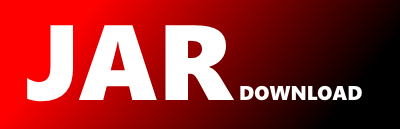
com.prowidesoftware.swift.model.mx.dic.Setr00100102 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The RedemptionBulkOrder message is sent by an instructing party, eg, an investment manager or its authorised representative, to an executing party, eg, a transfer agent. There may be one or more intermediary parties between the instructing party and the executing party. The intermediary party is, for example, an intermediary or a concentrator.
* This message is used to instruct the executing party to redeem a financial instrument for two or more accounts.
* Usage
* The RedemptionBulkOrder message is used to bulk several individual orders into one bulk order. The individual orders come from different instructing parties, ie, account owners, but are the same financial instrument. The RedemptionBulkOrder can result in one single bulk cash settlement or several individual cash settlements.
* This message will be typically used by a party collecting orders and bulking these individual orders into one bulk order before sending it to another party.
* For a single redemption order, the RedemptionMultipleOrder message, not the RedemptionBulkOrder message, must be used.
* If there are redemption orders for different financial instruments but for the same account, then the RedemptionMultipleOrder must be used.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "setr.001.001.02", propOrder = {
"mstrRef",
"poolRef",
"prvsRef",
"blkOrdrDtls",
"intrmyDtls",
"cpyDtls",
"xtnsn"
})
public class Setr00100102 {
@XmlElement(name = "MstrRef")
protected AdditionalReference3 mstrRef;
@XmlElement(name = "PoolRef")
protected AdditionalReference3 poolRef;
@XmlElement(name = "PrvsRef")
protected List prvsRef;
@XmlElement(name = "BlkOrdrDtls", required = true)
protected RedemptionBulkOrder2 blkOrdrDtls;
@XmlElement(name = "IntrmyDtls")
protected List intrmyDtls;
@XmlElement(name = "CpyDtls")
protected CopyInformation1 cpyDtls;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the mstrRef property.
*
* @return
* possible object is
* {@link AdditionalReference3 }
*
*/
public AdditionalReference3 getMstrRef() {
return mstrRef;
}
/**
* Sets the value of the mstrRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference3 }
*
*/
public Setr00100102 setMstrRef(AdditionalReference3 value) {
this.mstrRef = value;
return this;
}
/**
* Gets the value of the poolRef property.
*
* @return
* possible object is
* {@link AdditionalReference3 }
*
*/
public AdditionalReference3 getPoolRef() {
return poolRef;
}
/**
* Sets the value of the poolRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference3 }
*
*/
public Setr00100102 setPoolRef(AdditionalReference3 value) {
this.poolRef = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the prvsRef property.
*
*
* For example, to add a new item, do as follows:
*
* getPrvsRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalReference3 }
*
*
* @return
* The value of the prvsRef property.
*/
public List getPrvsRef() {
if (prvsRef == null) {
prvsRef = new ArrayList<>();
}
return this.prvsRef;
}
/**
* Gets the value of the blkOrdrDtls property.
*
* @return
* possible object is
* {@link RedemptionBulkOrder2 }
*
*/
public RedemptionBulkOrder2 getBlkOrdrDtls() {
return blkOrdrDtls;
}
/**
* Sets the value of the blkOrdrDtls property.
*
* @param value
* allowed object is
* {@link RedemptionBulkOrder2 }
*
*/
public Setr00100102 setBlkOrdrDtls(RedemptionBulkOrder2 value) {
this.blkOrdrDtls = value;
return this;
}
/**
* Gets the value of the intrmyDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrmyDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrmyDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary4 }
*
*
* @return
* The value of the intrmyDtls property.
*/
public List getIntrmyDtls() {
if (intrmyDtls == null) {
intrmyDtls = new ArrayList<>();
}
return this.intrmyDtls;
}
/**
* Gets the value of the cpyDtls property.
*
* @return
* possible object is
* {@link CopyInformation1 }
*
*/
public CopyInformation1 getCpyDtls() {
return cpyDtls;
}
/**
* Sets the value of the cpyDtls property.
*
* @param value
* allowed object is
* {@link CopyInformation1 }
*
*/
public Setr00100102 setCpyDtls(CopyInformation1 value) {
this.cpyDtls = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the prvsRef list.
* @see #getPrvsRef()
*
*/
public Setr00100102 addPrvsRef(AdditionalReference3 prvsRef) {
getPrvsRef().add(prvsRef);
return this;
}
/**
* Adds a new item to the intrmyDtls list.
* @see #getIntrmyDtls()
*
*/
public Setr00100102 addIntrmyDtls(Intermediary4 intrmyDtls) {
getIntrmyDtls().add(intrmyDtls);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public Setr00100102 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}