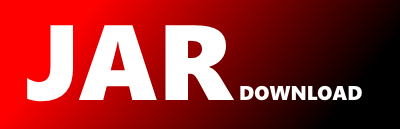
com.prowidesoftware.swift.model.mx.dic.Vote4 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Decision of the voting party for one resolution. Several types of decisions can be indicated to allow for split vote specification.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Vote4", propOrder = {
"issrLabl",
"_for",
"agnst",
"abstn",
"wthhld",
"wthMgmt",
"agnstMgmt",
"dscrtnry",
"noActn"
})
public class Vote4 {
@XmlElement(name = "IssrLabl", required = true)
protected String issrLabl;
@XmlElement(name = "For")
protected BigDecimal _for;
@XmlElement(name = "Agnst")
protected BigDecimal agnst;
@XmlElement(name = "Abstn")
protected BigDecimal abstn;
@XmlElement(name = "Wthhld")
protected BigDecimal wthhld;
@XmlElement(name = "WthMgmt")
protected BigDecimal wthMgmt;
@XmlElement(name = "AgnstMgmt")
protected BigDecimal agnstMgmt;
@XmlElement(name = "Dscrtnry")
protected BigDecimal dscrtnry;
@XmlElement(name = "NoActn")
protected BigDecimal noActn;
/**
* Gets the value of the issrLabl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIssrLabl() {
return issrLabl;
}
/**
* Sets the value of the issrLabl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Vote4 setIssrLabl(String value) {
this.issrLabl = value;
return this;
}
/**
* Gets the value of the for property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getFor() {
return _for;
}
/**
* Sets the value of the for property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setFor(BigDecimal value) {
this._for = value;
return this;
}
/**
* Gets the value of the agnst property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAgnst() {
return agnst;
}
/**
* Sets the value of the agnst property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setAgnst(BigDecimal value) {
this.agnst = value;
return this;
}
/**
* Gets the value of the abstn property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAbstn() {
return abstn;
}
/**
* Sets the value of the abstn property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setAbstn(BigDecimal value) {
this.abstn = value;
return this;
}
/**
* Gets the value of the wthhld property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getWthhld() {
return wthhld;
}
/**
* Sets the value of the wthhld property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setWthhld(BigDecimal value) {
this.wthhld = value;
return this;
}
/**
* Gets the value of the wthMgmt property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getWthMgmt() {
return wthMgmt;
}
/**
* Sets the value of the wthMgmt property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setWthMgmt(BigDecimal value) {
this.wthMgmt = value;
return this;
}
/**
* Gets the value of the agnstMgmt property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAgnstMgmt() {
return agnstMgmt;
}
/**
* Sets the value of the agnstMgmt property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setAgnstMgmt(BigDecimal value) {
this.agnstMgmt = value;
return this;
}
/**
* Gets the value of the dscrtnry property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getDscrtnry() {
return dscrtnry;
}
/**
* Sets the value of the dscrtnry property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setDscrtnry(BigDecimal value) {
this.dscrtnry = value;
return this;
}
/**
* Gets the value of the noActn property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getNoActn() {
return noActn;
}
/**
* Sets the value of the noActn property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Vote4 setNoActn(BigDecimal value) {
this.noActn = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy