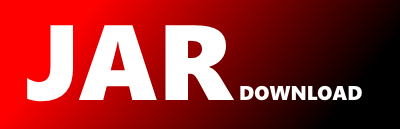
com.prowidesoftware.swift.model.mx.dic.Security14 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.time.LocalDate;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Identification of security used as collateral.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Security14", propOrder = {
"id",
"clssfctnTp",
"qtyOrNmnlVal",
"unitPric",
"mktVal",
"hrcutOrMrgn",
"qlty",
"mtrty",
"issr",
"tp",
"avlblForCollReuse"
})
public class Security14 {
@XmlElement(name = "Id")
protected String id;
@XmlElement(name = "ClssfctnTp")
protected String clssfctnTp;
@XmlElement(name = "QtyOrNmnlVal")
protected QuantityNominalValue1Choice qtyOrNmnlVal;
@XmlElement(name = "UnitPric")
protected SecuritiesTransactionPrice2Choice unitPric;
@XmlElement(name = "MktVal")
protected AmountAndDirection53 mktVal;
@XmlElement(name = "HrcutOrMrgn")
protected BigDecimal hrcutOrMrgn;
@XmlElement(name = "Qlty")
@XmlSchemaType(name = "string")
protected CollateralQualityType1Code qlty;
@XmlElement(name = "Mtrty", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate mtrty;
@XmlElement(name = "Issr")
protected SecurityIssuer2 issr;
@XmlElement(name = "Tp")
protected SecuritiesLendingType3Choice tp;
@XmlElement(name = "AvlblForCollReuse")
protected Boolean avlblForCollReuse;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Security14 setId(String value) {
this.id = value;
return this;
}
/**
* Gets the value of the clssfctnTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClssfctnTp() {
return clssfctnTp;
}
/**
* Sets the value of the clssfctnTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Security14 setClssfctnTp(String value) {
this.clssfctnTp = value;
return this;
}
/**
* Gets the value of the qtyOrNmnlVal property.
*
* @return
* possible object is
* {@link QuantityNominalValue1Choice }
*
*/
public QuantityNominalValue1Choice getQtyOrNmnlVal() {
return qtyOrNmnlVal;
}
/**
* Sets the value of the qtyOrNmnlVal property.
*
* @param value
* allowed object is
* {@link QuantityNominalValue1Choice }
*
*/
public Security14 setQtyOrNmnlVal(QuantityNominalValue1Choice value) {
this.qtyOrNmnlVal = value;
return this;
}
/**
* Gets the value of the unitPric property.
*
* @return
* possible object is
* {@link SecuritiesTransactionPrice2Choice }
*
*/
public SecuritiesTransactionPrice2Choice getUnitPric() {
return unitPric;
}
/**
* Sets the value of the unitPric property.
*
* @param value
* allowed object is
* {@link SecuritiesTransactionPrice2Choice }
*
*/
public Security14 setUnitPric(SecuritiesTransactionPrice2Choice value) {
this.unitPric = value;
return this;
}
/**
* Gets the value of the mktVal property.
*
* @return
* possible object is
* {@link AmountAndDirection53 }
*
*/
public AmountAndDirection53 getMktVal() {
return mktVal;
}
/**
* Sets the value of the mktVal property.
*
* @param value
* allowed object is
* {@link AmountAndDirection53 }
*
*/
public Security14 setMktVal(AmountAndDirection53 value) {
this.mktVal = value;
return this;
}
/**
* Gets the value of the hrcutOrMrgn property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getHrcutOrMrgn() {
return hrcutOrMrgn;
}
/**
* Sets the value of the hrcutOrMrgn property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Security14 setHrcutOrMrgn(BigDecimal value) {
this.hrcutOrMrgn = value;
return this;
}
/**
* Gets the value of the qlty property.
*
* @return
* possible object is
* {@link CollateralQualityType1Code }
*
*/
public CollateralQualityType1Code getQlty() {
return qlty;
}
/**
* Sets the value of the qlty property.
*
* @param value
* allowed object is
* {@link CollateralQualityType1Code }
*
*/
public Security14 setQlty(CollateralQualityType1Code value) {
this.qlty = value;
return this;
}
/**
* Gets the value of the mtrty property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getMtrty() {
return mtrty;
}
/**
* Sets the value of the mtrty property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Security14 setMtrty(LocalDate value) {
this.mtrty = value;
return this;
}
/**
* Gets the value of the issr property.
*
* @return
* possible object is
* {@link SecurityIssuer2 }
*
*/
public SecurityIssuer2 getIssr() {
return issr;
}
/**
* Sets the value of the issr property.
*
* @param value
* allowed object is
* {@link SecurityIssuer2 }
*
*/
public Security14 setIssr(SecurityIssuer2 value) {
this.issr = value;
return this;
}
/**
* Gets the value of the tp property.
*
* @return
* possible object is
* {@link SecuritiesLendingType3Choice }
*
*/
public SecuritiesLendingType3Choice getTp() {
return tp;
}
/**
* Sets the value of the tp property.
*
* @param value
* allowed object is
* {@link SecuritiesLendingType3Choice }
*
*/
public Security14 setTp(SecuritiesLendingType3Choice value) {
this.tp = value;
return this;
}
/**
* Gets the value of the avlblForCollReuse property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAvlblForCollReuse() {
return avlblForCollReuse;
}
/**
* Sets the value of the avlblForCollReuse property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public Security14 setAvlblForCollReuse(Boolean value) {
this.avlblForCollReuse = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy