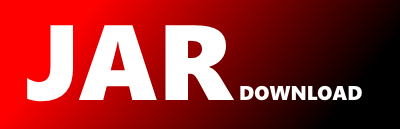
com.prowidesoftware.swift.model.mt.mt1xx.MT107 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/* * This library is free software; you can redistribute it and/or * modify it under the terms of the GNU Lesser General Public * License as published by the Free Software Foundation; either * version 2.1 of the License, or (at your option) any later version. * * This library is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * Lesser General Public License for more details. * */ package com.prowidesoftware.swift.model.mt.mt1xx; import java.io.Serializable; import java.util.ArrayList; import java.util.Collections; import java.util.List; import com.prowidesoftware.swift.model.*; import com.prowidesoftware.swift.model.field.*; import com.prowidesoftware.swift.model.mt.AbstractMT; /** * MT 107
if none is found.
* * * NOTE: this source code has been generated from template * * @author www.prowidesoftware.com */ public class MT107 extends AbstractMT implements Serializable { private static final long serialVersionUID = 1L; private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT107.class.getName()); /** * Creates an MT107 initialized with the parameter SwiftMessage * @param m swift message with the MT107 content */ public MT107(SwiftMessage m) { super(m); } /** * Creates an MT107 initialized with a new SwiftMessage */ public MT107() { super(); } /** * Returns this MT number * @return the message type number of this MT * @since 6.4 */ @Override public String getMessageType() { return "107"; } /** * Iterates through block4 fields and return the first one whose name matches 20, * ornull
if none is found.
* The first occurrence of field 20 at MT107 is expected to be the only one. * * @return a Field20 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field20 getField20() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("20"); if (t == null) { log.fine("field 20 not found"); return null; } else { return new Field20(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 30, * ornull
if none is found.
* The first occurrence of field 30 at MT107 is expected to be the only one. * * @return a Field30 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field30 getField30() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("30"); if (t == null) { log.fine("field 30 not found"); return null; } else { return new Field30(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 51A, * ornull
if none is found.
* The first occurrence of field 51A at MT107 is expected to be the only one. * * @return a Field51A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field51A getField51A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("51A"); if (t == null) { log.fine("field 51A not found"); return null; } else { return new Field51A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 72, * ornull
if none is found.
* The first occurrence of field 72 at MT107 is expected to be the only one. * * @return a Field72 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field72 getField72() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("72"); if (t == null) { log.fine("field 72 not found"); return null; } else { return new Field72(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 19, * ornull
if none is found.
* The first occurrence of field 19 at MT107 is expected to be the only one. * * @return a Field19 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field19 getField19() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("19"); if (t == null) { log.fine("field 19 not found"); return null; } else { return new Field19(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 53A, * ornull
if none is found.
* The first occurrence of field 53A at MT107 is expected to be the only one. * * @return a Field53A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field53A getField53A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("53A"); if (t == null) { log.fine("field 53A not found"); return null; } else { return new Field53A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 53B, * ornull
if none is found.
* The first occurrence of field 53B at MT107 is expected to be the only one. * * @return a Field53B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field53B getField53B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("53B"); if (t == null) { log.fine("field 53B not found"); return null; } else { return new Field53B(t.getValue()); } } } /** * Iterates through block4 fields and return all occurrences of fields whose names matches 21, * orCollections.emptyList()
if none is found.
* Multiple occurrences of field 21 at MT107 are expected at one sequence or across several sequences. * * @return a List of Field21 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField21() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("21"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList()
* Multiple occurrences of field 23E at MT107 are expected at one sequence or across several sequences. * * @return a List of Field23E objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField23E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("23E"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 21C at MT107 are expected at one sequence or across several sequences. * * @return a List of Field21C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField21C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("21C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 21D at MT107 are expected at one sequence or across several sequences. * * @return a List of Field21D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField21D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("21D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 21E at MT107 are expected at one sequence or across several sequences. * * @return a List of Field21E objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField21E() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("21E"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 32B at MT107 are expected at one sequence or across several sequences. * * @return a List of Field32B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField32B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("32B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 50C at MT107 are expected at one sequence or across several sequences. * * @return a List of Field50C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField50C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("50C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 50L at MT107 are expected at one sequence or across several sequences. * * @return a List of Field50L objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField50L() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("50L"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 50A at MT107 are expected at one sequence or across several sequences. * * @return a List of Field50A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField50A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("50A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 50K at MT107 are expected at one sequence or across several sequences. * * @return a List of Field50K objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField50K() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("50K"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52A at MT107 are expected at one sequence or across several sequences. * * @return a List of Field52A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52C at MT107 are expected at one sequence or across several sequences. * * @return a List of Field52C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52D at MT107 are expected at one sequence or across several sequences. * * @return a List of Field52D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57A at MT107 are expected at one sequence or across several sequences. * * @return a List of Field57A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57C at MT107 are expected at one sequence or across several sequences. * * @return a List of Field57C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57D at MT107 are expected at one sequence or across several sequences. * * @return a List of Field57D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 59A at MT107 are expected at one sequence or across several sequences. * * @return a List of Field59A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField59A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("59A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 59 at MT107 are expected at one sequence or across several sequences. * * @return a List of Field59 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField59() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("59"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 70 at MT107 are expected at one sequence or across several sequences. * * @return a List of Field70 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField70() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("70"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 26T at MT107 are expected at one sequence or across several sequences. * * @return a List of Field26T objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField26T() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("26T"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 77B at MT107 are expected at one sequence or across several sequences. * * @return a List of Field77B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField77B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("77B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 33B at MT107 are expected at one sequence or across several sequences. * * @return a List of Field33B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField33B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("33B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 71A at MT107 are expected at one sequence or across several sequences. * * @return a List of Field71A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField71A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("71A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 71F at MT107 are expected at one sequence or across several sequences. * * @return a List of Field71F objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField71F() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("71F"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 71G at MT107 are expected at one sequence or across several sequences. * * @return a List of Field71G objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField71G() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("71G"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 36 at MT107 are expected at one sequence or across several sequences. * * @return a List of Field36 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField36() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("36"); final List result = new ArrayList (); for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy