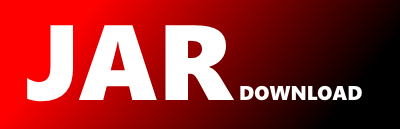
com.prowidesoftware.swift.model.mx.dic.SubscriptionMultipleExecution2 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Execution of a subscription order.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SubscriptionMultipleExecution2", propOrder = {
"plcOfTrad",
"ordrDtTm",
"cxlRght",
"invstmtAcctDtls",
"bnfcryDtls",
"indvExctnDtls",
"blkCshSttlmDtls"
})
public class SubscriptionMultipleExecution2 {
@XmlElement(name = "PlcOfTrad")
protected String plcOfTrad;
@XmlElement(name = "OrdrDtTm", type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime ordrDtTm;
@XmlElement(name = "CxlRght")
protected CancellationRight1 cxlRght;
@XmlElement(name = "InvstmtAcctDtls", required = true)
protected InvestmentAccount13 invstmtAcctDtls;
@XmlElement(name = "BnfcryDtls")
protected IndividualPerson2 bnfcryDtls;
@XmlElement(name = "IndvExctnDtls", required = true)
protected List indvExctnDtls;
@XmlElement(name = "BlkCshSttlmDtls")
protected PaymentTransaction13 blkCshSttlmDtls;
/**
* Gets the value of the plcOfTrad property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPlcOfTrad() {
return plcOfTrad;
}
/**
* Sets the value of the plcOfTrad property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SubscriptionMultipleExecution2 setPlcOfTrad(String value) {
this.plcOfTrad = value;
return this;
}
/**
* Gets the value of the ordrDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getOrdrDtTm() {
return ordrDtTm;
}
/**
* Sets the value of the ordrDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SubscriptionMultipleExecution2 setOrdrDtTm(OffsetDateTime value) {
this.ordrDtTm = value;
return this;
}
/**
* Gets the value of the cxlRght property.
*
* @return
* possible object is
* {@link CancellationRight1 }
*
*/
public CancellationRight1 getCxlRght() {
return cxlRght;
}
/**
* Sets the value of the cxlRght property.
*
* @param value
* allowed object is
* {@link CancellationRight1 }
*
*/
public SubscriptionMultipleExecution2 setCxlRght(CancellationRight1 value) {
this.cxlRght = value;
return this;
}
/**
* Gets the value of the invstmtAcctDtls property.
*
* @return
* possible object is
* {@link InvestmentAccount13 }
*
*/
public InvestmentAccount13 getInvstmtAcctDtls() {
return invstmtAcctDtls;
}
/**
* Sets the value of the invstmtAcctDtls property.
*
* @param value
* allowed object is
* {@link InvestmentAccount13 }
*
*/
public SubscriptionMultipleExecution2 setInvstmtAcctDtls(InvestmentAccount13 value) {
this.invstmtAcctDtls = value;
return this;
}
/**
* Gets the value of the bnfcryDtls property.
*
* @return
* possible object is
* {@link IndividualPerson2 }
*
*/
public IndividualPerson2 getBnfcryDtls() {
return bnfcryDtls;
}
/**
* Sets the value of the bnfcryDtls property.
*
* @param value
* allowed object is
* {@link IndividualPerson2 }
*
*/
public SubscriptionMultipleExecution2 setBnfcryDtls(IndividualPerson2 value) {
this.bnfcryDtls = value;
return this;
}
/**
* Gets the value of the indvExctnDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the indvExctnDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getIndvExctnDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SubscriptionExecution4 }
*
*
* @return
* The value of the indvExctnDtls property.
*/
public List getIndvExctnDtls() {
if (indvExctnDtls == null) {
indvExctnDtls = new ArrayList<>();
}
return this.indvExctnDtls;
}
/**
* Gets the value of the blkCshSttlmDtls property.
*
* @return
* possible object is
* {@link PaymentTransaction13 }
*
*/
public PaymentTransaction13 getBlkCshSttlmDtls() {
return blkCshSttlmDtls;
}
/**
* Sets the value of the blkCshSttlmDtls property.
*
* @param value
* allowed object is
* {@link PaymentTransaction13 }
*
*/
public SubscriptionMultipleExecution2 setBlkCshSttlmDtls(PaymentTransaction13 value) {
this.blkCshSttlmDtls = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the indvExctnDtls list.
* @see #getIndvExctnDtls()
*
*/
public SubscriptionMultipleExecution2 addIndvExctnDtls(SubscriptionExecution4 indvExctnDtls) {
getIndvExctnDtls().add(indvExctnDtls);
return this;
}
}