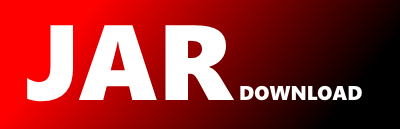
com.prowidesoftware.swift.model.mt.mt3xx.MT300 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/* * This library is free software; you can redistribute it and/or * modify it under the terms of the GNU Lesser General Public * License as published by the Free Software Foundation; either * version 2.1 of the License, or (at your option) any later version. * * This library is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * Lesser General Public License for more details. * */ package com.prowidesoftware.swift.model.mt.mt3xx; import java.io.Serializable; import java.util.ArrayList; import java.util.Collections; import java.util.List; import com.prowidesoftware.swift.model.*; import com.prowidesoftware.swift.model.field.*; import com.prowidesoftware.swift.model.mt.AbstractMT; /** * MT 300
if none is found.
* * * NOTE: this source code has been generated from template * * @author www.prowidesoftware.com */ public class MT300 extends AbstractMT implements Serializable { private static final long serialVersionUID = 1L; private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT300.class.getName()); /** * Creates an MT300 initialized with the parameter SwiftMessage * @param m swift message with the MT300 content */ public MT300(SwiftMessage m) { super(m); } /** * Creates an MT300 initialized with a new SwiftMessage */ public MT300() { super(); } /** * Returns this MT number * @return the message type number of this MT * @since 6.4 */ @Override public String getMessageType() { return "300"; } /** * Iterates through block4 fields and return the first one whose name matches 15A, * ornull
if none is found.
* The first occurrence of field 15A at MT300 is expected to be the only one. * * @return a Field15A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field15A getField15A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("15A"); if (t == null) { log.fine("field 15A not found"); return null; } else { return new Field15A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 20, * ornull
if none is found.
* The first occurrence of field 20 at MT300 is expected to be the only one. * * @return a Field20 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field20 getField20() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("20"); if (t == null) { log.fine("field 20 not found"); return null; } else { return new Field20(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 21, * ornull
if none is found.
* The first occurrence of field 21 at MT300 is expected to be the only one. * * @return a Field21 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field21 getField21() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("21"); if (t == null) { log.fine("field 21 not found"); return null; } else { return new Field21(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 22A, * ornull
if none is found.
* The first occurrence of field 22A at MT300 is expected to be the only one. * * @return a Field22A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field22A getField22A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("22A"); if (t == null) { log.fine("field 22A not found"); return null; } else { return new Field22A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 94A, * ornull
if none is found.
* The first occurrence of field 94A at MT300 is expected to be the only one. * * @return a Field94A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field94A getField94A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("94A"); if (t == null) { log.fine("field 94A not found"); return null; } else { return new Field94A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 22C, * ornull
if none is found.
* The first occurrence of field 22C at MT300 is expected to be the only one. * * @return a Field22C object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field22C getField22C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("22C"); if (t == null) { log.fine("field 22C not found"); return null; } else { return new Field22C(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 17T, * ornull
if none is found.
* The first occurrence of field 17T at MT300 is expected to be the only one. * * @return a Field17T object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field17T getField17T() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("17T"); if (t == null) { log.fine("field 17T not found"); return null; } else { return new Field17T(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 17U, * ornull
if none is found.
* The first occurrence of field 17U at MT300 is expected to be the only one. * * @return a Field17U object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field17U getField17U() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("17U"); if (t == null) { log.fine("field 17U not found"); return null; } else { return new Field17U(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 82A, * ornull
if none is found.
* The first occurrence of field 82A at MT300 is expected to be the only one. * * @return a Field82A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field82A getField82A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("82A"); if (t == null) { log.fine("field 82A not found"); return null; } else { return new Field82A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 82D, * ornull
if none is found.
* The first occurrence of field 82D at MT300 is expected to be the only one. * * @return a Field82D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field82D getField82D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("82D"); if (t == null) { log.fine("field 82D not found"); return null; } else { return new Field82D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 82J, * ornull
if none is found.
* The first occurrence of field 82J at MT300 is expected to be the only one. * * @return a Field82J object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field82J getField82J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("82J"); if (t == null) { log.fine("field 82J not found"); return null; } else { return new Field82J(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 87A, * ornull
if none is found.
* The first occurrence of field 87A at MT300 is expected to be the only one. * * @return a Field87A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field87A getField87A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("87A"); if (t == null) { log.fine("field 87A not found"); return null; } else { return new Field87A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 87D, * ornull
if none is found.
* The first occurrence of field 87D at MT300 is expected to be the only one. * * @return a Field87D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field87D getField87D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("87D"); if (t == null) { log.fine("field 87D not found"); return null; } else { return new Field87D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 87J, * ornull
if none is found.
* The first occurrence of field 87J at MT300 is expected to be the only one. * * @return a Field87J object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field87J getField87J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("87J"); if (t == null) { log.fine("field 87J not found"); return null; } else { return new Field87J(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 83A, * ornull
if none is found.
* The first occurrence of field 83A at MT300 is expected to be the only one. * * @return a Field83A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field83A getField83A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("83A"); if (t == null) { log.fine("field 83A not found"); return null; } else { return new Field83A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 83D, * ornull
if none is found.
* The first occurrence of field 83D at MT300 is expected to be the only one. * * @return a Field83D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field83D getField83D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("83D"); if (t == null) { log.fine("field 83D not found"); return null; } else { return new Field83D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 83J, * ornull
if none is found.
* The first occurrence of field 83J at MT300 is expected to be the only one. * * @return a Field83J object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field83J getField83J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("83J"); if (t == null) { log.fine("field 83J not found"); return null; } else { return new Field83J(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 77H, * ornull
if none is found.
* The first occurrence of field 77H at MT300 is expected to be the only one. * * @return a Field77H object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field77H getField77H() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("77H"); if (t == null) { log.fine("field 77H not found"); return null; } else { return new Field77H(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 77D, * ornull
if none is found.
* The first occurrence of field 77D at MT300 is expected to be the only one. * * @return a Field77D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field77D getField77D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("77D"); if (t == null) { log.fine("field 77D not found"); return null; } else { return new Field77D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 14C, * ornull
if none is found.
* The first occurrence of field 14C at MT300 is expected to be the only one. * * @return a Field14C object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field14C getField14C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("14C"); if (t == null) { log.fine("field 14C not found"); return null; } else { return new Field14C(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 15B, * ornull
if none is found.
* The first occurrence of field 15B at MT300 is expected to be the only one. * * @return a Field15B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field15B getField15B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("15B"); if (t == null) { log.fine("field 15B not found"); return null; } else { return new Field15B(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 30T, * ornull
if none is found.
* The first occurrence of field 30T at MT300 is expected to be the only one. * * @return a Field30T object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field30T getField30T() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("30T"); if (t == null) { log.fine("field 30T not found"); return null; } else { return new Field30T(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 30V, * ornull
if none is found.
* The first occurrence of field 30V at MT300 is expected to be the only one. * * @return a Field30V object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field30V getField30V() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("30V"); if (t == null) { log.fine("field 30V not found"); return null; } else { return new Field30V(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 36, * ornull
if none is found.
* The first occurrence of field 36 at MT300 is expected to be the only one. * * @return a Field36 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field36 getField36() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("36"); if (t == null) { log.fine("field 36 not found"); return null; } else { return new Field36(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 33B, * ornull
if none is found.
* The first occurrence of field 33B at MT300 is expected to be the only one. * * @return a Field33B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field33B getField33B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("33B"); if (t == null) { log.fine("field 33B not found"); return null; } else { return new Field33B(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 15C, * ornull
if none is found.
* The first occurrence of field 15C at MT300 is expected to be the only one. * * @return a Field15C object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field15C getField15C() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("15C"); if (t == null) { log.fine("field 15C not found"); return null; } else { return new Field15C(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 29A, * ornull
if none is found.
* The first occurrence of field 29A at MT300 is expected to be the only one. * * @return a Field29A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field29A getField29A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("29A"); if (t == null) { log.fine("field 29A not found"); return null; } else { return new Field29A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 24D, * ornull
if none is found.
* The first occurrence of field 24D at MT300 is expected to be the only one. * * @return a Field24D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field24D getField24D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("24D"); if (t == null) { log.fine("field 24D not found"); return null; } else { return new Field24D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 84A, * ornull
if none is found.
* The first occurrence of field 84A at MT300 is expected to be the only one. * * @return a Field84A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field84A getField84A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("84A"); if (t == null) { log.fine("field 84A not found"); return null; } else { return new Field84A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 84B, * ornull
if none is found.
* The first occurrence of field 84B at MT300 is expected to be the only one. * * @return a Field84B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field84B getField84B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("84B"); if (t == null) { log.fine("field 84B not found"); return null; } else { return new Field84B(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 84D, * ornull
if none is found.
* The first occurrence of field 84D at MT300 is expected to be the only one. * * @return a Field84D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field84D getField84D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("84D"); if (t == null) { log.fine("field 84D not found"); return null; } else { return new Field84D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 84J, * ornull
if none is found.
* The first occurrence of field 84J at MT300 is expected to be the only one. * * @return a Field84J object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field84J getField84J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("84J"); if (t == null) { log.fine("field 84J not found"); return null; } else { return new Field84J(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 85A, * ornull
if none is found.
* The first occurrence of field 85A at MT300 is expected to be the only one. * * @return a Field85A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field85A getField85A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("85A"); if (t == null) { log.fine("field 85A not found"); return null; } else { return new Field85A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 85B, * ornull
if none is found.
* The first occurrence of field 85B at MT300 is expected to be the only one. * * @return a Field85B object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field85B getField85B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("85B"); if (t == null) { log.fine("field 85B not found"); return null; } else { return new Field85B(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 85D, * ornull
if none is found.
* The first occurrence of field 85D at MT300 is expected to be the only one. * * @return a Field85D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field85D getField85D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("85D"); if (t == null) { log.fine("field 85D not found"); return null; } else { return new Field85D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 85J, * ornull
if none is found.
* The first occurrence of field 85J at MT300 is expected to be the only one. * * @return a Field85J object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field85J getField85J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("85J"); if (t == null) { log.fine("field 85J not found"); return null; } else { return new Field85J(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 88A, * ornull
if none is found.
* The first occurrence of field 88A at MT300 is expected to be the only one. * * @return a Field88A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field88A getField88A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("88A"); if (t == null) { log.fine("field 88A not found"); return null; } else { return new Field88A(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 88D, * ornull
if none is found.
* The first occurrence of field 88D at MT300 is expected to be the only one. * * @return a Field88D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field88D getField88D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("88D"); if (t == null) { log.fine("field 88D not found"); return null; } else { return new Field88D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 88J, * ornull
if none is found.
* The first occurrence of field 88J at MT300 is expected to be the only one. * * @return a Field88J object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field88J getField88J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("88J"); if (t == null) { log.fine("field 88J not found"); return null; } else { return new Field88J(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 71F, * ornull
if none is found.
* The first occurrence of field 71F at MT300 is expected to be the only one. * * @return a Field71F object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field71F getField71F() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("71F"); if (t == null) { log.fine("field 71F not found"); return null; } else { return new Field71F(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 26H, * ornull
if none is found.
* The first occurrence of field 26H at MT300 is expected to be the only one. * * @return a Field26H object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field26H getField26H() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("26H"); if (t == null) { log.fine("field 26H not found"); return null; } else { return new Field26H(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 21G, * ornull
if none is found.
* The first occurrence of field 21G at MT300 is expected to be the only one. * * @return a Field21G object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field21G getField21G() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("21G"); if (t == null) { log.fine("field 21G not found"); return null; } else { return new Field21G(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 72, * ornull
if none is found.
* The first occurrence of field 72 at MT300 is expected to be the only one. * * @return a Field72 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field72 getField72() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("72"); if (t == null) { log.fine("field 72 not found"); return null; } else { return new Field72(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 15D, * ornull
if none is found.
* The first occurrence of field 15D at MT300 is expected to be the only one. * * @return a Field15D object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field15D getField15D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("15D"); if (t == null) { log.fine("field 15D not found"); return null; } else { return new Field15D(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 16A, * ornull
if none is found.
* The first occurrence of field 16A at MT300 is expected to be the only one. * * @return a Field16A object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field16A getField16A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("16A"); if (t == null) { log.fine("field 16A not found"); return null; } else { return new Field16A(t.getValue()); } } } /** * Iterates through block4 fields and return all occurrences of fields whose names matches 53A, * orCollections.emptyList()
if none is found.
* Multiple occurrences of field 53A at MT300 are expected at one sequence or across several sequences. * * @return a List of Field53A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField53A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("53A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList()
* Multiple occurrences of field 53D at MT300 are expected at one sequence or across several sequences. * * @return a List of Field53D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField53D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("53D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 53J at MT300 are expected at one sequence or across several sequences. * * @return a List of Field53J objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField53J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("53J"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 56A at MT300 are expected at one sequence or across several sequences. * * @return a List of Field56A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField56A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("56A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 56D at MT300 are expected at one sequence or across several sequences. * * @return a List of Field56D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField56D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("56D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 56J at MT300 are expected at one sequence or across several sequences. * * @return a List of Field56J objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField56J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("56J"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57A at MT300 are expected at one sequence or across several sequences. * * @return a List of Field57A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57D at MT300 are expected at one sequence or across several sequences. * * @return a List of Field57D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 57J at MT300 are expected at one sequence or across several sequences. * * @return a List of Field57J objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField57J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("57J"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 17A at MT300 are expected at one sequence or across several sequences. * * @return a List of Field17A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField17A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("17A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 32B at MT300 are expected at one sequence or across several sequences. * * @return a List of Field32B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField32B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("32B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 58A at MT300 are expected at one sequence or across several sequences. * * @return a List of Field58A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField58A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("58A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 58D at MT300 are expected at one sequence or across several sequences. * * @return a List of Field58D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField58D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("58D"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 58J at MT300 are expected at one sequence or across several sequences. * * @return a List of Field58J objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField58J() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("58J"); final List result = new ArrayList (); for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy