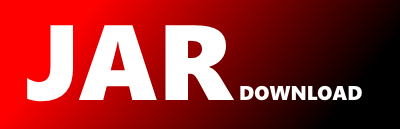
com.prowidesoftware.swift.model.mx.dic.SecuritiesTradeDetails70 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Details of the securities trade.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SecuritiesTradeDetails70", propOrder = {
"tradId",
"collTxId",
"plcOfTrad",
"plcOfClr",
"tradDt",
"sttlmDt",
"lateDlvryDt",
"ackdStsTmStmp",
"mtchdStsTmStmp",
"dealPric",
"nbOfDaysAcrd",
"opngClsg",
"rptg",
"tradTxCond",
"invstrCpcty",
"tradOrgtrRole",
"tpOfPric",
"ccyToBuyOrSell",
"mtchgSts",
"affirmSts",
"fxAddtlDtls",
"sttlmInstrPrcgAddtlDtls"
})
public class SecuritiesTradeDetails70 {
@XmlElement(name = "TradId")
protected List tradId;
@XmlElement(name = "CollTxId")
protected List collTxId;
@XmlElement(name = "PlcOfTrad")
protected PlaceOfTradeIdentification2 plcOfTrad;
@XmlElement(name = "PlcOfClr")
protected PlaceOfClearingIdentification1 plcOfClr;
@XmlElement(name = "TradDt")
protected TradeDate6Choice tradDt;
@XmlElement(name = "SttlmDt", required = true)
protected SettlementDate12Choice sttlmDt;
@XmlElement(name = "LateDlvryDt")
protected DateAndDateTimeChoice lateDlvryDt;
@XmlElement(name = "AckdStsTmStmp", type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime ackdStsTmStmp;
@XmlElement(name = "MtchdStsTmStmp", type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime mtchdStsTmStmp;
@XmlElement(name = "DealPric")
protected Price3 dealPric;
@XmlElement(name = "NbOfDaysAcrd")
protected BigDecimal nbOfDaysAcrd;
@XmlElement(name = "OpngClsg")
protected OpeningClosing4Choice opngClsg;
@XmlElement(name = "Rptg")
protected List rptg;
@XmlElement(name = "TradTxCond")
protected List tradTxCond;
@XmlElement(name = "InvstrCpcty")
protected InvestorCapacity5Choice invstrCpcty;
@XmlElement(name = "TradOrgtrRole")
protected TradeOriginator4Choice tradOrgtrRole;
@XmlElement(name = "TpOfPric")
protected TypeOfPrice32Choice tpOfPric;
@XmlElement(name = "CcyToBuyOrSell")
protected CurrencyToBuyOrSell1Choice ccyToBuyOrSell;
@XmlElement(name = "MtchgSts")
protected MatchingStatus28Choice mtchgSts;
@XmlElement(name = "AffirmSts")
protected AffirmationStatus9Choice affirmSts;
@XmlElement(name = "FxAddtlDtls")
protected String fxAddtlDtls;
@XmlElement(name = "SttlmInstrPrcgAddtlDtls")
protected String sttlmInstrPrcgAddtlDtls;
/**
* Gets the value of the tradId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tradId property.
*
*
* For example, to add a new item, do as follows:
*
* getTradId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the tradId property.
*/
public List getTradId() {
if (tradId == null) {
tradId = new ArrayList<>();
}
return this.tradId;
}
/**
* Gets the value of the collTxId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the collTxId property.
*
*
* For example, to add a new item, do as follows:
*
* getCollTxId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the collTxId property.
*/
public List getCollTxId() {
if (collTxId == null) {
collTxId = new ArrayList<>();
}
return this.collTxId;
}
/**
* Gets the value of the plcOfTrad property.
*
* @return
* possible object is
* {@link PlaceOfTradeIdentification2 }
*
*/
public PlaceOfTradeIdentification2 getPlcOfTrad() {
return plcOfTrad;
}
/**
* Sets the value of the plcOfTrad property.
*
* @param value
* allowed object is
* {@link PlaceOfTradeIdentification2 }
*
*/
public SecuritiesTradeDetails70 setPlcOfTrad(PlaceOfTradeIdentification2 value) {
this.plcOfTrad = value;
return this;
}
/**
* Gets the value of the plcOfClr property.
*
* @return
* possible object is
* {@link PlaceOfClearingIdentification1 }
*
*/
public PlaceOfClearingIdentification1 getPlcOfClr() {
return plcOfClr;
}
/**
* Sets the value of the plcOfClr property.
*
* @param value
* allowed object is
* {@link PlaceOfClearingIdentification1 }
*
*/
public SecuritiesTradeDetails70 setPlcOfClr(PlaceOfClearingIdentification1 value) {
this.plcOfClr = value;
return this;
}
/**
* Gets the value of the tradDt property.
*
* @return
* possible object is
* {@link TradeDate6Choice }
*
*/
public TradeDate6Choice getTradDt() {
return tradDt;
}
/**
* Sets the value of the tradDt property.
*
* @param value
* allowed object is
* {@link TradeDate6Choice }
*
*/
public SecuritiesTradeDetails70 setTradDt(TradeDate6Choice value) {
this.tradDt = value;
return this;
}
/**
* Gets the value of the sttlmDt property.
*
* @return
* possible object is
* {@link SettlementDate12Choice }
*
*/
public SettlementDate12Choice getSttlmDt() {
return sttlmDt;
}
/**
* Sets the value of the sttlmDt property.
*
* @param value
* allowed object is
* {@link SettlementDate12Choice }
*
*/
public SecuritiesTradeDetails70 setSttlmDt(SettlementDate12Choice value) {
this.sttlmDt = value;
return this;
}
/**
* Gets the value of the lateDlvryDt property.
*
* @return
* possible object is
* {@link DateAndDateTimeChoice }
*
*/
public DateAndDateTimeChoice getLateDlvryDt() {
return lateDlvryDt;
}
/**
* Sets the value of the lateDlvryDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeChoice }
*
*/
public SecuritiesTradeDetails70 setLateDlvryDt(DateAndDateTimeChoice value) {
this.lateDlvryDt = value;
return this;
}
/**
* Gets the value of the ackdStsTmStmp property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getAckdStsTmStmp() {
return ackdStsTmStmp;
}
/**
* Sets the value of the ackdStsTmStmp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails70 setAckdStsTmStmp(OffsetDateTime value) {
this.ackdStsTmStmp = value;
return this;
}
/**
* Gets the value of the mtchdStsTmStmp property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getMtchdStsTmStmp() {
return mtchdStsTmStmp;
}
/**
* Sets the value of the mtchdStsTmStmp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails70 setMtchdStsTmStmp(OffsetDateTime value) {
this.mtchdStsTmStmp = value;
return this;
}
/**
* Gets the value of the dealPric property.
*
* @return
* possible object is
* {@link Price3 }
*
*/
public Price3 getDealPric() {
return dealPric;
}
/**
* Sets the value of the dealPric property.
*
* @param value
* allowed object is
* {@link Price3 }
*
*/
public SecuritiesTradeDetails70 setDealPric(Price3 value) {
this.dealPric = value;
return this;
}
/**
* Gets the value of the nbOfDaysAcrd property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getNbOfDaysAcrd() {
return nbOfDaysAcrd;
}
/**
* Sets the value of the nbOfDaysAcrd property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public SecuritiesTradeDetails70 setNbOfDaysAcrd(BigDecimal value) {
this.nbOfDaysAcrd = value;
return this;
}
/**
* Gets the value of the opngClsg property.
*
* @return
* possible object is
* {@link OpeningClosing4Choice }
*
*/
public OpeningClosing4Choice getOpngClsg() {
return opngClsg;
}
/**
* Sets the value of the opngClsg property.
*
* @param value
* allowed object is
* {@link OpeningClosing4Choice }
*
*/
public SecuritiesTradeDetails70 setOpngClsg(OpeningClosing4Choice value) {
this.opngClsg = value;
return this;
}
/**
* Gets the value of the rptg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rptg property.
*
*
* For example, to add a new item, do as follows:
*
* getRptg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Reporting9Choice }
*
*
* @return
* The value of the rptg property.
*/
public List getRptg() {
if (rptg == null) {
rptg = new ArrayList<>();
}
return this.rptg;
}
/**
* Gets the value of the tradTxCond property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tradTxCond property.
*
*
* For example, to add a new item, do as follows:
*
* getTradTxCond().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TradeTransactionCondition6Choice }
*
*
* @return
* The value of the tradTxCond property.
*/
public List getTradTxCond() {
if (tradTxCond == null) {
tradTxCond = new ArrayList<>();
}
return this.tradTxCond;
}
/**
* Gets the value of the invstrCpcty property.
*
* @return
* possible object is
* {@link InvestorCapacity5Choice }
*
*/
public InvestorCapacity5Choice getInvstrCpcty() {
return invstrCpcty;
}
/**
* Sets the value of the invstrCpcty property.
*
* @param value
* allowed object is
* {@link InvestorCapacity5Choice }
*
*/
public SecuritiesTradeDetails70 setInvstrCpcty(InvestorCapacity5Choice value) {
this.invstrCpcty = value;
return this;
}
/**
* Gets the value of the tradOrgtrRole property.
*
* @return
* possible object is
* {@link TradeOriginator4Choice }
*
*/
public TradeOriginator4Choice getTradOrgtrRole() {
return tradOrgtrRole;
}
/**
* Sets the value of the tradOrgtrRole property.
*
* @param value
* allowed object is
* {@link TradeOriginator4Choice }
*
*/
public SecuritiesTradeDetails70 setTradOrgtrRole(TradeOriginator4Choice value) {
this.tradOrgtrRole = value;
return this;
}
/**
* Gets the value of the tpOfPric property.
*
* @return
* possible object is
* {@link TypeOfPrice32Choice }
*
*/
public TypeOfPrice32Choice getTpOfPric() {
return tpOfPric;
}
/**
* Sets the value of the tpOfPric property.
*
* @param value
* allowed object is
* {@link TypeOfPrice32Choice }
*
*/
public SecuritiesTradeDetails70 setTpOfPric(TypeOfPrice32Choice value) {
this.tpOfPric = value;
return this;
}
/**
* Gets the value of the ccyToBuyOrSell property.
*
* @return
* possible object is
* {@link CurrencyToBuyOrSell1Choice }
*
*/
public CurrencyToBuyOrSell1Choice getCcyToBuyOrSell() {
return ccyToBuyOrSell;
}
/**
* Sets the value of the ccyToBuyOrSell property.
*
* @param value
* allowed object is
* {@link CurrencyToBuyOrSell1Choice }
*
*/
public SecuritiesTradeDetails70 setCcyToBuyOrSell(CurrencyToBuyOrSell1Choice value) {
this.ccyToBuyOrSell = value;
return this;
}
/**
* Gets the value of the mtchgSts property.
*
* @return
* possible object is
* {@link MatchingStatus28Choice }
*
*/
public MatchingStatus28Choice getMtchgSts() {
return mtchgSts;
}
/**
* Sets the value of the mtchgSts property.
*
* @param value
* allowed object is
* {@link MatchingStatus28Choice }
*
*/
public SecuritiesTradeDetails70 setMtchgSts(MatchingStatus28Choice value) {
this.mtchgSts = value;
return this;
}
/**
* Gets the value of the affirmSts property.
*
* @return
* possible object is
* {@link AffirmationStatus9Choice }
*
*/
public AffirmationStatus9Choice getAffirmSts() {
return affirmSts;
}
/**
* Sets the value of the affirmSts property.
*
* @param value
* allowed object is
* {@link AffirmationStatus9Choice }
*
*/
public SecuritiesTradeDetails70 setAffirmSts(AffirmationStatus9Choice value) {
this.affirmSts = value;
return this;
}
/**
* Gets the value of the fxAddtlDtls property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFxAddtlDtls() {
return fxAddtlDtls;
}
/**
* Sets the value of the fxAddtlDtls property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails70 setFxAddtlDtls(String value) {
this.fxAddtlDtls = value;
return this;
}
/**
* Gets the value of the sttlmInstrPrcgAddtlDtls property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSttlmInstrPrcgAddtlDtls() {
return sttlmInstrPrcgAddtlDtls;
}
/**
* Sets the value of the sttlmInstrPrcgAddtlDtls property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SecuritiesTradeDetails70 setSttlmInstrPrcgAddtlDtls(String value) {
this.sttlmInstrPrcgAddtlDtls = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the tradId list.
* @see #getTradId()
*
*/
public SecuritiesTradeDetails70 addTradId(String tradId) {
getTradId().add(tradId);
return this;
}
/**
* Adds a new item to the collTxId list.
* @see #getCollTxId()
*
*/
public SecuritiesTradeDetails70 addCollTxId(String collTxId) {
getCollTxId().add(collTxId);
return this;
}
/**
* Adds a new item to the rptg list.
* @see #getRptg()
*
*/
public SecuritiesTradeDetails70 addRptg(Reporting9Choice rptg) {
getRptg().add(rptg);
return this;
}
/**
* Adds a new item to the tradTxCond list.
* @see #getTradTxCond()
*
*/
public SecuritiesTradeDetails70 addTradTxCond(TradeTransactionCondition6Choice tradTxCond) {
getTradTxCond().add(tradTxCond);
return this;
}
}