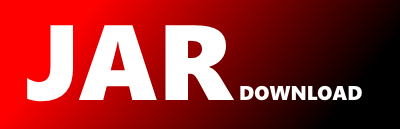
com.prowidesoftware.swift.model.field.Field50F Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
*/
package com.prowidesoftware.swift.model.field;
import java.io.Serializable;
import org.apache.commons.lang.StringUtils;
import com.prowidesoftware.swift.model.field.PatternContainer;
import com.prowidesoftware.swift.model.*;
import com.prowidesoftware.swift.utils.SwiftFormatUtils;
/**
* Field 50F
*
* validation pattern: CUSTOM
* parser pattern: S$S/S[$S/S]0-3
* components pattern: SNSNSNSNS
*
* Components Data types
*
* - component1:
String
* - component2:
Number
* - component3:
String
* - component4:
Number
* - component5:
String
* - component6:
Number
* - component7:
String
* - component8:
Number
* - component9:
String
*
*
* NOTE: this source code has been generated.
*
* @author www.prowidesoftware.com
*/
@SuppressWarnings("unused")
public class Field50F extends Field implements Serializable, PatternContainer {
private static final long serialVersionUID = 1L;
public static final String NAME = "50F";
public static final String PARSER_PATTERN ="S$S/S[$S/S]0-3";
public static final String COMPONENTS_PATTERN = "SNSNSNSNS";
/**
* Default constructor
*/
public Field50F() {
super(9);
}
/**
* Creates the field parsing the parameter value into fields' components
* @param value
*/
public Field50F(String value) {
this();
java.util.List lines = SwiftParseUtils.getLines(value);
if (lines.size() > 0) {
setComponent1(lines.get(0));
}
if (lines.size() > 1) {
setComponent2(SwiftParseUtils.getTokenFirst(lines.get(1), "/"));
setComponent3(SwiftParseUtils.getTokenSecondLast(lines.get(1), "/"));
}
if (lines.size() > 2) {
setComponent4(SwiftParseUtils.getTokenFirst(lines.get(2), "/"));
setComponent5(SwiftParseUtils.getTokenSecondLast(lines.get(2), "/"));
}
if (lines.size() > 3) {
setComponent6(SwiftParseUtils.getTokenFirst(lines.get(3), "/"));
setComponent7(SwiftParseUtils.getTokenSecondLast(lines.get(3), "/"));
}
if (lines.size() > 4) {
setComponent8(SwiftParseUtils.getTokenFirst(lines.get(4), "/"));
setComponent9(SwiftParseUtils.getTokenSecondLast(lines.get(4), "/"));
}
}
/**
* Serializes the fields' components into the single string value (SWIFT format)
*/
@Override
public String getValue() {
final StringBuilder result = new StringBuilder();
result.append(StringUtils.trimToEmpty(getComponent1()));
result.append(com.prowidesoftware.swift.io.writer.FINWriterVisitor.SWIFT_EOL);
result.append(StringUtils.trimToEmpty(getComponent2()));
result.append("/");
result.append(StringUtils.trimToEmpty(getComponent3()));
if (StringUtils.isNotEmpty(getComponent4()) || StringUtils.isNotEmpty(getComponent5())) {
result.append(com.prowidesoftware.swift.io.writer.FINWriterVisitor.SWIFT_EOL);
result.append(StringUtils.trimToEmpty(getComponent4()));
result.append("/");
result.append(StringUtils.trimToEmpty(getComponent5()));
}
if (StringUtils.isNotEmpty(getComponent6()) || StringUtils.isNotEmpty(getComponent7())) {
result.append(com.prowidesoftware.swift.io.writer.FINWriterVisitor.SWIFT_EOL);
result.append(StringUtils.trimToEmpty(getComponent6()));
result.append("/");
result.append(StringUtils.trimToEmpty(getComponent7()));
}
if (StringUtils.isNotEmpty(getComponent8()) || StringUtils.isNotEmpty(getComponent9())) {
result.append(com.prowidesoftware.swift.io.writer.FINWriterVisitor.SWIFT_EOL);
result.append(StringUtils.trimToEmpty(getComponent8()));
result.append("/");
result.append(StringUtils.trimToEmpty(getComponent9()));
}
return result.toString();
}
/**
* Get the component1
* @return the component1
*/
public String getComponent1() {
return getComponent(1);
}
/**
* Set the component1.
* @param component1 the component1 to set
*/
public void setComponent1(String component1) {
setComponent(1, component1);
}
/**
* Get the component2
* @return the component2
*/
public String getComponent2() {
return getComponent(2);
}
/**
* Get the component2 as Number
* @return the component2 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent2AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(2));
}
/**
* Set the component2.
* @param component2 the component2 to set
*/
public void setComponent2(String component2) {
setComponent(2, component2);
}
/**
* Set the component2.
* @param Number with the component2 content to set
*/
public void setComponent2(java.lang.Number component2) {
setComponent(2, SwiftFormatUtils.getNumber(component2));
}
/**
* Get the component3
* @return the component3
*/
public String getComponent3() {
return getComponent(3);
}
/**
* Set the component3.
* @param component3 the component3 to set
*/
public void setComponent3(String component3) {
setComponent(3, component3);
}
/**
* Get the component4
* @return the component4
*/
public String getComponent4() {
return getComponent(4);
}
/**
* Get the component4 as Number
* @return the component4 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent4AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(4));
}
/**
* Set the component4.
* @param component4 the component4 to set
*/
public void setComponent4(String component4) {
setComponent(4, component4);
}
/**
* Set the component4.
* @param Number with the component4 content to set
*/
public void setComponent4(java.lang.Number component4) {
setComponent(4, SwiftFormatUtils.getNumber(component4));
}
/**
* Get the component5
* @return the component5
*/
public String getComponent5() {
return getComponent(5);
}
/**
* Set the component5.
* @param component5 the component5 to set
*/
public void setComponent5(String component5) {
setComponent(5, component5);
}
/**
* Get the component6
* @return the component6
*/
public String getComponent6() {
return getComponent(6);
}
/**
* Get the component6 as Number
* @return the component6 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent6AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(6));
}
/**
* Set the component6.
* @param component6 the component6 to set
*/
public void setComponent6(String component6) {
setComponent(6, component6);
}
/**
* Set the component6.
* @param Number with the component6 content to set
*/
public void setComponent6(java.lang.Number component6) {
setComponent(6, SwiftFormatUtils.getNumber(component6));
}
/**
* Get the component7
* @return the component7
*/
public String getComponent7() {
return getComponent(7);
}
/**
* Set the component7.
* @param component7 the component7 to set
*/
public void setComponent7(String component7) {
setComponent(7, component7);
}
/**
* Get the component8
* @return the component8
*/
public String getComponent8() {
return getComponent(8);
}
/**
* Get the component8 as Number
* @return the component8 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent8AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(8));
}
/**
* Set the component8.
* @param component8 the component8 to set
*/
public void setComponent8(String component8) {
setComponent(8, component8);
}
/**
* Set the component8.
* @param Number with the component8 content to set
*/
public void setComponent8(java.lang.Number component8) {
setComponent(8, SwiftFormatUtils.getNumber(component8));
}
/**
* Get the component9
* @return the component9
*/
public String getComponent9() {
return getComponent(9);
}
/**
* Set the component9.
* @param component9 the component9 to set
*/
public void setComponent9(String component9) {
setComponent(9, component9);
}
/**
* Given a component number it returns true if the component is optional,
* regardless of the field being mandatory in a particular message.
* Being the field's value conformed by a composition of one or several
* internal component values, the field may be present in a message with
* a proper value but with some of its internal components not set.
*
* @param component component number, first component of a field is referenced as 1
* @return true if the component is optional for this field, false otherwise
*/
@Override
public boolean isOptional(int component) {
if (component == 4) {
return true;
}
if (component == 5) {
return true;
}
if (component == 6) {
return true;
}
if (component == 7) {
return true;
}
if (component == 8) {
return true;
}
if (component == 9) {
return true;
}
return false;
}
/**
* Returns true if the field is a GENERIC FIELD as specified by the standard.
*
* @return true if the field is generic, false otherwise
*/
@Override
public boolean isGeneric() {
return false;
}
public String componentsPattern() {
return COMPONENTS_PATTERN;
}
public String parserPattern() {
return PARSER_PATTERN;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy