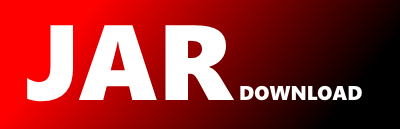
com.prowidesoftware.swift.model.mx.dic.Transaction86 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Contains transaction details.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Transaction86", propOrder = {
"txTp",
"txSubTp",
"txAttr",
"othrTxAttr",
"spclPrgrmmQlfctn",
"txId",
"txAmts",
"addtlAmts",
"addtlFees",
"orgnlAddtlFees",
"acctFr",
"acctTo",
"txDesc",
"addtlData"
})
public class Transaction86 {
@XmlElement(name = "TxTp", required = true)
protected String txTp;
@XmlElement(name = "TxSubTp")
protected String txSubTp;
@XmlElement(name = "TxAttr")
@XmlSchemaType(name = "string")
protected TransactionAttribute1Code txAttr;
@XmlElement(name = "OthrTxAttr")
protected String othrTxAttr;
@XmlElement(name = "SpclPrgrmmQlfctn")
protected List spclPrgrmmQlfctn;
@XmlElement(name = "TxId", required = true)
protected TransactionIdentification8 txId;
@XmlElement(name = "TxAmts", required = true)
protected TransactionAmounts1 txAmts;
@XmlElement(name = "AddtlAmts")
protected List addtlAmts;
@XmlElement(name = "AddtlFees")
protected List addtlFees;
@XmlElement(name = "OrgnlAddtlFees")
protected List orgnlAddtlFees;
@XmlElement(name = "AcctFr")
protected AccountDetails2 acctFr;
@XmlElement(name = "AcctTo")
protected AccountDetails2 acctTo;
@XmlElement(name = "TxDesc")
protected String txDesc;
@XmlElement(name = "AddtlData")
protected List addtlData;
/**
* Gets the value of the txTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxTp() {
return txTp;
}
/**
* Sets the value of the txTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction86 setTxTp(String value) {
this.txTp = value;
return this;
}
/**
* Gets the value of the txSubTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxSubTp() {
return txSubTp;
}
/**
* Sets the value of the txSubTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction86 setTxSubTp(String value) {
this.txSubTp = value;
return this;
}
/**
* Gets the value of the txAttr property.
*
* @return
* possible object is
* {@link TransactionAttribute1Code }
*
*/
public TransactionAttribute1Code getTxAttr() {
return txAttr;
}
/**
* Sets the value of the txAttr property.
*
* @param value
* allowed object is
* {@link TransactionAttribute1Code }
*
*/
public Transaction86 setTxAttr(TransactionAttribute1Code value) {
this.txAttr = value;
return this;
}
/**
* Gets the value of the othrTxAttr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOthrTxAttr() {
return othrTxAttr;
}
/**
* Sets the value of the othrTxAttr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction86 setOthrTxAttr(String value) {
this.othrTxAttr = value;
return this;
}
/**
* Gets the value of the spclPrgrmmQlfctn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the spclPrgrmmQlfctn property.
*
*
* For example, to add a new item, do as follows:
*
* getSpclPrgrmmQlfctn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SpecialProgrammeQualification1 }
*
*
* @return
* The value of the spclPrgrmmQlfctn property.
*/
public List getSpclPrgrmmQlfctn() {
if (spclPrgrmmQlfctn == null) {
spclPrgrmmQlfctn = new ArrayList<>();
}
return this.spclPrgrmmQlfctn;
}
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link TransactionIdentification8 }
*
*/
public TransactionIdentification8 getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link TransactionIdentification8 }
*
*/
public Transaction86 setTxId(TransactionIdentification8 value) {
this.txId = value;
return this;
}
/**
* Gets the value of the txAmts property.
*
* @return
* possible object is
* {@link TransactionAmounts1 }
*
*/
public TransactionAmounts1 getTxAmts() {
return txAmts;
}
/**
* Sets the value of the txAmts property.
*
* @param value
* allowed object is
* {@link TransactionAmounts1 }
*
*/
public Transaction86 setTxAmts(TransactionAmounts1 value) {
this.txAmts = value;
return this;
}
/**
* Gets the value of the addtlAmts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlAmts property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlAmts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalAmounts1 }
*
*
* @return
* The value of the addtlAmts property.
*/
public List getAddtlAmts() {
if (addtlAmts == null) {
addtlAmts = new ArrayList<>();
}
return this.addtlAmts;
}
/**
* Gets the value of the addtlFees property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlFees property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlFees().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalFee1 }
*
*
* @return
* The value of the addtlFees property.
*/
public List getAddtlFees() {
if (addtlFees == null) {
addtlFees = new ArrayList<>();
}
return this.addtlFees;
}
/**
* Gets the value of the orgnlAddtlFees property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the orgnlAddtlFees property.
*
*
* For example, to add a new item, do as follows:
*
* getOrgnlAddtlFees().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalFee1 }
*
*
* @return
* The value of the orgnlAddtlFees property.
*/
public List getOrgnlAddtlFees() {
if (orgnlAddtlFees == null) {
orgnlAddtlFees = new ArrayList<>();
}
return this.orgnlAddtlFees;
}
/**
* Gets the value of the acctFr property.
*
* @return
* possible object is
* {@link AccountDetails2 }
*
*/
public AccountDetails2 getAcctFr() {
return acctFr;
}
/**
* Sets the value of the acctFr property.
*
* @param value
* allowed object is
* {@link AccountDetails2 }
*
*/
public Transaction86 setAcctFr(AccountDetails2 value) {
this.acctFr = value;
return this;
}
/**
* Gets the value of the acctTo property.
*
* @return
* possible object is
* {@link AccountDetails2 }
*
*/
public AccountDetails2 getAcctTo() {
return acctTo;
}
/**
* Sets the value of the acctTo property.
*
* @param value
* allowed object is
* {@link AccountDetails2 }
*
*/
public Transaction86 setAcctTo(AccountDetails2 value) {
this.acctTo = value;
return this;
}
/**
* Gets the value of the txDesc property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxDesc() {
return txDesc;
}
/**
* Sets the value of the txDesc property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Transaction86 setTxDesc(String value) {
this.txDesc = value;
return this;
}
/**
* Gets the value of the addtlData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlData property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalData1 }
*
*
* @return
* The value of the addtlData property.
*/
public List getAddtlData() {
if (addtlData == null) {
addtlData = new ArrayList<>();
}
return this.addtlData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the spclPrgrmmQlfctn list.
* @see #getSpclPrgrmmQlfctn()
*
*/
public Transaction86 addSpclPrgrmmQlfctn(SpecialProgrammeQualification1 spclPrgrmmQlfctn) {
getSpclPrgrmmQlfctn().add(spclPrgrmmQlfctn);
return this;
}
/**
* Adds a new item to the addtlAmts list.
* @see #getAddtlAmts()
*
*/
public Transaction86 addAddtlAmts(AdditionalAmounts1 addtlAmts) {
getAddtlAmts().add(addtlAmts);
return this;
}
/**
* Adds a new item to the addtlFees list.
* @see #getAddtlFees()
*
*/
public Transaction86 addAddtlFees(AdditionalFee1 addtlFees) {
getAddtlFees().add(addtlFees);
return this;
}
/**
* Adds a new item to the orgnlAddtlFees list.
* @see #getOrgnlAddtlFees()
*
*/
public Transaction86 addOrgnlAddtlFees(AdditionalFee1 orgnlAddtlFees) {
getOrgnlAddtlFees().add(orgnlAddtlFees);
return this;
}
/**
* Adds a new item to the addtlData list.
* @see #getAddtlData()
*
*/
public Transaction86 addAddtlData(AdditionalData1 addtlData) {
getAddtlData().add(addtlData);
return this;
}
}