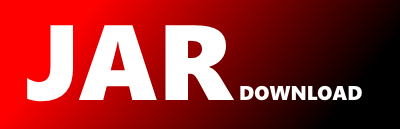
com.prowidesoftware.swift.model.field.Field68B Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
*/
package com.prowidesoftware.swift.model.field;
import java.io.Serializable;
import java.util.List;
import java.util.ArrayList;
import java.util.Currency;
import java.util.Calendar;
import java.math.BigDecimal;
import org.apache.commons.lang.StringUtils;
import com.prowidesoftware.swift.model.field.PatternContainer;
import com.prowidesoftware.swift.model.*;
import com.prowidesoftware.swift.utils.SwiftFormatUtils;
/**
* Field 68B
*
* validation pattern: 16x/1a$3!a15$15/15
* parser pattern: S/cSN$SN/SN
* components pattern: EEScSNCNECN
*
* Components Data types
*
* - component1:
Calendar
* - component2:
Calendar
* - component3:
String
* - component4:
Character
* - component5:
String
* - component6:
Number
* - component7:
Currency
* - component8:
Number
* - component9:
Calendar
* - component10:
Currency
* - component11:
Number
*
*
* NOTE: this source code has been generated.
*
* @author www.prowidesoftware.com
*/
@SuppressWarnings("unused")
public class Field68B extends Field implements Serializable, PatternContainer, CurrencyContainer, DateContainer, AmountContainer {
private static final long serialVersionUID = 1L;
public static final String NAME = "68B";
public static final String PARSER_PATTERN ="S/cSN$SN/SN";
public static final String COMPONENTS_PATTERN = "EEScSNCNECN";
/**
* Default constructor
*/
public Field68B() {
super(11);
}
/**
* Creates the field parsing the parameter value into fields' components
* @param value
*/
public Field68B(String value) {
this();
java.util.List lines = SwiftParseUtils.getLines(value);
if (lines.size() > 0) {
if (lines.get(0) != null) {
String toparse = SwiftParseUtils.getTokenFirst(lines.get(0), "/");
if (toparse != null && toparse.length() >= 6) {
setComponent1(org.apache.commons.lang.StringUtils.substring(toparse, 0, 6));
}
if (toparse != null && toparse.length() >= 12) {
setComponent2(org.apache.commons.lang.StringUtils.substring(toparse, 6, 12));
}
if (toparse != null && toparse.length() > 12) {
setComponent3(org.apache.commons.lang.StringUtils.substring(toparse, 12));
}
toparse = SwiftParseUtils.getAlphaPrefix(SwiftParseUtils.getTokenSecond(lines.get(0), "/"));
if (toparse != null && toparse.length() >= 1) {
setComponent4(org.apache.commons.lang.StringUtils.substring(toparse, 0, 1));
}
if (toparse != null && toparse.length() > 1) {
setComponent5(org.apache.commons.lang.StringUtils.substring(toparse, 1));
}
setComponent6(SwiftParseUtils.getNumericSuffix(SwiftParseUtils.getTokenSecond(lines.get(0), "/")));
}
}
if (lines.size() > 1) {
if (lines.get(1) != null) {
String toparse = SwiftParseUtils.getTokenFirst(lines.get(1), "/");
setComponent7(SwiftParseUtils.getAlphaPrefix(toparse));
setComponent8(SwiftParseUtils.getNumericSuffix(toparse));
toparse = SwiftParseUtils.getTokenSecondLast(lines.get(1), "/");
if (toparse != null && toparse.length() >= 6) {
setComponent9(org.apache.commons.lang.StringUtils.substring(toparse, 0, 6));
String toparse2 = org.apache.commons.lang.StringUtils.substring(toparse, 6);
setComponent10(SwiftParseUtils.getAlphaPrefix(toparse2));
setComponent11(SwiftParseUtils.getNumericSuffix(toparse2));
}
}
}
}
/**
* Serializes the fields' components into the single string value (SWIFT format)
*/
@Override
public String getValue() {
final StringBuilder result = new StringBuilder();
//TODO serialization
return result.toString();
}
/**
* Get the component1
* @return the component1
*/
public String getComponent1() {
return getComponent(1);
}
/**
* Get the component1 as Calendar
* @return the component1 converted to Calendar or null
if cannot be converted
*/
public java.util.Calendar getComponent1AsCalendar() {
return SwiftFormatUtils.getDate2(getComponent(1));
}
/**
* Get the Trade Date (component1).
* @return the Trade Date from component1
*/
public String getTradeDate() {
return getComponent(1);
}
/**
* Get the Trade Date (component1) as Calendar
* @return the Trade Date from component1 converted to Calendar or null
if cannot be converted
*/
public java.util.Calendar getTradeDateAsCalendar() {
return SwiftFormatUtils.getDate2(getComponent(1));
}
/**
* Set the component1.
* @param component1 the component1 to set
*/
public void setComponent1(String component1) {
setComponent(1, component1);
}
/**
* Set the component1.
* @param Calendar with the component1 content to set
*/
public void setComponent1(java.util.Calendar component1) {
setComponent(1, SwiftFormatUtils.getDate2(component1));
}
/**
* Set the Trade Date (component1).
* @param component1 the Trade Date to set
*/
public void setTradeDate(String component1) {
setComponent(1, component1);
}
/**
* Set the Trade Date (component1) as Calendar
* @param Calendar with the Trade Date content to set
*/
public void setTradeDate(java.util.Calendar component1) {
setComponent(1, SwiftFormatUtils.getDate2(component1));
}
/**
* Get the component2
* @return the component2
*/
public String getComponent2() {
return getComponent(2);
}
/**
* Get the component2 as Calendar
* @return the component2 converted to Calendar or null
if cannot be converted
*/
public java.util.Calendar getComponent2AsCalendar() {
return SwiftFormatUtils.getDate2(getComponent(2));
}
/**
* Get the Maturity Date (component2).
* @return the Maturity Date from component2
*/
public String getMaturityDate() {
return getComponent(2);
}
/**
* Get the Maturity Date (component2) as Calendar
* @return the Maturity Date from component2 converted to Calendar or null
if cannot be converted
*/
public java.util.Calendar getMaturityDateAsCalendar() {
return SwiftFormatUtils.getDate2(getComponent(2));
}
/**
* Set the component2.
* @param component2 the component2 to set
*/
public void setComponent2(String component2) {
setComponent(2, component2);
}
/**
* Set the component2.
* @param Calendar with the component2 content to set
*/
public void setComponent2(java.util.Calendar component2) {
setComponent(2, SwiftFormatUtils.getDate2(component2));
}
/**
* Set the Maturity Date (component2).
* @param component2 the Maturity Date to set
*/
public void setMaturityDate(String component2) {
setComponent(2, component2);
}
/**
* Set the Maturity Date (component2) as Calendar
* @param Calendar with the Maturity Date content to set
*/
public void setMaturityDate(java.util.Calendar component2) {
setComponent(2, SwiftFormatUtils.getDate2(component2));
}
/**
* Get the component3
* @return the component3
*/
public String getComponent3() {
return getComponent(3);
}
/**
* Get the Contract Number (component3).
* @return the Contract Number from component3
*/
public String getContractNumber() {
return getComponent(3);
}
/**
* Set the component3.
* @param component3 the component3 to set
*/
public void setComponent3(String component3) {
setComponent(3, component3);
}
/**
* Set the Contract Number (component3).
* @param component3 the Contract Number to set
*/
public void setContractNumber(String component3) {
setComponent(3, component3);
}
/**
* Get the component4
* @return the component4
*/
public String getComponent4() {
return getComponent(4);
}
/**
* Get the Indicator (component4).
* @return the Indicator from component4
*/
public String getIndicator() {
return getComponent(4);
}
/**
* Set the component4.
* @param component4 the component4 to set
*/
public void setComponent4(String component4) {
setComponent(4, component4);
}
/**
* Set the Indicator (component4).
* @param component4 the Indicator to set
*/
public void setIndicator(String component4) {
setComponent(4, component4);
}
/**
* Get the component5
* @return the component5
*/
public String getComponent5() {
return getComponent(5);
}
/**
* Get the Unit (component5).
* @return the Unit from component5
*/
public String getUnit() {
return getComponent(5);
}
/**
* Set the component5.
* @param component5 the component5 to set
*/
public void setComponent5(String component5) {
setComponent(5, component5);
}
/**
* Set the Unit (component5).
* @param component5 the Unit to set
*/
public void setUnit(String component5) {
setComponent(5, component5);
}
/**
* Get the component6
* @return the component6
*/
public String getComponent6() {
return getComponent(6);
}
/**
* Get the component6 as Number
* @return the component6 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent6AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(6));
}
/**
* Get the Quantity Of The Commodity (component6).
* @return the Quantity Of The Commodity from component6
*/
public String getQuantityOfTheCommodity() {
return getComponent(6);
}
/**
* Get the Quantity Of The Commodity (component6) as Number
* @return the Quantity Of The Commodity from component6 converted to Number or null
if cannot be converted
*/
public java.lang.Number getQuantityOfTheCommodityAsNumber() {
return SwiftFormatUtils.getNumber(getComponent(6));
}
/**
* Set the component6.
* @param component6 the component6 to set
*/
public void setComponent6(String component6) {
setComponent(6, component6);
}
/**
* Set the component6.
* @param Number with the component6 content to set
*/
public void setComponent6(java.lang.Number component6) {
setComponent(6, SwiftFormatUtils.getNumber(component6));
}
/**
* Set the Quantity Of The Commodity (component6).
* @param component6 the Quantity Of The Commodity to set
*/
public void setQuantityOfTheCommodity(String component6) {
setComponent(6, component6);
}
/**
* Set the Quantity Of The Commodity (component6) as Number
* @param Number with the Quantity Of The Commodity content to set
*/
public void setQuantityOfTheCommodity(java.lang.Number component6) {
setComponent(6, SwiftFormatUtils.getNumber(component6));
}
/**
* Get the component7
* @return the component7
*/
public String getComponent7() {
return getComponent(7);
}
/**
* Get the component7 as Currency
* @return the component7 converted to Currency or null
if cannot be converted
*/
public java.util.Currency getComponent7AsCurrency() {
return SwiftFormatUtils.getCurrency(getComponent(7));
}
/**
* Get the Price Per Unit Currency (component7).
* @return the Price Per Unit Currency from component7
*/
public String getPricePerUnitCurrency() {
return getComponent(7);
}
/**
* Get the Price Per Unit Currency (component7) as Currency
* @return the Price Per Unit Currency from component7 converted to Currency or null
if cannot be converted
*/
public java.util.Currency getPricePerUnitCurrencyAsCurrency() {
return SwiftFormatUtils.getCurrency(getComponent(7));
}
/**
* Set the component7.
* @param component7 the component7 to set
*/
public void setComponent7(String component7) {
setComponent(7, component7);
}
/**
* Set the component7.
* @param Currency with the component7 content to set
*/
public void setComponent7(java.util.Currency component7) {
setComponent(7, SwiftFormatUtils.getCurrency(component7));
}
/**
* Set the Price Per Unit Currency (component7).
* @param component7 the Price Per Unit Currency to set
*/
public void setPricePerUnitCurrency(String component7) {
setComponent(7, component7);
}
/**
* Set the Price Per Unit Currency (component7) as Currency
* @param Currency with the Price Per Unit Currency content to set
*/
public void setPricePerUnitCurrency(java.util.Currency component7) {
setComponent(7, SwiftFormatUtils.getCurrency(component7));
}
/**
* Get the component8
* @return the component8
*/
public String getComponent8() {
return getComponent(8);
}
/**
* Get the component8 as Number
* @return the component8 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent8AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(8));
}
/**
* Get the Price Per Unit (component8).
* @return the Price Per Unit from component8
*/
public String getPricePerUnit() {
return getComponent(8);
}
/**
* Get the Price Per Unit (component8) as Number
* @return the Price Per Unit from component8 converted to Number or null
if cannot be converted
*/
public java.lang.Number getPricePerUnitAsNumber() {
return SwiftFormatUtils.getNumber(getComponent(8));
}
/**
* Set the component8.
* @param component8 the component8 to set
*/
public void setComponent8(String component8) {
setComponent(8, component8);
}
/**
* Set the component8.
* @param Number with the component8 content to set
*/
public void setComponent8(java.lang.Number component8) {
setComponent(8, SwiftFormatUtils.getNumber(component8));
}
/**
* Set the Price Per Unit (component8).
* @param component8 the Price Per Unit to set
*/
public void setPricePerUnit(String component8) {
setComponent(8, component8);
}
/**
* Set the Price Per Unit (component8) as Number
* @param Number with the Price Per Unit content to set
*/
public void setPricePerUnit(java.lang.Number component8) {
setComponent(8, SwiftFormatUtils.getNumber(component8));
}
/**
* Get the component9
* @return the component9
*/
public String getComponent9() {
return getComponent(9);
}
/**
* Get the component9 as Calendar
* @return the component9 converted to Calendar or null
if cannot be converted
*/
public java.util.Calendar getComponent9AsCalendar() {
return SwiftFormatUtils.getDate2(getComponent(9));
}
/**
* Get the Value Date Of The Consideration (component9).
* @return the Value Date Of The Consideration from component9
*/
public String getValueDateOfTheConsideration() {
return getComponent(9);
}
/**
* Get the Value Date Of The Consideration (component9) as Calendar
* @return the Value Date Of The Consideration from component9 converted to Calendar or null
if cannot be converted
*/
public java.util.Calendar getValueDateOfTheConsiderationAsCalendar() {
return SwiftFormatUtils.getDate2(getComponent(9));
}
/**
* Set the component9.
* @param component9 the component9 to set
*/
public void setComponent9(String component9) {
setComponent(9, component9);
}
/**
* Set the component9.
* @param Calendar with the component9 content to set
*/
public void setComponent9(java.util.Calendar component9) {
setComponent(9, SwiftFormatUtils.getDate2(component9));
}
/**
* Set the Value Date Of The Consideration (component9).
* @param component9 the Value Date Of The Consideration to set
*/
public void setValueDateOfTheConsideration(String component9) {
setComponent(9, component9);
}
/**
* Set the Value Date Of The Consideration (component9) as Calendar
* @param Calendar with the Value Date Of The Consideration content to set
*/
public void setValueDateOfTheConsideration(java.util.Calendar component9) {
setComponent(9, SwiftFormatUtils.getDate2(component9));
}
/**
* Get the component10
* @return the component10
*/
public String getComponent10() {
return getComponent(10);
}
/**
* Get the component10 as Currency
* @return the component10 converted to Currency or null
if cannot be converted
*/
public java.util.Currency getComponent10AsCurrency() {
return SwiftFormatUtils.getCurrency(getComponent(10));
}
/**
* Get the Consideration Currency (component10).
* @return the Consideration Currency from component10
*/
public String getConsiderationCurrency() {
return getComponent(10);
}
/**
* Get the Consideration Currency (component10) as Currency
* @return the Consideration Currency from component10 converted to Currency or null
if cannot be converted
*/
public java.util.Currency getConsiderationCurrencyAsCurrency() {
return SwiftFormatUtils.getCurrency(getComponent(10));
}
/**
* Set the component10.
* @param component10 the component10 to set
*/
public void setComponent10(String component10) {
setComponent(10, component10);
}
/**
* Set the component10.
* @param Currency with the component10 content to set
*/
public void setComponent10(java.util.Currency component10) {
setComponent(10, SwiftFormatUtils.getCurrency(component10));
}
/**
* Set the Consideration Currency (component10).
* @param component10 the Consideration Currency to set
*/
public void setConsiderationCurrency(String component10) {
setComponent(10, component10);
}
/**
* Set the Consideration Currency (component10) as Currency
* @param Currency with the Consideration Currency content to set
*/
public void setConsiderationCurrency(java.util.Currency component10) {
setComponent(10, SwiftFormatUtils.getCurrency(component10));
}
/**
* Get the component11
* @return the component11
*/
public String getComponent11() {
return getComponent(11);
}
/**
* Get the component11 as Number
* @return the component11 converted to Number or null
if cannot be converted
*/
public java.lang.Number getComponent11AsNumber() {
return SwiftFormatUtils.getNumber(getComponent(11));
}
/**
* Get the Consideration (component11).
* @return the Consideration from component11
*/
public String getConsideration() {
return getComponent(11);
}
/**
* Get the Consideration (component11) as Number
* @return the Consideration from component11 converted to Number or null
if cannot be converted
*/
public java.lang.Number getConsiderationAsNumber() {
return SwiftFormatUtils.getNumber(getComponent(11));
}
/**
* Set the component11.
* @param component11 the component11 to set
*/
public void setComponent11(String component11) {
setComponent(11, component11);
}
/**
* Set the component11.
* @param Number with the component11 content to set
*/
public void setComponent11(java.lang.Number component11) {
setComponent(11, SwiftFormatUtils.getNumber(component11));
}
/**
* Set the Consideration (component11).
* @param component11 the Consideration to set
*/
public void setConsideration(String component11) {
setComponent(11, component11);
}
/**
* Set the Consideration (component11) as Number
* @param Number with the Consideration content to set
*/
public void setConsideration(java.lang.Number component11) {
setComponent(11, SwiftFormatUtils.getNumber(component11));
}
public List currencyStrings() {
List result = new ArrayList();
result = CurrencyResolver.resolveComponentsPattern(COMPONENTS_PATTERN, components);
return result;
}
public List currencies() {
final List l = currencyStrings();
if (l.isEmpty()) {
return java.util.Collections.emptyList();
}
final ArrayList result = new ArrayList();
for (String s: l) {
result.add(Currency.getInstance(s));
}
return result;
}
public Currency currency() {
return CurrencyResolver.resolveCurrency(this);
}
public String currencyString() {
return CurrencyResolver.resolveCurrencyString(this);
}
public void initializeCurrencies(String cur) {
CurrencyResolver.resolveSetCurrency(this, cur);
}
public void initializeCurrencies(Currency cur) {
CurrencyResolver.resolveSetCurrency(this, cur);
}
public List dates() {
List result = new java.util.ArrayList();
result.add(SwiftFormatUtils.getDate2(getComponent(1)));
result.add(SwiftFormatUtils.getDate2(getComponent(2)));
result.add(SwiftFormatUtils.getDate2(getComponent(9)));
return result;
}
public List amounts() {
return AmountResolver.amounts(this);
}
public BigDecimal amount() {
return AmountResolver.amount(this);
}
/**
* Given a component number it returns true if the component is optional,
* regardless of the field being mandatory in a particular message.
* Being the field's value conformed by a composition of one or several
* internal component values, the field may be present in a message with
* a proper value but with some of its internal components not set.
*
* @param component component number, first component of a field is referenced as 1
* @return true if the component is optional for this field, false otherwise
*/
@Override
public boolean isOptional(int component) {
return false;
}
/**
* Returns true if the field is a GENERIC FIELD as specified by the standard.
*
* @return true if the field is generic, false otherwise
*/
@Override
public boolean isGeneric() {
return false;
}
public String componentsPattern() {
return COMPONENTS_PATTERN;
}
public String parserPattern() {
return PARSER_PATTERN;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy