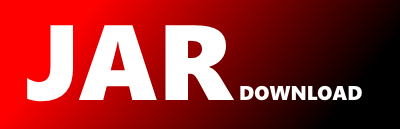
com.prowidesoftware.swift.model.mx.dic.SecuritiesSettlementTransactionAllegementNotificationV03 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An account servicer sends a SecuritiesSettlementTransactionAllegementNotification to an account owner to advise the account owner that a counterparty has alleged an instruction against the account owner's account at the account servicer and that the account servicer could not find the corresponding instruction of the account owner.
* The account servicer/owner relationship may be:
* - a central securities depository or another settlement market infrastructure acting on behalf of their participants
* - an agent (sub-custodian) acting on behalf of their global custodian customer, or
* - a custodian acting on behalf of an investment management institution or a broker/dealer.
*
* Usage
* The message may also be used to:
* - re-send a message previously sent,
* - provide a third party with a copy of a message for information,
* - re-send to a third party a copy of a message for information
* using the relevant elements in the Business Application Header.
*
* ISO 15022 - 20022 Coexistence
* This ISO 20022 message is reversed engineered from ISO 15022. Both standards will coexist for a certain number of years. Until this coexistence period ends, the usage of certain data types is restricted to ensure interoperability between ISO 15022 and 20022 users. Compliance to these rules is mandatory in a coexistence environment. The coexistence restrictions are described in a Textual Rule linked to the Message Items they concern. These coexistence textual rules are clearly identified as follows: “CoexistenceXxxxRule”.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesSettlementTransactionAllegementNotificationV03", propOrder = {
"txId",
"sttlmTpAndAddtlParams",
"mktInfrstrctrTxId",
"tradDtls",
"finInstrmId",
"finInstrmAttrbts",
"qtyAndAcctDtls",
"sctiesFincgDtls",
"sttlmParams",
"dlvrgSttlmPties",
"rcvgSttlmPties",
"cshPties",
"sttlmAmt",
"othrAmts",
"othrBizPties",
"splmtryData"
})
public class SecuritiesSettlementTransactionAllegementNotificationV03 {
@XmlElement(name = "TxId", required = true)
protected String txId;
@XmlElement(name = "SttlmTpAndAddtlParams", required = true)
protected SettlementTypeAndAdditionalParameters2 sttlmTpAndAddtlParams;
@XmlElement(name = "MktInfrstrctrTxId")
protected Identification1 mktInfrstrctrTxId;
@XmlElement(name = "TradDtls", required = true)
protected SecuritiesTradeDetails18 tradDtls;
@XmlElement(name = "FinInstrmId", required = true)
protected SecurityIdentification14 finInstrmId;
@XmlElement(name = "FinInstrmAttrbts")
protected FinancialInstrumentAttributes35 finInstrmAttrbts;
@XmlElement(name = "QtyAndAcctDtls", required = true)
protected QuantityAndAccount27 qtyAndAcctDtls;
@XmlElement(name = "SctiesFincgDtls")
protected SecuritiesFinancingTransactionDetails7 sctiesFincgDtls;
@XmlElement(name = "SttlmParams", required = true)
protected SettlementDetails49 sttlmParams;
@XmlElement(name = "DlvrgSttlmPties")
protected SettlementParties11 dlvrgSttlmPties;
@XmlElement(name = "RcvgSttlmPties")
protected SettlementParties11 rcvgSttlmPties;
@XmlElement(name = "CshPties")
protected CashParties11 cshPties;
@XmlElement(name = "SttlmAmt")
protected AmountAndDirection22 sttlmAmt;
@XmlElement(name = "OthrAmts")
protected OtherAmounts8 othrAmts;
@XmlElement(name = "OthrBizPties")
protected OtherParties11 othrBizPties;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setTxId(String value) {
this.txId = value;
return this;
}
/**
* Gets the value of the sttlmTpAndAddtlParams property.
*
* @return
* possible object is
* {@link SettlementTypeAndAdditionalParameters2 }
*
*/
public SettlementTypeAndAdditionalParameters2 getSttlmTpAndAddtlParams() {
return sttlmTpAndAddtlParams;
}
/**
* Sets the value of the sttlmTpAndAddtlParams property.
*
* @param value
* allowed object is
* {@link SettlementTypeAndAdditionalParameters2 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setSttlmTpAndAddtlParams(SettlementTypeAndAdditionalParameters2 value) {
this.sttlmTpAndAddtlParams = value;
return this;
}
/**
* Gets the value of the mktInfrstrctrTxId property.
*
* @return
* possible object is
* {@link Identification1 }
*
*/
public Identification1 getMktInfrstrctrTxId() {
return mktInfrstrctrTxId;
}
/**
* Sets the value of the mktInfrstrctrTxId property.
*
* @param value
* allowed object is
* {@link Identification1 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setMktInfrstrctrTxId(Identification1 value) {
this.mktInfrstrctrTxId = value;
return this;
}
/**
* Gets the value of the tradDtls property.
*
* @return
* possible object is
* {@link SecuritiesTradeDetails18 }
*
*/
public SecuritiesTradeDetails18 getTradDtls() {
return tradDtls;
}
/**
* Sets the value of the tradDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesTradeDetails18 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setTradDtls(SecuritiesTradeDetails18 value) {
this.tradDtls = value;
return this;
}
/**
* Gets the value of the finInstrmId property.
*
* @return
* possible object is
* {@link SecurityIdentification14 }
*
*/
public SecurityIdentification14 getFinInstrmId() {
return finInstrmId;
}
/**
* Sets the value of the finInstrmId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification14 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setFinInstrmId(SecurityIdentification14 value) {
this.finInstrmId = value;
return this;
}
/**
* Gets the value of the finInstrmAttrbts property.
*
* @return
* possible object is
* {@link FinancialInstrumentAttributes35 }
*
*/
public FinancialInstrumentAttributes35 getFinInstrmAttrbts() {
return finInstrmAttrbts;
}
/**
* Sets the value of the finInstrmAttrbts property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentAttributes35 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setFinInstrmAttrbts(FinancialInstrumentAttributes35 value) {
this.finInstrmAttrbts = value;
return this;
}
/**
* Gets the value of the qtyAndAcctDtls property.
*
* @return
* possible object is
* {@link QuantityAndAccount27 }
*
*/
public QuantityAndAccount27 getQtyAndAcctDtls() {
return qtyAndAcctDtls;
}
/**
* Sets the value of the qtyAndAcctDtls property.
*
* @param value
* allowed object is
* {@link QuantityAndAccount27 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setQtyAndAcctDtls(QuantityAndAccount27 value) {
this.qtyAndAcctDtls = value;
return this;
}
/**
* Gets the value of the sctiesFincgDtls property.
*
* @return
* possible object is
* {@link SecuritiesFinancingTransactionDetails7 }
*
*/
public SecuritiesFinancingTransactionDetails7 getSctiesFincgDtls() {
return sctiesFincgDtls;
}
/**
* Sets the value of the sctiesFincgDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesFinancingTransactionDetails7 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setSctiesFincgDtls(SecuritiesFinancingTransactionDetails7 value) {
this.sctiesFincgDtls = value;
return this;
}
/**
* Gets the value of the sttlmParams property.
*
* @return
* possible object is
* {@link SettlementDetails49 }
*
*/
public SettlementDetails49 getSttlmParams() {
return sttlmParams;
}
/**
* Sets the value of the sttlmParams property.
*
* @param value
* allowed object is
* {@link SettlementDetails49 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setSttlmParams(SettlementDetails49 value) {
this.sttlmParams = value;
return this;
}
/**
* Gets the value of the dlvrgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties11 }
*
*/
public SettlementParties11 getDlvrgSttlmPties() {
return dlvrgSttlmPties;
}
/**
* Sets the value of the dlvrgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties11 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setDlvrgSttlmPties(SettlementParties11 value) {
this.dlvrgSttlmPties = value;
return this;
}
/**
* Gets the value of the rcvgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties11 }
*
*/
public SettlementParties11 getRcvgSttlmPties() {
return rcvgSttlmPties;
}
/**
* Sets the value of the rcvgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties11 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setRcvgSttlmPties(SettlementParties11 value) {
this.rcvgSttlmPties = value;
return this;
}
/**
* Gets the value of the cshPties property.
*
* @return
* possible object is
* {@link CashParties11 }
*
*/
public CashParties11 getCshPties() {
return cshPties;
}
/**
* Sets the value of the cshPties property.
*
* @param value
* allowed object is
* {@link CashParties11 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setCshPties(CashParties11 value) {
this.cshPties = value;
return this;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link AmountAndDirection22 }
*
*/
public AmountAndDirection22 getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link AmountAndDirection22 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setSttlmAmt(AmountAndDirection22 value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the othrAmts property.
*
* @return
* possible object is
* {@link OtherAmounts8 }
*
*/
public OtherAmounts8 getOthrAmts() {
return othrAmts;
}
/**
* Sets the value of the othrAmts property.
*
* @param value
* allowed object is
* {@link OtherAmounts8 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setOthrAmts(OtherAmounts8 value) {
this.othrAmts = value;
return this;
}
/**
* Gets the value of the othrBizPties property.
*
* @return
* possible object is
* {@link OtherParties11 }
*
*/
public OtherParties11 getOthrBizPties() {
return othrBizPties;
}
/**
* Sets the value of the othrBizPties property.
*
* @param value
* allowed object is
* {@link OtherParties11 }
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 setOthrBizPties(OtherParties11 value) {
this.othrBizPties = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public SecuritiesSettlementTransactionAllegementNotificationV03 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}