElement(name = "IncmTp")
protected GenericIdentification47 incmTp;
@XmlElement(name = "OthrIncmTp")
protected List othrIncmTp;
@XmlElement(name = "XmptnTp")
protected List xmptnTp;
@XmlElement(name = "EntitldQty")
protected Quantity54Choice entitldQty;
@XmlElement(name = "SfkpgPlc")
protected SafekeepingPlaceFormat39Choice sfkpgPlc;
@XmlElement(name = "CtryOfIncmSrc")
protected String ctryOfIncmSrc;
@XmlElement(name = "FrctnDspstn")
protected FractionDispositionType31Choice frctnDspstn;
@XmlElement(name = "CcyOptn")
protected String ccyOptn;
@XmlElement(name = "TradgPrd")
protected Period6Choice tradgPrd;
@XmlElement(name = "DtDtls", required = true)
protected SecurityDate24 dtDtls;
@XmlElement(name = "RateDtls")
protected CorporateActionRate116 rateDtls;
@XmlElement(name = "PricDtls")
protected CorporateActionPrice79 pricDtls;
@XmlElement(name = "AmtDtls")
protected CorporateActionAmounts61 amtDtls;
/**
* Gets the value of the sctyDtls property.
*
* @return
* possible object is
* {@link FinancialInstrumentAttributes115 }
*
*/
public FinancialInstrumentAttributes115 getSctyDtls() {
return sctyDtls;
}
/**
* Sets the value of the sctyDtls property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentAttributes115 }
*
*/
public SecuritiesOption107 setSctyDtls(FinancialInstrumentAttributes115 value) {
this.sctyDtls = value;
return this;
}
/**
* Gets the value of the cdtDbtInd property.
*
* @return
* possible object is
* {@link CreditDebitCode }
*
*/
public CreditDebitCode getCdtDbtInd() {
return cdtDbtInd;
}
/**
* Sets the value of the cdtDbtInd property.
*
* @param value
* allowed object is
* {@link CreditDebitCode }
*
*/
public SecuritiesOption107 setCdtDbtInd(CreditDebitCode value) {
this.cdtDbtInd = value;
return this;
}
/**
* Gets the value of the tempFinInstrmInd property.
*
* @return
* possible object is
* {@link TemporaryFinancialInstrumentIndicator4Choice }
*
*/
public TemporaryFinancialInstrumentIndicator4Choice getTempFinInstrmInd() {
return tempFinInstrmInd;
}
/**
* Sets the value of the tempFinInstrmInd property.
*
* @param value
* allowed object is
* {@link TemporaryFinancialInstrumentIndicator4Choice }
*
*/
public SecuritiesOption107 setTempFinInstrmInd(TemporaryFinancialInstrumentIndicator4Choice value) {
this.tempFinInstrmInd = value;
return this;
}
/**
* Gets the value of the nonElgblPrcdsInd property.
*
* @return
* possible object is
* {@link NonEligibleProceedsIndicator6Choice }
*
*/
public NonEligibleProceedsIndicator6Choice getNonElgblPrcdsInd() {
return nonElgblPrcdsInd;
}
/**
* Sets the value of the nonElgblPrcdsInd property.
*
* @param value
* allowed object is
* {@link NonEligibleProceedsIndicator6Choice }
*
*/
public SecuritiesOption107 setNonElgblPrcdsInd(NonEligibleProceedsIndicator6Choice value) {
this.nonElgblPrcdsInd = value;
return this;
}
/**
* Gets the value of the issrOfferrTaxbltyInd property.
*
* @return
* possible object is
* {@link IssuerOfferorTaxabilityIndicator1Choice }
*
*/
public IssuerOfferorTaxabilityIndicator1Choice getIssrOfferrTaxbltyInd() {
return issrOfferrTaxbltyInd;
}
/**
* Sets the value of the issrOfferrTaxbltyInd property.
*
* @param value
* allowed object is
* {@link IssuerOfferorTaxabilityIndicator1Choice }
*
*/
public SecuritiesOption107 setIssrOfferrTaxbltyInd(IssuerOfferorTaxabilityIndicator1Choice value) {
this.issrOfferrTaxbltyInd = value;
return this;
}
/**
* Gets the value of the newSctiesIssncInd property.
*
* @return
* possible object is
* {@link NewSecuritiesIssuanceType5Code }
*
*/
public NewSecuritiesIssuanceType5Code getNewSctiesIssncInd() {
return newSctiesIssncInd;
}
/**
* Sets the value of the newSctiesIssncInd property.
*
* @param value
* allowed object is
* {@link NewSecuritiesIssuanceType5Code }
*
*/
public SecuritiesOption107 setNewSctiesIssncInd(NewSecuritiesIssuanceType5Code value) {
this.newSctiesIssncInd = value;
return this;
}
/**
* Gets the value of the incmTp property.
*
* @return
* possible object is
* {@link GenericIdentification47 }
*
*/
public GenericIdentification47 getIncmTp() {
return incmTp;
}
/**
* Sets the value of the incmTp property.
*
* @param value
* allowed object is
* {@link GenericIdentification47 }
*
*/
public SecuritiesOption107 setIncmTp(GenericIdentification47 value) {
this.incmTp = value;
return this;
}
/**
* Gets the value of the othrIncmTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrIncmTp property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrIncmTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link GenericIdentification47 }
*
*
* @return
* The value of the othrIncmTp property.
*/
public List getOthrIncmTp() {
if (othrIncmTp == null) {
othrIncmTp = new ArrayList<>();
}
return this.othrIncmTp;
}
/**
* Gets the value of the xmptnTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xmptnTp property.
*
*
* For example, to add a new item, do as follows:
*
* getXmptnTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link GenericIdentification47 }
*
*
* @return
* The value of the xmptnTp property.
*/
public List getXmptnTp() {
if (xmptnTp == null) {
xmptnTp = new ArrayList<>();
}
return this.xmptnTp;
}
/**
* Gets the value of the entitldQty property.
*
* @return
* possible object is
* {@link Quantity54Choice }
*
*/
public Quantity54Choice getEntitldQty() {
return entitldQty;
}
/**
* Sets the value of the entitldQty property.
*
* @param value
* allowed object is
* {@link Quantity54Choice }
*
*/
public SecuritiesOption107 setEntitldQty(Quantity54Choice value) {
this.entitldQty = value;
return this;
}
/**
* Gets the value of the sfkpgPlc property.
*
* @return
* possible object is
* {@link SafekeepingPlaceFormat39Choice }
*
*/
public SafekeepingPlaceFormat39Choice getSfkpgPlc() {
return sfkpgPlc;
}
/**
* Sets the value of the sfkpgPlc property.
*
* @param value
* allowed object is
* {@link SafekeepingPlaceFormat39Choice }
*
*/
public SecuritiesOption107 setSfkpgPlc(SafekeepingPlaceFormat39Choice value) {
this.sfkpgPlc = value;
return this;
}
/**
* Gets the value of the ctryOfIncmSrc property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCtryOfIncmSrc() {
return ctryOfIncmSrc;
}
/**
* Sets the value of the ctryOfIncmSrc property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesOption107 setCtryOfIncmSrc(String value) {
this.ctryOfIncmSrc = value;
return this;
}
/**
* Gets the value of the frctnDspstn property.
*
* @return
* possible object is
* {@link FractionDispositionType31Choice }
*
*/
public FractionDispositionType31Choice getFrctnDspstn() {
return frctnDspstn;
}
/**
* Sets the value of the frctnDspstn property.
*
* @param value
* allowed object is
* {@link FractionDispositionType31Choice }
*
*/
public SecuritiesOption107 setFrctnDspstn(FractionDispositionType31Choice value) {
this.frctnDspstn = value;
return this;
}
/**
* Gets the value of the ccyOptn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCcyOptn() {
return ccyOptn;
}
/**
* Sets the value of the ccyOptn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesOption107 setCcyOptn(String value) {
this.ccyOptn = value;
return this;
}
/**
* Gets the value of the tradgPrd property.
*
* @return
* possible object is
* {@link Period6Choice }
*
*/
public Period6Choice getTradgPrd() {
return tradgPrd;
}
/**
* Sets the value of the tradgPrd property.
*
* @param value
* allowed object is
* {@link Period6Choice }
*
*/
public SecuritiesOption107 setTradgPrd(Period6Choice value) {
this.tradgPrd = value;
return this;
}
/**
* Gets the value of the dtDtls property.
*
* @return
* possible object is
* {@link SecurityDate24 }
*
*/
public SecurityDate24 getDtDtls() {
return dtDtls;
}
/**
* Sets the value of the dtDtls property.
*
* @param value
* allowed object is
* {@link SecurityDate24 }
*
*/
public SecuritiesOption107 setDtDtls(SecurityDate24 value) {
this.dtDtls = value;
return this;
}
/**
* Gets the value of the rateDtls property.
*
* @return
* possible object is
* {@link CorporateActionRate116 }
*
*/
public CorporateActionRate116 getRateDtls() {
return rateDtls;
}
/**
* Sets the value of the rateDtls property.
*
* @param value
* allowed object is
* {@link CorporateActionRate116 }
*
*/
public SecuritiesOption107 setRateDtls(CorporateActionRate116 value) {
this.rateDtls = value;
return this;
}
/**
* Gets the value of the pricDtls property.
*
* @return
* possible object is
* {@link CorporateActionPrice79 }
*
*/
public CorporateActionPrice79 getPricDtls() {
return pricDtls;
}
/**
* Sets the value of the pricDtls property.
*
* @param value
* allowed object is
* {@link CorporateActionPrice79 }
*
*/
public SecuritiesOption107 setPricDtls(CorporateActionPrice79 value) {
this.pricDtls = value;
return this;
}
/**
* Gets the value of the amtDtls property.
*
* @return
* possible object is
* {@link CorporateActionAmounts61 }
*
*/
public CorporateActionAmounts61 getAmtDtls() {
return amtDtls;
}
/**
* Sets the value of the amtDtls property.
*
* @param value
* allowed object is
* {@link CorporateActionAmounts61 }
*
*/
public SecuritiesOption107 setAmtDtls(CorporateActionAmounts61 value) {
this.amtDtls = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the othrIncmTp list.
* @see #getOthrIncmTp()
*
*/
public SecuritiesOption107 addOthrIncmTp(GenericIdentification47 othrIncmTp) {
getOthrIncmTp().add(othrIncmTp);
return this;
}
/**
* Adds a new item to the xmptnTp list.
* @see #getXmptnTp()
*
*/
public SecuritiesOption107 addXmptnTp(GenericIdentification47 xmptnTp) {
getXmptnTp().add(xmptnTp);
return this;
}
}