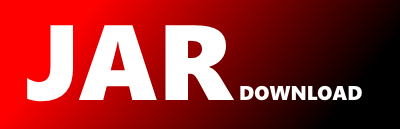
com.prowidesoftware.swift.model.mx.dic.SecuritiesSettlementTransactionInstructionV02 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An account owner sends a SecuritiesSettlementTransactionInstruction to an account servicer to instruct the receipt or delivery of financial instruments with or without payment, physically or by book-entry.
* The account owner/servicer relationship may be:
* - a global custodian which has an account with a local custodian, or
* - an investment management institution which manages a fund account opened at a custodian, or
* - a broker which has an account with a custodian, or
* - a central securities depository participant which has an account with a central securities depository, or
* - a central securities depository which has an account with a custodian, another central securities depository or another settlement market infrastructure, or
* - a central counterparty or a stock exchange or a trade matching utility which need to instruct the settlement of transactions to a central securities depository or another settlement market infrastructure
* Usage
* The instruction may be linked to other settlement instructions, for example, for a turnaround or back-to-back, or other transactions, for example, foreign exchange deal, using the linkage functionality.
* The message may also be used to:
* - re-send a message previously sent,
* - provide a third party with a copy of a message for information,
* - re-send to a third party a copy of a message for information.
* using the relevant elements in the Business Application Header.
* ISO 15022 - 20022 Coexistence
* This ISO 20022 message is reversed engineered from ISO 15022. Both standards will coexist for a certain number of years. Until this coexistence period ends, the usage of certain data types is restricted to ensure interoperability between ISO 15022 and 20022 users. Compliance to these rules is mandatory in a coexistence environment. The coexistence restrictions are described in a Textual Rule linked to the Message Items they concern. These coexistence textual rules are clearly identified as follows: “CoexistenceXxxxRule”.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesSettlementTransactionInstructionV02", propOrder = {
"txId",
"sttlmTpAndAddtlParams",
"nbCounts",
"lnkgs",
"tradDtls",
"finInstrmId",
"finInstrmAttrbts",
"qtyAndAcctDtls",
"sttlmParams",
"stgSttlmInstrDtls",
"dlvrgSttlmPties",
"rcvgSttlmPties",
"cshPties",
"sttlmAmt",
"othrAmts",
"othrBizPties",
"addtlPhysOrRegnDtls",
"splmtryData"
})
public class SecuritiesSettlementTransactionInstructionV02 {
@XmlElement(name = "TxId", required = true)
protected String txId;
@XmlElement(name = "SttlmTpAndAddtlParams", required = true)
protected SettlementTypeAndAdditionalParameters1 sttlmTpAndAddtlParams;
@XmlElement(name = "NbCounts")
protected NumberCount1Choice nbCounts;
@XmlElement(name = "Lnkgs")
protected List lnkgs;
@XmlElement(name = "TradDtls", required = true)
protected SecuritiesTradeDetails1 tradDtls;
@XmlElement(name = "FinInstrmId", required = true)
protected SecurityIdentification14 finInstrmId;
@XmlElement(name = "FinInstrmAttrbts")
protected FinancialInstrumentAttributes20 finInstrmAttrbts;
@XmlElement(name = "QtyAndAcctDtls", required = true)
protected QuantityAndAccount17 qtyAndAcctDtls;
@XmlElement(name = "SttlmParams", required = true)
protected SettlementDetails22 sttlmParams;
@XmlElement(name = "StgSttlmInstrDtls")
protected StandingSettlementInstruction4 stgSttlmInstrDtls;
@XmlElement(name = "DlvrgSttlmPties")
protected SettlementParties11 dlvrgSttlmPties;
@XmlElement(name = "RcvgSttlmPties")
protected SettlementParties11 rcvgSttlmPties;
@XmlElement(name = "CshPties")
protected CashParties8 cshPties;
@XmlElement(name = "SttlmAmt")
protected AmountAndDirection2 sttlmAmt;
@XmlElement(name = "OthrAmts")
protected OtherAmounts12 othrAmts;
@XmlElement(name = "OthrBizPties")
protected OtherParties8 othrBizPties;
@XmlElement(name = "AddtlPhysOrRegnDtls")
protected RegistrationParameters1 addtlPhysOrRegnDtls;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setTxId(String value) {
this.txId = value;
return this;
}
/**
* Gets the value of the sttlmTpAndAddtlParams property.
*
* @return
* possible object is
* {@link SettlementTypeAndAdditionalParameters1 }
*
*/
public SettlementTypeAndAdditionalParameters1 getSttlmTpAndAddtlParams() {
return sttlmTpAndAddtlParams;
}
/**
* Sets the value of the sttlmTpAndAddtlParams property.
*
* @param value
* allowed object is
* {@link SettlementTypeAndAdditionalParameters1 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setSttlmTpAndAddtlParams(SettlementTypeAndAdditionalParameters1 value) {
this.sttlmTpAndAddtlParams = value;
return this;
}
/**
* Gets the value of the nbCounts property.
*
* @return
* possible object is
* {@link NumberCount1Choice }
*
*/
public NumberCount1Choice getNbCounts() {
return nbCounts;
}
/**
* Sets the value of the nbCounts property.
*
* @param value
* allowed object is
* {@link NumberCount1Choice }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setNbCounts(NumberCount1Choice value) {
this.nbCounts = value;
return this;
}
/**
* Gets the value of the lnkgs property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the lnkgs property.
*
*
* For example, to add a new item, do as follows:
*
* getLnkgs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Linkages7 }
*
*
* @return
* The value of the lnkgs property.
*/
public List getLnkgs() {
if (lnkgs == null) {
lnkgs = new ArrayList<>();
}
return this.lnkgs;
}
/**
* Gets the value of the tradDtls property.
*
* @return
* possible object is
* {@link SecuritiesTradeDetails1 }
*
*/
public SecuritiesTradeDetails1 getTradDtls() {
return tradDtls;
}
/**
* Sets the value of the tradDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesTradeDetails1 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setTradDtls(SecuritiesTradeDetails1 value) {
this.tradDtls = value;
return this;
}
/**
* Gets the value of the finInstrmId property.
*
* @return
* possible object is
* {@link SecurityIdentification14 }
*
*/
public SecurityIdentification14 getFinInstrmId() {
return finInstrmId;
}
/**
* Sets the value of the finInstrmId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification14 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setFinInstrmId(SecurityIdentification14 value) {
this.finInstrmId = value;
return this;
}
/**
* Gets the value of the finInstrmAttrbts property.
*
* @return
* possible object is
* {@link FinancialInstrumentAttributes20 }
*
*/
public FinancialInstrumentAttributes20 getFinInstrmAttrbts() {
return finInstrmAttrbts;
}
/**
* Sets the value of the finInstrmAttrbts property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentAttributes20 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setFinInstrmAttrbts(FinancialInstrumentAttributes20 value) {
this.finInstrmAttrbts = value;
return this;
}
/**
* Gets the value of the qtyAndAcctDtls property.
*
* @return
* possible object is
* {@link QuantityAndAccount17 }
*
*/
public QuantityAndAccount17 getQtyAndAcctDtls() {
return qtyAndAcctDtls;
}
/**
* Sets the value of the qtyAndAcctDtls property.
*
* @param value
* allowed object is
* {@link QuantityAndAccount17 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setQtyAndAcctDtls(QuantityAndAccount17 value) {
this.qtyAndAcctDtls = value;
return this;
}
/**
* Gets the value of the sttlmParams property.
*
* @return
* possible object is
* {@link SettlementDetails22 }
*
*/
public SettlementDetails22 getSttlmParams() {
return sttlmParams;
}
/**
* Sets the value of the sttlmParams property.
*
* @param value
* allowed object is
* {@link SettlementDetails22 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setSttlmParams(SettlementDetails22 value) {
this.sttlmParams = value;
return this;
}
/**
* Gets the value of the stgSttlmInstrDtls property.
*
* @return
* possible object is
* {@link StandingSettlementInstruction4 }
*
*/
public StandingSettlementInstruction4 getStgSttlmInstrDtls() {
return stgSttlmInstrDtls;
}
/**
* Sets the value of the stgSttlmInstrDtls property.
*
* @param value
* allowed object is
* {@link StandingSettlementInstruction4 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setStgSttlmInstrDtls(StandingSettlementInstruction4 value) {
this.stgSttlmInstrDtls = value;
return this;
}
/**
* Gets the value of the dlvrgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties11 }
*
*/
public SettlementParties11 getDlvrgSttlmPties() {
return dlvrgSttlmPties;
}
/**
* Sets the value of the dlvrgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties11 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setDlvrgSttlmPties(SettlementParties11 value) {
this.dlvrgSttlmPties = value;
return this;
}
/**
* Gets the value of the rcvgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties11 }
*
*/
public SettlementParties11 getRcvgSttlmPties() {
return rcvgSttlmPties;
}
/**
* Sets the value of the rcvgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties11 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setRcvgSttlmPties(SettlementParties11 value) {
this.rcvgSttlmPties = value;
return this;
}
/**
* Gets the value of the cshPties property.
*
* @return
* possible object is
* {@link CashParties8 }
*
*/
public CashParties8 getCshPties() {
return cshPties;
}
/**
* Sets the value of the cshPties property.
*
* @param value
* allowed object is
* {@link CashParties8 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setCshPties(CashParties8 value) {
this.cshPties = value;
return this;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link AmountAndDirection2 }
*
*/
public AmountAndDirection2 getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link AmountAndDirection2 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setSttlmAmt(AmountAndDirection2 value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the othrAmts property.
*
* @return
* possible object is
* {@link OtherAmounts12 }
*
*/
public OtherAmounts12 getOthrAmts() {
return othrAmts;
}
/**
* Sets the value of the othrAmts property.
*
* @param value
* allowed object is
* {@link OtherAmounts12 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setOthrAmts(OtherAmounts12 value) {
this.othrAmts = value;
return this;
}
/**
* Gets the value of the othrBizPties property.
*
* @return
* possible object is
* {@link OtherParties8 }
*
*/
public OtherParties8 getOthrBizPties() {
return othrBizPties;
}
/**
* Sets the value of the othrBizPties property.
*
* @param value
* allowed object is
* {@link OtherParties8 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setOthrBizPties(OtherParties8 value) {
this.othrBizPties = value;
return this;
}
/**
* Gets the value of the addtlPhysOrRegnDtls property.
*
* @return
* possible object is
* {@link RegistrationParameters1 }
*
*/
public RegistrationParameters1 getAddtlPhysOrRegnDtls() {
return addtlPhysOrRegnDtls;
}
/**
* Sets the value of the addtlPhysOrRegnDtls property.
*
* @param value
* allowed object is
* {@link RegistrationParameters1 }
*
*/
public SecuritiesSettlementTransactionInstructionV02 setAddtlPhysOrRegnDtls(RegistrationParameters1 value) {
this.addtlPhysOrRegnDtls = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the lnkgs list.
* @see #getLnkgs()
*
*/
public SecuritiesSettlementTransactionInstructionV02 addLnkgs(Linkages7 lnkgs) {
getLnkgs().add(lnkgs);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public SecuritiesSettlementTransactionInstructionV02 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}