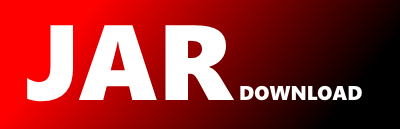
com.prowidesoftware.swift.model.IBAN Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.prowidesoftware.swift.model;
import java.math.BigInteger;
import java.util.logging.Level;
/**
* Utility class to validate IBAN codes.
*
* The IBAN consists of a ISO 3166-1 alpha-2 country code, followed by two check
* digits (represented by kk in the examples below), and up to thirty alphanumeric
* characters for the domestic bank account number, called the BBAN (Basic Bank
* Account Number).
*
* Exampe usage scenario
* IBAN iban = new IBAN("ES2153893489");
* if (iban.isValid())
* System.out.println("ok");
* else
* System.out.println("problem with iban: "+iban.getInvalidCause());
*
*
* @author www.prowidesoftware.com
* @since 3.3
* @version $Id: IBAN.java,v 1.2 2010/10/17 01:22:01 zubri Exp $
*/
public class IBAN {
private static final BigInteger BD_97 = new BigInteger("97");
private static final BigInteger BD_98 = new BigInteger("98");
private String invalidCause = null;
private String iban;
private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(IBAN.class.getName());
/**
* Get the IBAN
* @return a string with the IBAN
*/
public String getIban() {
return iban;
}
/**
* Set the IBAN
* @param iban the IBAN to set
*/
public void setIban(String iban) {
this.iban = iban;
}
/**
* Create an IBAN object with the given iban code.
* This constructor does not perform any validation on the iban, only
* @param iban
*/
public IBAN(String iban) {
this.iban = iban;
}
/**
* Completely validate an IBAN
* Currently validation checks that the length is at least 5 chars:
* (2 country code, 2 verifying digits, and 1 BBAN)
* checks the country code to be valid an the BBAN to match the verifying digits
*
* @return true
if the IBAN is found to be valid and false
in other case
* @throws IllegalStateException if iban is null
*/
public boolean isValid() {
if (this.iban==null)
throw new IllegalStateException("iban is null");
invalidCause = null;
final String code = removeNonAlpha(this.iban);
final int len = code.length();
if (len<4) {
this.invalidCause="Too short (expected at least 4, got "+len+")";
return false;
}
final String country = code.substring(0, 2);
if (!ISOCountries.getInstance().isValidCode(country)) {
this.invalidCause = "Invalid ISO country code: "+country;
return false;
}
// int verification;
// try {
// verification = new Integer(code.substring(2, 4)).intValue();
// } catch (NumberFormatException e) {
// this.invalidCause = "Bad verification code: "+e;
// return false;
// }
final StringBuffer bban = new StringBuffer(code.substring(4));
if (bban.length()==0) {
this.invalidCause="Empty Basic Bank Account Number";
return false;
}
bban.append(code.substring(0, 4));
if (log.isLoggable(Level.FINE)) log.fine("bban: "+bban);
String workString = translateChars(bban);
int mod = modulo97(workString);
if (mod!=1) {
this.invalidCause = "Verification failed (expected 1 and obtained "+mod+")";
return false;
}
return true;
}
/**
* Translate letters to numbers, also ignoring non alphanumeric characters
*
* @param bban
* @return the translated value
*/
public String translateChars(final StringBuffer bban) {
final StringBuffer result = new StringBuffer();
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy