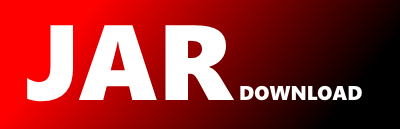
com.prowidesoftware.swift.model.mx.dic.VehicleRentalInvoice2 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.time.OffsetTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import com.prowidesoftware.swift.model.mx.adapters.IsoTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Invoice related to a vehicle rental service.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "VehicleRentalInvoice2", propOrder = {
"noShowInd",
"adjstdInd",
"rtrLctn",
"chckOutDt",
"chckOutTm",
"chckInDt",
"chckInTm",
"drtn",
"vhclClssInvcd",
"vhclClssPrvdd",
"trvlDstnc",
"rntlChrg",
"summryCmmdtyId",
"insrncInd",
"addtlAmt",
"tax"
})
public class VehicleRentalInvoice2 {
@XmlElement(name = "NoShowInd")
protected Boolean noShowInd;
@XmlElement(name = "AdjstdInd")
protected Boolean adjstdInd;
@XmlElement(name = "RtrLctn")
protected Address2 rtrLctn;
@XmlElement(name = "ChckOutDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate chckOutDt;
@XmlElement(name = "ChckOutTm", type = String.class)
@XmlJavaTypeAdapter(IsoTimeAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime chckOutTm;
@XmlElement(name = "ChckInDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate chckInDt;
@XmlElement(name = "ChckInTm", type = String.class)
@XmlJavaTypeAdapter(IsoTimeAdapter.class)
@XmlSchemaType(name = "time")
protected OffsetTime chckInTm;
@XmlElement(name = "Drtn")
protected String drtn;
@XmlElement(name = "VhclClssInvcd")
protected Vehicle4 vhclClssInvcd;
@XmlElement(name = "VhclClssPrvdd")
protected Vehicle4 vhclClssPrvdd;
@XmlElement(name = "TrvlDstnc")
protected Distance1 trvlDstnc;
@XmlElement(name = "RntlChrg")
protected List rntlChrg;
@XmlElement(name = "SummryCmmdtyId")
protected String summryCmmdtyId;
@XmlElement(name = "InsrncInd")
protected Boolean insrncInd;
@XmlElement(name = "AddtlAmt")
protected List addtlAmt;
@XmlElement(name = "Tax")
protected List tax;
/**
* Gets the value of the noShowInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isNoShowInd() {
return noShowInd;
}
/**
* Sets the value of the noShowInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public VehicleRentalInvoice2 setNoShowInd(Boolean value) {
this.noShowInd = value;
return this;
}
/**
* Gets the value of the adjstdInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAdjstdInd() {
return adjstdInd;
}
/**
* Sets the value of the adjstdInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public VehicleRentalInvoice2 setAdjstdInd(Boolean value) {
this.adjstdInd = value;
return this;
}
/**
* Gets the value of the rtrLctn property.
*
* @return
* possible object is
* {@link Address2 }
*
*/
public Address2 getRtrLctn() {
return rtrLctn;
}
/**
* Sets the value of the rtrLctn property.
*
* @param value
* allowed object is
* {@link Address2 }
*
*/
public VehicleRentalInvoice2 setRtrLctn(Address2 value) {
this.rtrLctn = value;
return this;
}
/**
* Gets the value of the chckOutDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getChckOutDt() {
return chckOutDt;
}
/**
* Sets the value of the chckOutDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public VehicleRentalInvoice2 setChckOutDt(LocalDate value) {
this.chckOutDt = value;
return this;
}
/**
* Gets the value of the chckOutTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getChckOutTm() {
return chckOutTm;
}
/**
* Sets the value of the chckOutTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public VehicleRentalInvoice2 setChckOutTm(OffsetTime value) {
this.chckOutTm = value;
return this;
}
/**
* Gets the value of the chckInDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getChckInDt() {
return chckInDt;
}
/**
* Sets the value of the chckInDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public VehicleRentalInvoice2 setChckInDt(LocalDate value) {
this.chckInDt = value;
return this;
}
/**
* Gets the value of the chckInTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetTime getChckInTm() {
return chckInTm;
}
/**
* Sets the value of the chckInTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public VehicleRentalInvoice2 setChckInTm(OffsetTime value) {
this.chckInTm = value;
return this;
}
/**
* Gets the value of the drtn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDrtn() {
return drtn;
}
/**
* Sets the value of the drtn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public VehicleRentalInvoice2 setDrtn(String value) {
this.drtn = value;
return this;
}
/**
* Gets the value of the vhclClssInvcd property.
*
* @return
* possible object is
* {@link Vehicle4 }
*
*/
public Vehicle4 getVhclClssInvcd() {
return vhclClssInvcd;
}
/**
* Sets the value of the vhclClssInvcd property.
*
* @param value
* allowed object is
* {@link Vehicle4 }
*
*/
public VehicleRentalInvoice2 setVhclClssInvcd(Vehicle4 value) {
this.vhclClssInvcd = value;
return this;
}
/**
* Gets the value of the vhclClssPrvdd property.
*
* @return
* possible object is
* {@link Vehicle4 }
*
*/
public Vehicle4 getVhclClssPrvdd() {
return vhclClssPrvdd;
}
/**
* Sets the value of the vhclClssPrvdd property.
*
* @param value
* allowed object is
* {@link Vehicle4 }
*
*/
public VehicleRentalInvoice2 setVhclClssPrvdd(Vehicle4 value) {
this.vhclClssPrvdd = value;
return this;
}
/**
* Gets the value of the trvlDstnc property.
*
* @return
* possible object is
* {@link Distance1 }
*
*/
public Distance1 getTrvlDstnc() {
return trvlDstnc;
}
/**
* Sets the value of the trvlDstnc property.
*
* @param value
* allowed object is
* {@link Distance1 }
*
*/
public VehicleRentalInvoice2 setTrvlDstnc(Distance1 value) {
this.trvlDstnc = value;
return this;
}
/**
* Gets the value of the rntlChrg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rntlChrg property.
*
*
* For example, to add a new item, do as follows:
*
* getRntlChrg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RentalRate1 }
*
*
* @return
* The value of the rntlChrg property.
*/
public List getRntlChrg() {
if (rntlChrg == null) {
rntlChrg = new ArrayList<>();
}
return this.rntlChrg;
}
/**
* Gets the value of the summryCmmdtyId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSummryCmmdtyId() {
return summryCmmdtyId;
}
/**
* Sets the value of the summryCmmdtyId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public VehicleRentalInvoice2 setSummryCmmdtyId(String value) {
this.summryCmmdtyId = value;
return this;
}
/**
* Gets the value of the insrncInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isInsrncInd() {
return insrncInd;
}
/**
* Sets the value of the insrncInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public VehicleRentalInvoice2 setInsrncInd(Boolean value) {
this.insrncInd = value;
return this;
}
/**
* Gets the value of the addtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Amount18 }
*
*
* @return
* The value of the addtlAmt property.
*/
public List getAddtlAmt() {
if (addtlAmt == null) {
addtlAmt = new ArrayList<>();
}
return this.addtlAmt;
}
/**
* Gets the value of the tax property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tax property.
*
*
* For example, to add a new item, do as follows:
*
* getTax().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Tax39 }
*
*
* @return
* The value of the tax property.
*/
public List getTax() {
if (tax == null) {
tax = new ArrayList<>();
}
return this.tax;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the rntlChrg list.
* @see #getRntlChrg()
*
*/
public VehicleRentalInvoice2 addRntlChrg(RentalRate1 rntlChrg) {
getRntlChrg().add(rntlChrg);
return this;
}
/**
* Adds a new item to the addtlAmt list.
* @see #getAddtlAmt()
*
*/
public VehicleRentalInvoice2 addAddtlAmt(Amount18 addtlAmt) {
getAddtlAmt().add(addtlAmt);
return this;
}
/**
* Adds a new item to the tax list.
* @see #getTax()
*
*/
public VehicleRentalInvoice2 addTax(Tax39 tax) {
getTax().add(tax);
return this;
}
}