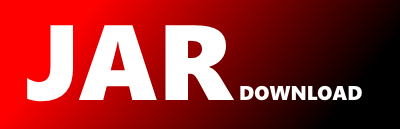
com.prowidesoftware.swift.model.mx.dic.TradeSettlement1 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Trade settlement details for this invoice which involves the payment of an outstanding debt, account, or charge.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TradeSettlement1", propOrder = {
"duePyblAmt",
"cdtrRef",
"pmtRef",
"invcCcyCd",
"invcr",
"invcee",
"pyee",
"pyer",
"taxCcyXchg",
"invcCcyXchg",
"pmtCcyXchg",
"pmtMeans",
"tax",
"bllgPrd",
"allwncChrg",
"subTtlClctdTax",
"logstcsChrg",
"pmtTerms",
"mntrySummtn",
"adjstmntAmtAndRsn",
"invcRefdDoc",
"profrmInvcRefdDoc",
"lttrOfCdtRefdDoc",
"finCard",
"purchsAcctgAcct",
"issrFactrgListId",
"issrFactrgAgrmtId"
})
public class TradeSettlement1 {
@XmlElement(name = "DuePyblAmt")
protected List duePyblAmt;
@XmlElement(name = "CdtrRef")
protected List cdtrRef;
@XmlElement(name = "PmtRef")
protected List pmtRef;
@XmlElement(name = "InvcCcyCd")
protected String invcCcyCd;
@XmlElement(name = "Invcr")
protected TradeParty1 invcr;
@XmlElement(name = "Invcee")
protected TradeParty1 invcee;
@XmlElement(name = "Pyee")
protected TradeParty1 pyee;
@XmlElement(name = "Pyer")
protected TradeParty1 pyer;
@XmlElement(name = "TaxCcyXchg")
protected CurrencyReference2 taxCcyXchg;
@XmlElement(name = "InvcCcyXchg")
protected CurrencyReference2 invcCcyXchg;
@XmlElement(name = "PmtCcyXchg")
protected CurrencyReference2 pmtCcyXchg;
@XmlElement(name = "PmtMeans")
protected List pmtMeans;
@XmlElement(name = "Tax")
protected List tax;
@XmlElement(name = "BllgPrd")
protected Period1 bllgPrd;
@XmlElement(name = "AllwncChrg")
protected List allwncChrg;
@XmlElement(name = "SubTtlClctdTax")
protected List subTtlClctdTax;
@XmlElement(name = "LogstcsChrg")
protected List logstcsChrg;
@XmlElement(name = "PmtTerms")
protected List pmtTerms;
@XmlElement(name = "MntrySummtn", required = true)
protected SettlementMonetarySummation1 mntrySummtn;
@XmlElement(name = "AdjstmntAmtAndRsn")
protected List adjstmntAmtAndRsn;
@XmlElement(name = "InvcRefdDoc")
protected DocumentIdentification22 invcRefdDoc;
@XmlElement(name = "ProfrmInvcRefdDoc")
protected DocumentIdentification22 profrmInvcRefdDoc;
@XmlElement(name = "LttrOfCdtRefdDoc")
protected DocumentIdentification7 lttrOfCdtRefdDoc;
@XmlElement(name = "FinCard")
protected List finCard;
@XmlElement(name = "PurchsAcctgAcct")
protected List purchsAcctgAcct;
@XmlElement(name = "IssrFactrgListId")
protected List issrFactrgListId;
@XmlElement(name = "IssrFactrgAgrmtId")
protected List issrFactrgAgrmtId;
/**
* Gets the value of the duePyblAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the duePyblAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getDuePyblAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
* @return
* The value of the duePyblAmt property.
*/
public List getDuePyblAmt() {
if (duePyblAmt == null) {
duePyblAmt = new ArrayList<>();
}
return this.duePyblAmt;
}
/**
* Gets the value of the cdtrRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cdtrRef property.
*
*
* For example, to add a new item, do as follows:
*
* getCdtrRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CreditorReferenceInformation2 }
*
*
* @return
* The value of the cdtrRef property.
*/
public List getCdtrRef() {
if (cdtrRef == null) {
cdtrRef = new ArrayList<>();
}
return this.cdtrRef;
}
/**
* Gets the value of the pmtRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtRef property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the pmtRef property.
*/
public List getPmtRef() {
if (pmtRef == null) {
pmtRef = new ArrayList<>();
}
return this.pmtRef;
}
/**
* Gets the value of the invcCcyCd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInvcCcyCd() {
return invcCcyCd;
}
/**
* Sets the value of the invcCcyCd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public TradeSettlement1 setInvcCcyCd(String value) {
this.invcCcyCd = value;
return this;
}
/**
* Gets the value of the invcr property.
*
* @return
* possible object is
* {@link TradeParty1 }
*
*/
public TradeParty1 getInvcr() {
return invcr;
}
/**
* Sets the value of the invcr property.
*
* @param value
* allowed object is
* {@link TradeParty1 }
*
*/
public TradeSettlement1 setInvcr(TradeParty1 value) {
this.invcr = value;
return this;
}
/**
* Gets the value of the invcee property.
*
* @return
* possible object is
* {@link TradeParty1 }
*
*/
public TradeParty1 getInvcee() {
return invcee;
}
/**
* Sets the value of the invcee property.
*
* @param value
* allowed object is
* {@link TradeParty1 }
*
*/
public TradeSettlement1 setInvcee(TradeParty1 value) {
this.invcee = value;
return this;
}
/**
* Gets the value of the pyee property.
*
* @return
* possible object is
* {@link TradeParty1 }
*
*/
public TradeParty1 getPyee() {
return pyee;
}
/**
* Sets the value of the pyee property.
*
* @param value
* allowed object is
* {@link TradeParty1 }
*
*/
public TradeSettlement1 setPyee(TradeParty1 value) {
this.pyee = value;
return this;
}
/**
* Gets the value of the pyer property.
*
* @return
* possible object is
* {@link TradeParty1 }
*
*/
public TradeParty1 getPyer() {
return pyer;
}
/**
* Sets the value of the pyer property.
*
* @param value
* allowed object is
* {@link TradeParty1 }
*
*/
public TradeSettlement1 setPyer(TradeParty1 value) {
this.pyer = value;
return this;
}
/**
* Gets the value of the taxCcyXchg property.
*
* @return
* possible object is
* {@link CurrencyReference2 }
*
*/
public CurrencyReference2 getTaxCcyXchg() {
return taxCcyXchg;
}
/**
* Sets the value of the taxCcyXchg property.
*
* @param value
* allowed object is
* {@link CurrencyReference2 }
*
*/
public TradeSettlement1 setTaxCcyXchg(CurrencyReference2 value) {
this.taxCcyXchg = value;
return this;
}
/**
* Gets the value of the invcCcyXchg property.
*
* @return
* possible object is
* {@link CurrencyReference2 }
*
*/
public CurrencyReference2 getInvcCcyXchg() {
return invcCcyXchg;
}
/**
* Sets the value of the invcCcyXchg property.
*
* @param value
* allowed object is
* {@link CurrencyReference2 }
*
*/
public TradeSettlement1 setInvcCcyXchg(CurrencyReference2 value) {
this.invcCcyXchg = value;
return this;
}
/**
* Gets the value of the pmtCcyXchg property.
*
* @return
* possible object is
* {@link CurrencyReference2 }
*
*/
public CurrencyReference2 getPmtCcyXchg() {
return pmtCcyXchg;
}
/**
* Sets the value of the pmtCcyXchg property.
*
* @param value
* allowed object is
* {@link CurrencyReference2 }
*
*/
public TradeSettlement1 setPmtCcyXchg(CurrencyReference2 value) {
this.pmtCcyXchg = value;
return this;
}
/**
* Gets the value of the pmtMeans property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtMeans property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtMeans().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentMeans1 }
*
*
* @return
* The value of the pmtMeans property.
*/
public List getPmtMeans() {
if (pmtMeans == null) {
pmtMeans = new ArrayList<>();
}
return this.pmtMeans;
}
/**
* Gets the value of the tax property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tax property.
*
*
* For example, to add a new item, do as follows:
*
* getTax().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SettlementTax1 }
*
*
* @return
* The value of the tax property.
*/
public List getTax() {
if (tax == null) {
tax = new ArrayList<>();
}
return this.tax;
}
/**
* Gets the value of the bllgPrd property.
*
* @return
* possible object is
* {@link Period1 }
*
*/
public Period1 getBllgPrd() {
return bllgPrd;
}
/**
* Sets the value of the bllgPrd property.
*
* @param value
* allowed object is
* {@link Period1 }
*
*/
public TradeSettlement1 setBllgPrd(Period1 value) {
this.bllgPrd = value;
return this;
}
/**
* Gets the value of the allwncChrg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the allwncChrg property.
*
*
* For example, to add a new item, do as follows:
*
* getAllwncChrg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SettlementAllowanceCharge1 }
*
*
* @return
* The value of the allwncChrg property.
*/
public List getAllwncChrg() {
if (allwncChrg == null) {
allwncChrg = new ArrayList<>();
}
return this.allwncChrg;
}
/**
* Gets the value of the subTtlClctdTax property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the subTtlClctdTax property.
*
*
* For example, to add a new item, do as follows:
*
* getSubTtlClctdTax().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SettlementSubTotalCalculatedTax1 }
*
*
* @return
* The value of the subTtlClctdTax property.
*/
public List getSubTtlClctdTax() {
if (subTtlClctdTax == null) {
subTtlClctdTax = new ArrayList<>();
}
return this.subTtlClctdTax;
}
/**
* Gets the value of the logstcsChrg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the logstcsChrg property.
*
*
* For example, to add a new item, do as follows:
*
* getLogstcsChrg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ChargesDetails2 }
*
*
* @return
* The value of the logstcsChrg property.
*/
public List getLogstcsChrg() {
if (logstcsChrg == null) {
logstcsChrg = new ArrayList<>();
}
return this.logstcsChrg;
}
/**
* Gets the value of the pmtTerms property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtTerms property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtTerms().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentTerms3 }
*
*
* @return
* The value of the pmtTerms property.
*/
public List getPmtTerms() {
if (pmtTerms == null) {
pmtTerms = new ArrayList<>();
}
return this.pmtTerms;
}
/**
* Gets the value of the mntrySummtn property.
*
* @return
* possible object is
* {@link SettlementMonetarySummation1 }
*
*/
public SettlementMonetarySummation1 getMntrySummtn() {
return mntrySummtn;
}
/**
* Sets the value of the mntrySummtn property.
*
* @param value
* allowed object is
* {@link SettlementMonetarySummation1 }
*
*/
public TradeSettlement1 setMntrySummtn(SettlementMonetarySummation1 value) {
this.mntrySummtn = value;
return this;
}
/**
* Gets the value of the adjstmntAmtAndRsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the adjstmntAmtAndRsn property.
*
*
* For example, to add a new item, do as follows:
*
* getAdjstmntAmtAndRsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DocumentAdjustment2 }
*
*
* @return
* The value of the adjstmntAmtAndRsn property.
*/
public List getAdjstmntAmtAndRsn() {
if (adjstmntAmtAndRsn == null) {
adjstmntAmtAndRsn = new ArrayList<>();
}
return this.adjstmntAmtAndRsn;
}
/**
* Gets the value of the invcRefdDoc property.
*
* @return
* possible object is
* {@link DocumentIdentification22 }
*
*/
public DocumentIdentification22 getInvcRefdDoc() {
return invcRefdDoc;
}
/**
* Sets the value of the invcRefdDoc property.
*
* @param value
* allowed object is
* {@link DocumentIdentification22 }
*
*/
public TradeSettlement1 setInvcRefdDoc(DocumentIdentification22 value) {
this.invcRefdDoc = value;
return this;
}
/**
* Gets the value of the profrmInvcRefdDoc property.
*
* @return
* possible object is
* {@link DocumentIdentification22 }
*
*/
public DocumentIdentification22 getProfrmInvcRefdDoc() {
return profrmInvcRefdDoc;
}
/**
* Sets the value of the profrmInvcRefdDoc property.
*
* @param value
* allowed object is
* {@link DocumentIdentification22 }
*
*/
public TradeSettlement1 setProfrmInvcRefdDoc(DocumentIdentification22 value) {
this.profrmInvcRefdDoc = value;
return this;
}
/**
* Gets the value of the lttrOfCdtRefdDoc property.
*
* @return
* possible object is
* {@link DocumentIdentification7 }
*
*/
public DocumentIdentification7 getLttrOfCdtRefdDoc() {
return lttrOfCdtRefdDoc;
}
/**
* Sets the value of the lttrOfCdtRefdDoc property.
*
* @param value
* allowed object is
* {@link DocumentIdentification7 }
*
*/
public TradeSettlement1 setLttrOfCdtRefdDoc(DocumentIdentification7 value) {
this.lttrOfCdtRefdDoc = value;
return this;
}
/**
* Gets the value of the finCard property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the finCard property.
*
*
* For example, to add a new item, do as follows:
*
* getFinCard().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FinancialCard1 }
*
*
* @return
* The value of the finCard property.
*/
public List getFinCard() {
if (finCard == null) {
finCard = new ArrayList<>();
}
return this.finCard;
}
/**
* Gets the value of the purchsAcctgAcct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the purchsAcctgAcct property.
*
*
* For example, to add a new item, do as follows:
*
* getPurchsAcctgAcct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AccountingAccount1 }
*
*
* @return
* The value of the purchsAcctgAcct property.
*/
public List getPurchsAcctgAcct() {
if (purchsAcctgAcct == null) {
purchsAcctgAcct = new ArrayList<>();
}
return this.purchsAcctgAcct;
}
/**
* Gets the value of the issrFactrgListId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the issrFactrgListId property.
*
*
* For example, to add a new item, do as follows:
*
* getIssrFactrgListId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the issrFactrgListId property.
*/
public List getIssrFactrgListId() {
if (issrFactrgListId == null) {
issrFactrgListId = new ArrayList<>();
}
return this.issrFactrgListId;
}
/**
* Gets the value of the issrFactrgAgrmtId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the issrFactrgAgrmtId property.
*
*
* For example, to add a new item, do as follows:
*
* getIssrFactrgAgrmtId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the issrFactrgAgrmtId property.
*/
public List getIssrFactrgAgrmtId() {
if (issrFactrgAgrmtId == null) {
issrFactrgAgrmtId = new ArrayList<>();
}
return this.issrFactrgAgrmtId;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the duePyblAmt list.
* @see #getDuePyblAmt()
*
*/
public TradeSettlement1 addDuePyblAmt(CurrencyAndAmount duePyblAmt) {
getDuePyblAmt().add(duePyblAmt);
return this;
}
/**
* Adds a new item to the cdtrRef list.
* @see #getCdtrRef()
*
*/
public TradeSettlement1 addCdtrRef(CreditorReferenceInformation2 cdtrRef) {
getCdtrRef().add(cdtrRef);
return this;
}
/**
* Adds a new item to the pmtRef list.
* @see #getPmtRef()
*
*/
public TradeSettlement1 addPmtRef(String pmtRef) {
getPmtRef().add(pmtRef);
return this;
}
/**
* Adds a new item to the pmtMeans list.
* @see #getPmtMeans()
*
*/
public TradeSettlement1 addPmtMeans(PaymentMeans1 pmtMeans) {
getPmtMeans().add(pmtMeans);
return this;
}
/**
* Adds a new item to the tax list.
* @see #getTax()
*
*/
public TradeSettlement1 addTax(SettlementTax1 tax) {
getTax().add(tax);
return this;
}
/**
* Adds a new item to the allwncChrg list.
* @see #getAllwncChrg()
*
*/
public TradeSettlement1 addAllwncChrg(SettlementAllowanceCharge1 allwncChrg) {
getAllwncChrg().add(allwncChrg);
return this;
}
/**
* Adds a new item to the subTtlClctdTax list.
* @see #getSubTtlClctdTax()
*
*/
public TradeSettlement1 addSubTtlClctdTax(SettlementSubTotalCalculatedTax1 subTtlClctdTax) {
getSubTtlClctdTax().add(subTtlClctdTax);
return this;
}
/**
* Adds a new item to the logstcsChrg list.
* @see #getLogstcsChrg()
*
*/
public TradeSettlement1 addLogstcsChrg(ChargesDetails2 logstcsChrg) {
getLogstcsChrg().add(logstcsChrg);
return this;
}
/**
* Adds a new item to the pmtTerms list.
* @see #getPmtTerms()
*
*/
public TradeSettlement1 addPmtTerms(PaymentTerms3 pmtTerms) {
getPmtTerms().add(pmtTerms);
return this;
}
/**
* Adds a new item to the adjstmntAmtAndRsn list.
* @see #getAdjstmntAmtAndRsn()
*
*/
public TradeSettlement1 addAdjstmntAmtAndRsn(DocumentAdjustment2 adjstmntAmtAndRsn) {
getAdjstmntAmtAndRsn().add(adjstmntAmtAndRsn);
return this;
}
/**
* Adds a new item to the finCard list.
* @see #getFinCard()
*
*/
public TradeSettlement1 addFinCard(FinancialCard1 finCard) {
getFinCard().add(finCard);
return this;
}
/**
* Adds a new item to the purchsAcctgAcct list.
* @see #getPurchsAcctgAcct()
*
*/
public TradeSettlement1 addPurchsAcctgAcct(AccountingAccount1 purchsAcctgAcct) {
getPurchsAcctgAcct().add(purchsAcctgAcct);
return this;
}
/**
* Adds a new item to the issrFactrgListId list.
* @see #getIssrFactrgListId()
*
*/
public TradeSettlement1 addIssrFactrgListId(String issrFactrgListId) {
getIssrFactrgListId().add(issrFactrgListId);
return this;
}
/**
* Adds a new item to the issrFactrgAgrmtId list.
* @see #getIssrFactrgAgrmtId()
*
*/
public TradeSettlement1 addIssrFactrgAgrmtId(String issrFactrgAgrmtId) {
getIssrFactrgAgrmtId().add(issrFactrgAgrmtId);
return this;
}
}