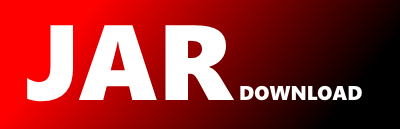
com.prowidesoftware.swift.model.mx.dic.SecuritiesSettlementTransactionAllegementReportV01 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An account servicer sends a SecuritiesSettlementTransactionAllegementReport to an account owner to provide, at a specified time, the status and details of pending settlement allegements, for all or selected securities in a specified safekeeping account.
* The account servicer/owner relationship may be:
* - a central securities depository or another settlement market infrastructure acting on behalf of their participants
* - an agent (sub-custodian) acting on behalf of their global custodian customer, or
* - a custodian acting on behalf of an investment management institution or a broker/dealer.
* Usage
* The message may also be used to:
* - re-send a message previously sent (the sub-function of the message is Duplicate),
* - provide a third party with a copy of a message for information (the sub-function of the message is Copy),
* - re-send to a third party a copy of a message for information (the sub-function of the message is Copy Duplicate).
* ISO 15022 - 20022 Coexistence
* This ISO 20022 message is reversed engineered from ISO 15022. Both standards will coexist for a certain number of years. Until this coexistence period ends, the usage of certain data types is restricted to ensure interoperability between ISO 15022 and 20022 users. Compliance to these rules is mandatory in a coexistence environment. The coexistence restrictions are described in a Textual Rule linked to the Message Items they concern. These coexistence textual rules are clearly identified as follows: “CoexistenceXxxxRule”.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesSettlementTransactionAllegementReportV01", propOrder = {
"id",
"pgntn",
"stmtGnlDtls",
"acctOwnr",
"sfkpgAcct",
"allgmtDtls",
"msgOrgtr",
"msgRcpt"
})
public class SecuritiesSettlementTransactionAllegementReportV01 {
@XmlElement(name = "Id", required = true)
protected DocumentIdentification11 id;
@XmlElement(name = "Pgntn", required = true)
protected Pagination pgntn;
@XmlElement(name = "StmtGnlDtls", required = true)
protected Statement17 stmtGnlDtls;
@XmlElement(name = "AcctOwnr")
protected PartyIdentification13Choice acctOwnr;
@XmlElement(name = "SfkpgAcct", required = true)
protected SecuritiesAccount13 sfkpgAcct;
@XmlElement(name = "AllgmtDtls")
protected List allgmtDtls;
@XmlElement(name = "MsgOrgtr")
protected PartyIdentification10Choice msgOrgtr;
@XmlElement(name = "MsgRcpt")
protected PartyIdentification10Choice msgRcpt;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link DocumentIdentification11 }
*
*/
public DocumentIdentification11 getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link DocumentIdentification11 }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setId(DocumentIdentification11 value) {
this.id = value;
return this;
}
/**
* Gets the value of the pgntn property.
*
* @return
* possible object is
* {@link Pagination }
*
*/
public Pagination getPgntn() {
return pgntn;
}
/**
* Sets the value of the pgntn property.
*
* @param value
* allowed object is
* {@link Pagination }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setPgntn(Pagination value) {
this.pgntn = value;
return this;
}
/**
* Gets the value of the stmtGnlDtls property.
*
* @return
* possible object is
* {@link Statement17 }
*
*/
public Statement17 getStmtGnlDtls() {
return stmtGnlDtls;
}
/**
* Sets the value of the stmtGnlDtls property.
*
* @param value
* allowed object is
* {@link Statement17 }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setStmtGnlDtls(Statement17 value) {
this.stmtGnlDtls = value;
return this;
}
/**
* Gets the value of the acctOwnr property.
*
* @return
* possible object is
* {@link PartyIdentification13Choice }
*
*/
public PartyIdentification13Choice getAcctOwnr() {
return acctOwnr;
}
/**
* Sets the value of the acctOwnr property.
*
* @param value
* allowed object is
* {@link PartyIdentification13Choice }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setAcctOwnr(PartyIdentification13Choice value) {
this.acctOwnr = value;
return this;
}
/**
* Gets the value of the sfkpgAcct property.
*
* @return
* possible object is
* {@link SecuritiesAccount13 }
*
*/
public SecuritiesAccount13 getSfkpgAcct() {
return sfkpgAcct;
}
/**
* Sets the value of the sfkpgAcct property.
*
* @param value
* allowed object is
* {@link SecuritiesAccount13 }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setSfkpgAcct(SecuritiesAccount13 value) {
this.sfkpgAcct = value;
return this;
}
/**
* Gets the value of the allgmtDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the allgmtDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getAllgmtDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SecuritiesTradeDetails4 }
*
*
* @return
* The value of the allgmtDtls property.
*/
public List getAllgmtDtls() {
if (allgmtDtls == null) {
allgmtDtls = new ArrayList<>();
}
return this.allgmtDtls;
}
/**
* Gets the value of the msgOrgtr property.
*
* @return
* possible object is
* {@link PartyIdentification10Choice }
*
*/
public PartyIdentification10Choice getMsgOrgtr() {
return msgOrgtr;
}
/**
* Sets the value of the msgOrgtr property.
*
* @param value
* allowed object is
* {@link PartyIdentification10Choice }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setMsgOrgtr(PartyIdentification10Choice value) {
this.msgOrgtr = value;
return this;
}
/**
* Gets the value of the msgRcpt property.
*
* @return
* possible object is
* {@link PartyIdentification10Choice }
*
*/
public PartyIdentification10Choice getMsgRcpt() {
return msgRcpt;
}
/**
* Sets the value of the msgRcpt property.
*
* @param value
* allowed object is
* {@link PartyIdentification10Choice }
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 setMsgRcpt(PartyIdentification10Choice value) {
this.msgRcpt = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the allgmtDtls list.
* @see #getAllgmtDtls()
*
*/
public SecuritiesSettlementTransactionAllegementReportV01 addAllgmtDtls(SecuritiesTradeDetails4 allgmtDtls) {
getAllgmtDtls().add(allgmtDtls);
return this;
}
}