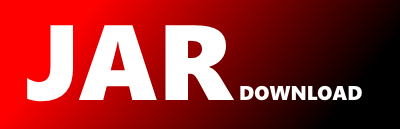
com.prowidesoftware.swift.model.mx.dic.SwitchExecution4 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Execution of a switch order.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SwitchExecution4", propOrder = {
"mstrRef",
"ordrDtTm",
"invstmtAcctDtls",
"dealRef",
"ordrRef",
"clntRef",
"ttlRedAmt",
"ttlSbcptAmt",
"rltdPtyDtls",
"reqdFutrTradDt",
"addtlCshIn",
"rsltgCshOut",
"sttlmAmt",
"cshSttlmDt",
"sttlmMtd",
"bestExctn",
"redLegDtls",
"sbcptLegDtls",
"cshSttlmDtls",
"fxDtls",
"finAdvc",
"ngtdTrad",
"lateRpt"
})
public class SwitchExecution4 {
@XmlElement(name = "MstrRef")
protected String mstrRef;
@XmlElement(name = "OrdrDtTm", type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime ordrDtTm;
@XmlElement(name = "InvstmtAcctDtls")
protected InvestmentAccount21 invstmtAcctDtls;
@XmlElement(name = "DealRef", required = true)
protected String dealRef;
@XmlElement(name = "OrdrRef", required = true)
protected String ordrRef;
@XmlElement(name = "ClntRef")
protected String clntRef;
@XmlElement(name = "TtlRedAmt")
protected ActiveCurrencyAndAmount ttlRedAmt;
@XmlElement(name = "TtlSbcptAmt")
protected ActiveCurrencyAndAmount ttlSbcptAmt;
@XmlElement(name = "RltdPtyDtls")
protected List rltdPtyDtls;
@XmlElement(name = "ReqdFutrTradDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate reqdFutrTradDt;
@XmlElement(name = "AddtlCshIn")
protected ActiveCurrencyAndAmount addtlCshIn;
@XmlElement(name = "RsltgCshOut")
protected ActiveCurrencyAndAmount rsltgCshOut;
@XmlElement(name = "SttlmAmt")
protected ActiveCurrencyAndAmount sttlmAmt;
@XmlElement(name = "CshSttlmDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate cshSttlmDt;
@XmlElement(name = "SttlmMtd")
@XmlSchemaType(name = "string")
protected DeliveryReceiptType2Code sttlmMtd;
@XmlElement(name = "BestExctn")
@XmlSchemaType(name = "string")
protected BestExecution1Code bestExctn;
@XmlElement(name = "RedLegDtls", required = true)
protected List redLegDtls;
@XmlElement(name = "SbcptLegDtls", required = true)
protected List sbcptLegDtls;
@XmlElement(name = "CshSttlmDtls")
protected PaymentTransaction26 cshSttlmDtls;
@XmlElement(name = "FXDtls")
protected List fxDtls;
@XmlElement(name = "FinAdvc")
@XmlSchemaType(name = "string")
protected FinancialAdvice1Code finAdvc;
@XmlElement(name = "NgtdTrad")
@XmlSchemaType(name = "string")
protected NegotiatedTrade1Code ngtdTrad;
@XmlElement(name = "LateRpt")
@XmlSchemaType(name = "string")
protected LateReport1Code lateRpt;
/**
* Gets the value of the mstrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMstrRef() {
return mstrRef;
}
/**
* Sets the value of the mstrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setMstrRef(String value) {
this.mstrRef = value;
return this;
}
/**
* Gets the value of the ordrDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getOrdrDtTm() {
return ordrDtTm;
}
/**
* Sets the value of the ordrDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setOrdrDtTm(OffsetDateTime value) {
this.ordrDtTm = value;
return this;
}
/**
* Gets the value of the invstmtAcctDtls property.
*
* @return
* possible object is
* {@link InvestmentAccount21 }
*
*/
public InvestmentAccount21 getInvstmtAcctDtls() {
return invstmtAcctDtls;
}
/**
* Sets the value of the invstmtAcctDtls property.
*
* @param value
* allowed object is
* {@link InvestmentAccount21 }
*
*/
public SwitchExecution4 setInvstmtAcctDtls(InvestmentAccount21 value) {
this.invstmtAcctDtls = value;
return this;
}
/**
* Gets the value of the dealRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDealRef() {
return dealRef;
}
/**
* Sets the value of the dealRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setDealRef(String value) {
this.dealRef = value;
return this;
}
/**
* Gets the value of the ordrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrdrRef() {
return ordrRef;
}
/**
* Sets the value of the ordrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setOrdrRef(String value) {
this.ordrRef = value;
return this;
}
/**
* Gets the value of the clntRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClntRef() {
return clntRef;
}
/**
* Sets the value of the clntRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setClntRef(String value) {
this.clntRef = value;
return this;
}
/**
* Gets the value of the ttlRedAmt property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getTtlRedAmt() {
return ttlRedAmt;
}
/**
* Sets the value of the ttlRedAmt property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public SwitchExecution4 setTtlRedAmt(ActiveCurrencyAndAmount value) {
this.ttlRedAmt = value;
return this;
}
/**
* Gets the value of the ttlSbcptAmt property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getTtlSbcptAmt() {
return ttlSbcptAmt;
}
/**
* Sets the value of the ttlSbcptAmt property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public SwitchExecution4 setTtlSbcptAmt(ActiveCurrencyAndAmount value) {
this.ttlSbcptAmt = value;
return this;
}
/**
* Gets the value of the rltdPtyDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rltdPtyDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getRltdPtyDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary9 }
*
*
* @return
* The value of the rltdPtyDtls property.
*/
public List getRltdPtyDtls() {
if (rltdPtyDtls == null) {
rltdPtyDtls = new ArrayList<>();
}
return this.rltdPtyDtls;
}
/**
* Gets the value of the reqdFutrTradDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getReqdFutrTradDt() {
return reqdFutrTradDt;
}
/**
* Sets the value of the reqdFutrTradDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setReqdFutrTradDt(LocalDate value) {
this.reqdFutrTradDt = value;
return this;
}
/**
* Gets the value of the addtlCshIn property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getAddtlCshIn() {
return addtlCshIn;
}
/**
* Sets the value of the addtlCshIn property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public SwitchExecution4 setAddtlCshIn(ActiveCurrencyAndAmount value) {
this.addtlCshIn = value;
return this;
}
/**
* Gets the value of the rsltgCshOut property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getRsltgCshOut() {
return rsltgCshOut;
}
/**
* Sets the value of the rsltgCshOut property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public SwitchExecution4 setRsltgCshOut(ActiveCurrencyAndAmount value) {
this.rsltgCshOut = value;
return this;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public SwitchExecution4 setSttlmAmt(ActiveCurrencyAndAmount value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the cshSttlmDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getCshSttlmDt() {
return cshSttlmDt;
}
/**
* Sets the value of the cshSttlmDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public SwitchExecution4 setCshSttlmDt(LocalDate value) {
this.cshSttlmDt = value;
return this;
}
/**
* Gets the value of the sttlmMtd property.
*
* @return
* possible object is
* {@link DeliveryReceiptType2Code }
*
*/
public DeliveryReceiptType2Code getSttlmMtd() {
return sttlmMtd;
}
/**
* Sets the value of the sttlmMtd property.
*
* @param value
* allowed object is
* {@link DeliveryReceiptType2Code }
*
*/
public SwitchExecution4 setSttlmMtd(DeliveryReceiptType2Code value) {
this.sttlmMtd = value;
return this;
}
/**
* Gets the value of the bestExctn property.
*
* @return
* possible object is
* {@link BestExecution1Code }
*
*/
public BestExecution1Code getBestExctn() {
return bestExctn;
}
/**
* Sets the value of the bestExctn property.
*
* @param value
* allowed object is
* {@link BestExecution1Code }
*
*/
public SwitchExecution4 setBestExctn(BestExecution1Code value) {
this.bestExctn = value;
return this;
}
/**
* Gets the value of the redLegDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the redLegDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getRedLegDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SwitchRedemptionLegExecution3 }
*
*
* @return
* The value of the redLegDtls property.
*/
public List getRedLegDtls() {
if (redLegDtls == null) {
redLegDtls = new ArrayList<>();
}
return this.redLegDtls;
}
/**
* Gets the value of the sbcptLegDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the sbcptLegDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getSbcptLegDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SwitchSubscriptionLegExecution3 }
*
*
* @return
* The value of the sbcptLegDtls property.
*/
public List getSbcptLegDtls() {
if (sbcptLegDtls == null) {
sbcptLegDtls = new ArrayList<>();
}
return this.sbcptLegDtls;
}
/**
* Gets the value of the cshSttlmDtls property.
*
* @return
* possible object is
* {@link PaymentTransaction26 }
*
*/
public PaymentTransaction26 getCshSttlmDtls() {
return cshSttlmDtls;
}
/**
* Sets the value of the cshSttlmDtls property.
*
* @param value
* allowed object is
* {@link PaymentTransaction26 }
*
*/
public SwitchExecution4 setCshSttlmDtls(PaymentTransaction26 value) {
this.cshSttlmDtls = value;
return this;
}
/**
* Gets the value of the fxDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the fxDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getFXDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ForeignExchangeTerms7 }
*
*
* @return
* The value of the fxDtls property.
*/
public List getFXDtls() {
if (fxDtls == null) {
fxDtls = new ArrayList<>();
}
return this.fxDtls;
}
/**
* Gets the value of the finAdvc property.
*
* @return
* possible object is
* {@link FinancialAdvice1Code }
*
*/
public FinancialAdvice1Code getFinAdvc() {
return finAdvc;
}
/**
* Sets the value of the finAdvc property.
*
* @param value
* allowed object is
* {@link FinancialAdvice1Code }
*
*/
public SwitchExecution4 setFinAdvc(FinancialAdvice1Code value) {
this.finAdvc = value;
return this;
}
/**
* Gets the value of the ngtdTrad property.
*
* @return
* possible object is
* {@link NegotiatedTrade1Code }
*
*/
public NegotiatedTrade1Code getNgtdTrad() {
return ngtdTrad;
}
/**
* Sets the value of the ngtdTrad property.
*
* @param value
* allowed object is
* {@link NegotiatedTrade1Code }
*
*/
public SwitchExecution4 setNgtdTrad(NegotiatedTrade1Code value) {
this.ngtdTrad = value;
return this;
}
/**
* Gets the value of the lateRpt property.
*
* @return
* possible object is
* {@link LateReport1Code }
*
*/
public LateReport1Code getLateRpt() {
return lateRpt;
}
/**
* Sets the value of the lateRpt property.
*
* @param value
* allowed object is
* {@link LateReport1Code }
*
*/
public SwitchExecution4 setLateRpt(LateReport1Code value) {
this.lateRpt = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the rltdPtyDtls list.
* @see #getRltdPtyDtls()
*
*/
public SwitchExecution4 addRltdPtyDtls(Intermediary9 rltdPtyDtls) {
getRltdPtyDtls().add(rltdPtyDtls);
return this;
}
/**
* Adds a new item to the redLegDtls list.
* @see #getRedLegDtls()
*
*/
public SwitchExecution4 addRedLegDtls(SwitchRedemptionLegExecution3 redLegDtls) {
getRedLegDtls().add(redLegDtls);
return this;
}
/**
* Adds a new item to the sbcptLegDtls list.
* @see #getSbcptLegDtls()
*
*/
public SwitchExecution4 addSbcptLegDtls(SwitchSubscriptionLegExecution3 sbcptLegDtls) {
getSbcptLegDtls().add(sbcptLegDtls);
return this;
}
/**
* Adds a new item to the fXDtls list.
* @see #getFXDtls()
*
*/
public SwitchExecution4 addFXDtls(ForeignExchangeTerms7 fXDtls) {
getFXDtls().add(fXDtls);
return this;
}
}