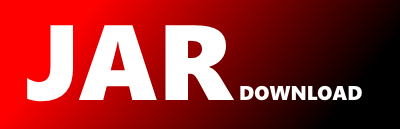
com.prowidesoftware.swift.utils.IMessageVisitor Maven / Gradle / Ivy
Show all versions of pw-swift-core Show documentation
/*
* Copyright 2006-2023 Prowide
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.prowidesoftware.swift.utils;
import com.prowidesoftware.swift.model.*;
/**
* Interface to be implemented by classes that will 'visit' a swift message.
*
* There method call sequence is as follows:
*
*
* startMessage
* startBlock1 -> value -> endBlock1
(if block 1 exists)
* startBlock2 -> value -> endBlock2
(if block 2 exists)
* startBlock3 -> tag (for every tag) -> endBlock3
(if block 3 exists)
* startBlock4 -> tag (for every tag) -> endBlock4
(if block 4 exists)
* startBlock5 -> tag (for every tag) -> endBlock5
(if block 5 exists)
* startBlockUser -> tag (for every tag) -> endBlockUser
(for every user defined block and every tag of that block)
* endMessage
*
*
* Notice that the tag
and value
methods are overloaded for every type of SwiftBlock
* derived class.
*
*
NOTE: this API has changed since 4.0 with SwiftBlocks 1-5 in each start/end
* method pairs.
*/
public interface IMessageVisitor {
/**
* @param b block to visit
*/
void startBlock1(SwiftBlock1 b);
/**
* @param b block to visit
*/
void startBlock2(SwiftBlock2 b);
/**
* @param b block to visit
*/
void startBlock3(SwiftBlock3 b);
/**
* @param b block to visit
*/
void startBlock4(SwiftBlock4 b);
/**
* @param b block to visit
*/
void startBlock5(SwiftBlock5 b);
/**
* @param b block to visit
*/
void startBlockUser(SwiftBlockUser b);
/**
* @param b block to visit
*/
void endBlock1(SwiftBlock1 b);
/**
* @param b block to visit
*/
void endBlock2(SwiftBlock2 b);
/**
* @param b block to visit
*/
void endBlock3(SwiftBlock3 b);
/**
* @param b block to visit
*/
void endBlock4(SwiftBlock4 b);
/**
* @param b block to visit
*/
void endBlock5(SwiftBlock5 b);
/**
* @param b block to visit
*/
void endBlockUser(SwiftBlockUser b);
void tag(SwiftBlock3 b, Tag t);
void tag(SwiftBlock4 b, Tag t);
void tag(SwiftBlock5 b, Tag t);
void tag(SwiftBlockUser b, Tag t);
void value(SwiftBlock1 b, String v);
void value(SwiftBlock2 b, String v);
void startMessage(SwiftMessage m);
void endMessage(SwiftMessage m);
}