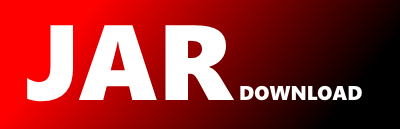
com.prowidesoftware.swift.model.mx.dic.TransactionParties11 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Provides further details on the parties specific to the individual transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TransactionParties11", propOrder = {
"ultmtDbtr",
"dbtr",
"dbtrAcct",
"initgPty",
"dbtrAgt",
"dbtrAgtAcct",
"prvsInstgAgt1",
"prvsInstgAgt1Acct",
"prvsInstgAgt2",
"prvsInstgAgt2Acct",
"prvsInstgAgt3",
"prvsInstgAgt3Acct",
"intrmyAgt1",
"intrmyAgt1Acct",
"intrmyAgt2",
"intrmyAgt2Acct",
"intrmyAgt3",
"intrmyAgt3Acct",
"cdtrAgt",
"cdtrAgtAcct",
"cdtr",
"cdtrAcct",
"ultmtCdtr"
})
public class TransactionParties11 {
@XmlElement(name = "UltmtDbtr")
protected Party50Choice ultmtDbtr;
@XmlElement(name = "Dbtr", required = true)
protected Party50Choice dbtr;
@XmlElement(name = "DbtrAcct")
protected CashAccount40 dbtrAcct;
@XmlElement(name = "InitgPty")
protected Party50Choice initgPty;
@XmlElement(name = "DbtrAgt")
protected BranchAndFinancialInstitutionIdentification8 dbtrAgt;
@XmlElement(name = "DbtrAgtAcct")
protected CashAccount40 dbtrAgtAcct;
@XmlElement(name = "PrvsInstgAgt1")
protected BranchAndFinancialInstitutionIdentification8 prvsInstgAgt1;
@XmlElement(name = "PrvsInstgAgt1Acct")
protected CashAccount40 prvsInstgAgt1Acct;
@XmlElement(name = "PrvsInstgAgt2")
protected BranchAndFinancialInstitutionIdentification8 prvsInstgAgt2;
@XmlElement(name = "PrvsInstgAgt2Acct")
protected CashAccount40 prvsInstgAgt2Acct;
@XmlElement(name = "PrvsInstgAgt3")
protected BranchAndFinancialInstitutionIdentification8 prvsInstgAgt3;
@XmlElement(name = "PrvsInstgAgt3Acct")
protected CashAccount40 prvsInstgAgt3Acct;
@XmlElement(name = "IntrmyAgt1")
protected BranchAndFinancialInstitutionIdentification8 intrmyAgt1;
@XmlElement(name = "IntrmyAgt1Acct")
protected CashAccount40 intrmyAgt1Acct;
@XmlElement(name = "IntrmyAgt2")
protected BranchAndFinancialInstitutionIdentification8 intrmyAgt2;
@XmlElement(name = "IntrmyAgt2Acct")
protected CashAccount40 intrmyAgt2Acct;
@XmlElement(name = "IntrmyAgt3")
protected BranchAndFinancialInstitutionIdentification8 intrmyAgt3;
@XmlElement(name = "IntrmyAgt3Acct")
protected CashAccount40 intrmyAgt3Acct;
@XmlElement(name = "CdtrAgt")
protected BranchAndFinancialInstitutionIdentification8 cdtrAgt;
@XmlElement(name = "CdtrAgtAcct")
protected CashAccount40 cdtrAgtAcct;
@XmlElement(name = "Cdtr", required = true)
protected Party50Choice cdtr;
@XmlElement(name = "CdtrAcct")
protected CashAccount40 cdtrAcct;
@XmlElement(name = "UltmtCdtr")
protected Party50Choice ultmtCdtr;
/**
* Gets the value of the ultmtDbtr property.
*
* @return
* possible object is
* {@link Party50Choice }
*
*/
public Party50Choice getUltmtDbtr() {
return ultmtDbtr;
}
/**
* Sets the value of the ultmtDbtr property.
*
* @param value
* allowed object is
* {@link Party50Choice }
*
*/
public TransactionParties11 setUltmtDbtr(Party50Choice value) {
this.ultmtDbtr = value;
return this;
}
/**
* Gets the value of the dbtr property.
*
* @return
* possible object is
* {@link Party50Choice }
*
*/
public Party50Choice getDbtr() {
return dbtr;
}
/**
* Sets the value of the dbtr property.
*
* @param value
* allowed object is
* {@link Party50Choice }
*
*/
public TransactionParties11 setDbtr(Party50Choice value) {
this.dbtr = value;
return this;
}
/**
* Gets the value of the dbtrAcct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getDbtrAcct() {
return dbtrAcct;
}
/**
* Sets the value of the dbtrAcct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setDbtrAcct(CashAccount40 value) {
this.dbtrAcct = value;
return this;
}
/**
* Gets the value of the initgPty property.
*
* @return
* possible object is
* {@link Party50Choice }
*
*/
public Party50Choice getInitgPty() {
return initgPty;
}
/**
* Sets the value of the initgPty property.
*
* @param value
* allowed object is
* {@link Party50Choice }
*
*/
public TransactionParties11 setInitgPty(Party50Choice value) {
this.initgPty = value;
return this;
}
/**
* Gets the value of the dbtrAgt property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getDbtrAgt() {
return dbtrAgt;
}
/**
* Sets the value of the dbtrAgt property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setDbtrAgt(BranchAndFinancialInstitutionIdentification8 value) {
this.dbtrAgt = value;
return this;
}
/**
* Gets the value of the dbtrAgtAcct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getDbtrAgtAcct() {
return dbtrAgtAcct;
}
/**
* Sets the value of the dbtrAgtAcct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setDbtrAgtAcct(CashAccount40 value) {
this.dbtrAgtAcct = value;
return this;
}
/**
* Gets the value of the prvsInstgAgt1 property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getPrvsInstgAgt1() {
return prvsInstgAgt1;
}
/**
* Sets the value of the prvsInstgAgt1 property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setPrvsInstgAgt1(BranchAndFinancialInstitutionIdentification8 value) {
this.prvsInstgAgt1 = value;
return this;
}
/**
* Gets the value of the prvsInstgAgt1Acct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getPrvsInstgAgt1Acct() {
return prvsInstgAgt1Acct;
}
/**
* Sets the value of the prvsInstgAgt1Acct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setPrvsInstgAgt1Acct(CashAccount40 value) {
this.prvsInstgAgt1Acct = value;
return this;
}
/**
* Gets the value of the prvsInstgAgt2 property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getPrvsInstgAgt2() {
return prvsInstgAgt2;
}
/**
* Sets the value of the prvsInstgAgt2 property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setPrvsInstgAgt2(BranchAndFinancialInstitutionIdentification8 value) {
this.prvsInstgAgt2 = value;
return this;
}
/**
* Gets the value of the prvsInstgAgt2Acct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getPrvsInstgAgt2Acct() {
return prvsInstgAgt2Acct;
}
/**
* Sets the value of the prvsInstgAgt2Acct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setPrvsInstgAgt2Acct(CashAccount40 value) {
this.prvsInstgAgt2Acct = value;
return this;
}
/**
* Gets the value of the prvsInstgAgt3 property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getPrvsInstgAgt3() {
return prvsInstgAgt3;
}
/**
* Sets the value of the prvsInstgAgt3 property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setPrvsInstgAgt3(BranchAndFinancialInstitutionIdentification8 value) {
this.prvsInstgAgt3 = value;
return this;
}
/**
* Gets the value of the prvsInstgAgt3Acct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getPrvsInstgAgt3Acct() {
return prvsInstgAgt3Acct;
}
/**
* Sets the value of the prvsInstgAgt3Acct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setPrvsInstgAgt3Acct(CashAccount40 value) {
this.prvsInstgAgt3Acct = value;
return this;
}
/**
* Gets the value of the intrmyAgt1 property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getIntrmyAgt1() {
return intrmyAgt1;
}
/**
* Sets the value of the intrmyAgt1 property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setIntrmyAgt1(BranchAndFinancialInstitutionIdentification8 value) {
this.intrmyAgt1 = value;
return this;
}
/**
* Gets the value of the intrmyAgt1Acct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getIntrmyAgt1Acct() {
return intrmyAgt1Acct;
}
/**
* Sets the value of the intrmyAgt1Acct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setIntrmyAgt1Acct(CashAccount40 value) {
this.intrmyAgt1Acct = value;
return this;
}
/**
* Gets the value of the intrmyAgt2 property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getIntrmyAgt2() {
return intrmyAgt2;
}
/**
* Sets the value of the intrmyAgt2 property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setIntrmyAgt2(BranchAndFinancialInstitutionIdentification8 value) {
this.intrmyAgt2 = value;
return this;
}
/**
* Gets the value of the intrmyAgt2Acct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getIntrmyAgt2Acct() {
return intrmyAgt2Acct;
}
/**
* Sets the value of the intrmyAgt2Acct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setIntrmyAgt2Acct(CashAccount40 value) {
this.intrmyAgt2Acct = value;
return this;
}
/**
* Gets the value of the intrmyAgt3 property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getIntrmyAgt3() {
return intrmyAgt3;
}
/**
* Sets the value of the intrmyAgt3 property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setIntrmyAgt3(BranchAndFinancialInstitutionIdentification8 value) {
this.intrmyAgt3 = value;
return this;
}
/**
* Gets the value of the intrmyAgt3Acct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getIntrmyAgt3Acct() {
return intrmyAgt3Acct;
}
/**
* Sets the value of the intrmyAgt3Acct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setIntrmyAgt3Acct(CashAccount40 value) {
this.intrmyAgt3Acct = value;
return this;
}
/**
* Gets the value of the cdtrAgt property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getCdtrAgt() {
return cdtrAgt;
}
/**
* Sets the value of the cdtrAgt property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public TransactionParties11 setCdtrAgt(BranchAndFinancialInstitutionIdentification8 value) {
this.cdtrAgt = value;
return this;
}
/**
* Gets the value of the cdtrAgtAcct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getCdtrAgtAcct() {
return cdtrAgtAcct;
}
/**
* Sets the value of the cdtrAgtAcct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setCdtrAgtAcct(CashAccount40 value) {
this.cdtrAgtAcct = value;
return this;
}
/**
* Gets the value of the cdtr property.
*
* @return
* possible object is
* {@link Party50Choice }
*
*/
public Party50Choice getCdtr() {
return cdtr;
}
/**
* Sets the value of the cdtr property.
*
* @param value
* allowed object is
* {@link Party50Choice }
*
*/
public TransactionParties11 setCdtr(Party50Choice value) {
this.cdtr = value;
return this;
}
/**
* Gets the value of the cdtrAcct property.
*
* @return
* possible object is
* {@link CashAccount40 }
*
*/
public CashAccount40 getCdtrAcct() {
return cdtrAcct;
}
/**
* Sets the value of the cdtrAcct property.
*
* @param value
* allowed object is
* {@link CashAccount40 }
*
*/
public TransactionParties11 setCdtrAcct(CashAccount40 value) {
this.cdtrAcct = value;
return this;
}
/**
* Gets the value of the ultmtCdtr property.
*
* @return
* possible object is
* {@link Party50Choice }
*
*/
public Party50Choice getUltmtCdtr() {
return ultmtCdtr;
}
/**
* Sets the value of the ultmtCdtr property.
*
* @param value
* allowed object is
* {@link Party50Choice }
*
*/
public TransactionParties11 setUltmtCdtr(Party50Choice value) {
this.ultmtCdtr = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy