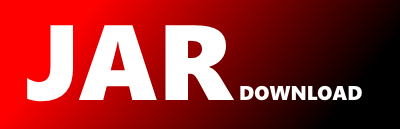
com.prowidesoftware.swift.model.mt.mt2xx.MT205COV Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/* * This library is free software; you can redistribute it and/or * modify it under the terms of the GNU Lesser General Public * License as published by the Free Software Foundation; either * version 2.1 of the License, or (at your option) any later version. * * This library is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * Lesser General Public License for more details. * */ package com.prowidesoftware.swift.model.mt.mt2xx; import java.io.Serializable; import java.util.ArrayList; import java.util.Collections; import java.util.List; import com.prowidesoftware.swift.model.*; import com.prowidesoftware.swift.model.field.*; import com.prowidesoftware.swift.model.mt.AbstractMT; /** * MT 205COV
if none is found.
* * * NOTE: this source code has been generated from template * * @author www.prowidesoftware.com */ public class MT205COV extends AbstractMT implements Serializable { private static final long serialVersionUID = 1L; private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT205COV.class.getName()); /** * Creates an MT205COV initialized with the parameter SwiftMessage * @param m swift message with the MT205COV content */ public MT205COV(SwiftMessage m) { super(m); } /** * Creates an MT205COV initialized with a new SwiftMessage */ public MT205COV() { super(); } /** * Returns this MT number * @return the message type number of this MT * @since 6.4 */ @Override public String getMessageType() { return "205"; } /** * Iterates through block4 fields and return the first one whose name matches 20, * ornull
if none is found.
* The first occurrence of field 20 at MT205COV is expected to be the only one. * * @return a Field20 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field20 getField20() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("20"); if (t == null) { log.fine("field 20 not found"); return null; } else { return new Field20(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 21, * ornull
if none is found.
* The first occurrence of field 21 at MT205COV is expected to be the only one. * * @return a Field21 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field21 getField21() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("21"); if (t == null) { log.fine("field 21 not found"); return null; } else { return new Field21(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 32A, * ornull
if none is found.
* The first occurrence of field 32A at MT205COV is expected to be the only one. * * @return a Field32A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field32A getField32A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("32A"); if (t == null) { log.fine("field 32A not found"); return null; } else { return new Field32A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 53A, * ornull
if none is found.
* The first occurrence of field 53A at MT205COV is expected to be the only one. * * @return a Field53A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field53A getField53A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("53A"); if (t == null) { log.fine("field 53A not found"); return null; } else { return new Field53A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 53B, * ornull
if none is found.
* The first occurrence of field 53B at MT205COV is expected to be the only one. * * @return a Field53B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field53B getField53B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("53B"); if (t == null) { log.fine("field 53B not found"); return null; } else { return new Field53B(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 53D, * ornull
if none is found.
* The first occurrence of field 53D at MT205COV is expected to be the only one. * * @return a Field53D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field53D getField53D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("53D"); if (t == null) { log.fine("field 53D not found"); return null; } else { return new Field53D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 58A, * ornull
if none is found.
* The first occurrence of field 58A at MT205COV is expected to be the only one. * * @return a Field58A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field58A getField58A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("58A"); if (t == null) { log.fine("field 58A not found"); return null; } else { return new Field58A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 58D, * ornull
if none is found.
* The first occurrence of field 58D at MT205COV is expected to be the only one. * * @return a Field58D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field58D getField58D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("58D"); if (t == null) { log.fine("field 58D not found"); return null; } else { return new Field58D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 50A, * ornull
if none is found.
* The first occurrence of field 50A at MT205COV is expected to be the only one. * * @return a Field50A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field50A getField50A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("50A"); if (t == null) { log.fine("field 50A not found"); return null; } else { return new Field50A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 50F, * ornull
if none is found.
* The first occurrence of field 50F at MT205COV is expected to be the only one. * * @return a Field50F object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field50F getField50F() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("50F"); if (t == null) { log.fine("field 50F not found"); return null; } else { return new Field50F(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 50K, * ornull
if none is found.
* The first occurrence of field 50K at MT205COV is expected to be the only one. * * @return a Field50K object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field50K getField50K() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("50K"); if (t == null) { log.fine("field 50K not found"); return null; } else { return new Field50K(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 56C, * ornull
if none is found.
* The first occurrence of field 56C at MT205COV is expected to be the only one. * * @return a Field56C object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field56C getField56C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("56C"); if (t == null) { log.fine("field 56C not found"); return null; } else { return new Field56C(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 57C, * ornull
if none is found.
* The first occurrence of field 57C at MT205COV is expected to be the only one. * * @return a Field57C object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field57C getField57C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("57C"); if (t == null) { log.fine("field 57C not found"); return null; } else { return new Field57C(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 59A, * ornull
if none is found.
* The first occurrence of field 59A at MT205COV is expected to be the only one. * * @return a Field59A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field59A getField59A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("59A"); if (t == null) { log.fine("field 59A not found"); return null; } else { return new Field59A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 59, * ornull
if none is found.
* The first occurrence of field 59 at MT205COV is expected to be the only one. * * @return a Field59 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field59 getField59() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("59"); if (t == null) { log.fine("field 59 not found"); return null; } else { return new Field59(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 70, * ornull
if none is found.
* The first occurrence of field 70 at MT205COV is expected to be the only one. * * @return a Field70 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field70 getField70() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("70"); if (t == null) { log.fine("field 70 not found"); return null; } else { return new Field70(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 33B, * ornull
if none is found.
* The first occurrence of field 33B at MT205COV is expected to be the only one. * * @return a Field33B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field33B getField33B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("33B"); if (t == null) { log.fine("field 33B not found"); return null; } else { return new Field33B(t.getValue()); } } } /** * Iterates through block4 fields and return all occurrences of fields whose names matches 13C, * orCollections.emptyList()
if none is found.
* Multiple occurrences of field 13C at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field13C objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField13C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("13C"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList()
* Multiple occurrences of field 52A at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field52A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52D at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field52D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 56A at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field56A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField56A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("56A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 56D at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field56D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField56D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("56D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57A at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field57A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57B at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field57B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57D at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field57D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 72 at MT205COV are expected at one sequence or across several sequences. * * @return a List of Field72 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField72() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("72"); final List result = new ArrayList (); for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy