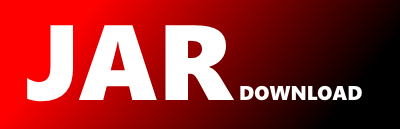
com.prowidesoftware.swift.model.mx.dic.SecuritiesSettlementTransactionConfirmationV01 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An account servicer sends a SecuritiesSettlementTransactionConfirmation to an account owner to confirm the partial or full delivery or receipt of financial instruments, free or against of payment, physically or by book-entry.
* The account servicer/owner relationship may be:
* - a central securities depository or another settlement market infrastructure acting on behalf of their participants
* - an agent (sub-custodian) acting on behalf of their global custodian customer, or
* - a custodian acting on behalf of an investment management institution or a broker/dealer.
* Usage
* The message may also be used to:
* - re-send a message previously sent (the sub-function of the message is Duplicate),
* - provide a third party with a copy of a message for information (the sub-function of the message is Copy),
* - re-send to a third party a copy of a message for information (the sub-function of the message is Copy Duplicate).
* ISO 15022 - 20022 Coexistence
* This ISO 20022 message is reversed engineered from ISO 15022. Both standards will coexist for a certain number of years. Until this coexistence period ends, the usage of certain data types is restricted to ensure interoperability between ISO 15022 and 20022 users. Compliance to these rules is mandatory in a coexistence environment. The coexistence restrictions are described in a Textual Rule linked to the Message Items they concern. These coexistence textual rules are clearly identified as follows: “CoexistenceXxxxRule”.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesSettlementTransactionConfirmationV01", propOrder = {
"id",
"txIdDtls",
"addtlParams",
"tradDtls",
"finInstrmId",
"finInstrmAttrbts",
"qtyAndAcctDtls",
"sttlmParams",
"stgSttlmInstrDtls",
"dlvrgSttlmPties",
"rcvgSttlmPties",
"cshPties",
"sttldAmt",
"othrAmts",
"othrBizPties",
"addtlPhysOrRegnDtls",
"msgOrgtr",
"msgRcpt",
"xtnsn"
})
public class SecuritiesSettlementTransactionConfirmationV01 {
@XmlElement(name = "Id", required = true)
protected DocumentIdentification11 id;
@XmlElement(name = "TxIdDtls", required = true)
protected SettlementTypeAndIdentification1 txIdDtls;
@XmlElement(name = "AddtlParams")
protected AdditionalParameters2 addtlParams;
@XmlElement(name = "TradDtls", required = true)
protected SecuritiesTradeDetails2 tradDtls;
@XmlElement(name = "FinInstrmId", required = true)
protected SecurityIdentification11 finInstrmId;
@XmlElement(name = "FinInstrmAttrbts")
protected FinancialInstrumentAttributes8 finInstrmAttrbts;
@XmlElement(name = "QtyAndAcctDtls", required = true)
protected QuantityAndAccount2 qtyAndAcctDtls;
@XmlElement(name = "SttlmParams", required = true)
protected SettlementDetails6 sttlmParams;
@XmlElement(name = "StgSttlmInstrDtls")
protected StandingSettlementInstruction1 stgSttlmInstrDtls;
@XmlElement(name = "DlvrgSttlmPties")
protected SettlementParties5 dlvrgSttlmPties;
@XmlElement(name = "RcvgSttlmPties")
protected SettlementParties5 rcvgSttlmPties;
@XmlElement(name = "CshPties")
protected CashParties3 cshPties;
@XmlElement(name = "SttldAmt")
protected AmountAndDirection2 sttldAmt;
@XmlElement(name = "OthrAmts")
protected OtherAmounts4 othrAmts;
@XmlElement(name = "OthrBizPties")
protected OtherParties2 othrBizPties;
@XmlElement(name = "AddtlPhysOrRegnDtls")
protected RegistrationParameters1 addtlPhysOrRegnDtls;
@XmlElement(name = "MsgOrgtr")
protected PartyIdentification10Choice msgOrgtr;
@XmlElement(name = "MsgRcpt")
protected PartyIdentification10Choice msgRcpt;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link DocumentIdentification11 }
*
*/
public DocumentIdentification11 getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link DocumentIdentification11 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setId(DocumentIdentification11 value) {
this.id = value;
return this;
}
/**
* Gets the value of the txIdDtls property.
*
* @return
* possible object is
* {@link SettlementTypeAndIdentification1 }
*
*/
public SettlementTypeAndIdentification1 getTxIdDtls() {
return txIdDtls;
}
/**
* Sets the value of the txIdDtls property.
*
* @param value
* allowed object is
* {@link SettlementTypeAndIdentification1 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setTxIdDtls(SettlementTypeAndIdentification1 value) {
this.txIdDtls = value;
return this;
}
/**
* Gets the value of the addtlParams property.
*
* @return
* possible object is
* {@link AdditionalParameters2 }
*
*/
public AdditionalParameters2 getAddtlParams() {
return addtlParams;
}
/**
* Sets the value of the addtlParams property.
*
* @param value
* allowed object is
* {@link AdditionalParameters2 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setAddtlParams(AdditionalParameters2 value) {
this.addtlParams = value;
return this;
}
/**
* Gets the value of the tradDtls property.
*
* @return
* possible object is
* {@link SecuritiesTradeDetails2 }
*
*/
public SecuritiesTradeDetails2 getTradDtls() {
return tradDtls;
}
/**
* Sets the value of the tradDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesTradeDetails2 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setTradDtls(SecuritiesTradeDetails2 value) {
this.tradDtls = value;
return this;
}
/**
* Gets the value of the finInstrmId property.
*
* @return
* possible object is
* {@link SecurityIdentification11 }
*
*/
public SecurityIdentification11 getFinInstrmId() {
return finInstrmId;
}
/**
* Sets the value of the finInstrmId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification11 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setFinInstrmId(SecurityIdentification11 value) {
this.finInstrmId = value;
return this;
}
/**
* Gets the value of the finInstrmAttrbts property.
*
* @return
* possible object is
* {@link FinancialInstrumentAttributes8 }
*
*/
public FinancialInstrumentAttributes8 getFinInstrmAttrbts() {
return finInstrmAttrbts;
}
/**
* Sets the value of the finInstrmAttrbts property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentAttributes8 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setFinInstrmAttrbts(FinancialInstrumentAttributes8 value) {
this.finInstrmAttrbts = value;
return this;
}
/**
* Gets the value of the qtyAndAcctDtls property.
*
* @return
* possible object is
* {@link QuantityAndAccount2 }
*
*/
public QuantityAndAccount2 getQtyAndAcctDtls() {
return qtyAndAcctDtls;
}
/**
* Sets the value of the qtyAndAcctDtls property.
*
* @param value
* allowed object is
* {@link QuantityAndAccount2 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setQtyAndAcctDtls(QuantityAndAccount2 value) {
this.qtyAndAcctDtls = value;
return this;
}
/**
* Gets the value of the sttlmParams property.
*
* @return
* possible object is
* {@link SettlementDetails6 }
*
*/
public SettlementDetails6 getSttlmParams() {
return sttlmParams;
}
/**
* Sets the value of the sttlmParams property.
*
* @param value
* allowed object is
* {@link SettlementDetails6 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setSttlmParams(SettlementDetails6 value) {
this.sttlmParams = value;
return this;
}
/**
* Gets the value of the stgSttlmInstrDtls property.
*
* @return
* possible object is
* {@link StandingSettlementInstruction1 }
*
*/
public StandingSettlementInstruction1 getStgSttlmInstrDtls() {
return stgSttlmInstrDtls;
}
/**
* Sets the value of the stgSttlmInstrDtls property.
*
* @param value
* allowed object is
* {@link StandingSettlementInstruction1 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setStgSttlmInstrDtls(StandingSettlementInstruction1 value) {
this.stgSttlmInstrDtls = value;
return this;
}
/**
* Gets the value of the dlvrgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties5 }
*
*/
public SettlementParties5 getDlvrgSttlmPties() {
return dlvrgSttlmPties;
}
/**
* Sets the value of the dlvrgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties5 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setDlvrgSttlmPties(SettlementParties5 value) {
this.dlvrgSttlmPties = value;
return this;
}
/**
* Gets the value of the rcvgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties5 }
*
*/
public SettlementParties5 getRcvgSttlmPties() {
return rcvgSttlmPties;
}
/**
* Sets the value of the rcvgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties5 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setRcvgSttlmPties(SettlementParties5 value) {
this.rcvgSttlmPties = value;
return this;
}
/**
* Gets the value of the cshPties property.
*
* @return
* possible object is
* {@link CashParties3 }
*
*/
public CashParties3 getCshPties() {
return cshPties;
}
/**
* Sets the value of the cshPties property.
*
* @param value
* allowed object is
* {@link CashParties3 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setCshPties(CashParties3 value) {
this.cshPties = value;
return this;
}
/**
* Gets the value of the sttldAmt property.
*
* @return
* possible object is
* {@link AmountAndDirection2 }
*
*/
public AmountAndDirection2 getSttldAmt() {
return sttldAmt;
}
/**
* Sets the value of the sttldAmt property.
*
* @param value
* allowed object is
* {@link AmountAndDirection2 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setSttldAmt(AmountAndDirection2 value) {
this.sttldAmt = value;
return this;
}
/**
* Gets the value of the othrAmts property.
*
* @return
* possible object is
* {@link OtherAmounts4 }
*
*/
public OtherAmounts4 getOthrAmts() {
return othrAmts;
}
/**
* Sets the value of the othrAmts property.
*
* @param value
* allowed object is
* {@link OtherAmounts4 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setOthrAmts(OtherAmounts4 value) {
this.othrAmts = value;
return this;
}
/**
* Gets the value of the othrBizPties property.
*
* @return
* possible object is
* {@link OtherParties2 }
*
*/
public OtherParties2 getOthrBizPties() {
return othrBizPties;
}
/**
* Sets the value of the othrBizPties property.
*
* @param value
* allowed object is
* {@link OtherParties2 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setOthrBizPties(OtherParties2 value) {
this.othrBizPties = value;
return this;
}
/**
* Gets the value of the addtlPhysOrRegnDtls property.
*
* @return
* possible object is
* {@link RegistrationParameters1 }
*
*/
public RegistrationParameters1 getAddtlPhysOrRegnDtls() {
return addtlPhysOrRegnDtls;
}
/**
* Sets the value of the addtlPhysOrRegnDtls property.
*
* @param value
* allowed object is
* {@link RegistrationParameters1 }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setAddtlPhysOrRegnDtls(RegistrationParameters1 value) {
this.addtlPhysOrRegnDtls = value;
return this;
}
/**
* Gets the value of the msgOrgtr property.
*
* @return
* possible object is
* {@link PartyIdentification10Choice }
*
*/
public PartyIdentification10Choice getMsgOrgtr() {
return msgOrgtr;
}
/**
* Sets the value of the msgOrgtr property.
*
* @param value
* allowed object is
* {@link PartyIdentification10Choice }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setMsgOrgtr(PartyIdentification10Choice value) {
this.msgOrgtr = value;
return this;
}
/**
* Gets the value of the msgRcpt property.
*
* @return
* possible object is
* {@link PartyIdentification10Choice }
*
*/
public PartyIdentification10Choice getMsgRcpt() {
return msgRcpt;
}
/**
* Sets the value of the msgRcpt property.
*
* @param value
* allowed object is
* {@link PartyIdentification10Choice }
*
*/
public SecuritiesSettlementTransactionConfirmationV01 setMsgRcpt(PartyIdentification10Choice value) {
this.msgRcpt = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension2 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public SecuritiesSettlementTransactionConfirmationV01 addXtnsn(Extension2 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}