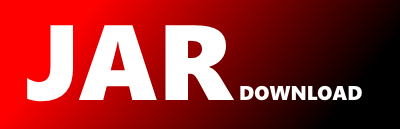
com.prowidesoftware.swift.model.mx.dic.Transaction156 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Fee transaction type.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Transaction156", propOrder = {
"txTp",
"txSubTp",
"cxlInd",
"msgRsn",
"altrnMsgRsn",
"spclPrgrmmQlfctn",
"txId",
"txAmts",
"addtlAmt",
"addtlFee",
"addtlData",
"feeColltnCycl",
"feeColltnRef"
})
public class Transaction156 {
@XmlElement(name = "TxTp", required = true)
protected String txTp;
@XmlElement(name = "TxSubTp")
protected String txSubTp;
@XmlElement(name = "CxlInd")
protected Boolean cxlInd;
@XmlElement(name = "MsgRsn")
protected List msgRsn;
@XmlElement(name = "AltrnMsgRsn")
protected List altrnMsgRsn;
@XmlElement(name = "SpclPrgrmmQlfctn")
protected List spclPrgrmmQlfctn;
@XmlElement(name = "TxId", required = true)
protected TransactionIdentification51 txId;
@XmlElement(name = "TxAmts", required = true)
protected TransactionAmounts2 txAmts;
@XmlElement(name = "AddtlAmt")
protected List addtlAmt;
@XmlElement(name = "AddtlFee")
protected List addtlFee;
@XmlElement(name = "AddtlData")
protected List addtlData;
@XmlElement(name = "FeeColltnCycl")
protected String feeColltnCycl;
@XmlElement(name = "FeeColltnRef")
protected FeeCollectionReference1 feeColltnRef;
/**
* Gets the value of the txTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxTp() {
return txTp;
}
/**
* Sets the value of the txTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction156 setTxTp(String value) {
this.txTp = value;
return this;
}
/**
* Gets the value of the txSubTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxSubTp() {
return txSubTp;
}
/**
* Sets the value of the txSubTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction156 setTxSubTp(String value) {
this.txSubTp = value;
return this;
}
/**
* Gets the value of the cxlInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCxlInd() {
return cxlInd;
}
/**
* Sets the value of the cxlInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public Transaction156 setCxlInd(Boolean value) {
this.cxlInd = value;
return this;
}
/**
* Gets the value of the msgRsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the msgRsn property.
*
*
* For example, to add a new item, do as follows:
*
* getMsgRsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the msgRsn property.
*/
public List getMsgRsn() {
if (msgRsn == null) {
msgRsn = new ArrayList<>();
}
return this.msgRsn;
}
/**
* Gets the value of the altrnMsgRsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the altrnMsgRsn property.
*
*
* For example, to add a new item, do as follows:
*
* getAltrnMsgRsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the altrnMsgRsn property.
*/
public List getAltrnMsgRsn() {
if (altrnMsgRsn == null) {
altrnMsgRsn = new ArrayList<>();
}
return this.altrnMsgRsn;
}
/**
* Gets the value of the spclPrgrmmQlfctn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the spclPrgrmmQlfctn property.
*
*
* For example, to add a new item, do as follows:
*
* getSpclPrgrmmQlfctn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SpecialProgrammeQualification1 }
*
*
* @return
* The value of the spclPrgrmmQlfctn property.
*/
public List getSpclPrgrmmQlfctn() {
if (spclPrgrmmQlfctn == null) {
spclPrgrmmQlfctn = new ArrayList<>();
}
return this.spclPrgrmmQlfctn;
}
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link TransactionIdentification51 }
*
*/
public TransactionIdentification51 getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link TransactionIdentification51 }
*
*/
public Transaction156 setTxId(TransactionIdentification51 value) {
this.txId = value;
return this;
}
/**
* Gets the value of the txAmts property.
*
* @return
* possible object is
* {@link TransactionAmounts2 }
*
*/
public TransactionAmounts2 getTxAmts() {
return txAmts;
}
/**
* Sets the value of the txAmts property.
*
* @param value
* allowed object is
* {@link TransactionAmounts2 }
*
*/
public Transaction156 setTxAmts(TransactionAmounts2 value) {
this.txAmts = value;
return this;
}
/**
* Gets the value of the addtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalAmounts3 }
*
*
* @return
* The value of the addtlAmt property.
*/
public List getAddtlAmt() {
if (addtlAmt == null) {
addtlAmt = new ArrayList<>();
}
return this.addtlAmt;
}
/**
* Gets the value of the addtlFee property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlFee property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlFee().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalFee2 }
*
*
* @return
* The value of the addtlFee property.
*/
public List getAddtlFee() {
if (addtlFee == null) {
addtlFee = new ArrayList<>();
}
return this.addtlFee;
}
/**
* Gets the value of the addtlData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlData property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalData1 }
*
*
* @return
* The value of the addtlData property.
*/
public List getAddtlData() {
if (addtlData == null) {
addtlData = new ArrayList<>();
}
return this.addtlData;
}
/**
* Gets the value of the feeColltnCycl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFeeColltnCycl() {
return feeColltnCycl;
}
/**
* Sets the value of the feeColltnCycl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction156 setFeeColltnCycl(String value) {
this.feeColltnCycl = value;
return this;
}
/**
* Gets the value of the feeColltnRef property.
*
* @return
* possible object is
* {@link FeeCollectionReference1 }
*
*/
public FeeCollectionReference1 getFeeColltnRef() {
return feeColltnRef;
}
/**
* Sets the value of the feeColltnRef property.
*
* @param value
* allowed object is
* {@link FeeCollectionReference1 }
*
*/
public Transaction156 setFeeColltnRef(FeeCollectionReference1 value) {
this.feeColltnRef = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the msgRsn list.
* @see #getMsgRsn()
*
*/
public Transaction156 addMsgRsn(String msgRsn) {
getMsgRsn().add(msgRsn);
return this;
}
/**
* Adds a new item to the altrnMsgRsn list.
* @see #getAltrnMsgRsn()
*
*/
public Transaction156 addAltrnMsgRsn(String altrnMsgRsn) {
getAltrnMsgRsn().add(altrnMsgRsn);
return this;
}
/**
* Adds a new item to the spclPrgrmmQlfctn list.
* @see #getSpclPrgrmmQlfctn()
*
*/
public Transaction156 addSpclPrgrmmQlfctn(SpecialProgrammeQualification1 spclPrgrmmQlfctn) {
getSpclPrgrmmQlfctn().add(spclPrgrmmQlfctn);
return this;
}
/**
* Adds a new item to the addtlAmt list.
* @see #getAddtlAmt()
*
*/
public Transaction156 addAddtlAmt(AdditionalAmounts3 addtlAmt) {
getAddtlAmt().add(addtlAmt);
return this;
}
/**
* Adds a new item to the addtlFee list.
* @see #getAddtlFee()
*
*/
public Transaction156 addAddtlFee(AdditionalFee2 addtlFee) {
getAddtlFee().add(addtlFee);
return this;
}
/**
* Adds a new item to the addtlData list.
* @see #getAddtlData()
*
*/
public Transaction156 addAddtlData(AdditionalData1 addtlData) {
getAddtlData().add(addtlData);
return this;
}
}