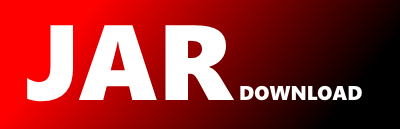
com.prowidesoftware.swift.model.mx.dic.TransferOutConfirmationV05 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An executing party, for example, a transfer agent, sends the TransferOutConfirmation message to the instructing party, for example, an investment manager or its authorised representative, to confirm the delivery of a financial instrument, free of payment, on a given date, to a specified party.
* This message may also be used to confirm the delivery of a financial instrument, free of payment, to another of the instructing parties own accounts or to a third party.
* Usage
* The TransferOutConfirmation message is used to confirm the withdrawal of a financial instrument from the owner's account and its delivery to another own account, or to a third party, has taken place.
* The reference of the transfer confirmation is identified in TransferConfirmationReference. The reference of the original transfer instruction is specified in TransferReference. The message identification of the TransferOutInstruction message in which the transfer instruction was conveyed may also be quoted in RelatedReference.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TransferOutConfirmationV05", propOrder = {
"msgId",
"poolRef",
"prvsRef",
"rltdRef",
"mstrRef",
"trfDtls",
"acctDtls",
"sttlmDtls",
"mktPrctcVrsn",
"cpyDtls",
"xtnsn"
})
public class TransferOutConfirmationV05 {
@XmlElement(name = "MsgId", required = true)
protected MessageIdentification1 msgId;
@XmlElement(name = "PoolRef")
protected AdditionalReference2 poolRef;
@XmlElement(name = "PrvsRef")
protected AdditionalReference2 prvsRef;
@XmlElement(name = "RltdRef")
protected AdditionalReference2 rltdRef;
@XmlElement(name = "MstrRef")
protected String mstrRef;
@XmlElement(name = "TrfDtls", required = true)
protected List trfDtls;
@XmlElement(name = "AcctDtls", required = true)
protected InvestmentAccount40 acctDtls;
@XmlElement(name = "SttlmDtls")
protected ReceiveInformation12 sttlmDtls;
@XmlElement(name = "MktPrctcVrsn")
protected MarketPracticeVersion1 mktPrctcVrsn;
@XmlElement(name = "CpyDtls")
protected CopyInformation2 cpyDtls;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgId property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getMsgId() {
return msgId;
}
/**
* Sets the value of the msgId property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public TransferOutConfirmationV05 setMsgId(MessageIdentification1 value) {
this.msgId = value;
return this;
}
/**
* Gets the value of the poolRef property.
*
* @return
* possible object is
* {@link AdditionalReference2 }
*
*/
public AdditionalReference2 getPoolRef() {
return poolRef;
}
/**
* Sets the value of the poolRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference2 }
*
*/
public TransferOutConfirmationV05 setPoolRef(AdditionalReference2 value) {
this.poolRef = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
* @return
* possible object is
* {@link AdditionalReference2 }
*
*/
public AdditionalReference2 getPrvsRef() {
return prvsRef;
}
/**
* Sets the value of the prvsRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference2 }
*
*/
public TransferOutConfirmationV05 setPrvsRef(AdditionalReference2 value) {
this.prvsRef = value;
return this;
}
/**
* Gets the value of the rltdRef property.
*
* @return
* possible object is
* {@link AdditionalReference2 }
*
*/
public AdditionalReference2 getRltdRef() {
return rltdRef;
}
/**
* Sets the value of the rltdRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference2 }
*
*/
public TransferOutConfirmationV05 setRltdRef(AdditionalReference2 value) {
this.rltdRef = value;
return this;
}
/**
* Gets the value of the mstrRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMstrRef() {
return mstrRef;
}
/**
* Sets the value of the mstrRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public TransferOutConfirmationV05 setMstrRef(String value) {
this.mstrRef = value;
return this;
}
/**
* Gets the value of the trfDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the trfDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getTrfDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Transfer28 }
*
*
* @return
* The value of the trfDtls property.
*/
public List getTrfDtls() {
if (trfDtls == null) {
trfDtls = new ArrayList<>();
}
return this.trfDtls;
}
/**
* Gets the value of the acctDtls property.
*
* @return
* possible object is
* {@link InvestmentAccount40 }
*
*/
public InvestmentAccount40 getAcctDtls() {
return acctDtls;
}
/**
* Sets the value of the acctDtls property.
*
* @param value
* allowed object is
* {@link InvestmentAccount40 }
*
*/
public TransferOutConfirmationV05 setAcctDtls(InvestmentAccount40 value) {
this.acctDtls = value;
return this;
}
/**
* Gets the value of the sttlmDtls property.
*
* @return
* possible object is
* {@link ReceiveInformation12 }
*
*/
public ReceiveInformation12 getSttlmDtls() {
return sttlmDtls;
}
/**
* Sets the value of the sttlmDtls property.
*
* @param value
* allowed object is
* {@link ReceiveInformation12 }
*
*/
public TransferOutConfirmationV05 setSttlmDtls(ReceiveInformation12 value) {
this.sttlmDtls = value;
return this;
}
/**
* Gets the value of the mktPrctcVrsn property.
*
* @return
* possible object is
* {@link MarketPracticeVersion1 }
*
*/
public MarketPracticeVersion1 getMktPrctcVrsn() {
return mktPrctcVrsn;
}
/**
* Sets the value of the mktPrctcVrsn property.
*
* @param value
* allowed object is
* {@link MarketPracticeVersion1 }
*
*/
public TransferOutConfirmationV05 setMktPrctcVrsn(MarketPracticeVersion1 value) {
this.mktPrctcVrsn = value;
return this;
}
/**
* Gets the value of the cpyDtls property.
*
* @return
* possible object is
* {@link CopyInformation2 }
*
*/
public CopyInformation2 getCpyDtls() {
return cpyDtls;
}
/**
* Sets the value of the cpyDtls property.
*
* @param value
* allowed object is
* {@link CopyInformation2 }
*
*/
public TransferOutConfirmationV05 setCpyDtls(CopyInformation2 value) {
this.cpyDtls = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the trfDtls list.
* @see #getTrfDtls()
*
*/
public TransferOutConfirmationV05 addTrfDtls(Transfer28 trfDtls) {
getTrfDtls().add(trfDtls);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public TransferOutConfirmationV05 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}