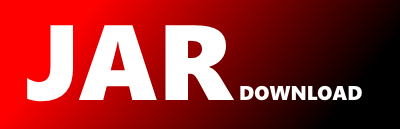
com.prowidesoftware.swift.model.mx.dic.TransferInConfirmationV09 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The TransferInConfirmation message is sent by an executing party, for example, a transfer agent, to the instructing party, for example, an investment manager or its authorised representative, to confirm the receipt of a financial instrument, free of payment, on a given date, from a specified party.
* This message may also be used to confirm the receipt of a financial instrument, free of payment, from another of the instructing parties own accounts or from a third party.
* This message may also be used as an advice, that is, the message is used to provide account information.
* Usage
* The TransferInConfirmation message is used to confirm receipt of a financial instrument, either from another account owned by the instructing party or from a third party. The reference of the transfer confirmation is identified in TransferConfirmationReference.
* The reference of the original transfer instruction is specified in TransferReference. The message identification of the TransferInInstruction message in which the transfer instruction was conveyed may also be quoted in RelatedReference.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TransferInConfirmationV09", propOrder = {
"msgId",
"poolRef",
"prvsRef",
"rltdRef",
"fctn",
"mstrRef",
"trfDtls",
"acctDtls",
"sttlmDtls",
"mktPrctcVrsn",
"cpyDtls",
"xtnsn"
})
public class TransferInConfirmationV09 {
@XmlElement(name = "MsgId", required = true)
protected MessageIdentification1 msgId;
@XmlElement(name = "PoolRef")
protected AdditionalReference11 poolRef;
@XmlElement(name = "PrvsRef")
protected AdditionalReference10 prvsRef;
@XmlElement(name = "RltdRef")
protected AdditionalReference10 rltdRef;
@XmlElement(name = "Fctn")
@XmlSchemaType(name = "string")
protected TransferInFunction2Code fctn;
@XmlElement(name = "MstrRef")
protected String mstrRef;
@XmlElement(name = "TrfDtls", required = true)
protected List trfDtls;
@XmlElement(name = "AcctDtls", required = true)
protected InvestmentAccount71 acctDtls;
@XmlElement(name = "SttlmDtls")
protected DeliverInformation20 sttlmDtls;
@XmlElement(name = "MktPrctcVrsn")
protected MarketPracticeVersion1 mktPrctcVrsn;
@XmlElement(name = "CpyDtls")
protected CopyInformation5 cpyDtls;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgId property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getMsgId() {
return msgId;
}
/**
* Sets the value of the msgId property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public TransferInConfirmationV09 setMsgId(MessageIdentification1 value) {
this.msgId = value;
return this;
}
/**
* Gets the value of the poolRef property.
*
* @return
* possible object is
* {@link AdditionalReference11 }
*
*/
public AdditionalReference11 getPoolRef() {
return poolRef;
}
/**
* Sets the value of the poolRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference11 }
*
*/
public TransferInConfirmationV09 setPoolRef(AdditionalReference11 value) {
this.poolRef = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
* @return
* possible object is
* {@link AdditionalReference10 }
*
*/
public AdditionalReference10 getPrvsRef() {
return prvsRef;
}
/**
* Sets the value of the prvsRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference10 }
*
*/
public TransferInConfirmationV09 setPrvsRef(AdditionalReference10 value) {
this.prvsRef = value;
return this;
}
/**
* Gets the value of the rltdRef property.
*
* @return
* possible object is
* {@link AdditionalReference10 }
*
*/
public AdditionalReference10 getRltdRef() {
return rltdRef;
}
/**
* Sets the value of the rltdRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference10 }
*
*/
public TransferInConfirmationV09 setRltdRef(AdditionalReference10 value) {
this.rltdRef = value;
return this;
}
/**
* Gets the value of the fctn property.
*
* @return
* possible object is
* {@link TransferInFunction2Code }
*
*/
public TransferInFunction2Code getFctn() {
return fctn;
}
/**
* Sets the value of the fctn property.
*
* @param value
* allowed object is
* {@link TransferInFunction2Code }
*
*/
public TransferInConfirmationV09 setFctn(TransferInFunction2Code value) {
this.fctn = value;
return this;
}
/**
* Gets the value of the mstrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMstrRef() {
return mstrRef;
}
/**
* Sets the value of the mstrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public TransferInConfirmationV09 setMstrRef(String value) {
this.mstrRef = value;
return this;
}
/**
* Gets the value of the trfDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the trfDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getTrfDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Transfer37 }
*
*
* @return
* The value of the trfDtls property.
*/
public List getTrfDtls() {
if (trfDtls == null) {
trfDtls = new ArrayList<>();
}
return this.trfDtls;
}
/**
* Gets the value of the acctDtls property.
*
* @return
* possible object is
* {@link InvestmentAccount71 }
*
*/
public InvestmentAccount71 getAcctDtls() {
return acctDtls;
}
/**
* Sets the value of the acctDtls property.
*
* @param value
* allowed object is
* {@link InvestmentAccount71 }
*
*/
public TransferInConfirmationV09 setAcctDtls(InvestmentAccount71 value) {
this.acctDtls = value;
return this;
}
/**
* Gets the value of the sttlmDtls property.
*
* @return
* possible object is
* {@link DeliverInformation20 }
*
*/
public DeliverInformation20 getSttlmDtls() {
return sttlmDtls;
}
/**
* Sets the value of the sttlmDtls property.
*
* @param value
* allowed object is
* {@link DeliverInformation20 }
*
*/
public TransferInConfirmationV09 setSttlmDtls(DeliverInformation20 value) {
this.sttlmDtls = value;
return this;
}
/**
* Gets the value of the mktPrctcVrsn property.
*
* @return
* possible object is
* {@link MarketPracticeVersion1 }
*
*/
public MarketPracticeVersion1 getMktPrctcVrsn() {
return mktPrctcVrsn;
}
/**
* Sets the value of the mktPrctcVrsn property.
*
* @param value
* allowed object is
* {@link MarketPracticeVersion1 }
*
*/
public TransferInConfirmationV09 setMktPrctcVrsn(MarketPracticeVersion1 value) {
this.mktPrctcVrsn = value;
return this;
}
/**
* Gets the value of the cpyDtls property.
*
* @return
* possible object is
* {@link CopyInformation5 }
*
*/
public CopyInformation5 getCpyDtls() {
return cpyDtls;
}
/**
* Sets the value of the cpyDtls property.
*
* @param value
* allowed object is
* {@link CopyInformation5 }
*
*/
public TransferInConfirmationV09 setCpyDtls(CopyInformation5 value) {
this.cpyDtls = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the trfDtls list.
* @see #getTrfDtls()
*
*/
public TransferInConfirmationV09 addTrfDtls(Transfer37 trfDtls) {
getTrfDtls().add(trfDtls);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public TransferInConfirmationV09 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}