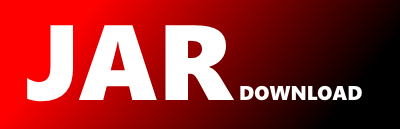
com.prowidesoftware.swift.model.SwiftBlock1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
/*
* Created on Sep 23, 2005
*/
package com.prowidesoftware.swift.model;
import java.io.Serializable;
import org.apache.commons.lang.Validate;
/**
* Base class for SWIFT Basic Header Block (block 1).
* It contains information about the source of the message.
*
* The basic header block is fixed-length and continuous with
* no field delimiters. This class contains its
* elements as individual attributes for easier management
* of the block value.
* This block is mandatory for all SWIFT messages.
*
* @author www.prowidesoftware.com
* @since 4.0
* @version $Id: SwiftBlock1.java,v 1.5 2013/03/07 00:58:28 zubri Exp $
*/
//TODO: add parameter checks (Validate.*) and complete javadocs
public class SwiftBlock1 extends SwiftValueBlock implements Serializable {
//private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(SwiftBlock1.class);
private static final long serialVersionUID = 1L;
/**
* Constant for FIN messages in application id
* @since 4.1
*/
public static transient final String APPLICATION_ID_FIN = "F";
/**
* Constant for GPA (General Purpose Application) messages in application id
* @since 4.1
*/
public static transient final String APPLICATION_ID_GPA = "A";
/**
* Constant for Logins and so messages in application id
* @since 4.1
*/
public static transient final String APPLICATION_ID_LOGINS = "L";
/**
* String of 1 character containing the Application ID as follows:
* F = FIN (financial application)
* A = GPA (general purpose application)
* L = GPA (for logins, and so on)
* This designates the application that has established the
* association used to convey the message. You always use F for FIN messages.
* It is set by default to F (FIN messages).
*/
private String applicationId = "F";
/**
* String of 2 characters containing Service ID as follows:
* 01 = GPA/FIN Message (system and user-to-user)
* 02 = GPA Login
* 03 = GPA Select
* 05 = FIN Quit
* 06 = GPA Logout
* 12 = GPA System Remove AP Request
* 13 = GPA System Abort AP Confirmation
* 14 = GPA System Remove LT Request
* 15 = GPA System Abort LT Confirmation
* 21 = GPA/FIN Message (ACK/NAK/UAK/UNK)
* 22 = GPA Login ACK (LAK)
* 23 = GPA Select ACK (SAK)
* 25 = FIN Quit ACK
* 26 = GPA Logout ACK
* 33 = GPA User Abort AP Request
* 35 = GPA User Abort LT Request
* 42 = GPA Login NAK (LNK)
* 43 = GPA Select NAK (SNK)
* It is set by default to 01 (FIN messages).
*/
private String serviceId = "01";
/**
* The Logical Terminal address of the sender for messages
* sent or the receiver for messages received from the
* SWIFT network.
* It is fixed at 12 characters; it must not have X in position 9
* (padded with "X" if no branch is required).
*/
private String logicalTerminal;
/**
* Session number. 4 characters. It is generated by the user's computer.
* As appropriate, the current application session number based
* on the Login. It is padded with zeros.
*/
private String sessionNumber = "0000";
/**
* Sequence number is a 6 characters string that is generated by the
* user's computer.
* For all FIN messages with a Service Identifier of 01 or 05,
* this number is the next expected sequence number appropriate to
* the direction of the transmission.
* For FIN messages with a Service Identifier of 21 or 25,
* the sequence number is that of the acknowledged service message.
* It is padded with zeros.
*/
private String sequenceNumber = "000000";
/**
* Constructor for specific values
*
* @param applicationId the application id
* @param serviceId the service id
* @param logicalTerminal the logical terminal name
* @param sessionNumber the session number
* @param sequenceNumber the message sequence number
*/
public SwiftBlock1(final String applicationId, final String serviceId, final String logicalTerminal, final String sessionNumber, final String sequenceNumber) {
this.applicationId = applicationId;
this.serviceId = serviceId;
this.logicalTerminal = logicalTerminal;
this.sessionNumber = sessionNumber;
this.sequenceNumber = sequenceNumber;
}
/**
* Default constructor
*/
public SwiftBlock1() {
super();
}
/**
* Creates a block 1 object setting attributes by parsing the fixed string argument;
* for example "F01BANKBEBBAXXX2222123456" or "1:F01BANKBEBBAXXX2222123456"
*
* @param value a fixed length string of 25 or 27 (which must start with '1:') characters containing the blocks value
*/
public SwiftBlock1(final String value) {
this.setValue(value);
}
/**
* Sets the block number.
* @param blockNumber the block number to set
* @throws IllegalArgumentException if parameter blockName is not the integer 1
* @since 5.0
*/
protected void setBlockNumber(final Integer blockNumber) {
// sanity check
Validate.notNull(blockNumber, "parameter 'blockNumber' cannot be null");
Validate.isTrue(blockNumber.intValue() == 1, "blockNumber must be 1");
}
/**
* Sets the block name. Will cause an exception unless setting block number to 1.
* @param blockName the block name to set
* @throws IllegalArgumentException if parameter blockName is not the string "1"
* @since 5.0
*/
protected void setBlockName(final String blockName) {
// sanity check
Validate.notNull(blockName, "parameter 'blockName' cannot be null");
Validate.isTrue(blockName.compareTo("1") == 0, "blockName must be string '1'");
}
/**
* Returns the block number (the value 1 as an integer)
* @return Integer containing the block's number
*/
public Integer getNumber() {
return new Integer(1);
}
/**
* Returns the block name (the value 1 as a string)
* @return block name
*
* @since 5.0
*/
public String getName() {
return ("1");
}
/**
* Sets the applicationId
*
* @param applicationId String of 1 character containing the Application ID (F, A or L)
*/
public void setApplicationId(final String applicationId) {
this.applicationId = applicationId;
}
/**
* Gets the application ID field in block 1
* @return application ID field in block 1
*/
public String getApplicationId() {
return applicationId;
}
/**
* Sets the Service ID
*
* @param serviceId string of 2 characters containing Service ID (01, 02, 03, etc...)
*/
public void setServiceId(final String serviceId) {
this.serviceId = serviceId;
}
/**
* Gets the service ID field in block 1
* @return service ID field in block 1
*/
public String getServiceId() {
return serviceId;
}
/**
* Sets the The Logical Terminal address of the sender for messages
* sent or the receiver for messages received from the SWIFT network.
*
* @param logicalTerminal it is fixed at 12 characters; it must not have X in position 9 (padded with "X" if no branch is required).
*/
public void setLogicalTerminal(final String logicalTerminal) {
this.logicalTerminal = logicalTerminal;
}
/**
* Sets the Logical Terminal.
*
* @see #setLogicalTerminal(String)
* @since 6.4
*/
public void setSender(final String BIC) {
setLogicalTerminal(BIC);
}
/**
* Gets the The Logical Terminal address of the sender for messages
* sent or the receiver for messages received from the SWIFT network.
*
* @return the 12 characters logical terminal address
*/
public String getLogicalTerminal() {
return logicalTerminal;
}
/**
* Sets the Session number. It is generated by the user's computer.
* As appropriate, the current application session number based
* on the Login. It is padded with zeros.
*
* @param sessionNumber 4 numbers.
*/
public void setSessionNumber(final String sessionNumber) {
this.sessionNumber = sessionNumber;
}
/**
* Gets the session number in block 1
* @return session number in block 1
*/
public String getSessionNumber() {
return sessionNumber;
}
/**
* Sets the Sequence number that is generated by the
* user's computer.
* For all FIN messages with a Service Identifier of 01 or 05,
* this number is the next expected sequence number appropriate to
* the direction of the transmission.
* For FIN messages with a Service Identifier of 21 or 25,
* the sequence number is that of the acknowledged service message.
* It is padded with zeros.
*
* @param sequenceNumber 6 numbers
*/
public void setSequenceNumber(final String sequenceNumber) {
this.sequenceNumber = sequenceNumber;
}
/**
* Gets the sequence number field in block 1
* @return sequence number field in block 1
*/
public String getSequenceNumber() {
return sequenceNumber;
}
/**
* Tell if this block is empty or not.
* This block is considered to be empty if all its attributes are set to null
.
* @return true
if all fields are null
and false in other case
*/
public boolean isEmpty() {
return (applicationId == null && serviceId == null && logicalTerminal == null && sessionNumber == null && sequenceNumber == null);
}
/**
* Gets the fixed length block 1 value, as a result of
* concatenating its individual elements as follow:
* Application ID Service ID +
* Logical terminal (LT) address +
* Session number +
* Sequence number.
* Notice that this method does not return the "1:" string.
*/
public String getValue() {
if (isEmpty()) {
return null;
}
final StringBuffer v = new StringBuffer();
if (applicationId != null) {
v.append(applicationId);
}
if (serviceId != null) {
v.append(serviceId);
}
if (logicalTerminal != null) {
v.append(logicalTerminal);
}
if (sessionNumber != null) {
v.append(sessionNumber);
}
if (sequenceNumber != null) {
v.append(sequenceNumber);
}
return v.toString();
}
/**
* @see #getValue()
*/
public String getBlockValue() {
return getValue();
}
/**
* Sets the block's attributes by parsing the fixed string argument;
* for example "F01BANKBEBBAXXX2222123456" or "1:F01BANKBEBBAXXX2222123456"
*
* @param value a fixed length string of 25 or 27 (which must start with '1:') characters containing the blocks value
*/
public void setValue(final String value) {
// check parameters
final int slen = value.length();
Validate.notNull(value, "value must not be null");
Validate.isTrue(slen == 25 || slen == 27 || slen == 15 || slen == 17, "expected a 25 (or 27) or 15 (or 17) chars string and obtained a " + value.length() + " chars string: " + value);
// figure out the starting point
int offset = 0;
if (slen == 27 || slen == 17) {
Validate.isTrue(value.startsWith("1:"), "expected '1:' at the beginning of value and found '" + value.substring(0, 1) + "'");
offset = 2;
}
;
// separate value fragments
int len;
len = 1;
this.setApplicationId(this.getValuePart(value, offset, len));
offset += len;
len = 2;
this.setServiceId(this.getValuePart(value, offset, len));
offset += len;
len = 12;
this.setLogicalTerminal(this.getValuePart(value, offset, len));
offset += len;
len = 4;
this.setSessionNumber(this.getValuePart(value, offset, len));
offset += len;
len = 6;
this.setSequenceNumber(this.getValuePart(value, offset, len));
offset += len;
}
/**
* @see #setValue(String)
*/
public void setBlockValue(final String value) {
setValue(value);
}
/**
* Sets all attributes to null
* @since 6.4
*/
public void clean() {
applicationId = null;
serviceId = null;
logicalTerminal = null;
sessionNumber = null;
sequenceNumber = null;
}
public int hashCode() {
final int prime = 31;
int result = super.hashCode();
result = prime * result + ((applicationId == null) ? 0 : applicationId.hashCode());
result = prime * result + ((logicalTerminal == null) ? 0 : logicalTerminal.hashCode());
result = prime * result + ((sequenceNumber == null) ? 0 : sequenceNumber.hashCode());
result = prime * result + ((serviceId == null) ? 0 : serviceId.hashCode());
result = prime * result + ((sessionNumber == null) ? 0 : sessionNumber.hashCode());
return result;
}
public boolean equals(final Object obj) {
if (this == obj) {
return true;
}
if (!super.equals(obj)) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final SwiftBlock1 other = (SwiftBlock1) obj;
if (applicationId == null) {
if (other.applicationId != null) {
return false;
}
} else if (!applicationId.equals(other.applicationId)) {
return false;
}
if (logicalTerminal == null) {
if (other.logicalTerminal != null) {
return false;
}
} else if (!logicalTerminal.equals(other.logicalTerminal)) {
return false;
}
if (sequenceNumber == null) {
if (other.sequenceNumber != null) {
return false;
}
} else if (!sequenceNumber.equals(other.sequenceNumber)) {
return false;
}
if (serviceId == null) {
if (other.serviceId != null) {
return false;
}
} else if (!serviceId.equals(other.serviceId)) {
return false;
}
if (sessionNumber == null) {
if (other.sessionNumber != null) {
return false;
}
} else if (!sessionNumber.equals(other.sessionNumber)) {
return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy