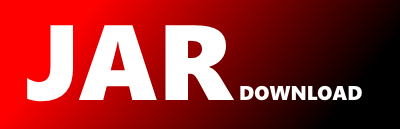
com.prowidesoftware.swift.model.mx.dic.SecuritiesTradeDetails35 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Details of the securities trade.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesTradeDetails35", propOrder = {
"acctOwnrTxId",
"acctSvcrTxId",
"mktInfrstrctrTxId",
"prcrTxId",
"tradId",
"cmonId",
"poolId",
"collTxId",
"sctiesMvmntTp",
"pmt",
"sts",
"plcOfTrad",
"plcOfClr",
"tradDt",
"sttlmDt",
"dealPric",
"nbOfDaysAcrd",
"finInstrmId",
"finInstrmAttrbts",
"tradTxCond",
"tpOfPric",
"qtyAndAcctDtls",
"sctiesFincgDtls",
"sttlmParams",
"dlvrgSttlmPties",
"rcvgSttlmPties",
"sttlmAmt",
"othrAmts",
"othrBizPties",
"splmtryData"
})
public class SecuritiesTradeDetails35 {
@XmlElement(name = "AcctOwnrTxId")
protected String acctOwnrTxId;
@XmlElement(name = "AcctSvcrTxId")
protected String acctSvcrTxId;
@XmlElement(name = "MktInfrstrctrTxId")
protected String mktInfrstrctrTxId;
@XmlElement(name = "PrcrTxId")
protected String prcrTxId;
@XmlElement(name = "TradId")
protected List tradId;
@XmlElement(name = "CmonId")
protected String cmonId;
@XmlElement(name = "PoolId")
protected String poolId;
@XmlElement(name = "CollTxId")
protected List collTxId;
@XmlElement(name = "SctiesMvmntTp", required = true)
@XmlSchemaType(name = "string")
protected ReceiveDelivery1Code sctiesMvmntTp;
@XmlElement(name = "Pmt", required = true)
@XmlSchemaType(name = "string")
protected DeliveryReceiptType2Code pmt;
@XmlElement(name = "Sts")
protected AllegementStatus1Choice sts;
@XmlElement(name = "PlcOfTrad")
protected MarketIdentification78 plcOfTrad;
@XmlElement(name = "PlcOfClr")
protected String plcOfClr;
@XmlElement(name = "TradDt")
protected TradeDate1Choice tradDt;
@XmlElement(name = "SttlmDt", required = true)
protected SettlementDate1Choice sttlmDt;
@XmlElement(name = "DealPric")
protected Price2 dealPric;
@XmlElement(name = "NbOfDaysAcrd")
protected BigDecimal nbOfDaysAcrd;
@XmlElement(name = "FinInstrmId", required = true)
protected SecurityIdentification14 finInstrmId;
@XmlElement(name = "FinInstrmAttrbts")
protected FinancialInstrumentAttributes35 finInstrmAttrbts;
@XmlElement(name = "TradTxCond")
protected List tradTxCond;
@XmlElement(name = "TpOfPric")
protected TypeOfPrice3Choice tpOfPric;
@XmlElement(name = "QtyAndAcctDtls", required = true)
protected QuantityAndAccount26 qtyAndAcctDtls;
@XmlElement(name = "SctiesFincgDtls")
protected SecuritiesFinancingTransactionDetails7 sctiesFincgDtls;
@XmlElement(name = "SttlmParams", required = true)
protected SettlementDetails49 sttlmParams;
@XmlElement(name = "DlvrgSttlmPties")
protected SettlementParties11 dlvrgSttlmPties;
@XmlElement(name = "RcvgSttlmPties")
protected SettlementParties11 rcvgSttlmPties;
@XmlElement(name = "SttlmAmt")
protected AmountAndDirection22 sttlmAmt;
@XmlElement(name = "OthrAmts")
protected OtherAmounts8 othrAmts;
@XmlElement(name = "OthrBizPties")
protected OtherParties11 othrBizPties;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the acctOwnrTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAcctOwnrTxId() {
return acctOwnrTxId;
}
/**
* Sets the value of the acctOwnrTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setAcctOwnrTxId(String value) {
this.acctOwnrTxId = value;
return this;
}
/**
* Gets the value of the acctSvcrTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAcctSvcrTxId() {
return acctSvcrTxId;
}
/**
* Sets the value of the acctSvcrTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setAcctSvcrTxId(String value) {
this.acctSvcrTxId = value;
return this;
}
/**
* Gets the value of the mktInfrstrctrTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMktInfrstrctrTxId() {
return mktInfrstrctrTxId;
}
/**
* Sets the value of the mktInfrstrctrTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setMktInfrstrctrTxId(String value) {
this.mktInfrstrctrTxId = value;
return this;
}
/**
* Gets the value of the prcrTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrcrTxId() {
return prcrTxId;
}
/**
* Sets the value of the prcrTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setPrcrTxId(String value) {
this.prcrTxId = value;
return this;
}
/**
* Gets the value of the tradId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tradId property.
*
*
* For example, to add a new item, do as follows:
*
* getTradId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the tradId property.
*/
public List getTradId() {
if (tradId == null) {
tradId = new ArrayList<>();
}
return this.tradId;
}
/**
* Gets the value of the cmonId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCmonId() {
return cmonId;
}
/**
* Sets the value of the cmonId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setCmonId(String value) {
this.cmonId = value;
return this;
}
/**
* Gets the value of the poolId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPoolId() {
return poolId;
}
/**
* Sets the value of the poolId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setPoolId(String value) {
this.poolId = value;
return this;
}
/**
* Gets the value of the collTxId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the collTxId property.
*
*
* For example, to add a new item, do as follows:
*
* getCollTxId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the collTxId property.
*/
public List getCollTxId() {
if (collTxId == null) {
collTxId = new ArrayList<>();
}
return this.collTxId;
}
/**
* Gets the value of the sctiesMvmntTp property.
*
* @return
* possible object is
* {@link ReceiveDelivery1Code }
*
*/
public ReceiveDelivery1Code getSctiesMvmntTp() {
return sctiesMvmntTp;
}
/**
* Sets the value of the sctiesMvmntTp property.
*
* @param value
* allowed object is
* {@link ReceiveDelivery1Code }
*
*/
public SecuritiesTradeDetails35 setSctiesMvmntTp(ReceiveDelivery1Code value) {
this.sctiesMvmntTp = value;
return this;
}
/**
* Gets the value of the pmt property.
*
* @return
* possible object is
* {@link DeliveryReceiptType2Code }
*
*/
public DeliveryReceiptType2Code getPmt() {
return pmt;
}
/**
* Sets the value of the pmt property.
*
* @param value
* allowed object is
* {@link DeliveryReceiptType2Code }
*
*/
public SecuritiesTradeDetails35 setPmt(DeliveryReceiptType2Code value) {
this.pmt = value;
return this;
}
/**
* Gets the value of the sts property.
*
* @return
* possible object is
* {@link AllegementStatus1Choice }
*
*/
public AllegementStatus1Choice getSts() {
return sts;
}
/**
* Sets the value of the sts property.
*
* @param value
* allowed object is
* {@link AllegementStatus1Choice }
*
*/
public SecuritiesTradeDetails35 setSts(AllegementStatus1Choice value) {
this.sts = value;
return this;
}
/**
* Gets the value of the plcOfTrad property.
*
* @return
* possible object is
* {@link MarketIdentification78 }
*
*/
public MarketIdentification78 getPlcOfTrad() {
return plcOfTrad;
}
/**
* Sets the value of the plcOfTrad property.
*
* @param value
* allowed object is
* {@link MarketIdentification78 }
*
*/
public SecuritiesTradeDetails35 setPlcOfTrad(MarketIdentification78 value) {
this.plcOfTrad = value;
return this;
}
/**
* Gets the value of the plcOfClr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPlcOfClr() {
return plcOfClr;
}
/**
* Sets the value of the plcOfClr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails35 setPlcOfClr(String value) {
this.plcOfClr = value;
return this;
}
/**
* Gets the value of the tradDt property.
*
* @return
* possible object is
* {@link TradeDate1Choice }
*
*/
public TradeDate1Choice getTradDt() {
return tradDt;
}
/**
* Sets the value of the tradDt property.
*
* @param value
* allowed object is
* {@link TradeDate1Choice }
*
*/
public SecuritiesTradeDetails35 setTradDt(TradeDate1Choice value) {
this.tradDt = value;
return this;
}
/**
* Gets the value of the sttlmDt property.
*
* @return
* possible object is
* {@link SettlementDate1Choice }
*
*/
public SettlementDate1Choice getSttlmDt() {
return sttlmDt;
}
/**
* Sets the value of the sttlmDt property.
*
* @param value
* allowed object is
* {@link SettlementDate1Choice }
*
*/
public SecuritiesTradeDetails35 setSttlmDt(SettlementDate1Choice value) {
this.sttlmDt = value;
return this;
}
/**
* Gets the value of the dealPric property.
*
* @return
* possible object is
* {@link Price2 }
*
*/
public Price2 getDealPric() {
return dealPric;
}
/**
* Sets the value of the dealPric property.
*
* @param value
* allowed object is
* {@link Price2 }
*
*/
public SecuritiesTradeDetails35 setDealPric(Price2 value) {
this.dealPric = value;
return this;
}
/**
* Gets the value of the nbOfDaysAcrd property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getNbOfDaysAcrd() {
return nbOfDaysAcrd;
}
/**
* Sets the value of the nbOfDaysAcrd property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public SecuritiesTradeDetails35 setNbOfDaysAcrd(BigDecimal value) {
this.nbOfDaysAcrd = value;
return this;
}
/**
* Gets the value of the finInstrmId property.
*
* @return
* possible object is
* {@link SecurityIdentification14 }
*
*/
public SecurityIdentification14 getFinInstrmId() {
return finInstrmId;
}
/**
* Sets the value of the finInstrmId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification14 }
*
*/
public SecuritiesTradeDetails35 setFinInstrmId(SecurityIdentification14 value) {
this.finInstrmId = value;
return this;
}
/**
* Gets the value of the finInstrmAttrbts property.
*
* @return
* possible object is
* {@link FinancialInstrumentAttributes35 }
*
*/
public FinancialInstrumentAttributes35 getFinInstrmAttrbts() {
return finInstrmAttrbts;
}
/**
* Sets the value of the finInstrmAttrbts property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentAttributes35 }
*
*/
public SecuritiesTradeDetails35 setFinInstrmAttrbts(FinancialInstrumentAttributes35 value) {
this.finInstrmAttrbts = value;
return this;
}
/**
* Gets the value of the tradTxCond property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tradTxCond property.
*
*
* For example, to add a new item, do as follows:
*
* getTradTxCond().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TradeTransactionCondition1Choice }
*
*
* @return
* The value of the tradTxCond property.
*/
public List getTradTxCond() {
if (tradTxCond == null) {
tradTxCond = new ArrayList<>();
}
return this.tradTxCond;
}
/**
* Gets the value of the tpOfPric property.
*
* @return
* possible object is
* {@link TypeOfPrice3Choice }
*
*/
public TypeOfPrice3Choice getTpOfPric() {
return tpOfPric;
}
/**
* Sets the value of the tpOfPric property.
*
* @param value
* allowed object is
* {@link TypeOfPrice3Choice }
*
*/
public SecuritiesTradeDetails35 setTpOfPric(TypeOfPrice3Choice value) {
this.tpOfPric = value;
return this;
}
/**
* Gets the value of the qtyAndAcctDtls property.
*
* @return
* possible object is
* {@link QuantityAndAccount26 }
*
*/
public QuantityAndAccount26 getQtyAndAcctDtls() {
return qtyAndAcctDtls;
}
/**
* Sets the value of the qtyAndAcctDtls property.
*
* @param value
* allowed object is
* {@link QuantityAndAccount26 }
*
*/
public SecuritiesTradeDetails35 setQtyAndAcctDtls(QuantityAndAccount26 value) {
this.qtyAndAcctDtls = value;
return this;
}
/**
* Gets the value of the sctiesFincgDtls property.
*
* @return
* possible object is
* {@link SecuritiesFinancingTransactionDetails7 }
*
*/
public SecuritiesFinancingTransactionDetails7 getSctiesFincgDtls() {
return sctiesFincgDtls;
}
/**
* Sets the value of the sctiesFincgDtls property.
*
* @param value
* allowed object is
* {@link SecuritiesFinancingTransactionDetails7 }
*
*/
public SecuritiesTradeDetails35 setSctiesFincgDtls(SecuritiesFinancingTransactionDetails7 value) {
this.sctiesFincgDtls = value;
return this;
}
/**
* Gets the value of the sttlmParams property.
*
* @return
* possible object is
* {@link SettlementDetails49 }
*
*/
public SettlementDetails49 getSttlmParams() {
return sttlmParams;
}
/**
* Sets the value of the sttlmParams property.
*
* @param value
* allowed object is
* {@link SettlementDetails49 }
*
*/
public SecuritiesTradeDetails35 setSttlmParams(SettlementDetails49 value) {
this.sttlmParams = value;
return this;
}
/**
* Gets the value of the dlvrgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties11 }
*
*/
public SettlementParties11 getDlvrgSttlmPties() {
return dlvrgSttlmPties;
}
/**
* Sets the value of the dlvrgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties11 }
*
*/
public SecuritiesTradeDetails35 setDlvrgSttlmPties(SettlementParties11 value) {
this.dlvrgSttlmPties = value;
return this;
}
/**
* Gets the value of the rcvgSttlmPties property.
*
* @return
* possible object is
* {@link SettlementParties11 }
*
*/
public SettlementParties11 getRcvgSttlmPties() {
return rcvgSttlmPties;
}
/**
* Sets the value of the rcvgSttlmPties property.
*
* @param value
* allowed object is
* {@link SettlementParties11 }
*
*/
public SecuritiesTradeDetails35 setRcvgSttlmPties(SettlementParties11 value) {
this.rcvgSttlmPties = value;
return this;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link AmountAndDirection22 }
*
*/
public AmountAndDirection22 getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link AmountAndDirection22 }
*
*/
public SecuritiesTradeDetails35 setSttlmAmt(AmountAndDirection22 value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the othrAmts property.
*
* @return
* possible object is
* {@link OtherAmounts8 }
*
*/
public OtherAmounts8 getOthrAmts() {
return othrAmts;
}
/**
* Sets the value of the othrAmts property.
*
* @param value
* allowed object is
* {@link OtherAmounts8 }
*
*/
public SecuritiesTradeDetails35 setOthrAmts(OtherAmounts8 value) {
this.othrAmts = value;
return this;
}
/**
* Gets the value of the othrBizPties property.
*
* @return
* possible object is
* {@link OtherParties11 }
*
*/
public OtherParties11 getOthrBizPties() {
return othrBizPties;
}
/**
* Sets the value of the othrBizPties property.
*
* @param value
* allowed object is
* {@link OtherParties11 }
*
*/
public SecuritiesTradeDetails35 setOthrBizPties(OtherParties11 value) {
this.othrBizPties = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the tradId list.
* @see #getTradId()
*
*/
public SecuritiesTradeDetails35 addTradId(String tradId) {
getTradId().add(tradId);
return this;
}
/**
* Adds a new item to the collTxId list.
* @see #getCollTxId()
*
*/
public SecuritiesTradeDetails35 addCollTxId(String collTxId) {
getCollTxId().add(collTxId);
return this;
}
/**
* Adds a new item to the tradTxCond list.
* @see #getTradTxCond()
*
*/
public SecuritiesTradeDetails35 addTradTxCond(TradeTransactionCondition1Choice tradTxCond) {
getTradTxCond().add(tradTxCond);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public SecuritiesTradeDetails35 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}