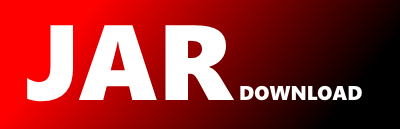
com.prowidesoftware.swift.model.mt.mt4xx.MT450 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/* * This library is free software; you can redistribute it and/or * modify it under the terms of the GNU Lesser General Public * License as published by the Free Software Foundation; either * version 2.1 of the License, or (at your option) any later version. * * This library is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * Lesser General Public License for more details. * */ package com.prowidesoftware.swift.model.mt.mt4xx; import java.io.Serializable; import java.util.ArrayList; import java.util.Collections; import java.util.List; import com.prowidesoftware.swift.model.*; import com.prowidesoftware.swift.model.field.*; import com.prowidesoftware.swift.model.mt.AbstractMT; /** * MT 450
if none is found.
* * * NOTE: this source code has been generated from template * * @author www.prowidesoftware.com */ public class MT450 extends AbstractMT implements Serializable { private static final long serialVersionUID = 1L; private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT450.class.getName()); /** * Creates an MT450 initialized with the parameter SwiftMessage * @param m swift message with the MT450 content */ public MT450(SwiftMessage m) { super(m); } /** * Creates an MT450 initialized with a new SwiftMessage */ public MT450() { super(); } /** * Returns this MT number * @return the message type number of this MT * @since 6.4 */ @Override public String getMessageType() { return "450"; } /** * Iterates through block4 fields and return the first one whose name matches 25, * ornull
if none is found.
* The first occurrence of field 25 at MT450 is expected to be the only one. * * @return a Field25 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field25 getField25() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("25"); if (t == null) { log.fine("field 25 not found"); return null; } else { return new Field25(t.getValue()); } } } /** * Iterates through block4 fields and return the first one whose name matches 72, * ornull
if none is found.
* The first occurrence of field 72 at MT450 is expected to be the only one. * * @return a Field72 object ornull
if the field is not found * @see SwiftTagListBlock#getTagByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public Field72 getField72() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return null; } else { final Tag t = getSwiftMessage().getBlock4().getTagByName("72"); if (t == null) { log.fine("field 72 not found"); return null; } else { return new Field72(t.getValue()); } } } /** * Iterates through block4 fields and return all occurrences of fields whose names matches 20, * orCollections.emptyList()
if none is found.
* Multiple occurrences of field 20 at MT450 are expected at one sequence or across several sequences. * * @return a List of Field20 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField20() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("20"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList()
* Multiple occurrences of field 21 at MT450 are expected at one sequence or across several sequences. * * @return a List of Field21 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField21() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("21"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 30 at MT450 are expected at one sequence or across several sequences. * * @return a List of Field30 objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField30() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("30"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 32A at MT450 are expected at one sequence or across several sequences. * * @return a List of Field32A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField32A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("32A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52A at MT450 are expected at one sequence or across several sequences. * * @return a List of Field52A objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52A() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52A"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52B at MT450 are expected at one sequence or across several sequences. * * @return a List of Field52B objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52B() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52B"); final List result = new ArrayList (); for (int i=0; i Collections.emptyList() if none is found.
* Multiple occurrences of field 52D at MT450 are expected at one sequence or across several sequences. * * @return a List of Field52D objects orCollections.emptyList()
if none is not found * @see SwiftTagListBlock#getTagsByName(String) * @throws IllegalStateException if SwiftMessage object is not initialized */ public ListgetField52D() { if (getSwiftMessage() == null) { throw new IllegalStateException("SwiftMessage was not initialized"); } if (getSwiftMessage().getBlock4() == null) { log.info("block4 is null"); return Collections.emptyList(); } else { final Tag[] tags = getSwiftMessage().getBlock4().getTagsByName("52D"); final List result = new ArrayList (); for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy