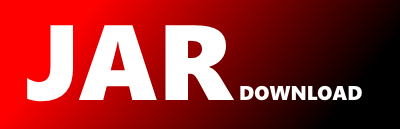
com.prowidesoftware.swift.model.mt.mt4xx.MT455 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wife Show documentation
Show all versions of wife Show documentation
Prowide Core Libraries for SWIFT (TM) messages
The newest version!
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
*/
package com.prowidesoftware.swift.model.mt.mt4xx;
import java.io.Serializable;
import com.prowidesoftware.swift.model.*;
import com.prowidesoftware.swift.model.field.*;
import com.prowidesoftware.swift.model.mt.AbstractMT;
/**
* MT 455
*
*
* NOTE: this source code has been generated from template
*
* @author www.prowidesoftware.com
*/
public class MT455 extends AbstractMT implements Serializable {
private static final long serialVersionUID = 1L;
private static transient final java.util.logging.Logger log = java.util.logging.Logger.getLogger(MT455.class.getName());
/**
* Creates an MT455 initialized with the parameter SwiftMessage
* @param m swift message with the MT455 content
*/
public MT455(SwiftMessage m) {
super(m);
}
/**
* Creates an MT455 initialized with a new SwiftMessage
*/
public MT455() {
super();
}
/**
* Returns this MT number
* @return the message type number of this MT
* @since 6.4
*/
@Override
public String getMessageType() {
return "455";
}
/**
* Iterates through block4 fields and return the first one whose name matches 20,
* or null
if none is found.
* The first occurrence of field 20 at MT455 is expected to be the only one.
*
* @return a Field20 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field20 getField20() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("20");
if (t == null) {
log.fine("field 20 not found");
return null;
} else {
return new Field20(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 21,
* or null
if none is found.
* The first occurrence of field 21 at MT455 is expected to be the only one.
*
* @return a Field21 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field21 getField21() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("21");
if (t == null) {
log.fine("field 21 not found");
return null;
} else {
return new Field21(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 25,
* or null
if none is found.
* The first occurrence of field 25 at MT455 is expected to be the only one.
*
* @return a Field25 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field25 getField25() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("25");
if (t == null) {
log.fine("field 25 not found");
return null;
} else {
return new Field25(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 30,
* or null
if none is found.
* The first occurrence of field 30 at MT455 is expected to be the only one.
*
* @return a Field30 object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field30 getField30() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("30");
if (t == null) {
log.fine("field 30 not found");
return null;
} else {
return new Field30(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 32A,
* or null
if none is found.
* The first occurrence of field 32A at MT455 is expected to be the only one.
*
* @return a Field32A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field32A getField32A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("32A");
if (t == null) {
log.fine("field 32A not found");
return null;
} else {
return new Field32A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 33C,
* or null
if none is found.
* The first occurrence of field 33C at MT455 is expected to be the only one.
*
* @return a Field33C object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field33C getField33C() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("33C");
if (t == null) {
log.fine("field 33C not found");
return null;
} else {
return new Field33C(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 33D,
* or null
if none is found.
* The first occurrence of field 33D at MT455 is expected to be the only one.
*
* @return a Field33D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field33D getField33D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("33D");
if (t == null) {
log.fine("field 33D not found");
return null;
} else {
return new Field33D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 52A,
* or null
if none is found.
* The first occurrence of field 52A at MT455 is expected to be the only one.
*
* @return a Field52A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field52A getField52A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("52A");
if (t == null) {
log.fine("field 52A not found");
return null;
} else {
return new Field52A(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 52B,
* or null
if none is found.
* The first occurrence of field 52B at MT455 is expected to be the only one.
*
* @return a Field52B object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field52B getField52B() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("52B");
if (t == null) {
log.fine("field 52B not found");
return null;
} else {
return new Field52B(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 52D,
* or null
if none is found.
* The first occurrence of field 52D at MT455 is expected to be the only one.
*
* @return a Field52D object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field52D getField52D() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("52D");
if (t == null) {
log.fine("field 52D not found");
return null;
} else {
return new Field52D(t.getValue());
}
}
}
/**
* Iterates through block4 fields and return the first one whose name matches 77A,
* or null
if none is found.
* The first occurrence of field 77A at MT455 is expected to be the only one.
*
* @return a Field77A object or null
if the field is not found
* @see SwiftTagListBlock#getTagByName(String)
* @throws IllegalStateException if SwiftMessage object is not initialized
*/
public Field77A getField77A() {
if (getSwiftMessage() == null) {
throw new IllegalStateException("SwiftMessage was not initialized");
}
if (getSwiftMessage().getBlock4() == null) {
log.info("block4 is null");
return null;
} else {
final Tag t = getSwiftMessage().getBlock4().getTagByName("77A");
if (t == null) {
log.fine("field 77A not found");
return null;
} else {
return new Field77A(t.getValue());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy